Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / ChainOfResponsibility.cs / 1 / ChainOfResponsibility.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All rights reserved. // //// An implementation of the 'Chain of Responsibility' from Design Patterns // // // History: // 08/28/2005: [....]: Initial implementation. //----------------------------------------------------------------------------- using System.Collections.Generic; namespace MS.Internal.Documents.Application { ////// An implementation of the 'Chain of Responsibility' from Design Patterns /// ////// Design Comments: /// /// The pattern is implemented as: /// /// - concrete coupling of Ts (successors) at construction /// - request are represented by ChainOfResponsiblity[T, S].Action delegate /// where S is the parameter data /// - IChainOfResponsibiltyNode[S] is used to determin if the member is willing /// to participate in the request. /// ///A common type for all members of the chain. ///A common type for data for all members of the chain. /// internal class ChainOfResponsiblitywhere T : IChainOfResponsibiltyNode { #region Constructors //------------------------------------------------------------------------- // Constructors //------------------------------------------------------------------------- ////// Provides for concrete coupling of T's at construction. /// /// internal ChainOfResponsiblity( params T[] members) { _members = new List(members); } #endregion Constructors #region Internal Methods //-------------------------------------------------------------------------- // Internal Methods //------------------------------------------------------------------------- /// /// Will dispatch the action first to last in the chain until a member /// reports handling the action. /// ///True if successfully handled by a member. /// The action to perform. /// The subject to perform it on. internal bool Dispatch(ChainOfResponsiblity.Action action, S subject) { bool handled = false; foreach (T member in _members) { if (member.IsResponsible(subject)) { Trace.SafeWrite( Trace.File, "Dispatching {0} to {1} using {2}.", action.Method.Name, member.GetType().Name, subject.GetType().Name); handled = action(member, subject); if (handled) { Trace.SafeWrite( Trace.File, "Finished {0} by {1} with {2}.", action.Method.Name, member.GetType().Name, subject.GetType().Name); break; } } } return handled; } #endregion Internal Methods #region Internal Delegates //-------------------------------------------------------------------------- // Internal Delegates //-------------------------------------------------------------------------- /// /// Actions which members T can be perform on S. /// /// The member to perform the action. /// The subject to perform the action on. ///True if handled by the member. internal delegate bool Action(T member, S subject); #endregion Internal Delegates #region Private Fields //------------------------------------------------------------------------- // Private Fields //-------------------------------------------------------------------------- ////// The concrete list of members. /// private List_members; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
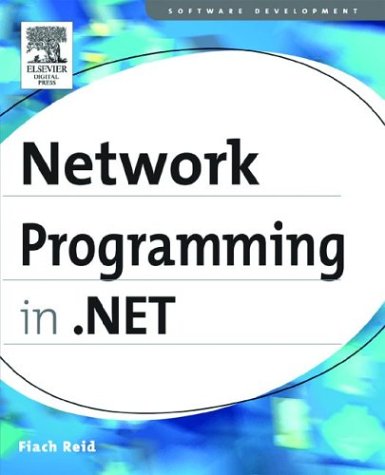
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EncoderParameter.cs
- ProfilePropertyMetadata.cs
- HotSpot.cs
- XmlCustomFormatter.cs
- SoundPlayerAction.cs
- BorderGapMaskConverter.cs
- DrawingDrawingContext.cs
- RegexNode.cs
- PagerSettings.cs
- FormDocumentDesigner.cs
- OleDbMetaDataFactory.cs
- PlatformNotSupportedException.cs
- WmlPanelAdapter.cs
- TabControlCancelEvent.cs
- SemaphoreFullException.cs
- HierarchicalDataBoundControl.cs
- OdbcPermission.cs
- WebPartCancelEventArgs.cs
- Sql8ConformanceChecker.cs
- HttpBrowserCapabilitiesWrapper.cs
- PointAnimationUsingPath.cs
- LexicalChunk.cs
- EncoderExceptionFallback.cs
- TransformerConfigurationWizardBase.cs
- AuthenticationService.cs
- MiniParameterInfo.cs
- DbProviderSpecificTypePropertyAttribute.cs
- SecurityTokenSerializer.cs
- Events.cs
- InputBinder.cs
- PageSetupDialog.cs
- BindingManagerDataErrorEventArgs.cs
- ParameterToken.cs
- OdbcCommand.cs
- CodeAttributeArgumentCollection.cs
- SafeUserTokenHandle.cs
- EntitySqlException.cs
- Exceptions.cs
- CompositeControl.cs
- WmlSelectionListAdapter.cs
- DependencyPropertyKey.cs
- WorkflowRuntimeService.cs
- XamlPointCollectionSerializer.cs
- SqlClientFactory.cs
- OleDbException.cs
- HtmlDocument.cs
- EventBookmark.cs
- CustomPopupPlacement.cs
- ControlIdConverter.cs
- ViewStateException.cs
- RunWorkerCompletedEventArgs.cs
- ParallelTimeline.cs
- ContextStaticAttribute.cs
- GlyphElement.cs
- Stroke.cs
- SqlServer2KCompatibilityAnnotation.cs
- ContainerAction.cs
- WebRequest.cs
- DiscoveryExceptionDictionary.cs
- UIPermission.cs
- OperationExecutionFault.cs
- TextServicesCompartmentContext.cs
- HtmlTextArea.cs
- LongPath.cs
- ReflectionPermission.cs
- ProcessModelInfo.cs
- ObjectPersistData.cs
- ThreadStartException.cs
- PresentationAppDomainManager.cs
- serverconfig.cs
- QueuePropertyVariants.cs
- AssemblyAssociatedContentFileAttribute.cs
- UserCancellationException.cs
- HtmlTableCell.cs
- DataGridColumn.cs
- SafeIUnknown.cs
- ItemTypeToolStripMenuItem.cs
- DataObjectCopyingEventArgs.cs
- IdentifierService.cs
- MonthCalendar.cs
- RawTextInputReport.cs
- BaseHashHelper.cs
- ListViewUpdatedEventArgs.cs
- ListQueryResults.cs
- EdmItemError.cs
- VirtualizingPanel.cs
- AssemblyFilter.cs
- IriParsingElement.cs
- EmptyEnumerable.cs
- MeshGeometry3D.cs
- _ChunkParse.cs
- EventSinkHelperWriter.cs
- DomainLiteralReader.cs
- columnmapfactory.cs
- DateTimeValueSerializerContext.cs
- TypeToken.cs
- WorkflowOwnershipException.cs
- TableStyle.cs
- IntranetCredentialPolicy.cs
- Application.cs