Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / BuildTasks / MS / Internal / Tasks / CompilerWrapper.cs / 1 / CompilerWrapper.cs
//---------------------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // A wrapper class which can take inputs from build task and call the underneath // MarkupCompiler, CompilationUnit and other related types to do the real // compilation work. // This wrapper runs in the same Appdomain as MarkupCompiler, CompilationUnit, // // It miminizes the communication between two domains among the build tasks and // the real compiler classes. // // History: // // 10/26/05: weibz Created // //--------------------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Reflection; using System.Runtime.InteropServices; using MS.Internal.Markup; using Microsoft.Build.Utilities; using Microsoft.Build.Framework; using MS.Internal.Tasks; using MS.Utility; namespace MS.Internal { //// CompilerWrapper // internal class CompilerWrapper : MarshalByRefObject { //// ctor // internal CompilerWrapper() { _mc = new MarkupCompiler(); _sourceDir = Directory.GetCurrentDirectory() + "\\"; _nErrors = 0; } //// The valid source file extension for the passed language. // Normally a language supports more valid source file extensions. // User could choose one of them in project file. // If this property is not set, we will take use of the default one for the language. // internal string LanguageSourceExtension { set { _mc.LanguageSourceExtension = value; } } //// OutputPath : Generated code files, Baml fles will be put in this directory. // internal string OutputPath { set { _mc.TargetPath = value; } } //// The version of the assembly // internal string AssemblyVersion { set { _mc.AssemblyVersion = value; } } //// The key token of the assembly // internal string AssemblyPublicKeyToken { set { _mc.AssemblyPublicKeyToken = value; } } //// ApplicationMarkup // internal string ApplicationMarkup { set { _applicationMarkup = value; } } //// Loose file content list // internal string[] ContentFiles { set { _mc.ContentList = value; } } ////// Splash screen image to be displayed before application init /// internal string SplashImage { set { _mc.SplashImage = value; } } //// Assembly References. // //internal ArrayList References { set { _mc.ReferenceAssemblyList = value; } } // //If true code for supporting hosting in Browser is generated // internal bool HostInBrowser { set { _mc.HostInBrowser = value; } } //// Generated debugging information in the BAML file. // internal bool XamlDebuggingInformation { set { _mc.XamlDebuggingInformation = value; } } //// Controls how to generate localization information for each xaml file. // Valid values: None, CommentsOnly, All. // // internal int LocalizationDirectivesToLocFile { set { _localizationDirectivesToLocFile = value; } } //// Keep the application definition file if it contains local types which are implemented // in current target assembly. // internal string LocalXamlApplication { get { return _mc.LocalXamlApplication; } } //// Keep the page markup files if they contain local types which are implemented // in current target assembly. // internal string[] LocalXamlPages { get { return _mc.LocalXamlPages; } } ////// Get/Sets the TaskFileService on MarkupCompiler /// internal ITaskFileService TaskFileService { get { return _mc.TaskFileService; } set { _mc.TaskFileService = value; } } // // A wrapper property which maps to MarkupCompiler class's corresponding property. // This property will be called by MarkupCompilePass1 and Pass2 build tasks. // // It indicates whether any xaml file in current xaml list references internal types // from friend assembly or local target assembly. // internal bool HasInternals { get { return MarkupCompiler.HasInternals; } } //// TaskLoggingHelper // internal TaskLoggingHelper TaskLogger { set { _taskLogger = value; } } internal string UnknownErrorID { set { _unknownErrorID = value; } } internal int ErrorTimes { get { return _nErrors; } } //// Start the compilation. // // // // // // //internal bool DoCompilation(string assemblyName, string language, string rootNamespace, string[] fileList, bool isSecondPass) { bool ret = true; CompilationUnit compUnit = new CompilationUnit(assemblyName, language, rootNamespace, fileList); compUnit.Pass2 = isSecondPass; // Set some properties required by the CompilationUnit compUnit.ApplicationFile = _applicationMarkup; compUnit.SourcePath = _sourceDir; //Set the properties required by MarkupCompiler _mc.SourceFileResolve += new SourceFileResolveEventHandler(OnSourceFileResolve); _mc.Error += new MarkupErrorEventHandler(OnCompilerError); LocalizationDirectivesToLocFile localizeFlag = (LocalizationDirectivesToLocFile)_localizationDirectivesToLocFile; // // Localization file should not be generated for Intellisense build. Thus // checking IsRealBuild. // if ((localizeFlag == MS.Internal.LocalizationDirectivesToLocFile.All || localizeFlag == MS.Internal.LocalizationDirectivesToLocFile.CommentsOnly) && (TaskFileService.IsRealBuild)) { _mc.ParserHooks = new LocalizationParserHooks(_mc, localizeFlag, isSecondPass); } if (isSecondPass) { for (int i = 0; i < _mc.ReferenceAssemblyList.Count; i++) { ReferenceAssembly asmReference = _mc.ReferenceAssemblyList[i] as ReferenceAssembly; if (asmReference != null) { if (String.Compare(asmReference.AssemblyName, assemblyName, StringComparison.OrdinalIgnoreCase) == 0) { // Set the local assembly file to markupCompiler _mc.LocalAssemblyFile = asmReference; } } } } // finally compile the app _mc.Compile(compUnit); return ret; } #region private method // // Event hanlder for the Compiler Errors // // sd brancx // private void OnCompilerError(Object sender, MarkupErrorEventArgs e) { _nErrors++; // // Since Output from LogError() cannot be recognized by VS TaskList, so // we replaced it with LogErrorFromText( ) and pass all the required information // such as filename, line, offset, etc. // string strErrorCode; // Generate error message by going through the whole exception chain, including // its inner exceptions. string message = TaskHelper.GetWholeExceptionMessage(e.Exception); string errorText; strErrorCode = _taskLogger.ExtractMessageCode(message, out errorText); if (String.IsNullOrEmpty(strErrorCode)) { // If the exception is a Xml exception, show a pre-asigned error id for it. if (IsXmlException(e.Exception)) { message = SR.Get(SRID.InvalidXml, message); strErrorCode = _taskLogger.ExtractMessageCode(message, out errorText); } else { strErrorCode = _unknownErrorID; errorText = SR.Get(SRID.UnknownBuildError, errorText); } } _taskLogger.LogError(null, strErrorCode, null, e.FileName, e.LineNumber, e.LinePosition, 0, 0, errorText); } // // Detect if the exception is xmlException // private bool IsXmlException(Exception e) { bool isXmlException = false; while (e != null) { if (e is System.Xml.XmlException) { isXmlException = true; break; } else { e = e.InnerException; } } return isXmlException; } // // Handle the SourceFileResolve Event from MarkupCompiler. // It tries to GetResolvedFilePath for the new SourceDir and new RelativePath. // private void OnSourceFileResolve(Object sender, SourceFileResolveEventArgs e) { SourceFileInfo sourceFileInfo = e.SourceFileInfo; string newSourceDir = _sourceDir; string newRelativeFilePath; if (String.IsNullOrEmpty(sourceFileInfo.OriginalFilePath)) { newRelativeFilePath = sourceFileInfo.OriginalFilePath; } else { newRelativeFilePath = GetResolvedFilePath(sourceFileInfo.OriginalFilePath, ref newSourceDir); _taskLogger.LogMessageFromResources(MessageImportance.Low, SRID.FileResolved, sourceFileInfo.OriginalFilePath, newRelativeFilePath, newSourceDir); } if (sourceFileInfo.IsXamlFile) { // // For Xaml Source file, we need to remove the .xaml extension part. // int fileExtIndex = newRelativeFilePath.LastIndexOf(MarkupCompiler.DOT, StringComparison.Ordinal); newRelativeFilePath = newRelativeFilePath.Substring(0, fileExtIndex); } // // Update the SourcePath and RelativeSourceFilePath property in SourceFileInfo object. // sourceFileInfo.SourcePath = newSourceDir; sourceFileInfo.RelativeSourceFilePath = newRelativeFilePath; // Put the stream here. sourceFileInfo.Stream = TaskFileService.GetContent(sourceFileInfo.OriginalFilePath); } // // Return a new sourceDir and relative filepath for a given filePath. // This is for supporting of fullpath or ..\ in the original FilePath. // private string GetResolvedFilePath(string filePath, ref string newSourceDir) { // make it an absolute path if not already so if (!Path.IsPathRooted(filePath)) { filePath = _sourceDir + filePath; } // get rid of '..' and '.' if any string fullFilePath = Path.GetFullPath(filePath); // Get the relative path based on sourceDir string relPath = String.Empty; string newRelativeFilePath; if (fullFilePath.StartsWith(_sourceDir,StringComparison.OrdinalIgnoreCase)) { relPath = fullFilePath.Substring(_sourceDir.Length); // the original file is relative to the SourceDir. newSourceDir = _sourceDir; newRelativeFilePath = relPath; } else { // the original file is not relative to the SourceDir. // it could have its own fullpath or contains "..\" etc. // // In this case, we want to put the filename as relative filepath // and put the deepest directory that file is in as the new // SourceDir. // int pathEndIndex = fullFilePath.LastIndexOf("\\", StringComparison.Ordinal); newSourceDir = fullFilePath.Substring(0, pathEndIndex + 1); newRelativeFilePath = fullFilePath.Substring(pathEndIndex + 1); } return newRelativeFilePath; } #endregion #region private data private MarkupCompiler _mc; private string _sourceDir; private TaskLoggingHelper _taskLogger; private int _nErrors; private string _unknownErrorID; private string _applicationMarkup; private int _localizationDirectivesToLocFile; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // A wrapper class which can take inputs from build task and call the underneath // MarkupCompiler, CompilationUnit and other related types to do the real // compilation work. // This wrapper runs in the same Appdomain as MarkupCompiler, CompilationUnit, // // It miminizes the communication between two domains among the build tasks and // the real compiler classes. // // History: // // 10/26/05: weibz Created // //--------------------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Reflection; using System.Runtime.InteropServices; using MS.Internal.Markup; using Microsoft.Build.Utilities; using Microsoft.Build.Framework; using MS.Internal.Tasks; using MS.Utility; namespace MS.Internal { //// CompilerWrapper // internal class CompilerWrapper : MarshalByRefObject { //// ctor // internal CompilerWrapper() { _mc = new MarkupCompiler(); _sourceDir = Directory.GetCurrentDirectory() + "\\"; _nErrors = 0; } //// The valid source file extension for the passed language. // Normally a language supports more valid source file extensions. // User could choose one of them in project file. // If this property is not set, we will take use of the default one for the language. // internal string LanguageSourceExtension { set { _mc.LanguageSourceExtension = value; } } //// OutputPath : Generated code files, Baml fles will be put in this directory. // internal string OutputPath { set { _mc.TargetPath = value; } } //// The version of the assembly // internal string AssemblyVersion { set { _mc.AssemblyVersion = value; } } //// The key token of the assembly // internal string AssemblyPublicKeyToken { set { _mc.AssemblyPublicKeyToken = value; } } //// ApplicationMarkup // internal string ApplicationMarkup { set { _applicationMarkup = value; } } //// Loose file content list // internal string[] ContentFiles { set { _mc.ContentList = value; } } ////// Splash screen image to be displayed before application init /// internal string SplashImage { set { _mc.SplashImage = value; } } //// Assembly References. // //internal ArrayList References { set { _mc.ReferenceAssemblyList = value; } } // //If true code for supporting hosting in Browser is generated // internal bool HostInBrowser { set { _mc.HostInBrowser = value; } } //// Generated debugging information in the BAML file. // internal bool XamlDebuggingInformation { set { _mc.XamlDebuggingInformation = value; } } //// Controls how to generate localization information for each xaml file. // Valid values: None, CommentsOnly, All. // // internal int LocalizationDirectivesToLocFile { set { _localizationDirectivesToLocFile = value; } } //// Keep the application definition file if it contains local types which are implemented // in current target assembly. // internal string LocalXamlApplication { get { return _mc.LocalXamlApplication; } } //// Keep the page markup files if they contain local types which are implemented // in current target assembly. // internal string[] LocalXamlPages { get { return _mc.LocalXamlPages; } } ////// Get/Sets the TaskFileService on MarkupCompiler /// internal ITaskFileService TaskFileService { get { return _mc.TaskFileService; } set { _mc.TaskFileService = value; } } // // A wrapper property which maps to MarkupCompiler class's corresponding property. // This property will be called by MarkupCompilePass1 and Pass2 build tasks. // // It indicates whether any xaml file in current xaml list references internal types // from friend assembly or local target assembly. // internal bool HasInternals { get { return MarkupCompiler.HasInternals; } } //// TaskLoggingHelper // internal TaskLoggingHelper TaskLogger { set { _taskLogger = value; } } internal string UnknownErrorID { set { _unknownErrorID = value; } } internal int ErrorTimes { get { return _nErrors; } } //// Start the compilation. // // // // // // //internal bool DoCompilation(string assemblyName, string language, string rootNamespace, string[] fileList, bool isSecondPass) { bool ret = true; CompilationUnit compUnit = new CompilationUnit(assemblyName, language, rootNamespace, fileList); compUnit.Pass2 = isSecondPass; // Set some properties required by the CompilationUnit compUnit.ApplicationFile = _applicationMarkup; compUnit.SourcePath = _sourceDir; //Set the properties required by MarkupCompiler _mc.SourceFileResolve += new SourceFileResolveEventHandler(OnSourceFileResolve); _mc.Error += new MarkupErrorEventHandler(OnCompilerError); LocalizationDirectivesToLocFile localizeFlag = (LocalizationDirectivesToLocFile)_localizationDirectivesToLocFile; // // Localization file should not be generated for Intellisense build. Thus // checking IsRealBuild. // if ((localizeFlag == MS.Internal.LocalizationDirectivesToLocFile.All || localizeFlag == MS.Internal.LocalizationDirectivesToLocFile.CommentsOnly) && (TaskFileService.IsRealBuild)) { _mc.ParserHooks = new LocalizationParserHooks(_mc, localizeFlag, isSecondPass); } if (isSecondPass) { for (int i = 0; i < _mc.ReferenceAssemblyList.Count; i++) { ReferenceAssembly asmReference = _mc.ReferenceAssemblyList[i] as ReferenceAssembly; if (asmReference != null) { if (String.Compare(asmReference.AssemblyName, assemblyName, StringComparison.OrdinalIgnoreCase) == 0) { // Set the local assembly file to markupCompiler _mc.LocalAssemblyFile = asmReference; } } } } // finally compile the app _mc.Compile(compUnit); return ret; } #region private method // // Event hanlder for the Compiler Errors // // sd brancx // private void OnCompilerError(Object sender, MarkupErrorEventArgs e) { _nErrors++; // // Since Output from LogError() cannot be recognized by VS TaskList, so // we replaced it with LogErrorFromText( ) and pass all the required information // such as filename, line, offset, etc. // string strErrorCode; // Generate error message by going through the whole exception chain, including // its inner exceptions. string message = TaskHelper.GetWholeExceptionMessage(e.Exception); string errorText; strErrorCode = _taskLogger.ExtractMessageCode(message, out errorText); if (String.IsNullOrEmpty(strErrorCode)) { // If the exception is a Xml exception, show a pre-asigned error id for it. if (IsXmlException(e.Exception)) { message = SR.Get(SRID.InvalidXml, message); strErrorCode = _taskLogger.ExtractMessageCode(message, out errorText); } else { strErrorCode = _unknownErrorID; errorText = SR.Get(SRID.UnknownBuildError, errorText); } } _taskLogger.LogError(null, strErrorCode, null, e.FileName, e.LineNumber, e.LinePosition, 0, 0, errorText); } // // Detect if the exception is xmlException // private bool IsXmlException(Exception e) { bool isXmlException = false; while (e != null) { if (e is System.Xml.XmlException) { isXmlException = true; break; } else { e = e.InnerException; } } return isXmlException; } // // Handle the SourceFileResolve Event from MarkupCompiler. // It tries to GetResolvedFilePath for the new SourceDir and new RelativePath. // private void OnSourceFileResolve(Object sender, SourceFileResolveEventArgs e) { SourceFileInfo sourceFileInfo = e.SourceFileInfo; string newSourceDir = _sourceDir; string newRelativeFilePath; if (String.IsNullOrEmpty(sourceFileInfo.OriginalFilePath)) { newRelativeFilePath = sourceFileInfo.OriginalFilePath; } else { newRelativeFilePath = GetResolvedFilePath(sourceFileInfo.OriginalFilePath, ref newSourceDir); _taskLogger.LogMessageFromResources(MessageImportance.Low, SRID.FileResolved, sourceFileInfo.OriginalFilePath, newRelativeFilePath, newSourceDir); } if (sourceFileInfo.IsXamlFile) { // // For Xaml Source file, we need to remove the .xaml extension part. // int fileExtIndex = newRelativeFilePath.LastIndexOf(MarkupCompiler.DOT, StringComparison.Ordinal); newRelativeFilePath = newRelativeFilePath.Substring(0, fileExtIndex); } // // Update the SourcePath and RelativeSourceFilePath property in SourceFileInfo object. // sourceFileInfo.SourcePath = newSourceDir; sourceFileInfo.RelativeSourceFilePath = newRelativeFilePath; // Put the stream here. sourceFileInfo.Stream = TaskFileService.GetContent(sourceFileInfo.OriginalFilePath); } // // Return a new sourceDir and relative filepath for a given filePath. // This is for supporting of fullpath or ..\ in the original FilePath. // private string GetResolvedFilePath(string filePath, ref string newSourceDir) { // make it an absolute path if not already so if (!Path.IsPathRooted(filePath)) { filePath = _sourceDir + filePath; } // get rid of '..' and '.' if any string fullFilePath = Path.GetFullPath(filePath); // Get the relative path based on sourceDir string relPath = String.Empty; string newRelativeFilePath; if (fullFilePath.StartsWith(_sourceDir,StringComparison.OrdinalIgnoreCase)) { relPath = fullFilePath.Substring(_sourceDir.Length); // the original file is relative to the SourceDir. newSourceDir = _sourceDir; newRelativeFilePath = relPath; } else { // the original file is not relative to the SourceDir. // it could have its own fullpath or contains "..\" etc. // // In this case, we want to put the filename as relative filepath // and put the deepest directory that file is in as the new // SourceDir. // int pathEndIndex = fullFilePath.LastIndexOf("\\", StringComparison.Ordinal); newSourceDir = fullFilePath.Substring(0, pathEndIndex + 1); newRelativeFilePath = fullFilePath.Substring(pathEndIndex + 1); } return newRelativeFilePath; } #endregion #region private data private MarkupCompiler _mc; private string _sourceDir; private TaskLoggingHelper _taskLogger; private int _nErrors; private string _unknownErrorID; private string _applicationMarkup; private int _localizationDirectivesToLocFile; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
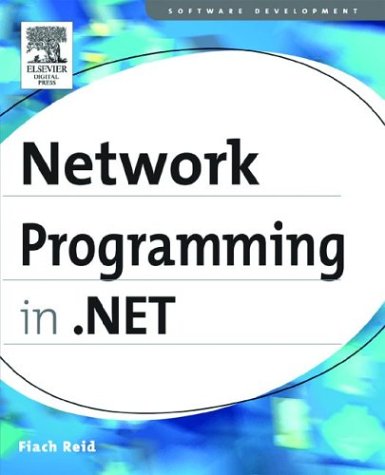
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeMultiPart.cs
- DelegatingConfigHost.cs
- ReliableSessionElement.cs
- SoapMessage.cs
- ExpandCollapseProviderWrapper.cs
- Imaging.cs
- DrawListViewSubItemEventArgs.cs
- DbExpressionRules.cs
- QueryAsyncResult.cs
- SimpleTableProvider.cs
- RouteParser.cs
- StoreContentChangedEventArgs.cs
- Region.cs
- CqlBlock.cs
- InputBinder.cs
- DescendantOverDescendantQuery.cs
- EntityContainerRelationshipSet.cs
- Listbox.cs
- DtdParser.cs
- FileInfo.cs
- XmlSchemaCompilationSettings.cs
- FunctionImportMapping.cs
- TdsParserSafeHandles.cs
- XmlNodeChangedEventArgs.cs
- IriParsingElement.cs
- XmlSubtreeReader.cs
- NodeLabelEditEvent.cs
- XmlSchemaSimpleTypeList.cs
- XmlWrappingReader.cs
- UrlPath.cs
- FormViewDeleteEventArgs.cs
- RoleService.cs
- IDQuery.cs
- PartialCachingControl.cs
- XMLSchema.cs
- BaseCodePageEncoding.cs
- DefaultBindingPropertyAttribute.cs
- Int32Collection.cs
- SoapTransportImporter.cs
- HttpModuleAction.cs
- XmlSchemaSimpleTypeRestriction.cs
- TagMapInfo.cs
- NamedPermissionSet.cs
- ComponentResourceKeyConverter.cs
- CompositeTypefaceMetrics.cs
- StreamInfo.cs
- PointConverter.cs
- UnknownWrapper.cs
- EntityDataSourceDesigner.cs
- ManifestSignedXml.cs
- UIElementAutomationPeer.cs
- ImageBrush.cs
- PropertyValueChangedEvent.cs
- XmlEntityReference.cs
- SQLRoleProvider.cs
- Pool.cs
- TrustManagerPromptUI.cs
- TypeSystem.cs
- XmlWriterSettings.cs
- DataPagerFieldCommandEventArgs.cs
- XmlWrappingWriter.cs
- SourceFileBuildProvider.cs
- XmlWhitespace.cs
- UnaryOperationBinder.cs
- DataPager.cs
- LoopExpression.cs
- ActivityTypeCodeDomSerializer.cs
- BaseParser.cs
- XmlSchemaSimpleTypeRestriction.cs
- VerificationException.cs
- ObjectViewEntityCollectionData.cs
- WindowsGraphicsWrapper.cs
- DetailsViewCommandEventArgs.cs
- OdbcReferenceCollection.cs
- ColumnReorderedEventArgs.cs
- SchemaElementDecl.cs
- PagesSection.cs
- MediaCommands.cs
- XmlReflectionMember.cs
- WindowsGraphics2.cs
- HtmlElement.cs
- SqlConnectionPoolGroupProviderInfo.cs
- UriParserTemplates.cs
- AutomationPatternInfo.cs
- ObjectItemAttributeAssemblyLoader.cs
- SHA512CryptoServiceProvider.cs
- TextControl.cs
- TimeEnumHelper.cs
- DataGridViewSelectedColumnCollection.cs
- XhtmlTextWriter.cs
- RewritingProcessor.cs
- TraceHandlerErrorFormatter.cs
- DataGridParentRows.cs
- CodeDomSerializerBase.cs
- Logging.cs
- DrawToolTipEventArgs.cs
- InstanceContextManager.cs
- TextBoxBase.cs
- RandomNumberGenerator.cs
- Operators.cs