Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Permissions / SiteIdentityPermission.cs / 1 / SiteIdentityPermission.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // SiteIdentityPermission.cs // namespace System.Security.Permissions { using System; using SecurityElement = System.Security.SecurityElement; using SiteString = System.Security.Util.SiteString; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.Serialization; [System.Runtime.InteropServices.ComVisible(true)] [Serializable()] sealed public class SiteIdentityPermission : CodeAccessPermission, IBuiltInPermission { //------------------------------------------------------ // // PRIVATE STATE DATA // //----------------------------------------------------- [OptionalField(VersionAdded = 2)] private bool m_unrestricted; [OptionalField(VersionAdded = 2)] private SiteString[] m_sites; // This field will be populated only for non X-AD scenarios where we create a XML-ised string of the Permission [OptionalField(VersionAdded = 2)] private String m_serializedPermission; // This field is legacy info from v1.x and is never used in v2.0 and beyond: purely for serialization purposes private SiteString m_site; [OnDeserialized] private void OnDeserialized(StreamingContext ctx) { // v2.0 and beyond XML case if (m_serializedPermission != null) { FromXml(SecurityElement.FromString(m_serializedPermission)); m_serializedPermission = null; } else if (m_site != null) //v1.x case where we read the m_site value { m_unrestricted = false; m_sites = new SiteString[1]; m_sites[0] = m_site; m_site = null; } } [OnSerializing] private void OnSerializing(StreamingContext ctx) { if ((ctx.State & ~(StreamingContextStates.Clone|StreamingContextStates.CrossAppDomain)) != 0) { m_serializedPermission = ToXml().ToString(); //for the v2 and beyond case if (m_sites != null && m_sites.Length == 1) // for the v1.x case m_site = m_sites[0]; } } [OnSerialized] private void OnSerialized(StreamingContext ctx) { if ((ctx.State & ~(StreamingContextStates.Clone|StreamingContextStates.CrossAppDomain)) != 0) { m_serializedPermission = null; m_site = null; } } //----------------------------------------------------- // // PUBLIC CONSTRUCTORS // //----------------------------------------------------- public SiteIdentityPermission(PermissionState state) { if (state == PermissionState.Unrestricted) { if(CodeAccessSecurityEngine.DoesFullTrustMeanFullTrust()) m_unrestricted = true; else throw new ArgumentException(Environment.GetResourceString("Argument_UnrestrictedIdentityPermission")); } else if (state == PermissionState.None) { m_unrestricted = false; } else { throw new ArgumentException(Environment.GetResourceString("Argument_InvalidPermissionState")); } } public SiteIdentityPermission( String site ) { Site = site; } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //----------------------------------------------------- public String Site { set { m_unrestricted = false; m_sites = new SiteString[1]; m_sites[0] = new SiteString( value ); } get { if(m_sites == null) return ""; if(m_sites.Length == 1) return m_sites[0].ToString(); throw new NotSupportedException(Environment.GetResourceString("NotSupported_AmbiguousIdentity")); } } //------------------------------------------------------ // // PRIVATE AND PROTECTED HELPERS FOR ACCESSORS AND CONSTRUCTORS // //------------------------------------------------------ //----------------------------------------------------- // // CODEACCESSPERMISSION IMPLEMENTATION // //------------------------------------------------------ //----------------------------------------------------- // // IPERMISSION IMPLEMENTATION // //----------------------------------------------------- public override IPermission Copy() { SiteIdentityPermission perm = new SiteIdentityPermission( PermissionState.None ); perm.m_unrestricted = this.m_unrestricted; if (this.m_sites != null) { perm.m_sites = new SiteString[this.m_sites.Length]; int n; for(n = 0; n < this.m_sites.Length; n++) perm.m_sites[n] = (SiteString)this.m_sites[n].Copy(); } return perm; } public override bool IsSubsetOf(IPermission target) { if (target == null) { if(m_unrestricted) return false; if(m_sites == null) return true; if(m_sites.Length == 0) return true; return false; } SiteIdentityPermission that = target as SiteIdentityPermission; if(that == null) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName)); if(that.m_unrestricted) return true; if(m_unrestricted) return false; if(this.m_sites != null) { foreach(SiteString ssThis in this.m_sites) { bool bOK = false; if(that.m_sites != null) { foreach(SiteString ssThat in that.m_sites) { if(ssThis.IsSubsetOf(ssThat)) { bOK = true; break; } } } if(!bOK) return false; } } return true; } public override IPermission Intersect(IPermission target) { if (target == null) return null; SiteIdentityPermission that = target as SiteIdentityPermission; if(that == null) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName)); if(this.m_unrestricted && that.m_unrestricted) { SiteIdentityPermission res = new SiteIdentityPermission(PermissionState.None); res.m_unrestricted = true; return res; } if(this.m_unrestricted) return that.Copy(); if(that.m_unrestricted) return this.Copy(); if(this.m_sites == null || that.m_sites == null || this.m_sites.Length == 0 || that.m_sites.Length == 0) return null; ArrayList alSites = new ArrayList(); foreach(SiteString ssThis in this.m_sites) { foreach(SiteString ssThat in that.m_sites) { SiteString ssInt = (SiteString)ssThis.Intersect(ssThat); if(ssInt != null) alSites.Add(ssInt); } } if(alSites.Count == 0) return null; SiteIdentityPermission result = new SiteIdentityPermission(PermissionState.None); result.m_sites = (SiteString[])alSites.ToArray(typeof(SiteString)); return result; } public override IPermission Union(IPermission target) { if (target == null) { if((this.m_sites == null || this.m_sites.Length == 0) && !this.m_unrestricted) return null; return this.Copy(); } SiteIdentityPermission that = target as SiteIdentityPermission; if(that == null) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName)); if(this.m_unrestricted || that.m_unrestricted) { SiteIdentityPermission res = new SiteIdentityPermission(PermissionState.None); res.m_unrestricted = true; return res; } if (this.m_sites == null || this.m_sites.Length == 0) { if(that.m_sites == null || that.m_sites.Length == 0) return null; return that.Copy(); } if(that.m_sites == null || that.m_sites.Length == 0) return this.Copy(); ArrayList alSites = new ArrayList(); foreach(SiteString ssThis in this.m_sites) alSites.Add(ssThis); foreach(SiteString ssThat in that.m_sites) { bool bDupe = false; foreach(SiteString ss in alSites) { if(ssThat.Equals(ss)) { bDupe = true; break; } } if(!bDupe) alSites.Add(ssThat); } SiteIdentityPermission result = new SiteIdentityPermission(PermissionState.None); result.m_sites = (SiteString[])alSites.ToArray(typeof(SiteString)); return result; } public override void FromXml(SecurityElement esd) { m_unrestricted = false; m_sites = null; CodeAccessPermission.ValidateElement( esd, this ); String unr = esd.Attribute( "Unrestricted" ); if(unr != null && String.Compare(unr, "true", StringComparison.OrdinalIgnoreCase) == 0) { m_unrestricted = true; return; } String elem = esd.Attribute( "Site" ); ArrayList al = new ArrayList(); if(elem != null) al.Add(new SiteString( elem )); ArrayList alChildren = esd.Children; if(alChildren != null) { foreach(SecurityElement child in alChildren) { elem = child.Attribute( "Site" ); if(elem != null) al.Add(new SiteString( elem )); } } if(al.Count != 0) m_sites = (SiteString[])al.ToArray(typeof(SiteString)); } public override SecurityElement ToXml() { SecurityElement esd = CodeAccessPermission.CreatePermissionElement( this, "System.Security.Permissions.SiteIdentityPermission" ); if (m_unrestricted) esd.AddAttribute( "Unrestricted", "true" ); else if (m_sites != null) { if (m_sites.Length == 1) esd.AddAttribute( "Site", m_sites[0].ToString() ); else { int n; for(n = 0; n < m_sites.Length; n++) { SecurityElement child = new SecurityElement("Site"); child.AddAttribute( "Site", m_sites[n].ToString() ); esd.AddChild(child); } } } return esd; } ///int IBuiltInPermission.GetTokenIndex() { return SiteIdentityPermission.GetTokenIndex(); } internal static int GetTokenIndex() { return BuiltInPermissionIndex.SiteIdentityPermissionIndex; } } }
Link Menu
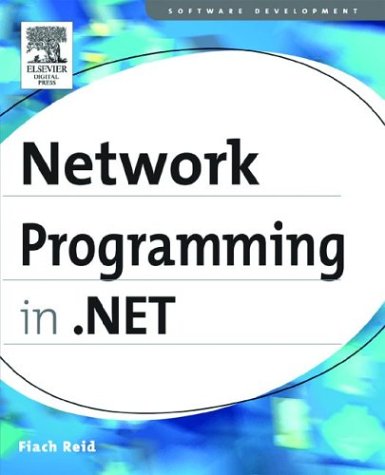
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- bindurihelper.cs
- SchemaImporterExtensionElement.cs
- DiscoveryDocumentReference.cs
- TypeSystem.cs
- MetadataSource.cs
- TemplateBindingExtension.cs
- HijriCalendar.cs
- BinaryMessageEncoder.cs
- ExitEventArgs.cs
- PropertyGridCommands.cs
- RemotingConfigParser.cs
- ResizeGrip.cs
- formatstringdialog.cs
- Operand.cs
- PageParser.cs
- TableSectionStyle.cs
- HierarchicalDataBoundControl.cs
- Misc.cs
- SafeNativeMethods.cs
- HtmlElementEventArgs.cs
- ProviderIncompatibleException.cs
- remotingproxy.cs
- Encoder.cs
- ReflectionServiceProvider.cs
- Triplet.cs
- Grant.cs
- EncryptedReference.cs
- Int32Storage.cs
- DataContract.cs
- MethodExpr.cs
- DataBindEngine.cs
- util.cs
- OrthographicCamera.cs
- PeerSecurityManager.cs
- Control.cs
- CompilerScopeManager.cs
- SqlStatistics.cs
- DeclarativeExpressionConditionDeclaration.cs
- DispatchWrapper.cs
- localization.cs
- SerialPinChanges.cs
- AuthenticationModulesSection.cs
- ResourceDescriptionAttribute.cs
- ComponentConverter.cs
- ConstantExpression.cs
- MimeWriter.cs
- compensatingcollection.cs
- CaseStatement.cs
- TextEffectCollection.cs
- FamilyMapCollection.cs
- TreeChangeInfo.cs
- Script.cs
- ColorContextHelper.cs
- WebScriptEndpoint.cs
- Validator.cs
- SafeLibraryHandle.cs
- WebPartChrome.cs
- PageParser.cs
- Size.cs
- FileSystemWatcher.cs
- DbgCompiler.cs
- ClientRolePrincipal.cs
- Cursors.cs
- HtmlInputImage.cs
- PropertyRecord.cs
- MembershipPasswordException.cs
- UpdateException.cs
- DataColumnMapping.cs
- ExecutedRoutedEventArgs.cs
- ValueType.cs
- StaticResourceExtension.cs
- TypeLibConverter.cs
- SByte.cs
- TemplateField.cs
- DataPointer.cs
- XmlDocumentType.cs
- SoapFormatExtensions.cs
- PagerSettings.cs
- BookmarkScopeHandle.cs
- ConsumerConnectionPointCollection.cs
- AdornerDecorator.cs
- BinaryFormatterWriter.cs
- InputLanguage.cs
- CompiledQuery.cs
- CodePropertyReferenceExpression.cs
- ISAPIApplicationHost.cs
- WinFormsComponentEditor.cs
- XmlSignatureManifest.cs
- ViewGenResults.cs
- METAHEADER.cs
- XsdDuration.cs
- EncryptedReference.cs
- IgnoreSection.cs
- SmiEventSink.cs
- InitializerFacet.cs
- SimpleMailWebEventProvider.cs
- PageClientProxyGenerator.cs
- EndpointAddressMessageFilter.cs
- WorkflowOwnerAsyncResult.cs
- OrderedHashRepartitionStream.cs