Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / DesignTimeData.cs / 1 / DesignTimeData.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Design; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using Microsoft.Win32; using System.Data; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Web.UI; ////// /// Helpers used by control designers to generate sample data /// for use in design time databinding. /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public sealed class DesignTimeData { ////// public static readonly EventHandler DataBindingHandler = new EventHandler(GlobalDataBindingHandler.OnDataBind); /// /// /// private DesignTimeData() { } ////// /// Creates a dummy datatable. /// public static DataTable CreateDummyDataTable() { DataTable dummyDataTable = new DataTable(); dummyDataTable.Locale = CultureInfo.InvariantCulture; DataColumnCollection columns = dummyDataTable.Columns; columns.Add(SR.GetString(SR.Sample_Column, 0), typeof(string)); columns.Add(SR.GetString(SR.Sample_Column, 1), typeof(string)); columns.Add(SR.GetString(SR.Sample_Column, 2), typeof(string)); return dummyDataTable; } ////// Creates a dummy datatable that indicates that the databound control is connected to a datasource. /// public static DataTable CreateDummyDataBoundDataTable() { DataTable dummyDataTable = new DataTable(); dummyDataTable.Locale = CultureInfo.InvariantCulture; DataColumnCollection columns = dummyDataTable.Columns; columns.Add(SR.GetString(SR.Sample_Databound_Column, 0), typeof(string)); columns.Add(SR.GetString(SR.Sample_Databound_Column, 1), typeof(int)); columns.Add(SR.GetString(SR.Sample_Databound_Column, 2), typeof(string)); return dummyDataTable; } ////// /// Creates a sample datatable with same schema as the supplied datasource. /// public static DataTable CreateSampleDataTable(IEnumerable referenceData) { return CreateSampleDataTableInternal(referenceData, false); } public static DataTable CreateSampleDataTable(IEnumerable referenceData, bool useDataBoundData) { return CreateSampleDataTableInternal(referenceData, useDataBoundData); } private static DataTable CreateSampleDataTableInternal(IEnumerable referenceData, bool useDataBoundData) { DataTable sampleDataTable = new DataTable(); sampleDataTable.Locale = CultureInfo.InvariantCulture; DataColumnCollection columns = sampleDataTable.Columns; PropertyDescriptorCollection props = GetDataFields(referenceData); if (props != null) { foreach (PropertyDescriptor propDesc in props) { Type propType = propDesc.PropertyType; if ((propType.IsPrimitive == false) && (propType != typeof(DateTime)) && (propType != typeof(Decimal))) { // we can't handle any remaining or custom types, so // we'll have to create a column of type string in the // design time table. // propType = typeof(string); } columns.Add(propDesc.Name, propType); } } if (columns.Count != 0) { return sampleDataTable; } else { if (useDataBoundData) { return CreateDummyDataBoundDataTable(); } return CreateDummyDataTable(); } } ////// /// public static PropertyDescriptorCollection GetDataFields(IEnumerable dataSource) { if (dataSource is ITypedList) { return ((ITypedList)dataSource).GetItemProperties(new PropertyDescriptor[0]); } Type dataSourceType = dataSource.GetType(); PropertyInfo itemProp = dataSourceType.GetProperty("Item", BindingFlags.Public | BindingFlags.Instance | BindingFlags.Static, null, null, new Type[] { typeof(int) }, null); if ((itemProp != null) && (itemProp.PropertyType != typeof(object))) { return TypeDescriptor.GetProperties(itemProp.PropertyType); } return null; } ////// /// public static string[] GetDataMembers(object dataSource) { IListSource listSource = dataSource as IListSource; if ((listSource != null) && listSource.ContainsListCollection) { IList memberList = ((IListSource)dataSource).GetList(); Debug.Assert(memberList != null, "Got back null from IListSource"); ITypedList typedList = memberList as ITypedList; if (typedList != null) { PropertyDescriptorCollection props = typedList.GetItemProperties(new PropertyDescriptor[0]); if (props != null) { ArrayList members = new ArrayList(props.Count); foreach (PropertyDescriptor pd in props) { members.Add(pd.Name); } return (string[])members.ToArray(typeof(string)); } } } return null; } ////// /// public static IEnumerable GetDataMember(IListSource dataSource, string dataMember) { IEnumerable list = null; IList memberList = dataSource.GetList(); if ((memberList != null) && (memberList is ITypedList)) { if (dataSource.ContainsListCollection == false) { Debug.Assert((dataMember == null) || (dataMember.Length == 0), "List does not contain data members"); if ((dataMember != null) && (dataMember.Length != 0)) { throw new ArgumentException(SR.GetString(SR.DesignTimeData_BadDataMember)); } list = (IEnumerable)memberList; } else { ITypedList typedMemberList = (ITypedList)memberList; PropertyDescriptorCollection propDescs = typedMemberList.GetItemProperties(new PropertyDescriptor[0]); if ((propDescs != null) && (propDescs.Count != 0)) { PropertyDescriptor listProperty = null; if ((dataMember == null) || (dataMember.Length == 0)) { listProperty = propDescs[0]; } else { listProperty = propDescs.Find(dataMember, true); } if (listProperty != null) { object listRow = memberList[0]; object listObject = listProperty.GetValue(listRow); if ((listObject != null) && (listObject is IEnumerable)) { list = (IEnumerable)listObject; } } } } } return list; } ////// /// Adds sample rows into the specified datatable, and returns a cursor on top of it. /// public static IEnumerable GetDesignTimeDataSource(DataTable dataTable, int minimumRows) { DataView dv = null; int rowCount = dataTable.Rows.Count; if (rowCount < minimumRows) { int rowsToAdd = minimumRows - rowCount; DataRowCollection rows = dataTable.Rows; DataColumnCollection columns = dataTable.Columns; int columnCount = columns.Count; DataRow[] rowsArray = new DataRow[rowsToAdd]; // add the sample rows for (int i = 0; i < rowsToAdd; i++) { DataRow row = dataTable.NewRow(); int rowIndex = rowCount + i; for (int c = 0; c < columnCount; c++) { Type dataType = columns[c].DataType; Object obj = null; if (dataType == typeof(String)) { obj = SR.GetString(SR.Sample_Databound_Text_Alt); } else if ((dataType == typeof(Int32)) || (dataType == typeof(Int16)) || (dataType == typeof(Int64)) || (dataType == typeof(UInt32)) || (dataType == typeof(UInt16)) || (dataType == typeof(UInt64))) { obj = rowIndex; } else if ((dataType == typeof(Byte)) || (dataType == typeof(SByte))) { obj = (rowIndex % 2) != 0 ? 1 : 0; } else if (dataType == typeof(Boolean)) { obj = (rowIndex % 2) != 0 ? true : false; } else if (dataType == typeof(DateTime)) { obj = DateTime.Today; } else if ((dataType == typeof(Double)) || (dataType == typeof(Single)) || (dataType == typeof(Decimal))) { obj = i / 10.0; } else if (dataType == typeof(Char)) { obj = 'x'; } else { Debug.Assert(false, "Unexpected type of column in design time datatable."); obj = System.DBNull.Value; } row[c] = obj; } rows.Add(row); } } // create a DataView on top of the effective design time datasource dv = new DataView(dataTable); return dv; } ////// /// public static object GetSelectedDataSource(IComponent component, string dataSource) { object selectedDataSource = null; ISite componentSite = component.Site; if (componentSite != null) { IContainer container = (IContainer)componentSite.GetService(typeof(IContainer)); if (container != null) { IComponent comp = container.Components[dataSource]; if ((comp is IEnumerable) || (comp is IListSource)) { selectedDataSource = comp; } } } return selectedDataSource; } ////// /// public static IEnumerable GetSelectedDataSource(IComponent component, string dataSource, string dataMember) { IEnumerable selectedDataSource = null; object selectedDataSourceObject = DesignTimeData.GetSelectedDataSource(component, dataSource); if (selectedDataSourceObject != null) { IListSource listSource = selectedDataSourceObject as IListSource; if (listSource != null) { if (listSource.ContainsListCollection == false) { // the returned list is itself the list we want to bind to selectedDataSource = (IEnumerable)listSource.GetList(); } else { selectedDataSource = GetDataMember(listSource, dataMember); } } else { Debug.Assert(selectedDataSourceObject is IEnumerable); selectedDataSource = (IEnumerable)selectedDataSourceObject; } } return selectedDataSource; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
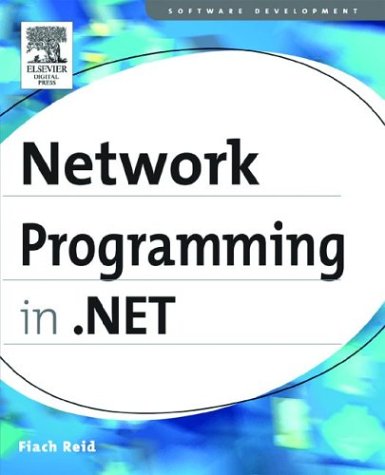
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GlobalizationAssembly.cs
- ProtocolViolationException.cs
- AnnotationMap.cs
- StateMachineDesignerPaint.cs
- SapiInterop.cs
- PathSegment.cs
- columnmapkeybuilder.cs
- FontUnit.cs
- CompositeDuplexBindingElement.cs
- RectAnimationUsingKeyFrames.cs
- CatalogPart.cs
- Executor.cs
- XamlReaderHelper.cs
- DataObjectEventArgs.cs
- DbCommandTree.cs
- FormsAuthenticationEventArgs.cs
- HwndSource.cs
- XPathEmptyIterator.cs
- TableLayout.cs
- XmlCodeExporter.cs
- ChildrenQuery.cs
- DataSpaceManager.cs
- VScrollBar.cs
- WindowsHyperlink.cs
- templategroup.cs
- SqlDataSourceFilteringEventArgs.cs
- FileRecordSequenceCompletedAsyncResult.cs
- PropertyEmitter.cs
- VerifyHashRequest.cs
- ExtensibleClassFactory.cs
- FixedDocumentSequencePaginator.cs
- DataGridView.cs
- AspNetSynchronizationContext.cs
- EdmComplexPropertyAttribute.cs
- SchemaImporter.cs
- PropertyValueUIItem.cs
- BaseTemplateBuildProvider.cs
- UInt32Converter.cs
- EmbossBitmapEffect.cs
- ProjectionPruner.cs
- ConfigXmlDocument.cs
- StyleReferenceConverter.cs
- CopyCodeAction.cs
- EpmSyndicationContentDeSerializer.cs
- DataServiceQueryOfT.cs
- IncrementalCompileAnalyzer.cs
- SiteMapSection.cs
- UnsafePeerToPeerMethods.cs
- WindowsClaimSet.cs
- Win32Exception.cs
- TemplateColumn.cs
- MetadataWorkspace.cs
- IODescriptionAttribute.cs
- IDReferencePropertyAttribute.cs
- Wizard.cs
- EventListener.cs
- WebPartCollection.cs
- PersistenceParticipant.cs
- ProfileSettingsCollection.cs
- InputReportEventArgs.cs
- ShaperBuffers.cs
- CssTextWriter.cs
- IdentitySection.cs
- InternalConfigRoot.cs
- LinkedResource.cs
- GroupBoxAutomationPeer.cs
- TCPClient.cs
- PageThemeBuildProvider.cs
- StyleSelector.cs
- Util.cs
- ExtractorMetadata.cs
- ManagementBaseObject.cs
- Profiler.cs
- SqlCommand.cs
- ContentElementAutomationPeer.cs
- HashHelper.cs
- GeneralTransform3DGroup.cs
- SortDescription.cs
- CodeMemberEvent.cs
- EventDescriptor.cs
- SqlDataSourceQueryEditorForm.cs
- Error.cs
- OneOfScalarConst.cs
- ICollection.cs
- DataGridCheckBoxColumn.cs
- CompiledIdentityConstraint.cs
- Point.cs
- sqlstateclientmanager.cs
- TextServicesCompartment.cs
- HtmlElementEventArgs.cs
- XmlILIndex.cs
- TdsParserHelperClasses.cs
- SQLChars.cs
- JsonServiceDocumentSerializer.cs
- WebAdminConfigurationHelper.cs
- WebBrowserHelper.cs
- Thread.cs
- DiagnosticStrings.cs
- sqlstateclientmanager.cs
- EncryptedKey.cs