Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / FontFace / TypefaceCollection.cs / 1 / TypefaceCollection.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: TypefaceCollection.cs // // Contents: Collection of typefaces // // Created: 5-15-2003 Michael Leonov (mleonov) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Media; using MS.Internal.FontCache; using System.Globalization; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.FontFace { internal unsafe struct TypefaceCollection : ICollection{ private CachedFontFamily _family; private FontFamily _fontFamily; public TypefaceCollection(FontFamily fontFamily, CachedFontFamily family) { _fontFamily = fontFamily; _family = family; } #region ICollection Members public void Add(Typeface item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(Typeface item) { foreach (Typeface t in this) { if (t.Equals(item)) return true; } return false; } public void CopyTo(Typeface[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } foreach (Typeface t in this) { array[arrayIndex++] = t; } } public int Count { get { return _family.NumberOfFaces; } } public bool IsReadOnly { get { return true; } } public bool Remove(Typeface item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { return new Enumerator(this); } #endregion #region IEnumerable Members System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new Enumerator(this); } #endregion private struct Enumerator : IEnumerator { public Enumerator(TypefaceCollection typefaceCollection) { _typefaceCollection = typefaceCollection; // Unfortunately we cannot call Reset() here because not all of the fields are initialized. _familyEnumerator = ((IEnumerable )typefaceCollection._family).GetEnumerator(); } #region IEnumerator Members public Typeface Current { get { CachedFontFace face = _familyEnumerator.Current; return new Typeface(_typefaceCollection._fontFamily, face.Style, face.Weight, face.Stretch); } } #endregion #region IDisposable Members public void Dispose() {} #endregion #region IEnumerator Members object System.Collections.IEnumerator.Current { get { return ((IEnumerator )this).Current; } } public bool MoveNext() { return _familyEnumerator.MoveNext(); } public void Reset() { _familyEnumerator = ((IEnumerable )_typefaceCollection._family).GetEnumerator(); } #endregion private IEnumerator _familyEnumerator; private TypefaceCollection _typefaceCollection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: TypefaceCollection.cs // // Contents: Collection of typefaces // // Created: 5-15-2003 Michael Leonov (mleonov) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Media; using MS.Internal.FontCache; using System.Globalization; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.FontFace { internal unsafe struct TypefaceCollection : ICollection { private CachedFontFamily _family; private FontFamily _fontFamily; public TypefaceCollection(FontFamily fontFamily, CachedFontFamily family) { _fontFamily = fontFamily; _family = family; } #region ICollection Members public void Add(Typeface item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(Typeface item) { foreach (Typeface t in this) { if (t.Equals(item)) return true; } return false; } public void CopyTo(Typeface[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } foreach (Typeface t in this) { array[arrayIndex++] = t; } } public int Count { get { return _family.NumberOfFaces; } } public bool IsReadOnly { get { return true; } } public bool Remove(Typeface item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { return new Enumerator(this); } #endregion #region IEnumerable Members System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new Enumerator(this); } #endregion private struct Enumerator : IEnumerator { public Enumerator(TypefaceCollection typefaceCollection) { _typefaceCollection = typefaceCollection; // Unfortunately we cannot call Reset() here because not all of the fields are initialized. _familyEnumerator = ((IEnumerable )typefaceCollection._family).GetEnumerator(); } #region IEnumerator Members public Typeface Current { get { CachedFontFace face = _familyEnumerator.Current; return new Typeface(_typefaceCollection._fontFamily, face.Style, face.Weight, face.Stretch); } } #endregion #region IDisposable Members public void Dispose() {} #endregion #region IEnumerator Members object System.Collections.IEnumerator.Current { get { return ((IEnumerator )this).Current; } } public bool MoveNext() { return _familyEnumerator.MoveNext(); } public void Reset() { _familyEnumerator = ((IEnumerable )_typefaceCollection._family).GetEnumerator(); } #endregion private IEnumerator _familyEnumerator; private TypefaceCollection _typefaceCollection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
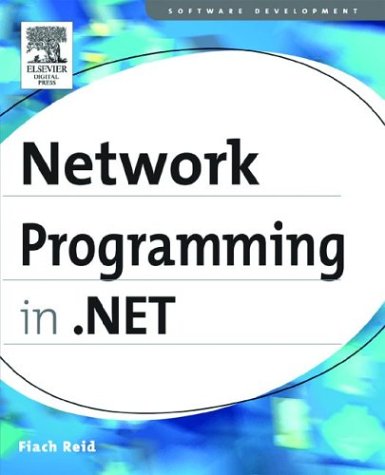
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlValueConverter.cs
- DrawingImage.cs
- ConfigurationCollectionAttribute.cs
- RadioButtonFlatAdapter.cs
- log.cs
- EncryptedData.cs
- CodeArrayIndexerExpression.cs
- TableItemPattern.cs
- SecurityElement.cs
- CursorConverter.cs
- ZipIOLocalFileHeader.cs
- GridLengthConverter.cs
- WbmpConverter.cs
- Viewport2DVisual3D.cs
- CompositeScriptReference.cs
- SID.cs
- Triangle.cs
- Int32Storage.cs
- UIPropertyMetadata.cs
- Cursor.cs
- EmissiveMaterial.cs
- GenericArgumentsUpdater.cs
- TitleStyle.cs
- ActivityExecutionContext.cs
- ConditionedDesigner.cs
- AnimationTimeline.cs
- ConnectionInterfaceCollection.cs
- TextRangeEditTables.cs
- LoginCancelEventArgs.cs
- NaturalLanguageHyphenator.cs
- CombinedGeometry.cs
- ScrollBarRenderer.cs
- PropertyBuilder.cs
- HttpModuleCollection.cs
- ThreadInterruptedException.cs
- SmtpCommands.cs
- ApplicationTrust.cs
- Mouse.cs
- UserMapPath.cs
- PathGeometry.cs
- infer.cs
- InputProcessorProfiles.cs
- MailAddress.cs
- DataSourceCacheDurationConverter.cs
- XmlSchemaComplexContentExtension.cs
- Page.cs
- uribuilder.cs
- SmtpNetworkElement.cs
- ConditionalAttribute.cs
- WebAdminConfigurationHelper.cs
- Int16Animation.cs
- ImageDesigner.cs
- UpdateEventArgs.cs
- Compilation.cs
- List.cs
- MetadataCacheItem.cs
- ObjectAnimationBase.cs
- ClearCollection.cs
- PackageDigitalSignature.cs
- Activity.cs
- DetailsViewUpdateEventArgs.cs
- SecurityException.cs
- SplitterCancelEvent.cs
- NTAccount.cs
- SoapExtensionTypeElementCollection.cs
- TransactionContext.cs
- ScaleTransform3D.cs
- SQLGuid.cs
- UIElement.cs
- BinaryConverter.cs
- COM2AboutBoxPropertyDescriptor.cs
- OdbcCommandBuilder.cs
- DesignTimeVisibleAttribute.cs
- RichTextBoxAutomationPeer.cs
- DataBoundLiteralControl.cs
- PropertySourceInfo.cs
- Typography.cs
- HtmlContainerControl.cs
- QilVisitor.cs
- IDictionary.cs
- ChannelSinkStacks.cs
- XamlFigureLengthSerializer.cs
- RegexWorker.cs
- MD5CryptoServiceProvider.cs
- ServiceBehaviorElement.cs
- Signature.cs
- XmlSchemaInfo.cs
- SqlMethodAttribute.cs
- EntitySetBaseCollection.cs
- AmbiguousMatchException.cs
- FileSecurity.cs
- ParentQuery.cs
- StorageAssociationTypeMapping.cs
- AnonymousIdentificationModule.cs
- ImageIndexConverter.cs
- BookmarkUndoUnit.cs
- DispatcherEventArgs.cs
- InputLanguage.cs
- DSACryptoServiceProvider.cs
- ThreadStaticAttribute.cs