Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Selectors / SecurityTokenSerializer.cs / 1 / SecurityTokenSerializer.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Selectors { using System.Xml; using System.IdentityModel.Tokens; ////// SecurityTokenSerializer is responsible for writing and reading SecurityKeyIdentifiers, SecurityKeyIdentifierClauses and SecurityTokens. /// In order to read SecurityTokens the SecurityTokenSerializer may need to resolve token references using the SecurityTokenResolvers that get passed in. /// The SecurityTokenSerializer is stateless /// Exceptions: XmlException, SecurityTokenException, NotSupportedException, InvalidOperationException, ArgumentException /// public abstract class SecurityTokenSerializer { // public methods public bool CanReadToken(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return CanReadTokenCore(reader); ; } public bool CanWriteToken(SecurityToken token) { if (token == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("token"); } return CanWriteTokenCore(token); ; } public bool CanReadKeyIdentifier(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return CanReadKeyIdentifierCore(reader); ; } public bool CanWriteKeyIdentifier(SecurityKeyIdentifier keyIdentifier) { if (keyIdentifier == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifier"); } return CanWriteKeyIdentifierCore(keyIdentifier); ; } public bool CanReadKeyIdentifierClause(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return CanReadKeyIdentifierClauseCore(reader); ; } public bool CanWriteKeyIdentifierClause(SecurityKeyIdentifierClause keyIdentifierClause) { if (keyIdentifierClause == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); } return CanWriteKeyIdentifierClauseCore(keyIdentifierClause); ; } public SecurityToken ReadToken(XmlReader reader, SecurityTokenResolver tokenResolver) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return ReadTokenCore(reader, tokenResolver); } public void WriteToken(XmlWriter writer, SecurityToken token) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (token == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("token"); } WriteTokenCore(writer, token); } public SecurityKeyIdentifier ReadKeyIdentifier(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return ReadKeyIdentifierCore(reader); } public void WriteKeyIdentifier(XmlWriter writer, SecurityKeyIdentifier keyIdentifier) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (keyIdentifier == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifier"); } WriteKeyIdentifierCore(writer, keyIdentifier); } public SecurityKeyIdentifierClause ReadKeyIdentifierClause(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return ReadKeyIdentifierClauseCore(reader); } public void WriteKeyIdentifierClause(XmlWriter writer, SecurityKeyIdentifierClause keyIdentifierClause) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (keyIdentifierClause == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); } WriteKeyIdentifierClauseCore(writer, keyIdentifierClause); } // protected abstract methods protected abstract bool CanReadTokenCore(XmlReader reader); protected abstract bool CanWriteTokenCore(SecurityToken token); protected abstract bool CanReadKeyIdentifierCore(XmlReader reader); protected abstract bool CanWriteKeyIdentifierCore(SecurityKeyIdentifier keyIdentifier); protected abstract bool CanReadKeyIdentifierClauseCore(XmlReader reader); protected abstract bool CanWriteKeyIdentifierClauseCore(SecurityKeyIdentifierClause keyIdentifierClause); protected abstract SecurityToken ReadTokenCore(XmlReader reader, SecurityTokenResolver tokenResolver); protected abstract void WriteTokenCore(XmlWriter writer, SecurityToken token); protected abstract SecurityKeyIdentifier ReadKeyIdentifierCore(XmlReader reader); protected abstract void WriteKeyIdentifierCore(XmlWriter writer, SecurityKeyIdentifier keyIdentifier); protected abstract SecurityKeyIdentifierClause ReadKeyIdentifierClauseCore(XmlReader reader); protected abstract void WriteKeyIdentifierClauseCore(XmlWriter writer, SecurityKeyIdentifierClause keyIdentifierClause); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
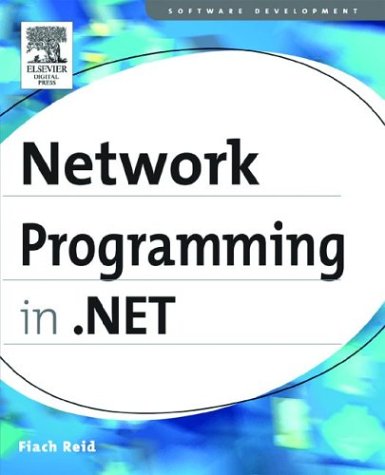
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AstTree.cs
- PixelFormat.cs
- SignatureHelper.cs
- autovalidator.cs
- Range.cs
- remotingproxy.cs
- Publisher.cs
- ValuePatternIdentifiers.cs
- X509Certificate2Collection.cs
- DayRenderEvent.cs
- CodeSubDirectory.cs
- DomainUpDown.cs
- ProtocolsConfigurationHandler.cs
- OdbcParameterCollection.cs
- XamlHostingSection.cs
- KnownTypeAttribute.cs
- XmlSchemaCompilationSettings.cs
- InfiniteTimeSpanConverter.cs
- UIElementPropertyUndoUnit.cs
- VersionedStream.cs
- QueryCacheManager.cs
- SchemaElementDecl.cs
- InfoCardArgumentException.cs
- XmlSchemaInferenceException.cs
- DocumentOrderQuery.cs
- EncoderNLS.cs
- SessionEndingCancelEventArgs.cs
- WebPartTransformerAttribute.cs
- XmlException.cs
- SQLDateTime.cs
- PasswordDeriveBytes.cs
- NavigationWindow.cs
- StreamWriter.cs
- ListViewItem.cs
- HtmlTextArea.cs
- EnumMember.cs
- ThreadLocal.cs
- ResourceProviderFactory.cs
- SchemaImporterExtensionElement.cs
- IntSecurity.cs
- RegexStringValidator.cs
- DefaultAssemblyResolver.cs
- DataViewManagerListItemTypeDescriptor.cs
- FileSystemInfo.cs
- CategoryNameCollection.cs
- ButtonChrome.cs
- LiteralText.cs
- TaskFileService.cs
- ConfigurationProperty.cs
- BuildResult.cs
- EntityTypeEmitter.cs
- COSERVERINFO.cs
- TranslateTransform3D.cs
- BooleanConverter.cs
- InputBindingCollection.cs
- TraceListeners.cs
- CreateUserWizard.cs
- ConnectionStringSettingsCollection.cs
- Button.cs
- ServiceChannelFactory.cs
- KeyEventArgs.cs
- UserControl.cs
- RadioButtonAutomationPeer.cs
- DataSourceControlBuilder.cs
- CaseCqlBlock.cs
- FocusManager.cs
- DataTableMapping.cs
- RegionInfo.cs
- DefaultBinder.cs
- BooleanStorage.cs
- WebPartVerbsEventArgs.cs
- UserMapPath.cs
- PointF.cs
- TreeChangeInfo.cs
- Delegate.cs
- ChannelCacheDefaults.cs
- EntityCommandDefinition.cs
- MobileCapabilities.cs
- TableFieldsEditor.cs
- ImagingCache.cs
- TextProperties.cs
- XmlRawWriter.cs
- StringBuilder.cs
- WebPartRestoreVerb.cs
- MailWebEventProvider.cs
- ObjectConverter.cs
- HttpCacheVary.cs
- XmlQualifiedName.cs
- webproxy.cs
- AutomationFocusChangedEventArgs.cs
- ThreadStateException.cs
- _ConnectOverlappedAsyncResult.cs
- TimeSpanSecondsConverter.cs
- RemoteDebugger.cs
- Exceptions.cs
- MeasureData.cs
- EdmToObjectNamespaceMap.cs
- XmlChoiceIdentifierAttribute.cs
- BitmapImage.cs
- CheckedListBox.cs