Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / ReturnType.cs / 1305376 / ReturnType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using Som = System.Data.EntityModel.SchemaObjectModel; using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Data; using System.IO; using System.Data.Metadata.Edm; using System.Data.Entity; using System.Text; namespace System.Data.EntityModel.SchemaObjectModel { class ReturnType : ModelFunctionTypeElement { private CollectionKind _collectionKind = CollectionKind.None; bool _isRefType; private ModelFunctionTypeElement _typeSubElement = null; #region constructor ////// /// /// internal ReturnType(Function parentElement) : base(parentElement) { _typeUsageBuilder = new TypeUsageBuilder(this); } #endregion #region Properties internal CollectionKind CollectionKind { get { return _collectionKind; } set { _collectionKind = value; } } internal override TypeUsage TypeUsage { get { if (_typeSubElement != null) { return _typeSubElement.GetTypeUsage(); } else if (_typeUsage != null) { return _typeUsage; } else if (base.TypeUsage == null) { return null; } else if (CollectionKind != CollectionKind.None) { return TypeUsage.Create(new CollectionType(base.TypeUsage)); } else { return base.TypeUsage; } } } #endregion internal override SchemaElement Clone(SchemaElement parentElement) { ReturnType parameter = new ReturnType((Function)parentElement); parameter._type = _type; parameter.Name = this.Name; parameter._typeUsageBuilder = this._typeUsageBuilder; parameter._unresolvedType = this._unresolvedType; return parameter; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.TypeElement)) { HandleTypeAttribute(reader); return true; } else if (_typeUsageBuilder.HandleAttribute(reader)) { return true; } return false; } internal bool ResolveNestedTypeNames(Converter.ConversionCache convertedItemCache, DictionarynewGlobalItems) { if (_typeSubElement != null) { return _typeSubElement.ResolveNameAndSetTypeUsage(convertedItemCache, newGlobalItems); } else if (_type != null) { if (_type is ScalarType) //Create and store type usage for scalar type { _typeUsageBuilder.ValidateAndSetTypeUsage(_type as ScalarType, false); _typeUsage = _typeUsageBuilder.TypeUsage; } else //Try to resolve edm type. If not now, it will resolve in the second pass { EdmType edmType = (EdmType)Converter.LoadSchemaElement(_type, _type.Schema.ProviderManifest, convertedItemCache, newGlobalItems); if (edmType != null) { if (_isRefType) { EntityType entityType = edmType as EntityType; Debug.Assert(entityType != null); _typeUsage = TypeUsage.Create(new RefType(entityType)); } else { _typeUsageBuilder.ValidateAndSetTypeUsage(edmType, false); //use typeusagebuilder so dont lose facet information _typeUsage = _typeUsageBuilder.TypeUsage; } } } if (_collectionKind != CollectionKind.None) { _typeUsage = TypeUsage.Create(new CollectionType(_typeUsage)); } return _typeUsage != null; } return true; } #region Private Methods /// /// /// /// private void HandleTypeAttribute(XmlReader reader) { Debug.Assert(reader != null); Debug.Assert(UnresolvedType == null); string type; if (!Utils.GetString(Schema, reader, out type)) return; TypeModifier typeModifier; Function.RemoveTypeModifier(ref type, out typeModifier, out _isRefType); switch (typeModifier) { case TypeModifier.Array: CollectionKind = CollectionKind.Bag; break; default: Debug.Assert(typeModifier == TypeModifier.None, string.Format(CultureInfo.CurrentCulture, "Type is not valid for property {0}: {1}. The modifier for the type cannot be used in this context.", FQName, reader.Value)); break; } if (!Utils.ValidateDottedName(Schema, reader, type)) return; UnresolvedType = type; } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.CollectionType)) { HandleCollectionTypeElement(reader); return true; } else if (CanHandleElement(reader, XmlConstants.ReferenceType)) { HandleReferenceTypeElement(reader); return true; } else if (CanHandleElement(reader, XmlConstants.TypeRef)) { HandleTypeRefElement(reader); return true; } else if (CanHandleElement(reader, XmlConstants.RowType)) { HandleRowTypeElement(reader); return true; } return false; } protected void HandleCollectionTypeElement(XmlReader reader) { Debug.Assert(reader != null); var subElement = new CollectionTypeElement(this); subElement.Parse(reader); _typeSubElement = subElement; } protected void HandleReferenceTypeElement(XmlReader reader) { Debug.Assert(reader != null); var subElement = new ReferenceTypeElement(this); subElement.Parse(reader); _typeSubElement = subElement; } protected void HandleTypeRefElement(XmlReader reader) { Debug.Assert(reader != null); var subElement = new TypeRefElement(this); subElement.Parse(reader); _typeSubElement = subElement; } protected void HandleRowTypeElement(XmlReader reader) { Debug.Assert(reader != null); var subElement = new RowTypeElement(this); subElement.Parse(reader); _typeSubElement = subElement; } #endregion internal override void ResolveTopLevelNames() { Debug.Assert(!this.ParentElement.IsFunctionImport, "FunctionImports have return type as an attribute, so we should NEVER see them here"); if (Schema.DataModel == SchemaDataModelOption.EntityDataModel) { if (_unresolvedType != null) { base.ResolveTopLevelNames(); } if(_typeSubElement != null) { _typeSubElement.ResolveTopLevelNames(); } } else { base.ResolveTopLevelNames(); if (Schema.ResolveTypeName(this, UnresolvedType, out _type)) { if (!(_type is ScalarType)) { Debug.Assert(Schema.DataModel == SchemaDataModelOption.ProviderManifestModel, "Only Provider manifest has the return type element"); AddError(ErrorCode.FunctionWithNonEdmTypeNotSupported, EdmSchemaErrorSeverity.Error, this, System.Data.Entity.Strings.FunctionWithNonEdmTypeNotSupported(_type.FQName, this.FQName)); } } } } internal void ValidateForModelFunction() { if (_type != null && _type is ScalarType == false && _typeUsageBuilder.HasUserDefinedFacets) { //Non-scalar return type should not have Facets AddError(ErrorCode.ModelFuncionFacetOnNonScalarType, EdmSchemaErrorSeverity.Error, Strings.FacetsOnNonScalarType(_type.FQName)); } if (_type == null && _typeUsageBuilder.HasUserDefinedFacets) { //Type attribute not specified but facets exist AddError(ErrorCode.ModelFunctionIncorrectlyPlacedFacet, EdmSchemaErrorSeverity.Error, Strings.FacetDeclarationRequiresTypeAttribute); } if (_type == null && _typeSubElement == null) { //Return type not declared as either attribute or subelement AddError(ErrorCode.ModelFunctionTypeNotDeclared, EdmSchemaErrorSeverity.Error, Strings.TypeMustBeDeclared); } if (_typeSubElement != null) { _typeSubElement.Validate(); } } internal override void WriteIdentity(StringBuilder builder) { } internal override TypeUsage GetTypeUsage() { return TypeUsage; } internal override bool ResolveNameAndSetTypeUsage(Converter.ConversionCache convertedItemCache, DictionarynewGlobalItems) { return ResolveNestedTypeNames(convertedItemCache, newGlobalItems); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
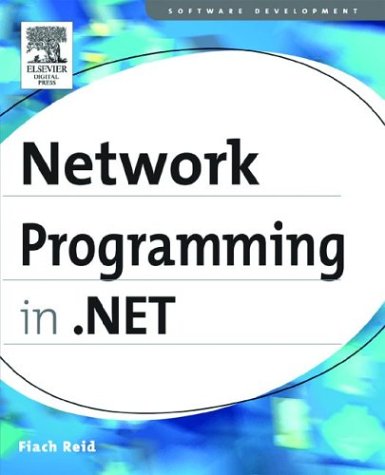
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DiagnosticStrings.cs
- PropertyDescriptor.cs
- TextRangeEdit.cs
- SegmentTree.cs
- Margins.cs
- NumericUpDownAcceleration.cs
- StringFormat.cs
- DataGridViewDataConnection.cs
- ListViewDesigner.cs
- regiisutil.cs
- GatewayIPAddressInformationCollection.cs
- PropertyGridCommands.cs
- ComplexTypeEmitter.cs
- ProfileSection.cs
- SecurityKeyUsage.cs
- XmlILTrace.cs
- OutputCacheSettingsSection.cs
- BitmapEffectRenderDataResource.cs
- OutputCacheSettings.cs
- TreeNodeStyle.cs
- EntitySetBase.cs
- tabpagecollectioneditor.cs
- DispatcherHookEventArgs.cs
- BitmapCache.cs
- ScrollItemPattern.cs
- EmptyControlCollection.cs
- CryptoApi.cs
- EncodingTable.cs
- DataContractSerializerSection.cs
- CellRelation.cs
- PagesSection.cs
- ConfigurationValues.cs
- OdbcStatementHandle.cs
- RowTypeElement.cs
- Identity.cs
- CodeNamespace.cs
- InkCanvasInnerCanvas.cs
- ComEventsMethod.cs
- HtmlTableRowCollection.cs
- ResourceSet.cs
- WebSysDisplayNameAttribute.cs
- CodeNamespaceImport.cs
- ListViewInsertEventArgs.cs
- SynchronizationLockException.cs
- XmlSchemaType.cs
- ObjectManager.cs
- ListViewItem.cs
- GeneratedContractType.cs
- TreeViewEvent.cs
- ToolStripDropTargetManager.cs
- DecoratedNameAttribute.cs
- DllNotFoundException.cs
- Signature.cs
- TreeViewItem.cs
- DesignBinding.cs
- WebBrowserProgressChangedEventHandler.cs
- QueryHandler.cs
- localization.cs
- RemotingServices.cs
- MenuStrip.cs
- PathSegmentCollection.cs
- CharEntityEncoderFallback.cs
- SystemResourceKey.cs
- DataGridViewColumnTypePicker.cs
- MetafileHeaderEmf.cs
- MetadataFile.cs
- Context.cs
- NetDataContractSerializer.cs
- BasicBrowserDialog.cs
- QueryCacheEntry.cs
- CollectionAdapters.cs
- SqlDataAdapter.cs
- MemberPath.cs
- ObjectComplexPropertyMapping.cs
- ServiceAuthorizationManager.cs
- QueryNode.cs
- TypefaceCollection.cs
- wmiutil.cs
- COAUTHIDENTITY.cs
- XmlNamespaceDeclarationsAttribute.cs
- DataChangedEventManager.cs
- PatternMatchRules.cs
- ImageMap.cs
- CustomAttributeFormatException.cs
- ClientSponsor.cs
- ServicesExceptionNotHandledEventArgs.cs
- NativeMethods.cs
- ReadOnlyState.cs
- GroupItem.cs
- ToolTip.cs
- OuterGlowBitmapEffect.cs
- TextRunProperties.cs
- PeerInvitationResponse.cs
- PatternMatcher.cs
- CompilerWrapper.cs
- TextServicesCompartmentEventSink.cs
- HttpBrowserCapabilitiesBase.cs
- SqlServer2KCompatibilityAnnotation.cs
- DocumentXPathNavigator.cs
- GorillaCodec.cs