Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Configuration / HostingPreferredMapPath.cs / 1305376 / HostingPreferredMapPath.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Configuration; using System.Collections; using System.Globalization; using System.Xml; using System.Text; using System.Web.Util; using System.Web.UI; using System.IO; using System.Web.Hosting; // // IConfigMapPath that uses the HostingEnvironment's IConfigMapPath for // paths that it maps, and uses the web server IConfigMapPath for // all other paths. // // This allows us to use mappings for an app using SimpleApplicationHost, // while still correctly mapping paths outside the app. // class HostingPreferredMapPath : IConfigMapPath { IConfigMapPath _iisConfigMapPath; IConfigMapPath _hostingConfigMapPath; internal static IConfigMapPath GetInstance() { IConfigMapPath iisConfigMapPath = IISMapPath.GetInstance(); IConfigMapPath hostingConfigMapPath = HostingEnvironment.ConfigMapPath; // Only delegate if the types implementing IConfigMapPath are different. if (hostingConfigMapPath == null || iisConfigMapPath.GetType() == hostingConfigMapPath.GetType()) return iisConfigMapPath; return new HostingPreferredMapPath(iisConfigMapPath, hostingConfigMapPath); } HostingPreferredMapPath(IConfigMapPath iisConfigMapPath, IConfigMapPath hostingConfigMapPath) { _iisConfigMapPath = iisConfigMapPath; _hostingConfigMapPath = hostingConfigMapPath; } public string GetMachineConfigFilename() { string filename = _hostingConfigMapPath.GetMachineConfigFilename(); if (string.IsNullOrEmpty(filename)) { filename = _iisConfigMapPath.GetMachineConfigFilename(); } return filename; } public string GetRootWebConfigFilename() { string filename = _hostingConfigMapPath.GetRootWebConfigFilename(); if (string.IsNullOrEmpty(filename)) { filename = _iisConfigMapPath.GetRootWebConfigFilename(); } return filename; } public void GetPathConfigFilename( string siteID, string path, out string directory, out string baseName) { _hostingConfigMapPath.GetPathConfigFilename(siteID, path, out directory, out baseName); if (string.IsNullOrEmpty(directory)) { _iisConfigMapPath.GetPathConfigFilename(siteID, path, out directory, out baseName); } } public void GetDefaultSiteNameAndID(out string siteName, out string siteID) { _hostingConfigMapPath.GetDefaultSiteNameAndID(out siteName, out siteID); if (string.IsNullOrEmpty(siteID)) { _iisConfigMapPath.GetDefaultSiteNameAndID(out siteName, out siteID); } } public void ResolveSiteArgument(string siteArgument, out string siteName, out string siteID) { _hostingConfigMapPath.ResolveSiteArgument(siteArgument, out siteName, out siteID); if (string.IsNullOrEmpty(siteID)) { _iisConfigMapPath.ResolveSiteArgument(siteArgument, out siteName, out siteID); } } public string MapPath(string siteID, string path) { string physicalPath = _hostingConfigMapPath.MapPath(siteID, path); if (string.IsNullOrEmpty(physicalPath)) { physicalPath = _iisConfigMapPath.MapPath(siteID, path); } return physicalPath; } public string GetAppPathForPath(string siteID, string path) { string appPath = _hostingConfigMapPath.GetAppPathForPath(siteID, path); if (appPath == null) { appPath = _iisConfigMapPath.GetAppPathForPath(siteID, path); } return appPath; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Configuration; using System.Collections; using System.Globalization; using System.Xml; using System.Text; using System.Web.Util; using System.Web.UI; using System.IO; using System.Web.Hosting; // // IConfigMapPath that uses the HostingEnvironment's IConfigMapPath for // paths that it maps, and uses the web server IConfigMapPath for // all other paths. // // This allows us to use mappings for an app using SimpleApplicationHost, // while still correctly mapping paths outside the app. // class HostingPreferredMapPath : IConfigMapPath { IConfigMapPath _iisConfigMapPath; IConfigMapPath _hostingConfigMapPath; internal static IConfigMapPath GetInstance() { IConfigMapPath iisConfigMapPath = IISMapPath.GetInstance(); IConfigMapPath hostingConfigMapPath = HostingEnvironment.ConfigMapPath; // Only delegate if the types implementing IConfigMapPath are different. if (hostingConfigMapPath == null || iisConfigMapPath.GetType() == hostingConfigMapPath.GetType()) return iisConfigMapPath; return new HostingPreferredMapPath(iisConfigMapPath, hostingConfigMapPath); } HostingPreferredMapPath(IConfigMapPath iisConfigMapPath, IConfigMapPath hostingConfigMapPath) { _iisConfigMapPath = iisConfigMapPath; _hostingConfigMapPath = hostingConfigMapPath; } public string GetMachineConfigFilename() { string filename = _hostingConfigMapPath.GetMachineConfigFilename(); if (string.IsNullOrEmpty(filename)) { filename = _iisConfigMapPath.GetMachineConfigFilename(); } return filename; } public string GetRootWebConfigFilename() { string filename = _hostingConfigMapPath.GetRootWebConfigFilename(); if (string.IsNullOrEmpty(filename)) { filename = _iisConfigMapPath.GetRootWebConfigFilename(); } return filename; } public void GetPathConfigFilename( string siteID, string path, out string directory, out string baseName) { _hostingConfigMapPath.GetPathConfigFilename(siteID, path, out directory, out baseName); if (string.IsNullOrEmpty(directory)) { _iisConfigMapPath.GetPathConfigFilename(siteID, path, out directory, out baseName); } } public void GetDefaultSiteNameAndID(out string siteName, out string siteID) { _hostingConfigMapPath.GetDefaultSiteNameAndID(out siteName, out siteID); if (string.IsNullOrEmpty(siteID)) { _iisConfigMapPath.GetDefaultSiteNameAndID(out siteName, out siteID); } } public void ResolveSiteArgument(string siteArgument, out string siteName, out string siteID) { _hostingConfigMapPath.ResolveSiteArgument(siteArgument, out siteName, out siteID); if (string.IsNullOrEmpty(siteID)) { _iisConfigMapPath.ResolveSiteArgument(siteArgument, out siteName, out siteID); } } public string MapPath(string siteID, string path) { string physicalPath = _hostingConfigMapPath.MapPath(siteID, path); if (string.IsNullOrEmpty(physicalPath)) { physicalPath = _iisConfigMapPath.MapPath(siteID, path); } return physicalPath; } public string GetAppPathForPath(string siteID, string path) { string appPath = _hostingConfigMapPath.GetAppPathForPath(siteID, path); if (appPath == null) { appPath = _iisConfigMapPath.GetAppPathForPath(siteID, path); } return appPath; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
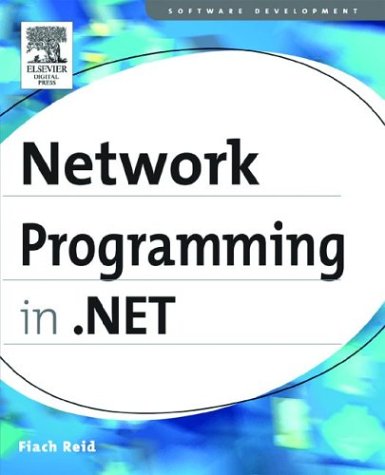
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StronglyTypedResourceBuilder.cs
- HierarchicalDataTemplate.cs
- TrailingSpaceComparer.cs
- ControlValuePropertyAttribute.cs
- SystemException.cs
- ProtectedConfiguration.cs
- OutputCache.cs
- AppSettingsSection.cs
- NetStream.cs
- SqlBooleanMismatchVisitor.cs
- ObjectListFieldCollection.cs
- WebPartCancelEventArgs.cs
- CalendarAutoFormatDialog.cs
- TextLine.cs
- PropertyValueChangedEvent.cs
- TargetInvocationException.cs
- TouchEventArgs.cs
- GetRecipientListRequest.cs
- SoapIgnoreAttribute.cs
- PopupEventArgs.cs
- HashSetEqualityComparer.cs
- DataControlField.cs
- DesignerForm.cs
- ManualResetEvent.cs
- IssuedTokenParametersElement.cs
- MouseGesture.cs
- XmlAttributeCache.cs
- PropertyValueUIItem.cs
- ScrollProviderWrapper.cs
- QuestionEventArgs.cs
- XmlUtilWriter.cs
- HtmlListAdapter.cs
- Int32AnimationBase.cs
- WindowsListView.cs
- SqlStatistics.cs
- SqlInternalConnection.cs
- ToolStripRenderEventArgs.cs
- iisPickupDirectory.cs
- SqlDataAdapter.cs
- Panel.cs
- ButtonFieldBase.cs
- DataList.cs
- MemoryPressure.cs
- PageThemeBuildProvider.cs
- DataKey.cs
- PageContent.cs
- DataGridViewCellStateChangedEventArgs.cs
- RoutedEventArgs.cs
- WCFBuildProvider.cs
- DiscreteKeyFrames.cs
- SafeRegistryKey.cs
- QilList.cs
- RequestCachePolicy.cs
- WebServiceMethodData.cs
- DataGridViewColumnHeaderCell.cs
- MemberListBinding.cs
- NumericUpDownAccelerationCollection.cs
- WorkflowInstanceProxy.cs
- HostingEnvironmentException.cs
- DocumentStream.cs
- AsymmetricAlgorithm.cs
- TagPrefixCollection.cs
- StatusBarAutomationPeer.cs
- TimeSpanOrInfiniteConverter.cs
- ReceiveSecurityHeaderEntry.cs
- DEREncoding.cs
- Pair.cs
- XmlObjectSerializerReadContext.cs
- InstanceKeyView.cs
- CodeTypeParameterCollection.cs
- CredentialCache.cs
- Sql8ConformanceChecker.cs
- ADRoleFactory.cs
- thaishape.cs
- Mouse.cs
- TransformPatternIdentifiers.cs
- RightsManagementProvider.cs
- IdentityHolder.cs
- ColorTransformHelper.cs
- CodePageUtils.cs
- safesecurityhelperavalon.cs
- JsonEncodingStreamWrapper.cs
- KoreanCalendar.cs
- SpecularMaterial.cs
- MetadataArtifactLoaderCompositeFile.cs
- KnownAssemblyEntry.cs
- RoleGroup.cs
- DBConnection.cs
- TableLayoutSettings.cs
- SafeEventHandle.cs
- Stylus.cs
- DescendentsWalker.cs
- MouseGestureValueSerializer.cs
- WebPartHelpVerb.cs
- ServiceManager.cs
- GetTokenRequest.cs
- PrimitiveXmlSerializers.cs
- DataGridViewTopRowAccessibleObject.cs
- PropertyTab.cs
- ListBindableAttribute.cs