Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / SingleAnimation.cs / 1 / SingleAnimation.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using MS.Internal.PresentationCore; namespace System.Windows.Media.Animation { ////// Animates the value of a Single property using linear interpolation /// between two values. The values are determined by the combination of /// From, To, or By values that are set on the animation. /// public partial class SingleAnimation : SingleAnimationBase { #region Data ////// This is used if the user has specified From, To, and/or By values. /// private Single[] _keyValues; private AnimationType _animationType; private bool _isAnimationFunctionValid; #endregion #region Constructors ////// Static ctor for SingleAnimation establishes /// dependency properties, using as much shared data as possible. /// static SingleAnimation() { Type typeofProp = typeof(Single?); Type typeofThis = typeof(SingleAnimation); PropertyChangedCallback propCallback = new PropertyChangedCallback(AnimationFunction_Changed); ValidateValueCallback validateCallback = new ValidateValueCallback(ValidateFromToOrByValue); FromProperty = DependencyProperty.Register( "From", typeofProp, typeofThis, new PropertyMetadata((Single?)null, propCallback), validateCallback); ToProperty = DependencyProperty.Register( "To", typeofProp, typeofThis, new PropertyMetadata((Single?)null, propCallback), validateCallback); ByProperty = DependencyProperty.Register( "By", typeofProp, typeofThis, new PropertyMetadata((Single?)null, propCallback), validateCallback); } ////// Creates a new SingleAnimation with all properties set to /// their default values. /// public SingleAnimation() : base() { } ////// Creates a new SingleAnimation that will animate a /// Single property from its base value to the value specified /// by the "toValue" parameter of this constructor. /// public SingleAnimation(Single toValue, Duration duration) : this() { To = toValue; Duration = duration; } ////// Creates a new SingleAnimation that will animate a /// Single property from its base value to the value specified /// by the "toValue" parameter of this constructor. /// public SingleAnimation(Single toValue, Duration duration, FillBehavior fillBehavior) : this() { To = toValue; Duration = duration; FillBehavior = fillBehavior; } ////// Creates a new SingleAnimation that will animate a /// Single property from the "fromValue" parameter of this constructor /// to the "toValue" parameter. /// public SingleAnimation(Single fromValue, Single toValue, Duration duration) : this() { From = fromValue; To = toValue; Duration = duration; } ////// Creates a new SingleAnimation that will animate a /// Single property from the "fromValue" parameter of this constructor /// to the "toValue" parameter. /// public SingleAnimation(Single fromValue, Single toValue, Duration duration, FillBehavior fillBehavior) : this() { From = fromValue; To = toValue; Duration = duration; FillBehavior = fillBehavior; } #endregion #region Freezable ////// Creates a copy of this SingleAnimation /// ///The copy public new SingleAnimation Clone() { return (SingleAnimation)base.Clone(); } // // Note that we don't override the Clone virtuals (CloneCore, CloneCurrentValueCore, // GetAsFrozenCore, and GetCurrentValueAsFrozenCore) even though this class has state // not stored in a DP. // // We don't need to clone _animationType and _keyValues because they are the the cached // results of animation function validation, which can be recomputed. The other remaining // field, isAnimationFunctionValid, defaults to false, which causes this recomputation to happen. // ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new SingleAnimation(); } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected override Single GetCurrentValueCore(Single defaultOriginValue, Single defaultDestinationValue, AnimationClock animationClock) { Debug.Assert(animationClock.CurrentState != ClockState.Stopped); if (!_isAnimationFunctionValid) { ValidateAnimationFunction(); } double progress = animationClock.CurrentProgress.Value; Single from = new Single(); Single to = new Single(); Single accumulated = new Single(); Single foundation = new Single(); // need to validate the default origin and destination values if // the animation uses them as the from, to, or foundation values bool validateOrigin = false; bool validateDestination = false; switch(_animationType) { case AnimationType.Automatic: from = defaultOriginValue; to = defaultDestinationValue; validateOrigin = true; validateDestination = true; break; case AnimationType.From: from = _keyValues[0]; to = defaultDestinationValue; validateDestination = true; break; case AnimationType.To: from = defaultOriginValue; to = _keyValues[0]; validateOrigin = true; break; case AnimationType.By: // According to the SMIL specification, a By animation is // always additive. But we don't force this so that a // user can re-use a By animation and have it replace the // animations that precede it in the list without having // to manually set the From value to the base value. to = _keyValues[0]; foundation = defaultOriginValue; validateOrigin = true; break; case AnimationType.FromTo: from = _keyValues[0]; to = _keyValues[1]; if (IsAdditive) { foundation = defaultOriginValue; validateOrigin = true; } break; case AnimationType.FromBy: from = _keyValues[0]; to = AnimatedTypeHelpers.AddSingle(_keyValues[0], _keyValues[1]); if (IsAdditive) { foundation = defaultOriginValue; validateOrigin = true; } break; default: Debug.Fail("Unknown animation type."); break; } if (validateOrigin && !AnimatedTypeHelpers.IsValidAnimationValueSingle(defaultOriginValue)) { throw new InvalidOperationException( SR.Get( SRID.Animation_Invalid_DefaultValue, this.GetType(), "origin", defaultOriginValue.ToString(CultureInfo.InvariantCulture))); } if (validateDestination && !AnimatedTypeHelpers.IsValidAnimationValueSingle(defaultDestinationValue)) { throw new InvalidOperationException( SR.Get( SRID.Animation_Invalid_DefaultValue, this.GetType(), "destination", defaultDestinationValue.ToString(CultureInfo.InvariantCulture))); } if (IsCumulative) { double currentRepeat = (double)(animationClock.CurrentIteration - 1); if (currentRepeat > 0.0) { Single accumulator = AnimatedTypeHelpers.SubtractSingle(to, from); accumulated = AnimatedTypeHelpers.ScaleSingle(accumulator, currentRepeat); } } // return foundation + accumulated + from + ((to - from) * progress) return AnimatedTypeHelpers.AddSingle( foundation, AnimatedTypeHelpers.AddSingle( accumulated, AnimatedTypeHelpers.InterpolateSingle(from, to, progress))); } private void ValidateAnimationFunction() { _animationType = AnimationType.Automatic; _keyValues = null; if (From.HasValue) { if (To.HasValue) { _animationType = AnimationType.FromTo; _keyValues = new Single[2]; _keyValues[0] = From.Value; _keyValues[1] = To.Value; } else if (By.HasValue) { _animationType = AnimationType.FromBy; _keyValues = new Single[2]; _keyValues[0] = From.Value; _keyValues[1] = By.Value; } else { _animationType = AnimationType.From; _keyValues = new Single[1]; _keyValues[0] = From.Value; } } else if (To.HasValue) { _animationType = AnimationType.To; _keyValues = new Single[1]; _keyValues[0] = To.Value; } else if (By.HasValue) { _animationType = AnimationType.By; _keyValues = new Single[1]; _keyValues[0] = By.Value; } _isAnimationFunctionValid = true; } #endregion #region Properties private static void AnimationFunction_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { SingleAnimation a = (SingleAnimation)d; a._isAnimationFunctionValid = false; a.PropertyChanged(e.Property); } private static bool ValidateFromToOrByValue(object value) { Single? typedValue = (Single?)value; if (typedValue.HasValue) { return AnimatedTypeHelpers.IsValidAnimationValueSingle(typedValue.Value); } else { return true; } } ////// FromProperty /// public static readonly DependencyProperty FromProperty; ////// From /// public Single? From { get { return (Single?)GetValue(FromProperty); } set { SetValueInternal(FromProperty, value); } } ////// ToProperty /// public static readonly DependencyProperty ToProperty; ////// To /// public Single? To { get { return (Single?)GetValue(ToProperty); } set { SetValueInternal(ToProperty, value); } } ////// ByProperty /// public static readonly DependencyProperty ByProperty; ////// By /// public Single? By { get { return (Single?)GetValue(ByProperty); } set { SetValueInternal(ByProperty, value); } } ////// If this property is set to true the animation will add its value to /// the base value instead of replacing it entirely. /// public bool IsAdditive { get { return (bool)GetValue(IsAdditiveProperty); } set { SetValueInternal(IsAdditiveProperty, BooleanBoxes.Box(value)); } } ////// It this property is set to true, the animation will accumulate its /// value over repeats. For instance if you have a From value of 0.0 and /// a To value of 1.0, the animation return values from 1.0 to 2.0 over /// the second reteat cycle, and 2.0 to 3.0 over the third, etc. /// public bool IsCumulative { get { return (bool)GetValue(IsCumulativeProperty); } set { SetValueInternal(IsCumulativeProperty, BooleanBoxes.Box(value)); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using MS.Internal.PresentationCore; namespace System.Windows.Media.Animation { ////// Animates the value of a Single property using linear interpolation /// between two values. The values are determined by the combination of /// From, To, or By values that are set on the animation. /// public partial class SingleAnimation : SingleAnimationBase { #region Data ////// This is used if the user has specified From, To, and/or By values. /// private Single[] _keyValues; private AnimationType _animationType; private bool _isAnimationFunctionValid; #endregion #region Constructors ////// Static ctor for SingleAnimation establishes /// dependency properties, using as much shared data as possible. /// static SingleAnimation() { Type typeofProp = typeof(Single?); Type typeofThis = typeof(SingleAnimation); PropertyChangedCallback propCallback = new PropertyChangedCallback(AnimationFunction_Changed); ValidateValueCallback validateCallback = new ValidateValueCallback(ValidateFromToOrByValue); FromProperty = DependencyProperty.Register( "From", typeofProp, typeofThis, new PropertyMetadata((Single?)null, propCallback), validateCallback); ToProperty = DependencyProperty.Register( "To", typeofProp, typeofThis, new PropertyMetadata((Single?)null, propCallback), validateCallback); ByProperty = DependencyProperty.Register( "By", typeofProp, typeofThis, new PropertyMetadata((Single?)null, propCallback), validateCallback); } ////// Creates a new SingleAnimation with all properties set to /// their default values. /// public SingleAnimation() : base() { } ////// Creates a new SingleAnimation that will animate a /// Single property from its base value to the value specified /// by the "toValue" parameter of this constructor. /// public SingleAnimation(Single toValue, Duration duration) : this() { To = toValue; Duration = duration; } ////// Creates a new SingleAnimation that will animate a /// Single property from its base value to the value specified /// by the "toValue" parameter of this constructor. /// public SingleAnimation(Single toValue, Duration duration, FillBehavior fillBehavior) : this() { To = toValue; Duration = duration; FillBehavior = fillBehavior; } ////// Creates a new SingleAnimation that will animate a /// Single property from the "fromValue" parameter of this constructor /// to the "toValue" parameter. /// public SingleAnimation(Single fromValue, Single toValue, Duration duration) : this() { From = fromValue; To = toValue; Duration = duration; } ////// Creates a new SingleAnimation that will animate a /// Single property from the "fromValue" parameter of this constructor /// to the "toValue" parameter. /// public SingleAnimation(Single fromValue, Single toValue, Duration duration, FillBehavior fillBehavior) : this() { From = fromValue; To = toValue; Duration = duration; FillBehavior = fillBehavior; } #endregion #region Freezable ////// Creates a copy of this SingleAnimation /// ///The copy public new SingleAnimation Clone() { return (SingleAnimation)base.Clone(); } // // Note that we don't override the Clone virtuals (CloneCore, CloneCurrentValueCore, // GetAsFrozenCore, and GetCurrentValueAsFrozenCore) even though this class has state // not stored in a DP. // // We don't need to clone _animationType and _keyValues because they are the the cached // results of animation function validation, which can be recomputed. The other remaining // field, isAnimationFunctionValid, defaults to false, which causes this recomputation to happen. // ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new SingleAnimation(); } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected override Single GetCurrentValueCore(Single defaultOriginValue, Single defaultDestinationValue, AnimationClock animationClock) { Debug.Assert(animationClock.CurrentState != ClockState.Stopped); if (!_isAnimationFunctionValid) { ValidateAnimationFunction(); } double progress = animationClock.CurrentProgress.Value; Single from = new Single(); Single to = new Single(); Single accumulated = new Single(); Single foundation = new Single(); // need to validate the default origin and destination values if // the animation uses them as the from, to, or foundation values bool validateOrigin = false; bool validateDestination = false; switch(_animationType) { case AnimationType.Automatic: from = defaultOriginValue; to = defaultDestinationValue; validateOrigin = true; validateDestination = true; break; case AnimationType.From: from = _keyValues[0]; to = defaultDestinationValue; validateDestination = true; break; case AnimationType.To: from = defaultOriginValue; to = _keyValues[0]; validateOrigin = true; break; case AnimationType.By: // According to the SMIL specification, a By animation is // always additive. But we don't force this so that a // user can re-use a By animation and have it replace the // animations that precede it in the list without having // to manually set the From value to the base value. to = _keyValues[0]; foundation = defaultOriginValue; validateOrigin = true; break; case AnimationType.FromTo: from = _keyValues[0]; to = _keyValues[1]; if (IsAdditive) { foundation = defaultOriginValue; validateOrigin = true; } break; case AnimationType.FromBy: from = _keyValues[0]; to = AnimatedTypeHelpers.AddSingle(_keyValues[0], _keyValues[1]); if (IsAdditive) { foundation = defaultOriginValue; validateOrigin = true; } break; default: Debug.Fail("Unknown animation type."); break; } if (validateOrigin && !AnimatedTypeHelpers.IsValidAnimationValueSingle(defaultOriginValue)) { throw new InvalidOperationException( SR.Get( SRID.Animation_Invalid_DefaultValue, this.GetType(), "origin", defaultOriginValue.ToString(CultureInfo.InvariantCulture))); } if (validateDestination && !AnimatedTypeHelpers.IsValidAnimationValueSingle(defaultDestinationValue)) { throw new InvalidOperationException( SR.Get( SRID.Animation_Invalid_DefaultValue, this.GetType(), "destination", defaultDestinationValue.ToString(CultureInfo.InvariantCulture))); } if (IsCumulative) { double currentRepeat = (double)(animationClock.CurrentIteration - 1); if (currentRepeat > 0.0) { Single accumulator = AnimatedTypeHelpers.SubtractSingle(to, from); accumulated = AnimatedTypeHelpers.ScaleSingle(accumulator, currentRepeat); } } // return foundation + accumulated + from + ((to - from) * progress) return AnimatedTypeHelpers.AddSingle( foundation, AnimatedTypeHelpers.AddSingle( accumulated, AnimatedTypeHelpers.InterpolateSingle(from, to, progress))); } private void ValidateAnimationFunction() { _animationType = AnimationType.Automatic; _keyValues = null; if (From.HasValue) { if (To.HasValue) { _animationType = AnimationType.FromTo; _keyValues = new Single[2]; _keyValues[0] = From.Value; _keyValues[1] = To.Value; } else if (By.HasValue) { _animationType = AnimationType.FromBy; _keyValues = new Single[2]; _keyValues[0] = From.Value; _keyValues[1] = By.Value; } else { _animationType = AnimationType.From; _keyValues = new Single[1]; _keyValues[0] = From.Value; } } else if (To.HasValue) { _animationType = AnimationType.To; _keyValues = new Single[1]; _keyValues[0] = To.Value; } else if (By.HasValue) { _animationType = AnimationType.By; _keyValues = new Single[1]; _keyValues[0] = By.Value; } _isAnimationFunctionValid = true; } #endregion #region Properties private static void AnimationFunction_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { SingleAnimation a = (SingleAnimation)d; a._isAnimationFunctionValid = false; a.PropertyChanged(e.Property); } private static bool ValidateFromToOrByValue(object value) { Single? typedValue = (Single?)value; if (typedValue.HasValue) { return AnimatedTypeHelpers.IsValidAnimationValueSingle(typedValue.Value); } else { return true; } } ////// FromProperty /// public static readonly DependencyProperty FromProperty; ////// From /// public Single? From { get { return (Single?)GetValue(FromProperty); } set { SetValueInternal(FromProperty, value); } } ////// ToProperty /// public static readonly DependencyProperty ToProperty; ////// To /// public Single? To { get { return (Single?)GetValue(ToProperty); } set { SetValueInternal(ToProperty, value); } } ////// ByProperty /// public static readonly DependencyProperty ByProperty; ////// By /// public Single? By { get { return (Single?)GetValue(ByProperty); } set { SetValueInternal(ByProperty, value); } } ////// If this property is set to true the animation will add its value to /// the base value instead of replacing it entirely. /// public bool IsAdditive { get { return (bool)GetValue(IsAdditiveProperty); } set { SetValueInternal(IsAdditiveProperty, BooleanBoxes.Box(value)); } } ////// It this property is set to true, the animation will accumulate its /// value over repeats. For instance if you have a From value of 0.0 and /// a To value of 1.0, the animation return values from 1.0 to 2.0 over /// the second reteat cycle, and 2.0 to 3.0 over the third, etc. /// public bool IsCumulative { get { return (bool)GetValue(IsCumulativeProperty); } set { SetValueInternal(IsCumulativeProperty, BooleanBoxes.Box(value)); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
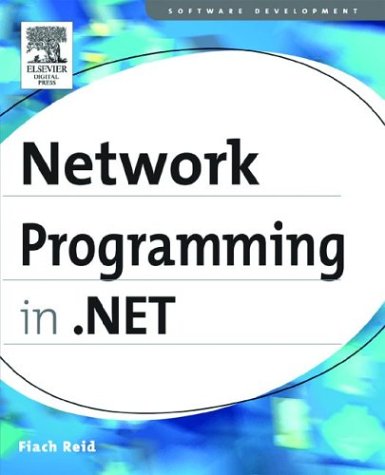
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- userdatakeys.cs
- ServiceDurableInstance.cs
- SettingsSection.cs
- Bezier.cs
- EntityDataSourceMemberPath.cs
- WorkItem.cs
- InputManager.cs
- ShapingEngine.cs
- ProcessInputEventArgs.cs
- FillBehavior.cs
- shaperfactoryquerycachekey.cs
- _SafeNetHandles.cs
- AnnotationHelper.cs
- EnumConverter.cs
- TextWriterEngine.cs
- DbParameterHelper.cs
- PreProcessor.cs
- Dynamic.cs
- DynamicResourceExtensionConverter.cs
- AppDomainInfo.cs
- CompositeFontParser.cs
- SqlProvider.cs
- StylusPointDescription.cs
- Camera.cs
- Stopwatch.cs
- ContainerSelectorBehavior.cs
- SiteMapNodeCollection.cs
- BuilderElements.cs
- ReturnValue.cs
- ObfuscateAssemblyAttribute.cs
- CompilationLock.cs
- BinaryFormatterWriter.cs
- DispatcherExceptionFilterEventArgs.cs
- GenerateTemporaryTargetAssembly.cs
- StyleConverter.cs
- SafeFileMappingHandle.cs
- ToolStripContentPanel.cs
- EntityExpressionVisitor.cs
- XmlDataSource.cs
- Opcode.cs
- MDIControlStrip.cs
- APCustomTypeDescriptor.cs
- WindowsPrincipal.cs
- RequestStatusBarUpdateEventArgs.cs
- ListViewInsertEventArgs.cs
- ExceptionUtil.cs
- SessionEndedEventArgs.cs
- BitmapFrameDecode.cs
- Calendar.cs
- XomlCompilerError.cs
- __TransparentProxy.cs
- ImageButton.cs
- ListViewGroupConverter.cs
- HostingEnvironmentSection.cs
- XamlGridLengthSerializer.cs
- SafeEventLogReadHandle.cs
- XPathExpr.cs
- BindMarkupExtensionSerializer.cs
- FontWeight.cs
- WindowsSysHeader.cs
- IdentitySection.cs
- DataGridViewElement.cs
- ArrayConverter.cs
- CngProvider.cs
- X509CertificateCollection.cs
- EventMap.cs
- XmlNamespaceDeclarationsAttribute.cs
- _ServiceNameStore.cs
- HitTestWithGeometryDrawingContextWalker.cs
- Rectangle.cs
- DataSourceXmlTextReader.cs
- AxDesigner.cs
- EncryptedPackage.cs
- RtfNavigator.cs
- HashHelper.cs
- DataGridPagerStyle.cs
- LookupNode.cs
- MenuTracker.cs
- PersonalizablePropertyEntry.cs
- FontEmbeddingManager.cs
- FullTextBreakpoint.cs
- SmtpNegotiateAuthenticationModule.cs
- StateValidator.cs
- DataContractSerializerSection.cs
- WebPartPersonalization.cs
- CodeDirectiveCollection.cs
- InvalidDataException.cs
- FormViewUpdateEventArgs.cs
- LoginCancelEventArgs.cs
- InternalRelationshipCollection.cs
- TitleStyle.cs
- RedBlackList.cs
- FormViewInsertedEventArgs.cs
- XmlTextWriter.cs
- XmlKeywords.cs
- ExtendedTransformFactory.cs
- SafeArrayRankMismatchException.cs
- SchemaSetCompiler.cs
- ContactManager.cs
- DrawToolTipEventArgs.cs