Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / xsp / System / Web / Extensions / resources / AtlasWeb.Designer.cs / 2 / AtlasWeb.Designer.cs
//------------------------------------------------------------------------------ //// This code was generated by a tool. // Runtime Version:2.0.50727.1433 // // Changes to this file may cause incorrect behavior and will be lost if // the code is regenerated. // //----------------------------------------------------------------------------- namespace System.Web.Resources { using System; ////// A strongly-typed resource class, for looking up localized strings, etc. /// // This class was auto-generated by the StronglyTypedResourceBuilder // class via a tool like ResGen or Visual Studio. // To add or remove a member, edit your .ResX file then rerun ResGen // with the /str option, or rebuild your VS project. [global::System.CodeDom.Compiler.GeneratedCodeAttribute("System.Resources.Tools.StronglyTypedResourceBuilder", "2.0.0.0")] [global::System.Diagnostics.DebuggerNonUserCodeAttribute()] [global::System.Runtime.CompilerServices.CompilerGeneratedAttribute()] internal class AtlasWeb { private static global::System.Resources.ResourceManager resourceMan; private static global::System.Globalization.CultureInfo resourceCulture; [global::System.Diagnostics.CodeAnalysis.SuppressMessageAttribute("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal AtlasWeb() { } ////// Returns the cached ResourceManager instance used by this class. /// [global::System.ComponentModel.EditorBrowsableAttribute(global::System.ComponentModel.EditorBrowsableState.Advanced)] internal static global::System.Resources.ResourceManager ResourceManager { get { if (object.ReferenceEquals(resourceMan, null)) { global::System.Resources.ResourceManager temp = new global::System.Resources.ResourceManager("System.Web.Resources.AtlasWeb", typeof(AtlasWeb).Assembly); resourceMan = temp; } return resourceMan; } } ////// Overrides the current thread's CurrentUICulture property for all /// resource lookups using this strongly typed resource class. /// [global::System.ComponentModel.EditorBrowsableAttribute(global::System.ComponentModel.EditorBrowsableState.Advanced)] internal static global::System.Globalization.CultureInfo Culture { get { return resourceCulture; } set { resourceCulture = value; } } ////// Looks up a localized string similar to Specifies the path to the web service.. /// internal static string ApplicationServiceManager_Path { get { return ResourceManager.GetString("ApplicationServiceManager_Path", resourceCulture); } } ////// Looks up a localized string similar to {0} is disabled.. /// internal static string AppService_Disabled { get { return ResourceManager.GetString("AppService_Disabled", resourceCulture); } } ////// Looks up a localized string similar to Cannot specify more than one unique path.. /// internal static string AppService_MultiplePaths { get { return ResourceManager.GetString("AppService_MultiplePaths", resourceCulture); } } ////// Looks up a localized string similar to SSL is required for this operation.. /// internal static string AppService_RequiredSSL { get { return ResourceManager.GetString("AppService_RequiredSSL", resourceCulture); } } ////// Looks up a localized string similar to Unknown profile property '{0}'.. /// internal static string AppService_UnknownProfileProperty { get { return ResourceManager.GetString("AppService_UnknownProfileProperty", resourceCulture); } } ////// Looks up a localized string similar to Argument must be null, empty or same as the current user.. /// internal static string ArgumentMustBeCurrentUser { get { return ResourceManager.GetString("ArgumentMustBeCurrentUser", resourceCulture); } } ////// Looks up a localized string similar to Argument must be null or empty.. /// internal static string ArgumentMustBeNull { get { return ResourceManager.GetString("ArgumentMustBeNull", resourceCulture); } } ////// Looks up a localized string similar to Could not find an event named '{0}' on associated control '{1}' for the trigger in UpdatePanel '{2}'.. /// internal static string AsyncPostBackTrigger_CannotFindEvent { get { return ResourceManager.GetString("AsyncPostBackTrigger_CannotFindEvent", resourceCulture); } } ////// Looks up a localized string similar to The event that the trigger will hook up to determine whether to refresh the UpdatePanel. If the property is not set then the UpdatePanel will be refreshed only if the postback was initiated by the target control.. /// internal static string AsyncPostBackTrigger_EventName { get { return ResourceManager.GetString("AsyncPostBackTrigger_EventName", resourceCulture); } } ////// Looks up a localized string similar to The '{0}' event on associated control '{1}' for the trigger in UpdatePanel '{2}' does not match the standard event handler signature.. /// internal static string AsyncPostBackTrigger_InvalidEvent { get { return ResourceManager.GetString("AsyncPostBackTrigger_InvalidEvent", resourceCulture); } } ////// Looks up a localized string similar to The following configuration attribute was not recognized: '{0}'. /// internal static string AttributeNotRecognized { get { return ResourceManager.GetString("AttributeNotRecognized", resourceCulture); } } ////// Looks up a localized string similar to Sorting. /// internal static string Category_Sorting { get { return ResourceManager.GetString("Category_Sorting", resourceCulture); } } ////// Looks up a localized string similar to The server method returned invalid data.. /// internal static string ClientService_BadJsonResponse { get { return ResourceManager.GetString("ClientService_BadJsonResponse", resourceCulture); } } ////// Looks up a localized string similar to Value must be of type '{0}'.. /// internal static string Common_ArgumentInvalidType { get { return ResourceManager.GetString("Common_ArgumentInvalidType", resourceCulture); } } ////// Looks up a localized string similar to Value must be greater than or equal to 0.. /// internal static string Common_GreaterThanOrEqualToZero { get { return ResourceManager.GetString("Common_GreaterThanOrEqualToZero", resourceCulture); } } ////// Looks up a localized string similar to Value must be greater than or equal to 0 and less than or equal to 1.. /// internal static string Common_GreaterThanOrEqualToZeroAndLessThanOrEqualToOne { get { return ResourceManager.GetString("Common_GreaterThanOrEqualToZeroAndLessThanOrEqualToOne", resourceCulture); } } ////// Looks up a localized string similar to Value cannot be null or empty.. /// internal static string Common_NullOrEmpty { get { return ResourceManager.GetString("Common_NullOrEmpty", resourceCulture); } } ////// Looks up a localized string similar to Page cannot be null. Please ensure that this operation is being performed in the context of an ASP.NET request.. /// internal static string Common_PageCannotBeNull { get { return ResourceManager.GetString("Common_PageCannotBeNull", resourceCulture); } } ////// Looks up a localized string similar to The control with ID '{0}' requires a ScriptManager on the page. The ScriptManager must appear before any controls that need it.. /// internal static string Common_ScriptManagerRequired { get { return ResourceManager.GetString("Common_ScriptManagerRequired", resourceCulture); } } ////// Looks up a localized string similar to A collection of script references that the CompositeScriptReference should include in the page.. /// internal static string CompositeScriptReference_Scripts { get { return ResourceManager.GetString("CompositeScriptReference_Scripts", resourceCulture); } } ////// Looks up a localized string similar to Type: '{0}' does not inherits from JavaScriptConverter.. /// internal static string ConvertersCollection_NotJavaScriptConverter { get { return ResourceManager.GetString("ConvertersCollection_NotJavaScriptConverter", resourceCulture); } } ////// Looks up a localized string similar to Type: '{0}' cannot be found.. /// internal static string ConvertersCollection_UnknownType { get { return ResourceManager.GetString("ConvertersCollection_UnknownType", resourceCulture); } } ////// Looks up a localized string similar to The {0} control '{1}' does not have a naming container. Ensure that the control is added to the page before calling DataBind.. /// internal static string DataBoundControlHelper_NoNamingContainer { get { return ResourceManager.GetString("DataBoundControlHelper_NoNamingContainer", resourceCulture); } } ////// Looks up a localized string similar to Control '{0}' does not implement IPageableItemContainer.. /// internal static string DataPager_ControlIsntPageable { get { return ResourceManager.GetString("DataPager_ControlIsntPageable", resourceCulture); } } ////// Looks up a localized string similar to The collection of DataPagerFields.. /// internal static string DataPager_Fields { get { return ResourceManager.GetString("DataPager_Fields", resourceCulture); } } ////// Looks up a localized string similar to The DataPager control '{0}' does not have a naming container. Ensure that the DataPager is added to the page before calling DataBind.. /// internal static string DataPager_NoNamingContainer { get { return ResourceManager.GetString("DataPager_NoNamingContainer", resourceCulture); } } ////// Looks up a localized string similar to No IPageableItemContainer was found. Verify that either the DataPager is inside an IPageableItemContainer or PagedControlID is set to the control ID of an IPageableItemContainer.. /// internal static string DataPager_NoPageableItemContainer { get { return ResourceManager.GetString("DataPager_NoPageableItemContainer", resourceCulture); } } ////// Looks up a localized string similar to IPageableItemContainer '{0}' not found.. /// internal static string DataPager_PageableItemContainerNotFound { get { return ResourceManager.GetString("DataPager_PageableItemContainerNotFound", resourceCulture); } } ////// Looks up a localized string similar to The ID of the control this DataPager should page.. /// internal static string DataPager_PagedControlID { get { return ResourceManager.GetString("DataPager_PagedControlID", resourceCulture); } } ////// Looks up a localized string similar to Page properties cannot be set because no IPageableItemContainer has been found.. /// internal static string DataPager_PagePropertiesCannotBeSet { get { return ResourceManager.GetString("DataPager_PagePropertiesCannotBeSet", resourceCulture); } } ////// Looks up a localized string similar to The number of records displayed in a page by the paged control.. /// internal static string DataPager_PageSize { get { return ResourceManager.GetString("DataPager_PageSize", resourceCulture); } } ////// Looks up a localized string similar to The name of the query string field for the current page index. The pager will use the query string when this property is set.. /// internal static string DataPager_QueryStringField { get { return ResourceManager.GetString("DataPager_QueryStringField", resourceCulture); } } ////// Looks up a localized string similar to Whether the data pager field is visible.. /// internal static string DataPagerField_Visible { get { return ResourceManager.GetString("DataPagerField_Visible", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the field value should be converted to a null reference.. /// internal static string DynamicControlBase_ConvertEmptyStringToNull { get { return ResourceManager.GetString("DynamicControlBase_ConvertEmptyStringToNull", resourceCulture); } } ////// Looks up a localized string similar to Specifies the name of the data field to which the DynamicControl will bind.. /// internal static string DynamicControlBase_DataField { get { return ResourceManager.GetString("DynamicControlBase_DataField", resourceCulture); } } ////// Looks up a localized string similar to Specifies the display format for the field value.. /// internal static string DynamicControlBase_DataFormatString { get { return ResourceManager.GetString("DynamicControlBase_DataFormatString", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the field value is HTML-encoded before it is displayed.. /// internal static string DynamicControlBase_HtmlEncode { get { return ResourceManager.GetString("DynamicControlBase_HtmlEncode", resourceCulture); } } ////// Looks up a localized string similar to Specifies the caption displayed when the field value is null.. /// internal static string DynamicControlBase_NullDisplayText { get { return ResourceManager.GetString("DynamicControlBase_NullDisplayText", resourceCulture); } } ////// Looks up a localized string similar to Specifies the user control with which the field should be rendered.. /// internal static string DynamicControlBase_UIHint { get { return ResourceManager.GetString("DynamicControlBase_UIHint", resourceCulture); } } ////// Looks up a localized string similar to Specifies the name of the validation group to which validation controls in the DynamicControl belong.. /// internal static string DynamicControlBase_ValidationGroup { get { return ResourceManager.GetString("DynamicControlBase_ValidationGroup", resourceCulture); } } ////// Looks up a localized string similar to The ID of the DynamicFilter control that exists in the ItemTemplate.. /// internal static string DynamicFilterRepeater_DynamicFilterContainerId { get { return ResourceManager.GetString("DynamicFilterRepeater_DynamicFilterContainerId", resourceCulture); } } ////// Looks up a localized string similar to No accessible tables found. Make sure scaffolds are enabled or custom templates exist for your model.. /// internal static string DynamicNavigatorDataSource_NoAccessibleTablesFound { get { return ResourceManager.GetString("DynamicNavigatorDataSource_NoAccessibleTablesFound", resourceCulture); } } ////// Looks up a localized string similar to No data models have been registered.. /// internal static string DynamicNavigatorDataSource_NoModelsRegistered { get { return ResourceManager.GetString("DynamicNavigatorDataSource_NoModelsRegistered", resourceCulture); } } ////// Looks up a localized string similar to There are no tables defined in the registered data models.. /// internal static string DynamicNavigatorDataSource_NoTablesInModels { get { return ResourceManager.GetString("DynamicNavigatorDataSource_NoTablesInModels", resourceCulture); } } ////// Looks up a localized string similar to Ambiguous invocation of '{0}' constructor. /// internal static string ExpressionParser_AmbiguousConstructorInvocation { get { return ResourceManager.GetString("ExpressionParser_AmbiguousConstructorInvocation", resourceCulture); } } ////// Looks up a localized string similar to Ambiguous invocation of indexer in type '{0}'. /// internal static string ExpressionParser_AmbiguousIndexerInvocation { get { return ResourceManager.GetString("ExpressionParser_AmbiguousIndexerInvocation", resourceCulture); } } ////// Looks up a localized string similar to Ambiguous invocation of method '{0}' in type '{1}'. /// internal static string ExpressionParser_AmbiguousMethodInvocation { get { return ResourceManager.GetString("ExpressionParser_AmbiguousMethodInvocation", resourceCulture); } } ////// Looks up a localized string similar to Argument list incompatible with lambda expression. /// internal static string ExpressionParser_ArgsIncompatibleWithLambda { get { return ResourceManager.GetString("ExpressionParser_ArgsIncompatibleWithLambda", resourceCulture); } } ////// Looks up a localized string similar to Both of the types '{0}' and '{1}' convert to the other. /// internal static string ExpressionParser_BothTypesConvertToOther { get { return ResourceManager.GetString("ExpressionParser_BothTypesConvertToOther", resourceCulture); } } ////// Looks up a localized string similar to A value of type '{0}' cannot be converted to type '{1}'. /// internal static string ExpressionParser_CannotConvertValue { get { return ResourceManager.GetString("ExpressionParser_CannotConvertValue", resourceCulture); } } ////// Looks up a localized string similar to Indexing of multiple-dimensional arrays is not supported. /// internal static string ExpressionParser_CannotIndexMultipleDimensionalArray { get { return ResourceManager.GetString("ExpressionParser_CannotIndexMultipleDimensionalArray", resourceCulture); } } ////// Looks up a localized string similar to ']' or ',' expected. /// internal static string ExpressionParser_CloseBracketOrCommaExpected { get { return ResourceManager.GetString("ExpressionParser_CloseBracketOrCommaExpected", resourceCulture); } } ////// Looks up a localized string similar to ')' or ',' expected. /// internal static string ExpressionParser_CloseParenOrCommaExpected { get { return ResourceManager.GetString("ExpressionParser_CloseParenOrCommaExpected", resourceCulture); } } ////// Looks up a localized string similar to ')' or operator expected. /// internal static string ExpressionParser_CloseParenOrOperatorExpected { get { return ResourceManager.GetString("ExpressionParser_CloseParenOrOperatorExpected", resourceCulture); } } ////// Looks up a localized string similar to ':' expected. /// internal static string ExpressionParser_ColonExpected { get { return ResourceManager.GetString("ExpressionParser_ColonExpected", resourceCulture); } } ////// Looks up a localized string similar to Digit expected. /// internal static string ExpressionParser_DigitExpected { get { return ResourceManager.GetString("ExpressionParser_DigitExpected", resourceCulture); } } ////// Looks up a localized string similar to '.' or '(' expected. /// internal static string ExpressionParser_DotOrOpenParenExpected { get { return ResourceManager.GetString("ExpressionParser_DotOrOpenParenExpected", resourceCulture); } } ////// Looks up a localized string similar to The identifier '{0}' was defined more than once. /// internal static string ExpressionParser_DuplicateIdentifier { get { return ResourceManager.GetString("ExpressionParser_DuplicateIdentifier", resourceCulture); } } ////// Looks up a localized string similar to Expression expected. /// internal static string ExpressionParser_ExpressionExpected { get { return ResourceManager.GetString("ExpressionParser_ExpressionExpected", resourceCulture); } } ////// Looks up a localized string similar to Expression of type '{0}' expected. /// internal static string ExpressionParser_ExpressionTypeMismatch { get { return ResourceManager.GetString("ExpressionParser_ExpressionTypeMismatch", resourceCulture); } } ////// Looks up a localized string similar to The first expression must be of type 'Boolean'. /// internal static string ExpressionParser_FirstExprMustBeBool { get { return ResourceManager.GetString("ExpressionParser_FirstExprMustBeBool", resourceCulture); } } ////// Looks up a localized string similar to Identifier expected. /// internal static string ExpressionParser_IdentifierExpected { get { return ResourceManager.GetString("ExpressionParser_IdentifierExpected", resourceCulture); } } ////// Looks up a localized string similar to The 'iif' function requires three arguments. /// internal static string ExpressionParser_IifRequiresThreeArgs { get { return ResourceManager.GetString("ExpressionParser_IifRequiresThreeArgs", resourceCulture); } } ////// Looks up a localized string similar to Operator '{0}' incompatible with operand type '{1}'. /// internal static string ExpressionParser_IncompatibleOperand { get { return ResourceManager.GetString("ExpressionParser_IncompatibleOperand", resourceCulture); } } ////// Looks up a localized string similar to Operator '{0}' incompatible with operand types '{1}' and '{2}'. /// internal static string ExpressionParser_IncompatibleOperands { get { return ResourceManager.GetString("ExpressionParser_IncompatibleOperands", resourceCulture); } } ////// Looks up a localized string similar to Syntax error '{0}'. /// internal static string ExpressionParser_InvalidCharacter { get { return ResourceManager.GetString("ExpressionParser_InvalidCharacter", resourceCulture); } } ////// Looks up a localized string similar to Character literal must contain exactly one character. /// internal static string ExpressionParser_InvalidCharacterLiteral { get { return ResourceManager.GetString("ExpressionParser_InvalidCharacterLiteral", resourceCulture); } } ////// Looks up a localized string similar to Array index must be an integer expression. /// internal static string ExpressionParser_InvalidIndex { get { return ResourceManager.GetString("ExpressionParser_InvalidIndex", resourceCulture); } } ////// Looks up a localized string similar to Invalid integer literal '{0}'. /// internal static string ExpressionParser_InvalidIntegerLiteral { get { return ResourceManager.GetString("ExpressionParser_InvalidIntegerLiteral", resourceCulture); } } ////// Looks up a localized string similar to Invalid real literal '{0}'. /// internal static string ExpressionParser_InvalidRealLiteral { get { return ResourceManager.GetString("ExpressionParser_InvalidRealLiteral", resourceCulture); } } ////// Looks up a localized string similar to Method '{0}' in type '{1}' does not return a value. /// internal static string ExpressionParser_MethodIsVoid { get { return ResourceManager.GetString("ExpressionParser_MethodIsVoid", resourceCulture); } } ////// Looks up a localized string similar to Methods on type '{0}' are not accessible. /// internal static string ExpressionParser_MethodsAreInaccessible { get { return ResourceManager.GetString("ExpressionParser_MethodsAreInaccessible", resourceCulture); } } ////// Looks up a localized string similar to Expression is missing an 'as' clause. /// internal static string ExpressionParser_MissingAsClause { get { return ResourceManager.GetString("ExpressionParser_MissingAsClause", resourceCulture); } } ////// Looks up a localized string similar to Neither of the types '{0}' and '{1}' converts to the other. /// internal static string ExpressionParser_NeitherTypeConvertsToOther { get { return ResourceManager.GetString("ExpressionParser_NeitherTypeConvertsToOther", resourceCulture); } } ////// Looks up a localized string similar to No applicable aggregate method '{0}' exists. /// internal static string ExpressionParser_NoApplicableAggregate { get { return ResourceManager.GetString("ExpressionParser_NoApplicableAggregate", resourceCulture); } } ////// Looks up a localized string similar to No applicable indexer exists in type '{0}'. /// internal static string ExpressionParser_NoApplicableIndexer { get { return ResourceManager.GetString("ExpressionParser_NoApplicableIndexer", resourceCulture); } } ////// Looks up a localized string similar to No applicable method '{0}' exists in type '{1}'. /// internal static string ExpressionParser_NoApplicableMethod { get { return ResourceManager.GetString("ExpressionParser_NoApplicableMethod", resourceCulture); } } ////// Looks up a localized string similar to No 'it' is in scope. /// internal static string ExpressionParser_NoItInScope { get { return ResourceManager.GetString("ExpressionParser_NoItInScope", resourceCulture); } } ////// Looks up a localized string similar to No matching constructor in type '{0}'. /// internal static string ExpressionParser_NoMatchingConstructor { get { return ResourceManager.GetString("ExpressionParser_NoMatchingConstructor", resourceCulture); } } ////// Looks up a localized string similar to '[' expected. /// internal static string ExpressionParser_OpenBracketExpected { get { return ResourceManager.GetString("ExpressionParser_OpenBracketExpected", resourceCulture); } } ////// Looks up a localized string similar to '(' expected. /// internal static string ExpressionParser_OpenParenExpected { get { return ResourceManager.GetString("ExpressionParser_OpenParenExpected", resourceCulture); } } ////// Looks up a localized string similar to Syntax error. /// internal static string ExpressionParser_SyntaxError { get { return ResourceManager.GetString("ExpressionParser_SyntaxError", resourceCulture); } } ////// Looks up a localized string similar to {0} expected. /// internal static string ExpressionParser_TokenExpected { get { return ResourceManager.GetString("ExpressionParser_TokenExpected", resourceCulture); } } ////// Looks up a localized string similar to Type '{0}' has no nullable form. /// internal static string ExpressionParser_TypeHasNoNullableForm { get { return ResourceManager.GetString("ExpressionParser_TypeHasNoNullableForm", resourceCulture); } } ////// Looks up a localized string similar to Unknown identifier '{0}'. /// internal static string ExpressionParser_UnknownIdentifier { get { return ResourceManager.GetString("ExpressionParser_UnknownIdentifier", resourceCulture); } } ////// Looks up a localized string similar to No property or field '{0}' exists in type '{1}'. /// internal static string ExpressionParser_UnknownPropertyOrField { get { return ResourceManager.GetString("ExpressionParser_UnknownPropertyOrField", resourceCulture); } } ////// Looks up a localized string similar to Unterminated string literal. /// internal static string ExpressionParser_UnterminatedStringLiteral { get { return ResourceManager.GetString("ExpressionParser_UnterminatedStringLiteral", resourceCulture); } } ////// Looks up a localized string similar to An extender can't be in a different UpdatePanel than the control it extends.. /// internal static string ExtenderControl_TargetControlDifferentUpdatePanel { get { return ResourceManager.GetString("ExtenderControl_TargetControlDifferentUpdatePanel", resourceCulture); } } ////// Looks up a localized string similar to Identifies the control to extend.. /// internal static string ExtenderControl_TargetControlID { get { return ResourceManager.GetString("ExtenderControl_TargetControlID", resourceCulture); } } ////// Looks up a localized string similar to The TargetControlID of '{0}' is not valid. The value cannot be null or empty.. /// internal static string ExtenderControl_TargetControlIDEmpty { get { return ResourceManager.GetString("ExtenderControl_TargetControlIDEmpty", resourceCulture); } } ////// Looks up a localized string similar to The TargetControlID of '{0}' is not valid. A control with ID '{1}' could not be found.. /// internal static string ExtenderControl_TargetControlIDInvalid { get { return ResourceManager.GetString("ExtenderControl_TargetControlIDInvalid", resourceCulture); } } ////// Looks up a localized string similar to Specifies an override for the table name used by the FilterRepeater. By default the table is inferred from the page URL.. /// internal static string FilterRepeater_TableName { get { return ResourceManager.GetString("FilterRepeater_TableName", resourceCulture); } } ////// Looks up a localized string similar to Type '{0}' is not supported for deserialization of an array.. /// internal static string JSON_ArrayTypeNotSupported { get { return ResourceManager.GetString("JSON_ArrayTypeNotSupported", resourceCulture); } } ////// Looks up a localized string similar to Unrecognized escape sequence.. /// internal static string JSON_BadEscape { get { return ResourceManager.GetString("JSON_BadEscape", resourceCulture); } } ////// Looks up a localized string similar to Cannot convert object of type '{0}' to type '{1}'. /// internal static string JSON_CannotConvertObjectToType { get { return ResourceManager.GetString("JSON_CannotConvertObjectToType", resourceCulture); } } ////// Looks up a localized string similar to Cannot create instance of {0}.. /// internal static string JSON_CannotCreateListType { get { return ResourceManager.GetString("JSON_CannotCreateListType", resourceCulture); } } ////// Looks up a localized string similar to A circular reference was detected while serializing an object of type '{0}'.. /// internal static string JSON_CircularReference { get { return ResourceManager.GetString("JSON_CircularReference", resourceCulture); } } ////// Looks up a localized string similar to RecursionLimit exceeded.. /// internal static string JSON_DepthLimitExceeded { get { return ResourceManager.GetString("JSON_DepthLimitExceeded", resourceCulture); } } ////// Looks up a localized string similar to Cannot deserialize object graph into type of '{0}'.. /// internal static string JSON_DeserializerTypeMismatch { get { return ResourceManager.GetString("JSON_DeserializerTypeMismatch", resourceCulture); } } ////// Looks up a localized string similar to Type '{0}' is not supported for serialization/deserialization of a dictionary, keys must be strings or objects.. /// internal static string JSON_DictionaryTypeNotSupported { get { return ResourceManager.GetString("JSON_DictionaryTypeNotSupported", resourceCulture); } } ////// Looks up a localized string similar to Invalid object passed in, '{' expected.. /// internal static string JSON_ExpectedOpenBrace { get { return ResourceManager.GetString("JSON_ExpectedOpenBrace", resourceCulture); } } ////// Looks up a localized string similar to Invalid JSON primitive: {0}.. /// internal static string JSON_IllegalPrimitive { get { return ResourceManager.GetString("JSON_IllegalPrimitive", resourceCulture); } } ////// Looks up a localized string similar to Invalid array passed in, ']' expected.. /// internal static string JSON_InvalidArrayEnd { get { return ResourceManager.GetString("JSON_InvalidArrayEnd", resourceCulture); } } ////// Looks up a localized string similar to Invalid array passed in, ',' expected.. /// internal static string JSON_InvalidArrayExpectComma { get { return ResourceManager.GetString("JSON_InvalidArrayExpectComma", resourceCulture); } } ////// Looks up a localized string similar to Invalid array passed in, extra trailing ','.. /// internal static string JSON_InvalidArrayExtraComma { get { return ResourceManager.GetString("JSON_InvalidArrayExtraComma", resourceCulture); } } ////// Looks up a localized string similar to Invalid array passed in, '[' expected.. /// internal static string JSON_InvalidArrayStart { get { return ResourceManager.GetString("JSON_InvalidArrayStart", resourceCulture); } } ////// Looks up a localized string similar to Enums based on System.Int64 or System.UInt64 are not JSON-serializable because JavaScript does not support the necessary precision.. /// internal static string JSON_InvalidEnumType { get { return ResourceManager.GetString("JSON_InvalidEnumType", resourceCulture); } } ////// Looks up a localized string similar to Value must be a positive integer.. /// internal static string JSON_InvalidMaxJsonLength { get { return ResourceManager.GetString("JSON_InvalidMaxJsonLength", resourceCulture); } } ////// Looks up a localized string similar to Invalid object passed in, member name expected.. /// internal static string JSON_InvalidMemberName { get { return ResourceManager.GetString("JSON_InvalidMemberName", resourceCulture); } } ////// Looks up a localized string similar to Invalid object passed in, ':' or '}' expected.. /// internal static string JSON_InvalidObject { get { return ResourceManager.GetString("JSON_InvalidObject", resourceCulture); } } ////// Looks up a localized string similar to RecursionLimit must be a positive integer.. /// internal static string JSON_InvalidRecursionLimit { get { return ResourceManager.GetString("JSON_InvalidRecursionLimit", resourceCulture); } } ////// Looks up a localized string similar to Error during serialization or deserialization using the JSON JavaScriptSerializer. The length of the string exceeds the value set on the maxJsonLength property.. /// internal static string JSON_MaxJsonLengthExceeded { get { return ResourceManager.GetString("JSON_MaxJsonLengthExceeded", resourceCulture); } } ////// Looks up a localized string similar to No parameterless constructor defined for type of '{0}'.. /// internal static string JSON_NoConstructor { get { return ResourceManager.GetString("JSON_NoConstructor", resourceCulture); } } ////// Looks up a localized string similar to Invalid string passed in, '\"' expected.. /// internal static string JSON_StringNotQuoted { get { return ResourceManager.GetString("JSON_StringNotQuoted", resourceCulture); } } ////// Looks up a localized string similar to Unterminated string passed in.. /// internal static string JSON_UnterminatedString { get { return ResourceManager.GetString("JSON_UnterminatedString", resourceCulture); } } ////// Looks up a localized string similar to Cannot convert null to a value type.. /// internal static string JSON_ValueTypeCannotBeNull { get { return ResourceManager.GetString("JSON_ValueTypeCannotBeNull", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether to automatically generate the OrderBy clause from the OrderByParameters.. /// internal static string LinqDataSource_AutoGenerateOrderByClause { get { return ResourceManager.GetString("LinqDataSource_AutoGenerateOrderByClause", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether to automatically generate the Where clause from the WhereParameters.. /// internal static string LinqDataSource_AutoGenerateWhereClause { get { return ResourceManager.GetString("LinqDataSource_AutoGenerateWhereClause", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether data is automatically paged.. /// internal static string LinqDataSource_AutoPage { get { return ResourceManager.GetString("LinqDataSource_AutoPage", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether data is automatically sorted.. /// internal static string LinqDataSource_AutoSort { get { return ResourceManager.GetString("LinqDataSource_AutoSort", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the context is created unless a query result is specified during the Selecting event.. /// internal static string LinqDataSource_ContextCreated { get { return ResourceManager.GetString("LinqDataSource_ContextCreated", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the context is created unless a query result is specified during the Selecting event.. /// internal static string LinqDataSource_ContextCreating { get { return ResourceManager.GetString("LinqDataSource_ContextCreating", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the context is disposed.. /// internal static string LinqDataSource_ContextDisposing { get { return ResourceManager.GetString("LinqDataSource_ContextDisposing", resourceCulture); } } ////// Looks up a localized string similar to The data context type that contains the table property.. /// internal static string LinqDataSource_ContextTypeName { get { return ResourceManager.GetString("LinqDataSource_ContextTypeName", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the Delete operation is completed.. /// internal static string LinqDataSource_Deleted { get { return ResourceManager.GetString("LinqDataSource_Deleted", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used during the Delete operation. These parameters are merged with the parameters provided by data-bound controls.. /// internal static string LinqDataSource_DeleteParameters { get { return ResourceManager.GetString("LinqDataSource_DeleteParameters", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the Delete operation is executed.. /// internal static string LinqDataSource_Deleting { get { return ResourceManager.GetString("LinqDataSource_Deleting", resourceCulture); } } ////// Looks up a localized string similar to Use LINQ to connect to a DataContext or object in the Bin or App_Code directory for the application.. /// internal static string LinqDataSource_Description { get { return ResourceManager.GetString("LinqDataSource_Description", resourceCulture); } } ////// Looks up a localized string similar to LINQ. /// internal static string LinqDataSource_DisplayName { get { return ResourceManager.GetString("LinqDataSource_DisplayName", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the Delete operation is enabled.. /// internal static string LinqDataSource_EnableDelete { get { return ResourceManager.GetString("LinqDataSource_EnableDelete", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the Insert operation is enabled.. /// internal static string LinqDataSource_EnableInsert { get { return ResourceManager.GetString("LinqDataSource_EnableInsert", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether ObjectTracking should be disabled on read-only Linq to SQL data contexts.. /// internal static string LinqDataSource_EnableObjectTracking { get { return ResourceManager.GetString("LinqDataSource_EnableObjectTracking", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the Update operation is enabled.. /// internal static string LinqDataSource_EnableUpdate { get { return ResourceManager.GetString("LinqDataSource_EnableUpdate", resourceCulture); } } ////// Looks up a localized string similar to The expression passed to the GroupBy operator during the Select query.. /// internal static string LinqDataSource_GroupBy { get { return ResourceManager.GetString("LinqDataSource_GroupBy", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used for the GroupBy operator during the Select query.. /// internal static string LinqDataSource_GroupByParameters { get { return ResourceManager.GetString("LinqDataSource_GroupByParameters", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the Insert operation is completed.. /// internal static string LinqDataSource_Inserted { get { return ResourceManager.GetString("LinqDataSource_Inserted", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the Insert operation is executed.. /// internal static string LinqDataSource_Inserting { get { return ResourceManager.GetString("LinqDataSource_Inserting", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used during the Insert operation. These parameters are merged with the parameters provided by data-bound controls.. /// internal static string LinqDataSource_InsertParameters { get { return ResourceManager.GetString("LinqDataSource_InsertParameters", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' only supports a single view named '{1}'. You may also leave the view name empty for the default view to be chosen.. /// internal static string LinqDataSource_InvalidViewName { get { return ResourceManager.GetString("LinqDataSource_InvalidViewName", resourceCulture); } } ////// Looks up a localized string similar to The expression passed to the OrderBy operator during the Select query.. /// internal static string LinqDataSource_OrderBy { get { return ResourceManager.GetString("LinqDataSource_OrderBy", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used for the OrderBy operator during the Select query.. /// internal static string LinqDataSource_OrderByParameters { get { return ResourceManager.GetString("LinqDataSource_OrderByParameters", resourceCulture); } } ////// Looks up a localized string similar to The expression passed to the OrderBy operator used for ordering groups after a GroupBy has been performed during the Select query.. /// internal static string LinqDataSource_OrderGroupsBy { get { return ResourceManager.GetString("LinqDataSource_OrderGroupsBy", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used for the OrderGroupsBy operator during the Select query.. /// internal static string LinqDataSource_OrderGroupsByParameters { get { return ResourceManager.GetString("LinqDataSource_OrderGroupsByParameters", resourceCulture); } } ////// Looks up a localized string similar to The expression defining a projection used during the Select query.. /// internal static string LinqDataSource_Select { get { return ResourceManager.GetString("LinqDataSource_Select", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the Select operation is completed.. /// internal static string LinqDataSource_Selected { get { return ResourceManager.GetString("LinqDataSource_Selected", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the Select operation is executed.. /// internal static string LinqDataSource_Selecting { get { return ResourceManager.GetString("LinqDataSource_Selecting", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used in the projection during the Select query.. /// internal static string LinqDataSource_SelectParameters { get { return ResourceManager.GetString("LinqDataSource_SelectParameters", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether to store original data values in ViewState. This property is used for conflict detection during Update and Delete operations.. /// internal static string LinqDataSource_StoreOriginalValuesInViewState { get { return ResourceManager.GetString("LinqDataSource_StoreOriginalValuesInViewState", resourceCulture); } } ////// Looks up a localized string similar to The name of the table property on the data context.. /// internal static string LinqDataSource_TableName { get { return ResourceManager.GetString("LinqDataSource_TableName", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the Update operation is completed.. /// internal static string LinqDataSource_Updated { get { return ResourceManager.GetString("LinqDataSource_Updated", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used during the Update operation. These parameters are merged with the parameters provided by data-bound controls.. /// internal static string LinqDataSource_UpdateParameters { get { return ResourceManager.GetString("LinqDataSource_UpdateParameters", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the Update operation is executed.. /// internal static string LinqDataSource_Updating { get { return ResourceManager.GetString("LinqDataSource_Updating", resourceCulture); } } ////// Looks up a localized string similar to The expression passed to the Where operator during the Select query.. /// internal static string LinqDataSource_Where { get { return ResourceManager.GetString("LinqDataSource_Where", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used for the Where operator during the Select query.. /// internal static string LinqDataSource_WhereParameters { get { return ResourceManager.GetString("LinqDataSource_WhereParameters", resourceCulture); } } ////// Looks up a localized string similar to Failed to set one or more properties on the data object. Ensure that the input values are valid and can be converted to the corresponding property types.. /// internal static string LinqDataSourceValidationException_ValidationFailed { get { return ResourceManager.GetString("LinqDataSourceValidationException_ValidationFailed", resourceCulture); } } ////// Looks up a localized string similar to Cannot convert value of parameter '{0}' from '{1}' to '{2}'.. /// internal static string LinqDataSourceView_CannotConvertType { get { return ResourceManager.GetString("LinqDataSourceView_CannotConvertType", resourceCulture); } } ////// Looks up a localized string similar to The ContextTypeName property of LinqDataSource '{0}' cannot be changed after the data context has been created.. /// internal static string LinqDataSourceView_ContextTypeNameChanged { get { return ResourceManager.GetString("LinqDataSourceView_ContextTypeNameChanged", resourceCulture); } } ////// Looks up a localized string similar to Could not find the type specified in the ContextTypeName property of LinqDataSource '{0}'.. /// internal static string LinqDataSourceView_ContextTypeNameNotFound { get { return ResourceManager.GetString("LinqDataSourceView_ContextTypeNameNotFound", resourceCulture); } } ////// Looks up a localized string similar to The ContextTypeName property of LinqDataSource '{0}' must specify a data context type.. /// internal static string LinqDataSourceView_ContextTypeNameNotSpecified { get { return ResourceManager.GetString("LinqDataSourceView_ContextTypeNameNotSpecified", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Delete operation unless EnableDelete is true.. /// internal static string LinqDataSourceView_DeleteNotSupported { get { return ResourceManager.GetString("LinqDataSourceView_DeleteNotSupported", resourceCulture); } } ////// Looks up a localized string similar to The EnableObjectTracking property of LinqDataSource '{0}' cannot be changed after the data context has been created.. /// internal static string LinqDataSourceView_EnableObjectTrackingChanged { get { return ResourceManager.GetString("LinqDataSourceView_EnableObjectTrackingChanged", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the GroupBy property when the Delete, Insert or Update operations are enabled.. /// internal static string LinqDataSourceView_GroupByNotSupportedOnEdit { get { return ResourceManager.GetString("LinqDataSourceView_GroupByNotSupportedOnEdit", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Insert operation unless EnableInsert is true.. /// internal static string LinqDataSourceView_InsertNotSupported { get { return ResourceManager.GetString("LinqDataSourceView_InsertNotSupported", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' has no values to insert. Check that the 'values' dictionary contains values.. /// internal static string LinqDataSourceView_InsertRequiresValues { get { return ResourceManager.GetString("LinqDataSourceView_InsertRequiresValues", resourceCulture); } } ////// Looks up a localized string similar to The data context used by LinqDataSource '{0}' must extend DataContext when the Delete, Insert or Update operations are enabled.. /// internal static string LinqDataSourceView_InvalidContextType { get { return ResourceManager.GetString("LinqDataSourceView_InvalidContextType", resourceCulture); } } ////// Looks up a localized string similar to The value '{0}' for parameter '{1}' is not a valid OrderBy field name.. /// internal static string LinqDataSourceView_InvalidOrderByFieldName { get { return ResourceManager.GetString("LinqDataSourceView_InvalidOrderByFieldName", resourceCulture); } } ////// Looks up a localized string similar to The name for parameter '{0}' on LinqDataSource '{1}' is not a valid identifier name.. /// internal static string LinqDataSourceView_InvalidParameterName { get { return ResourceManager.GetString("LinqDataSourceView_InvalidParameterName", resourceCulture); } } ////// Looks up a localized string similar to The table property used by LinqDataSource '{0}' must extend Table<T> when the Delete, Insert or Update operations are enabled.. /// internal static string LinqDataSourceView_InvalidTablePropertyType { get { return ResourceManager.GetString("LinqDataSourceView_InvalidTablePropertyType", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the OrderBy property when AutoGenerateOrderByClause is true.. /// internal static string LinqDataSourceView_OrderByAlreadySpecified { get { return ResourceManager.GetString("LinqDataSourceView_OrderByAlreadySpecified", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the OrderGroupsBy property when the GroupsBy property has not been set.. /// internal static string LinqDataSourceView_OrderGroupsByRequiresGroupBy { get { return ResourceManager.GetString("LinqDataSourceView_OrderGroupsByRequiresGroupBy", resourceCulture); } } ////// Looks up a localized string similar to Could not find a row that matches the given keys in the original values stored in ViewState. Ensure that the 'keys' dictionary contains unique key values that correspond to a row returned from the previous Select operation.. /// internal static string LinqDataSourceView_OriginalValuesNotFound { get { return ResourceManager.GetString("LinqDataSourceView_OriginalValuesNotFound", resourceCulture); } } ////// Looks up a localized string similar to AutoPage is disabled on LinqDataSource {0} but paging has not been handled. Ensure you have set the LinqDataSourceSelectArguments.Arguments.TotalRowCount property to the total number of rows.. /// internal static string LinqDataSourceView_PagingNotHandled { get { return ResourceManager.GetString("LinqDataSourceView_PagingNotHandled", resourceCulture); } } ////// Looks up a localized string similar to Parameters for LinqDataSource '{0}' that are not used for AutoGenerateOrderBy must be named.. /// internal static string LinqDataSourceView_ParametersMustBeNamed { get { return ResourceManager.GetString("LinqDataSourceView_ParametersMustBeNamed", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Select property when the Delete, Insert or Update operations are enabled.. /// internal static string LinqDataSourceView_SelectNewNotSupportedOnEdit { get { return ResourceManager.GetString("LinqDataSourceView_SelectNewNotSupportedOnEdit", resourceCulture); } } ////// Looks up a localized string similar to Member '{0}' on the data context type '{1}' of LinqDataSource '{2}' is not a valid table. For Insert, Update and Delete the table must not be a static member.. /// internal static string LinqDataSourceView_TableCannotBeStatic { get { return ResourceManager.GetString("LinqDataSourceView_TableCannotBeStatic", resourceCulture); } } ////// Looks up a localized string similar to The TableName property of LinqDataSource '{0}' cannot be changed after the data context has been created.. /// internal static string LinqDataSourceView_TableNameChanged { get { return ResourceManager.GetString("LinqDataSourceView_TableNameChanged", resourceCulture); } } ////// Looks up a localized string similar to Could not find a property or field called '{0}' on the data context type '{1}' of LinqDataSource '{2}'.. /// internal static string LinqDataSourceView_TableNameNotFound { get { return ResourceManager.GetString("LinqDataSourceView_TableNameNotFound", resourceCulture); } } ////// Looks up a localized string similar to The TableName property of LinqDataSource '{0}' must specify a table property or field on the data context type.. /// internal static string LinqDataSourceView_TableNameNotSpecified { get { return ResourceManager.GetString("LinqDataSourceView_TableNameNotSpecified", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Update operation unless EnableUpdate is true.. /// internal static string LinqDataSourceView_UpdateNotSupported { get { return ResourceManager.GetString("LinqDataSourceView_UpdateNotSupported", resourceCulture); } } ////// Looks up a localized string similar to Failed to set one or more properties on type {0}. {1}. /// internal static string LinqDataSourceView_ValidationFailed { get { return ResourceManager.GetString("LinqDataSourceView_ValidationFailed", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Where property when AutoGenerateWhereClause is true.. /// internal static string LinqDataSourceView_WhereAlreadySpecified { get { return ResourceManager.GetString("LinqDataSourceView_WhereAlreadySpecified", resourceCulture); } } ////// Looks up a localized string similar to The template used for alternating items.. /// internal static string ListView_AlternatingItemTemplate { get { return ResourceManager.GetString("ListView_AlternatingItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to The value of {0} must not be empty.. /// internal static string ListView_ContainerNameMustNotBeEmpty { get { return ResourceManager.GetString("ListView_ContainerNameMustNotBeEmpty", resourceCulture); } } ////// Looks up a localized string similar to Whether the ListView treats empty strings as null when the value is extracted from the item.. /// internal static string ListView_ConvertEmptyStringToNull { get { return ResourceManager.GetString("ListView_ConvertEmptyStringToNull", resourceCulture); } } ////// Looks up a localized string similar to A comma-separated list of key fields in the data source.. /// internal static string ListView_DataKeyNames { get { return ResourceManager.GetString("ListView_DataKeyNames", resourceCulture); } } ////// Looks up a localized string similar to Data keys must be specified on ListView '{0}' before the selected data keys can be retrieved. Use the DataKeyNames property to specify data keys.. /// internal static string ListView_DataKeyNamesMustBeSpecified { get { return ResourceManager.GetString("ListView_DataKeyNamesMustBeSpecified", resourceCulture); } } ////// Looks up a localized string similar to The collection of data key field values.. /// internal static string ListView_DataKeys { get { return ResourceManager.GetString("ListView_DataKeys", resourceCulture); } } ////// Looks up a localized string similar to The data source '{0}' does not support server-side paging and it returned non-ICollection. /// internal static string ListView_DataSourceDoesntSupportPaging { get { return ResourceManager.GetString("ListView_DataSourceDoesntSupportPaging", resourceCulture); } } ////// Looks up a localized string similar to Data source must implement ICollection when calling CreateChildControls with dataBinding=false.. /// internal static string ListView_DataSourceMustBeCollectionWhenNotDataBinding { get { return ResourceManager.GetString("ListView_DataSourceMustBeCollectionWhenNotDataBinding", resourceCulture); } } ////// Looks up a localized string similar to The index of the item shown in edit mode.. /// internal static string ListView_EditIndex { get { return ResourceManager.GetString("ListView_EditIndex", resourceCulture); } } ////// Looks up a localized string similar to The ListViewItem that is currently being edited.. /// internal static string ListView_EditItem { get { return ResourceManager.GetString("ListView_EditItem", resourceCulture); } } ////// Looks up a localized string similar to The template used for items in edit mode.. /// internal static string ListView_EditItemTemplate { get { return ResourceManager.GetString("ListView_EditItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to The template used when no data is returned from the data source. This template replaces the LayoutTemplate when used.. /// internal static string ListView_EmptyDataTemplate { get { return ResourceManager.GetString("ListView_EmptyDataTemplate", resourceCulture); } } ////// Looks up a localized string similar to The template used in the GroupTemplate when the number of remaining data items is less than the GroupItemCount.. /// internal static string ListView_EmptyItemTemplate { get { return ResourceManager.GetString("ListView_EmptyItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to Whether the data bound control will register itself with a data bound control manager on the page.. /// internal static string ListView_EnableDataBoundControlManager { get { return ResourceManager.GetString("ListView_EnableDataBoundControlManager", resourceCulture); } } ////// Looks up a localized string similar to Whether page validation will be performed after validation is done in the model.. /// internal static string ListView_EnableModelValidation { get { return ResourceManager.GetString("ListView_EnableModelValidation", resourceCulture); } } ////// Looks up a localized string similar to The ID of the server control that will be replaced with instances of the GroupTemplate.. /// internal static string ListView_GroupContainerID { get { return ResourceManager.GetString("ListView_GroupContainerID", resourceCulture); } } ////// Looks up a localized string similar to The number of items that are rendered inside the GroupTemplate.. /// internal static string ListView_GroupItemCount { get { return ResourceManager.GetString("ListView_GroupItemCount", resourceCulture); } } ////// Looks up a localized string similar to ListView '{0}' has a GroupItemCount specified on it but no GroupTemplate. A GroupTemplate must be present for ListView to render groups.. /// internal static string ListView_GroupItemCountNoGroupTemplate { get { return ResourceManager.GetString("ListView_GroupItemCountNoGroupTemplate", resourceCulture); } } ////// Looks up a localized string similar to The template used for group separators between GroupTemplates.. /// internal static string ListView_GroupSeparatorTemplate { get { return ResourceManager.GetString("ListView_GroupSeparatorTemplate", resourceCulture); } } ////// Looks up a localized string similar to The template used for item groups.. /// internal static string ListView_GroupTemplate { get { return ResourceManager.GetString("ListView_GroupTemplate", resourceCulture); } } ////// Looks up a localized string similar to The ListViewItem that is currently being inserted.. /// internal static string ListView_InsertItem { get { return ResourceManager.GetString("ListView_InsertItem", resourceCulture); } } ////// Looks up a localized string similar to The position of the insert item within the ListView.. /// internal static string ListView_InsertItemPosition { get { return ResourceManager.GetString("ListView_InsertItemPosition", resourceCulture); } } ////// Looks up a localized string similar to The template used for items in insert mode.. /// internal static string ListView_InsertItemTemplate { get { return ResourceManager.GetString("ListView_InsertItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to An InsertItemTemplate must be defined on ListView '{0}' if InsertItemPosition is set to FirstItem or LastItem.. /// internal static string ListView_InsertTemplateRequired { get { return ResourceManager.GetString("ListView_InsertTemplateRequired", resourceCulture); } } ////// Looks up a localized string similar to Cancel can only be called from the currently-edited record or an insert item.. /// internal static string ListView_InvalidCancel { get { return ResourceManager.GetString("ListView_InvalidCancel", resourceCulture); } } ////// Looks up a localized string similar to Delete can only be called on a valid data item.. /// internal static string ListView_InvalidDelete { get { return ResourceManager.GetString("ListView_InvalidDelete", resourceCulture); } } ////// Looks up a localized string similar to Edit can only be called on a valid data item.. /// internal static string ListView_InvalidEdit { get { return ResourceManager.GetString("ListView_InvalidEdit", resourceCulture); } } ////// Looks up a localized string similar to Insert can only be called on an insert item. Ensure only the InsertTemplate has a button with CommandName=Insert.. /// internal static string ListView_InvalidInsert { get { return ResourceManager.GetString("ListView_InvalidInsert", resourceCulture); } } ////// Looks up a localized string similar to Select can only be called on a valid data item.. /// internal static string ListView_InvalidSelect { get { return ResourceManager.GetString("ListView_InvalidSelect", resourceCulture); } } ////// Looks up a localized string similar to Update can only be called on a valid data item.. /// internal static string ListView_InvalidUpdate { get { return ResourceManager.GetString("ListView_InvalidUpdate", resourceCulture); } } ////// Looks up a localized string similar to The ID of the server control that will be replaced with instances of the ItemTemplate.. /// internal static string ListView_ItemPlaceholderID { get { return ResourceManager.GetString("ListView_ItemPlaceholderID", resourceCulture); } } ////// Looks up a localized string similar to The collection of visible items.. /// internal static string ListView_Items { get { return ResourceManager.GetString("ListView_Items", resourceCulture); } } ////// Looks up a localized string similar to The template used for separator items.. /// internal static string ListView_ItemSeparatorTemplate { get { return ResourceManager.GetString("ListView_ItemSeparatorTemplate", resourceCulture); } } ////// Looks up a localized string similar to ListViewItems that have type DataItem must be of type ListViewDataItem.. /// internal static string ListView_ItemsNotDataItems { get { return ResourceManager.GetString("ListView_ItemsNotDataItems", resourceCulture); } } ////// Looks up a localized string similar to The template used for items.. /// internal static string ListView_ItemTemplate { get { return ResourceManager.GetString("ListView_ItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to An ItemTemplate must be defined on ListView '{0}'.. /// internal static string ListView_ItemTemplateRequired { get { return ResourceManager.GetString("ListView_ItemTemplateRequired", resourceCulture); } } ////// Looks up a localized string similar to The template used for the ListView layout.. /// internal static string ListView_LayoutTemplate { get { return ResourceManager.GetString("ListView_LayoutTemplate", resourceCulture); } } ////// Looks up a localized string similar to ListView with id '{0}' must have a data source that either implements ICollection or can perform data source paging if AllowPaging is true.. /// internal static string ListView_Missing_VirtualItemCount { get { return ResourceManager.GetString("ListView_Missing_VirtualItemCount", resourceCulture); } } ////// Looks up a localized string similar to If a data source does not return ICollection and cannot return the total row count, it cannot be used by the {0} to implement server-side paging.. /// internal static string ListView_NeedICollectionOrTotalRowCount { get { return ResourceManager.GetString("ListView_NeedICollectionOrTotalRowCount", resourceCulture); } } ////// Looks up a localized string similar to A group placeholder must be specified on ListView '{0}' when the GroupTemplate is defined. Specify a group placeholder by setting its ID property to "{1}". The group placeholder control must also specify runat="server".. /// internal static string ListView_NoGroupPlaceholder { get { return ResourceManager.GetString("ListView_NoGroupPlaceholder", resourceCulture); } } ////// Looks up a localized string similar to An insert item wasn't found.. /// internal static string ListView_NoInsertItem { get { return ResourceManager.GetString("ListView_NoInsertItem", resourceCulture); } } ////// Looks up a localized string similar to An item placeholder must be specified on ListView '{0}'. Specify an item placeholder by setting a control's ID property to "{1}". The item placeholder control must also specify runat="server".. /// internal static string ListView_NoItemPlaceholder { get { return ResourceManager.GetString("ListView_NoItemPlaceholder", resourceCulture); } } ////// Looks up a localized string similar to The data source retrieved by '{0}' returned a null DataSourceView.. /// internal static string ListView_NullView { get { return ResourceManager.GetString("ListView_NullView", resourceCulture); } } ////// Looks up a localized string similar to Fires when a Cancel event is generated within the ListView.. /// internal static string ListView_OnItemCanceling { get { return ResourceManager.GetString("ListView_OnItemCanceling", resourceCulture); } } ////// Looks up a localized string similar to Fires when an event is generated within the ListView.. /// internal static string ListView_OnItemCommand { get { return ResourceManager.GetString("ListView_OnItemCommand", resourceCulture); } } ////// Looks up a localized string similar to Fires when an item is created.. /// internal static string ListView_OnItemCreated { get { return ResourceManager.GetString("ListView_OnItemCreated", resourceCulture); } } ////// Looks up a localized string similar to Fires after an item has been data-bound.. /// internal static string ListView_OnItemDataBound { get { return ResourceManager.GetString("ListView_OnItemDataBound", resourceCulture); } } ////// Looks up a localized string similar to Fires after a Delete Command is executed on the data source.. /// internal static string ListView_OnItemDeleted { get { return ResourceManager.GetString("ListView_OnItemDeleted", resourceCulture); } } ////// Looks up a localized string similar to Fires before a Delete Command is executed on the data source.. /// internal static string ListView_OnItemDeleting { get { return ResourceManager.GetString("ListView_OnItemDeleting", resourceCulture); } } ////// Looks up a localized string similar to Fires when an Edit event is generated within the ListView.. /// internal static string ListView_OnItemEditing { get { return ResourceManager.GetString("ListView_OnItemEditing", resourceCulture); } } ////// Looks up a localized string similar to Fires after an Insert Command is executed on the data source.. /// internal static string ListView_OnItemInserted { get { return ResourceManager.GetString("ListView_OnItemInserted", resourceCulture); } } ////// Looks up a localized string similar to Fires before an Insert Command is executed on the data source.. /// internal static string ListView_OnItemInserting { get { return ResourceManager.GetString("ListView_OnItemInserting", resourceCulture); } } ////// Looks up a localized string similar to Fires after an Update Command is executed on the data source.. /// internal static string ListView_OnItemUpdated { get { return ResourceManager.GetString("ListView_OnItemUpdated", resourceCulture); } } ////// Looks up a localized string similar to Fires before an Update Command is executed on the data source.. /// internal static string ListView_OnItemUpdating { get { return ResourceManager.GetString("ListView_OnItemUpdating", resourceCulture); } } ////// Looks up a localized string similar to Fires when the ListView's layout is created.. /// internal static string ListView_OnLayoutCreated { get { return ResourceManager.GetString("ListView_OnLayoutCreated", resourceCulture); } } ////// Looks up a localized string similar to Fires when the ListView's paging properties have changed.. /// internal static string ListView_OnPagePropertiesChanged { get { return ResourceManager.GetString("ListView_OnPagePropertiesChanged", resourceCulture); } } ////// Looks up a localized string similar to Fires when the ListView's paging properties are changing.. /// internal static string ListView_OnPagePropertiesChanging { get { return ResourceManager.GetString("ListView_OnPagePropertiesChanging", resourceCulture); } } ////// Looks up a localized string similar to Fires when an item is selected in the ListView, after the selection is complete.. /// internal static string ListView_OnSelectedIndexChanged { get { return ResourceManager.GetString("ListView_OnSelectedIndexChanged", resourceCulture); } } ////// Looks up a localized string similar to Fires when an item is selected in the ListView, before the item is selected.. /// internal static string ListView_OnSelectedIndexChanging { get { return ResourceManager.GetString("ListView_OnSelectedIndexChanging", resourceCulture); } } ////// Looks up a localized string similar to Fires when a field is sorted in the ListView, after the sort is complete.. /// internal static string ListView_OnSorted { get { return ResourceManager.GetString("ListView_OnSorted", resourceCulture); } } ////// Looks up a localized string similar to Fires when a field is sorted in the ListView, before the sort occurs.. /// internal static string ListView_OnSorting { get { return ResourceManager.GetString("ListView_OnSorting", resourceCulture); } } ////// Looks up a localized string similar to The index of the currently selected item.. /// internal static string ListView_SelectedIndex { get { return ResourceManager.GetString("ListView_SelectedIndex", resourceCulture); } } ////// Looks up a localized string similar to The template used for the currently selected item.. /// internal static string ListView_SelectedItemTemplate { get { return ResourceManager.GetString("ListView_SelectedItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to The direction in which to sort the field.. /// internal static string ListView_SortDirection { get { return ResourceManager.GetString("ListView_SortDirection", resourceCulture); } } ////// Looks up a localized string similar to Sort expression used to sort the data source to which the ListView is binding.. /// internal static string ListView_SortExpression { get { return ResourceManager.GetString("ListView_SortExpression", resourceCulture); } } ////// Looks up a localized string similar to Style properties are not supported on ListView.. /// internal static string ListView_StyleNotSupported { get { return ResourceManager.GetString("ListView_StyleNotSupported", resourceCulture); } } ////// Looks up a localized string similar to Style properties are not supported on ListView. Apply styling or CSS classes to the elements inside ListView's templates.. /// internal static string ListView_StylePropertiesNotSupported { get { return ResourceManager.GetString("ListView_StylePropertiesNotSupported", resourceCulture); } } ////// Looks up a localized string similar to The ListView '{0}' raised event {1} which wasn't handled.. /// internal static string ListView_UnhandledEvent { get { return ResourceManager.GetString("ListView_UnhandledEvent", resourceCulture); } } ////// Looks up a localized string similar to Cannot compute Count for a data source that does not implement ICollection.. /// internal static string ListViewPagedDataSource_CannotGetCount { get { return ResourceManager.GetString("ListViewPagedDataSource_CannotGetCount", resourceCulture); } } ////// Looks up a localized string similar to You must call MoveNext on IEnumerator before accessing the Current property.. /// internal static string ListViewPagedDataSource_EnumeratorMoveNextNotCalled { get { return ResourceManager.GetString("ListViewPagedDataSource_EnumeratorMoveNextNotCalled", resourceCulture); } } ////// Looks up a localized string similar to The CSS class applied to the next and previous buttons.. /// internal static string NextPreviousPagerField_ButtonCssClass { get { return ResourceManager.GetString("NextPreviousPagerField_ButtonCssClass", resourceCulture); } } ////// Looks up a localized string similar to The type of button contained within the pager field.. /// internal static string NextPreviousPagerField_ButtonType { get { return ResourceManager.GetString("NextPreviousPagerField_ButtonType", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the first page button if the ButtonType is Image.. /// internal static string NextPreviousPagerField_FirstPageImageUrl { get { return ResourceManager.GetString("NextPreviousPagerField_FirstPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the first page button.. /// internal static string NextPreviousPagerField_FirstPageText { get { return ResourceManager.GetString("NextPreviousPagerField_FirstPageText", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the last page button if the ButtonType is Image.. /// internal static string NextPreviousPagerField_LastPageImageUrl { get { return ResourceManager.GetString("NextPreviousPagerField_LastPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the last page button.. /// internal static string NextPreviousPagerField_LastPageText { get { return ResourceManager.GetString("NextPreviousPagerField_LastPageText", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the next page button if the ButtonType is Image.. /// internal static string NextPreviousPagerField_NextPageImageUrl { get { return ResourceManager.GetString("NextPreviousPagerField_NextPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the next page button.. /// internal static string NextPreviousPagerField_NextPageText { get { return ResourceManager.GetString("NextPreviousPagerField_NextPageText", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the previous page button if the ButtonType is Image.. /// internal static string NextPreviousPagerField_PreviousPageImageUrl { get { return ResourceManager.GetString("NextPreviousPagerField_PreviousPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the previous page button.. /// internal static string NextPreviousPagerField_PreviousPageText { get { return ResourceManager.GetString("NextPreviousPagerField_PreviousPageText", resourceCulture); } } ////// Looks up a localized string similar to Whether disabled pager links should be rendered as labels rather than buttons.. /// internal static string NextPreviousPagerField_RenderDisabledButtonsAsLabels { get { return ResourceManager.GetString("NextPreviousPagerField_RenderDisabledButtonsAsLabels", resourceCulture); } } ////// Looks up a localized string similar to Whether non-breaking spaces should be rendered between pager controls.. /// internal static string NextPreviousPagerField_RenderNonBreakingSpacesBetweenControls { get { return ResourceManager.GetString("NextPreviousPagerField_RenderNonBreakingSpacesBetweenControls", resourceCulture); } } ////// Looks up a localized string similar to Whether the pager field should display the first page button.. /// internal static string NextPreviousPagerField_ShowFirstPageButton { get { return ResourceManager.GetString("NextPreviousPagerField_ShowFirstPageButton", resourceCulture); } } ////// Looks up a localized string similar to Whether the pager field should display the last page button.. /// internal static string NextPreviousPagerField_ShowLastPageButton { get { return ResourceManager.GetString("NextPreviousPagerField_ShowLastPageButton", resourceCulture); } } ////// Looks up a localized string similar to Whether the pager field should display the next page button.. /// internal static string NextPreviousPagerField_ShowNextPageButton { get { return ResourceManager.GetString("NextPreviousPagerField_ShowNextPageButton", resourceCulture); } } ////// Looks up a localized string similar to Whether the pager field should display the previous page button.. /// internal static string NextPreviousPagerField_ShowPreviousPageButton { get { return ResourceManager.GetString("NextPreviousPagerField_ShowPreviousPageButton", resourceCulture); } } ////// Looks up a localized string similar to First. /// internal static string NextPrevPagerField_DefaultFirstPageText { get { return ResourceManager.GetString("NextPrevPagerField_DefaultFirstPageText", resourceCulture); } } ////// Looks up a localized string similar to Last. /// internal static string NextPrevPagerField_DefaultLastPageText { get { return ResourceManager.GetString("NextPrevPagerField_DefaultLastPageText", resourceCulture); } } ////// Looks up a localized string similar to Next. /// internal static string NextPrevPagerField_DefaultNextPageText { get { return ResourceManager.GetString("NextPrevPagerField_DefaultNextPageText", resourceCulture); } } ////// Looks up a localized string similar to Previous. /// internal static string NextPrevPagerField_DefaultPreviousPageText { get { return ResourceManager.GetString("NextPrevPagerField_DefaultPreviousPageText", resourceCulture); } } ////// Looks up a localized string similar to The maximum number of page number buttons that can be displayed by the pager field.. /// internal static string NumericPagerField_ButtonCount { get { return ResourceManager.GetString("NumericPagerField_ButtonCount", resourceCulture); } } ////// Looks up a localized string similar to The type of button contained within the pager field.. /// internal static string NumericPagerField_ButtonType { get { return ResourceManager.GetString("NumericPagerField_ButtonType", resourceCulture); } } ////// Looks up a localized string similar to The CSS class applied to the label containing the current page number.. /// internal static string NumericPagerField_CurrentPageLabelCssClass { get { return ResourceManager.GetString("NumericPagerField_CurrentPageLabelCssClass", resourceCulture); } } ////// Looks up a localized string similar to .... /// internal static string NumericPagerField_DefaultNextPageText { get { return ResourceManager.GetString("NumericPagerField_DefaultNextPageText", resourceCulture); } } ////// Looks up a localized string similar to .... /// internal static string NumericPagerField_DefaultPreviousPageText { get { return ResourceManager.GetString("NumericPagerField_DefaultPreviousPageText", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the next page button if the ButtonType is Image.. /// internal static string NumericPagerField_NextPageImageUrl { get { return ResourceManager.GetString("NumericPagerField_NextPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the next page button.. /// internal static string NumericPagerField_NextPageText { get { return ResourceManager.GetString("NumericPagerField_NextPageText", resourceCulture); } } ////// Looks up a localized string similar to The CSS class applied to the next and previous buttons.. /// internal static string NumericPagerField_NextPreviousButtonCssClass { get { return ResourceManager.GetString("NumericPagerField_NextPreviousButtonCssClass", resourceCulture); } } ////// Looks up a localized string similar to The CSS class applied to the numeric pager buttons.. /// internal static string NumericPagerField_NumericButtonCssClass { get { return ResourceManager.GetString("NumericPagerField_NumericButtonCssClass", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the previous page button if the ButtonType is Image.. /// internal static string NumericPagerField_PreviousPageImageUrl { get { return ResourceManager.GetString("NumericPagerField_PreviousPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the previous page button.. /// internal static string NumericPagerField_PreviousPageText { get { return ResourceManager.GetString("NumericPagerField_PreviousPageText", resourceCulture); } } ////// Looks up a localized string similar to Whether non-breaking spaces should be rendered between pager controls.. /// internal static string NumericPagerField_RenderNonBreakingSpacesBetweenControls { get { return ResourceManager.GetString("NumericPagerField_RenderNonBreakingSpacesBetweenControls", resourceCulture); } } ////// Looks up a localized string similar to RegisterDataItem can only be called during an async postback.. /// internal static string PageRequestManager_RegisterDataItemInNonAsyncRequest { get { return ResourceManager.GetString("PageRequestManager_RegisterDataItemInNonAsyncRequest", resourceCulture); } } ////// Looks up a localized string similar to The control '{0}' already has a data item registered.. /// internal static string PageRequestManager_RegisterDataItemTwice { get { return ResourceManager.GetString("PageRequestManager_RegisterDataItemTwice", resourceCulture); } } ////// Looks up a localized string similar to Object is not a DataPagerField.. /// internal static string PagerFieldCollection_InvalidType { get { return ResourceManager.GetString("PagerFieldCollection_InvalidType", resourceCulture); } } ////// Looks up a localized string similar to Type index is out of bounds.. /// internal static string PagerFieldCollection_InvalidTypeIndex { get { return ResourceManager.GetString("PagerFieldCollection_InvalidTypeIndex", resourceCulture); } } ////// Looks up a localized string similar to {0} (at index {1}). /// internal static string ParseException_ParseExceptionFormat { get { return ResourceManager.GetString("ParseException_ParseExceptionFormat", resourceCulture); } } ////// Looks up a localized string similar to The attribute 'LoadProperties' can only be used when using the default ProfileService.. /// internal static string ProfileServiceManager_LoadProperitesWithNonDefaultPath { get { return ResourceManager.GetString("ProfileServiceManager_LoadProperitesWithNonDefaultPath", resourceCulture); } } ////// Looks up a localized string similar to Specifies profile properties that should be rendered inline with the page.. /// internal static string ProfileServiceManager_LoadProperties { get { return ResourceManager.GetString("ProfileServiceManager_LoadProperties", resourceCulture); } } ////// Looks up a localized string similar to Type {0} is not supported.. /// internal static string ProxyGenerator_UnsupportedType { get { return ResourceManager.GetString("ProxyGenerator_UnsupportedType", resourceCulture); } } ////// Looks up a localized string similar to Error status code returned by the Web Service: {0}. Error details from service: {1}. /// internal static string ProxyHelper_BadStatusCode { get { return ResourceManager.GetString("ProxyHelper_BadStatusCode", resourceCulture); } } ////// Looks up a localized string similar to Role Provider could not be found.. /// internal static string RoleService_RoleProviderNotFound { get { return ResourceManager.GetString("RoleService_RoleProviderNotFound", resourceCulture); } } ////// Looks up a localized string similar to The Role Manager feature has not been enabled.. /// internal static string RoleService_RolesFeatureNotEnabled { get { return ResourceManager.GetString("RoleService_RolesFeatureNotEnabled", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether user roles are rendered inline with the page.. /// internal static string RoleServiceManager_LoadRoles { get { return ResourceManager.GetString("RoleServiceManager_LoadRoles", resourceCulture); } } ////// Looks up a localized string similar to For RoleService, 'loadRoles' property must be set to false when the 'path' property is set to a value different from the default value.. /// internal static string RoleServiceManager_LoadRolesWithNonDefaultPath { get { return ResourceManager.GetString("RoleServiceManager_LoadRolesWithNonDefaultPath", resourceCulture); } } ////// Looks up a localized string similar to The 'ID' property on ScriptControlDescriptor is not settable. The client ID of a script control is always equal to its element ID.. /// internal static string ScriptControlDescriptor_IDNotSettable { get { return ResourceManager.GetString("ScriptControlDescriptor_IDNotSettable", resourceCulture); } } ////// Looks up a localized string similar to Extender control '{0}' is not a registered extender control. Extender controls must be registered using RegisterExtenderControl() before calling RegisterScriptDescriptors().. /// internal static string ScriptControlManager_ExtenderControlNotRegistered { get { return ResourceManager.GetString("ScriptControlManager_ExtenderControlNotRegistered", resourceCulture); } } ////// Looks up a localized string similar to Extender control type '{0}' does not have any attributes of type '{1}'. Extender control types must have at least one attribute of type '{1}'.. /// internal static string ScriptControlManager_NoTargetControlTypes { get { return ResourceManager.GetString("ScriptControlManager_NoTargetControlTypes", resourceCulture); } } ////// Looks up a localized string similar to Extender controls may not be registered before PreRender.. /// internal static string ScriptControlManager_RegisterExtenderControlTooEarly { get { return ResourceManager.GetString("ScriptControlManager_RegisterExtenderControlTooEarly", resourceCulture); } } ////// Looks up a localized string similar to Extender controls may not be registered after PreRender.. /// internal static string ScriptControlManager_RegisterExtenderControlTooLate { get { return ResourceManager.GetString("ScriptControlManager_RegisterExtenderControlTooLate", resourceCulture); } } ////// Looks up a localized string similar to Script controls may not be registered before PreRender.. /// internal static string ScriptControlManager_RegisterScriptControlTooEarly { get { return ResourceManager.GetString("ScriptControlManager_RegisterScriptControlTooEarly", resourceCulture); } } ////// Looks up a localized string similar to Script controls may not be registered after PreRender.. /// internal static string ScriptControlManager_RegisterScriptControlTooLate { get { return ResourceManager.GetString("ScriptControlManager_RegisterScriptControlTooLate", resourceCulture); } } ////// Looks up a localized string similar to Script control '{0}' is not a registered script control. Script controls must be registered using RegisterScriptControl() before calling RegisterScriptDescriptors().. /// internal static string ScriptControlManager_ScriptControlNotRegistered { get { return ResourceManager.GetString("ScriptControlManager_ScriptControlNotRegistered", resourceCulture); } } ////// Looks up a localized string similar to Extender control '{0}' cannot extend '{1}'. Extender controls of type '{2}' cannot extend controls of type '{3}'.. /// internal static string ScriptControlManager_TargetControlTypeInvalid { get { return ResourceManager.GetString("ScriptControlManager_TargetControlTypeInvalid", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether custom error redirects will occur during an async postback.. /// internal static string ScriptManager_AllowCustomErrorsRedirect { get { return ResourceManager.GetString("ScriptManager_AllowCustomErrorsRedirect", resourceCulture); } } ////// Looks up a localized string similar to This event is raised to allow customization of the error message sent to the client during an async postback.. /// internal static string ScriptManager_AsyncPostBackError { get { return ResourceManager.GetString("ScriptManager_AsyncPostBackError", resourceCulture); } } ////// Looks up a localized string similar to The error message to be sent to the client when an unhandled exception occurs on the server. The property can be set declaratively in the page markup or during the ScriptManager's AsyncPostBackError event. If the value is empty the exception's message will be used.. /// internal static string ScriptManager_AsyncPostBackErrorMessage { get { return ResourceManager.GetString("ScriptManager_AsyncPostBackErrorMessage", resourceCulture); } } ////// Looks up a localized string similar to The page is performing an async postback but the ScriptManager.SupportsPartialRendering property is set to false. Ensure that the property is set to true during an async postback.. /// internal static string ScriptManager_AsyncPostBackNotInPartialRenderingMode { get { return ResourceManager.GetString("ScriptManager_AsyncPostBackNotInPartialRenderingMode", resourceCulture); } } ////// Looks up a localized string similar to The timeout period in seconds for async postbacks. A value of zero indicates no timeout.. /// internal static string ScriptManager_AsyncPostBackTimeout { get { return ResourceManager.GetString("ScriptManager_AsyncPostBackTimeout", resourceCulture); } } ////// Looks up a localized string similar to Contains preferences for the client side authentication service.. /// internal static string ScriptManager_AuthenticationService { get { return ResourceManager.GetString("ScriptManager_AuthenticationService", resourceCulture); } } ////// Looks up a localized string similar to A history point can only be created during an asynchronous postback.. /// internal static string ScriptManager_CannotAddHistoryPointOutsideOfAsyncPostBack { get { return ResourceManager.GetString("ScriptManager_CannotAddHistoryPointOutsideOfAsyncPostBack", resourceCulture); } } ////// Looks up a localized string similar to A history point can only be added if EnableHistory is set to true.. /// internal static string ScriptManager_CannotAddHistoryPointWithHistoryDisabled { get { return ResourceManager.GetString("ScriptManager_CannotAddHistoryPointWithHistoryDisabled", resourceCulture); } } ////// Looks up a localized string similar to The EnableHistory property cannot be changed after the Init event.. /// internal static string ScriptManager_CannotChangeEnableHistory { get { return ResourceManager.GetString("ScriptManager_CannotChangeEnableHistory", resourceCulture); } } ////// Looks up a localized string similar to The EnablePartialRendering property cannot be changed after the Init event.. /// internal static string ScriptManager_CannotChangeEnablePartialRendering { get { return ResourceManager.GetString("ScriptManager_CannotChangeEnablePartialRendering", resourceCulture); } } ////// Looks up a localized string similar to The EnableScriptGlobalization property cannot be changed during async postbacks or after the Init event.. /// internal static string ScriptManager_CannotChangeEnableScriptGlobalization { get { return ResourceManager.GetString("ScriptManager_CannotChangeEnableScriptGlobalization", resourceCulture); } } ////// Looks up a localized string similar to The SupportsPartialRendering property cannot be changed after the Init event.. /// internal static string ScriptManager_CannotChangeSupportsPartialRendering { get { return ResourceManager.GetString("ScriptManager_CannotChangeSupportsPartialRendering", resourceCulture); } } ////// Looks up a localized string similar to Control with ID '{0}' cannot be registered through both RegisterAsyncPostBackControl and RegisterPostBackControl. This can happen if you have conflicting triggers associated with the target control.. /// internal static string ScriptManager_CannotRegisterBothPostBacks { get { return ResourceManager.GetString("ScriptManager_CannotRegisterBothPostBacks", resourceCulture); } } ////// Looks up a localized string similar to A script reference cannot be included multiple times in composite script references.. /// internal static string ScriptManager_CannotRegisterScriptInMultipleCompositeReferences { get { return ResourceManager.GetString("ScriptManager_CannotRegisterScriptInMultipleCompositeReferences", resourceCulture); } } ////// Looks up a localized string similar to The SupportsPartialRendering property cannot be set when EnablePartialRendering is false.. /// internal static string ScriptManager_CannotSetSupportsPartialRenderingWhenDisabled { get { return ResourceManager.GetString("ScriptManager_CannotSetSupportsPartialRenderingWhenDisabled", resourceCulture); } } ////// Looks up a localized string similar to Specifies a client-side event handler name for the navigate event.. /// internal static string ScriptManager_ClientNavigateHandler { get { return ResourceManager.GetString("ScriptManager_ClientNavigateHandler", resourceCulture); } } ////// Looks up a localized string similar to Enables the composition of individual script references into one to minimize the number of requests to the server.. /// internal static string ScriptManager_CompositeScript { get { return ResourceManager.GetString("ScriptManager_CompositeScript", resourceCulture); } } ////// Looks up a localized string similar to The URL of an empty page that will be used to manage history on Internet Explorer. The script manager uses a built-in, resource-based page if this property is unspecified.. /// internal static string ScriptManager_EmptyPageUrl { get { return ResourceManager.GetString("ScriptManager_EmptyPageUrl", resourceCulture); } } ////// Looks up a localized string similar to Enables ScriptManager to manage browser history on supported browsers.. /// internal static string ScriptManager_EnableHistory { get { return ResourceManager.GetString("ScriptManager_EnableHistory", resourceCulture); } } ////// Looks up a localized string similar to Enables page methods.. /// internal static string ScriptManager_EnablePageMethods { get { return ResourceManager.GetString("ScriptManager_EnablePageMethods", resourceCulture); } } ////// Looks up a localized string similar to Enables asynchronous postbacks for the UpdatePanel control on supported browsers. To override the default browser support detection you can set the SupportsPartialRendering property.. /// internal static string ScriptManager_EnablePartialRendering { get { return ResourceManager.GetString("ScriptManager_EnablePartialRendering", resourceCulture); } } ////// Looks up a localized string similar to Enables ScriptManager to add client-side globalization information to the page for the current culture.. /// internal static string ScriptManager_EnableScriptGlobalization { get { return ResourceManager.GetString("ScriptManager_EnableScriptGlobalization", resourceCulture); } } ////// Looks up a localized string similar to Enables ScriptManager to generate localized versions of script files if they are available.. /// internal static string ScriptManager_EnableScriptLocalization { get { return ResourceManager.GetString("ScriptManager_EnableScriptLocalization", resourceCulture); } } ////// Looks up a localized string similar to When true, the server-side history state is hashed using the same settings as ViewState. When false, the server history state is a clear-text string dictionary that can be modified by the end user by modifying the url.. /// internal static string ScriptManager_EnableSecureHistoryState { get { return ResourceManager.GetString("ScriptManager_EnableSecureHistoryState", resourceCulture); } } ////// Looks up a localized string similar to ASP.NET Ajax client-side framework failed to load.. /// internal static string ScriptManager_FrameworkFailedToLoad { get { return ResourceManager.GetString("ScriptManager_FrameworkFailedToLoad", resourceCulture); } } ////// Looks up a localized string similar to Control with ID '{0}' being registered through RegisterAsyncPostBackControl or RegisterPostBackControl must implement either INamingContainer, IPostBackDataHandler, or IPostBackEventHandler.. /// internal static string ScriptManager_InvalidControlRegistration { get { return ResourceManager.GetString("ScriptManager_InvalidControlRegistration", resourceCulture); } } ////// Looks up a localized string similar to Specifies that script references should be loaded before the UI is rendered in the browser.. /// internal static string ScriptManager_LoadScriptsBeforeUI { get { return ResourceManager.GetString("ScriptManager_LoadScriptsBeforeUI", resourceCulture); } } ////// Looks up a localized string similar to This event is raised during asynchronous postbacks when the server-side history state changes.. /// internal static string ScriptManager_Navigate { get { return ResourceManager.GetString("ScriptManager_Navigate", resourceCulture); } } ////// Looks up a localized string similar to Only one instance of a ScriptManager can be added to the page.. /// internal static string ScriptManager_OnlyOneScriptManager { get { return ResourceManager.GetString("ScriptManager_OnlyOneScriptManager", resourceCulture); } } ////// Looks up a localized string similar to Untitled Page. /// internal static string ScriptManager_PageUntitled { get { return ResourceManager.GetString("ScriptManager_PageUntitled", resourceCulture); } } ////// Looks up a localized string similar to Contains preferences for the client side profile service.. /// internal static string ScriptManager_ProfileService { get { return ResourceManager.GetString("ScriptManager_ProfileService", resourceCulture); } } ////// Looks up a localized string similar to This event is raised to allow modifications to composite script references before they are rendered.. /// internal static string ScriptManager_ResolveCompositeScriptReference { get { return ResourceManager.GetString("ScriptManager_ResolveCompositeScriptReference", resourceCulture); } } ////// Looks up a localized string similar to This event is raised to allow modifications to script references before they are rendered.. /// internal static string ScriptManager_ResolveScriptReference { get { return ResourceManager.GetString("ScriptManager_ResolveScriptReference", resourceCulture); } } ////// Looks up a localized string similar to Contains preferences for the client side authentication service.. /// internal static string ScriptManager_RoleService { get { return ResourceManager.GetString("ScriptManager_RoleService", resourceCulture); } } ////// Looks up a localized string similar to Indicates the type of scripts to load when more than one type is available.. /// internal static string ScriptManager_ScriptMode { get { return ResourceManager.GetString("ScriptManager_ScriptMode", resourceCulture); } } ////// Looks up a localized string similar to Specifies that scripts should be loaded from this path instead of from assembly web resources.. /// internal static string ScriptManager_ScriptPath { get { return ResourceManager.GetString("ScriptManager_ScriptPath", resourceCulture); } } ////// Looks up a localized string similar to A collection of script references that the ScriptManager should include in the page. The Scripts collections on the ScriptManager and ScriptManagerProxy controls are merged at runtime.. /// internal static string ScriptManager_Scripts { get { return ResourceManager.GetString("ScriptManager_Scripts", resourceCulture); } } ////// Looks up a localized string similar to A collection of service references that the ScriptManager should include in the page. The Services collections on the ScriptManager and ScriptManagerProxy controls are merged at runtime.. /// internal static string ScriptManager_Services { get { return ResourceManager.GetString("ScriptManager_Services", resourceCulture); } } ////// Looks up a localized string similar to Cannot unregister UpdatePanel with ID '{0}' since it was not registered with the ScriptManager. This might occur if the UpdatePanel was removed from the control tree and later added again, which is not supported.. /// internal static string ScriptManager_UpdatePanelNotRegistered { get { return ResourceManager.GetString("ScriptManager_UpdatePanelNotRegistered", resourceCulture); } } ////// Looks up a localized string similar to The assembly that contains the script as a web resource.. /// internal static string ScriptReference_Assembly { get { return ResourceManager.GetString("ScriptReference_Assembly", resourceCulture); } } ////// Looks up a localized string similar to Assembly cannot be defined without Name.. /// internal static string ScriptReference_AssemblyRequiresName { get { return ResourceManager.GetString("ScriptReference_AssemblyRequiresName", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether this script reference should ignore the ScriptManager.ScriptPath property.. /// internal static string ScriptReference_IgnoreScriptPath { get { return ResourceManager.GetString("ScriptReference_IgnoreScriptPath", resourceCulture); } } ////// Looks up a localized string similar to '{0}' is not a valid script name. The name must end in '.js'.. /// internal static string ScriptReference_InvalidReleaseScriptName { get { return ResourceManager.GetString("ScriptReference_InvalidReleaseScriptName", resourceCulture); } } ////// Looks up a localized string similar to '{0}' is not a valid script path. The path must end in '.js'.. /// internal static string ScriptReference_InvalidReleaseScriptPath { get { return ResourceManager.GetString("ScriptReference_InvalidReleaseScriptPath", resourceCulture); } } ////// Looks up a localized string similar to The name of the web resource.. /// internal static string ScriptReference_Name { get { return ResourceManager.GetString("ScriptReference_Name", resourceCulture); } } ////// Looks up a localized string similar to Name and Path cannot both be empty.. /// internal static string ScriptReference_NameAndPathCannotBeEmpty { get { return ResourceManager.GetString("ScriptReference_NameAndPathCannotBeEmpty", resourceCulture); } } ////// Looks up a localized string similar to Specifies if the script resource loader should automatically append a script loaded notification statement.. /// internal static string ScriptReference_NotifyScriptLoaded { get { return ResourceManager.GetString("ScriptReference_NotifyScriptLoaded", resourceCulture); } } ////// Looks up a localized string similar to The path to the script.. /// internal static string ScriptReference_Path { get { return ResourceManager.GetString("ScriptReference_Path", resourceCulture); } } ////// Looks up a localized string similar to A comma-delimited string of valid UI cultures supported by the path. ResourceUICultures is only valid with Path.. /// internal static string ScriptReference_ResourceUICultures { get { return ResourceManager.GetString("ScriptReference_ResourceUICultures", resourceCulture); } } ////// Looks up a localized string similar to Specifies the algorithm for choosing between the debug and release scripts.. /// internal static string ScriptReference_ScriptMode { get { return ResourceManager.GetString("ScriptReference_ScriptMode", resourceCulture); } } ////// Looks up a localized string similar to The control must be in the control tree of a page.. /// internal static string ScriptRegistrationManager_ControlNotOnPage { get { return ResourceManager.GetString("ScriptRegistrationManager_ControlNotOnPage", resourceCulture); } } ////// Looks up a localized string similar to The script tag registered for type '{0}' and key '{1}' has invalid characters outside of the script tags: {2}. Only properly formatted script tags can be registered.. /// internal static string ScriptRegistrationManager_InvalidChars { get { return ResourceManager.GetString("ScriptRegistrationManager_InvalidChars", resourceCulture); } } ////// Looks up a localized string similar to The script tag registered for type '{0}' and key '{1}' is missing a matching close tag.. /// internal static string ScriptRegistrationManager_NoCloseTag { get { return ResourceManager.GetString("ScriptRegistrationManager_NoCloseTag", resourceCulture); } } ////// Looks up a localized string similar to The script tag registered for type '{0}' and key '{1}' does not contain any valid script tags.. /// internal static string ScriptRegistrationManager_NoTags { get { return ResourceManager.GetString("ScriptRegistrationManager_NoTags", resourceCulture); } } ////// Looks up a localized string similar to Script resource handler can only serve resources from file-based assemblies.. /// internal static string ScriptResourceHandler_AssemblyNotFileBased { get { return ResourceManager.GetString("ScriptResourceHandler_AssemblyNotFileBased", resourceCulture); } } ////// Looks up a localized string similar to More than one ScriptResourceAttribute points to script '{0}' in assembly '{1}'.. /// internal static string ScriptResourceHandler_DuplicateScriptResources { get { return ResourceManager.GetString("ScriptResourceHandler_DuplicateScriptResources", resourceCulture); } } ////// Looks up a localized string similar to This is an invalid script resource request.. /// internal static string ScriptResourceHandler_InvalidRequest { get { return ResourceManager.GetString("ScriptResourceHandler_InvalidRequest", resourceCulture); } } ////// Looks up a localized string similar to The resource URL cannot be longer than 1024 characters. If using a CompositeScriptReference, reduce the number of ScriptReferences it contains, or combine them into a single static file and set the Path property to the location of it.. /// internal static string ScriptResourceHandler_ResourceUrlLongerThan1024Characters { get { return ResourceManager.GetString("ScriptResourceHandler_ResourceUrlLongerThan1024Characters", resourceCulture); } } ////// Looks up a localized string similar to The type names for the debug and release versions of resource {0} don't match.. /// internal static string ScriptResourceHandler_TypeNameMismatch { get { return ResourceManager.GetString("ScriptResourceHandler_TypeNameMismatch", resourceCulture); } } ////// Looks up a localized string similar to Web resource '{0}' was not found.. /// internal static string ScriptResourceHandler_UnknownResource { get { return ResourceManager.GetString("ScriptResourceHandler_UnknownResource", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether this service reference should have its proxy script rendered inline in the page.. /// internal static string ServiceReference_InlineScript { get { return ResourceManager.GetString("ServiceReference_InlineScript", resourceCulture); } } ////// Looks up a localized string similar to The path to the service being referenced.. /// internal static string ServiceReference_Path { get { return ResourceManager.GetString("ServiceReference_Path", resourceCulture); } } ////// Looks up a localized string similar to Path cannot be empty.. /// internal static string ServiceReference_PathCannotBeEmpty { get { return ResourceManager.GetString("ServiceReference_PathCannotBeEmpty", resourceCulture); } } ////// Looks up a localized string similar to The serviceUri configuration setting was not found.. /// internal static string ServiceUriNotFound { get { return ResourceManager.GetString("ServiceUriNotFound", resourceCulture); } } ////// Looks up a localized string similar to Unable to connect to the Microsoft SQL Everywhere Service using the specified connection string. Make sure that Microsoft SQL Server Everywhere is correctly installed on this computer.. /// internal static string SqlHelper_SqlEverywhereNotInstalled { get { return ResourceManager.GetString("SqlHelper_SqlEverywhereNotInstalled", resourceCulture); } } ////// Looks up a localized string similar to Fires when an event is generated within the pager field.. /// internal static string TemplatePagerField_OnPagerCommand { get { return ResourceManager.GetString("TemplatePagerField_OnPagerCommand", resourceCulture); } } ////// Looks up a localized string similar to The template used in the pager field.. /// internal static string TemplatePagerField_PagerTemplate { get { return ResourceManager.GetString("TemplatePagerField_PagerTemplate", resourceCulture); } } ////// Looks up a localized string similar to The TemplatePagerField raised event {0} which wasn't handled.. /// internal static string TemplatePagerField_UnhandledEvent { get { return ResourceManager.GetString("TemplatePagerField_UnhandledEvent", resourceCulture); } } ////// Looks up a localized string similar to The interval must be greater than zero.. /// internal static string Timer_IntervalMustBeGreaterThanZero { get { return ResourceManager.GetString("Timer_IntervalMustBeGreaterThanZero", resourceCulture); } } ////// Looks up a localized string similar to Enables raising of Tick events.. /// internal static string Timer_TimerEnable { get { return ResourceManager.GetString("Timer_TimerEnable", resourceCulture); } } ////// Looks up a localized string similar to The duration between Tick events in milliseconds.. /// internal static string Timer_TimerInterval { get { return ResourceManager.GetString("Timer_TimerInterval", resourceCulture); } } ////// Looks up a localized string similar to Occurs whenever the specified interval time elapses.. /// internal static string Timer_TimerTick { get { return ResourceManager.GetString("Timer_TimerTick", resourceCulture); } } ////// Looks up a localized string similar to An unhandled exception has occurred.. /// internal static string UnhandledExceptionEventLogMessage { get { return ResourceManager.GetString("UnhandledExceptionEventLogMessage", resourceCulture); } } ////// Looks up a localized string similar to The Controls property of UpdatePanel with ID '{0}' cannot be modified directly. To change the contents of the UpdatePanel modify the child controls of the ContentTemplateContainer property.. /// internal static string UpdatePanel_CannotModifyControlCollection { get { return ResourceManager.GetString("UpdatePanel_CannotModifyControlCollection", resourceCulture); } } ////// Looks up a localized string similar to The ContentTemplate of UpdatePanel with ID '{0}' cannot be changed after it has been instantiated.. /// internal static string UpdatePanel_CannotSetContentTemplate { get { return ResourceManager.GetString("UpdatePanel_CannotSetContentTemplate", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether postbacks coming from the UpdatePanel's child controls will cause the UpdatePanel to refresh.. /// internal static string UpdatePanel_ChildrenAsTriggers { get { return ResourceManager.GetString("UpdatePanel_ChildrenAsTriggers", resourceCulture); } } ////// Looks up a localized string similar to ChildrenAsTriggers cannot be set to false when UpdateMode is set to Always on UpdatePanel '{0}'.. /// internal static string UpdatePanel_ChildrenTriggersAndUpdateAlways { get { return ResourceManager.GetString("UpdatePanel_ChildrenTriggersAndUpdateAlways", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether the UpdatePanel should render as a block tag (<div>) or an inline tag (<span>).. /// internal static string UpdatePanel_RenderMode { get { return ResourceManager.GetString("UpdatePanel_RenderMode", resourceCulture); } } ////// Looks up a localized string similar to SetPartialRenderingMode can only be called once.. /// internal static string UpdatePanel_SetPartialRenderingModeCalledOnce { get { return ResourceManager.GetString("UpdatePanel_SetPartialRenderingModeCalledOnce", resourceCulture); } } ////// Looks up a localized string similar to A collection of triggers that can cause the UpdatePanel to be updated.. /// internal static string UpdatePanel_Triggers { get { return ResourceManager.GetString("UpdatePanel_Triggers", resourceCulture); } } ////// Looks up a localized string similar to The Update method can only be called on UpdatePanel with ID '{0}' when UpdateMode is set to Conditional.. /// internal static string UpdatePanel_UpdateConditional { get { return ResourceManager.GetString("UpdatePanel_UpdateConditional", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether the UpdatePanel will refresh on every asynchronous postback or only as the result of a specific action, such as a call to UpdatePanel.Update().. /// internal static string UpdatePanel_UpdateMode { get { return ResourceManager.GetString("UpdatePanel_UpdateMode", resourceCulture); } } ////// Looks up a localized string similar to The Update method can only be called on UpdatePanel with ID '{0}' before Render.. /// internal static string UpdatePanel_UpdateTooLate { get { return ResourceManager.GetString("UpdatePanel_UpdateTooLate", resourceCulture); } } ////// Looks up a localized string similar to The trigger's target control ID.. /// internal static string UpdatePanelControlTrigger_ControlID { get { return ResourceManager.GetString("UpdatePanelControlTrigger_ControlID", resourceCulture); } } ////// Looks up a localized string similar to A control with ID '{0}' could not be found for the trigger in UpdatePanel '{1}'.. /// internal static string UpdatePanelControlTrigger_ControlNotFound { get { return ResourceManager.GetString("UpdatePanelControlTrigger_ControlNotFound", resourceCulture); } } ////// Looks up a localized string similar to The ControlID property must be set on the trigger in UpdatePanel '{0}'.. /// internal static string UpdatePanelControlTrigger_NoControlID { get { return ResourceManager.GetString("UpdatePanelControlTrigger_NoControlID", resourceCulture); } } ////// Looks up a localized string similar to UpdatePanel that this UpdateProgress is associated with.. /// internal static string UpdateProgress_AssociatedUpdatePanelID { get { return ResourceManager.GetString("UpdateProgress_AssociatedUpdatePanelID", resourceCulture); } } ////// Looks up a localized string similar to Time in ms after which the ProgressTemplate is displayed.. /// internal static string UpdateProgress_DisplayAfter { get { return ResourceManager.GetString("UpdateProgress_DisplayAfter", resourceCulture); } } ////// Looks up a localized string similar to DisplayAfter must be a non negative integer.. /// internal static string UpdateProgress_DisplayAfterInvalid { get { return ResourceManager.GetString("UpdateProgress_DisplayAfterInvalid", resourceCulture); } } ////// Looks up a localized string similar to Determines whether the progress template is dynamically rendered.. /// internal static string UpdateProgress_DynamicLayout { get { return ResourceManager.GetString("UpdateProgress_DynamicLayout", resourceCulture); } } ////// Looks up a localized string similar to No UpdatePanel found for AssociatedUpdatePanelID '{0}'.. /// internal static string UpdateProgress_NoUpdatePanel { get { return ResourceManager.GetString("UpdateProgress_NoUpdatePanel", resourceCulture); } } ////// Looks up a localized string similar to ProgressTemplate which is displayed during async postbacks.. /// internal static string UpdateProgress_ProgressTemplate { get { return ResourceManager.GetString("UpdateProgress_ProgressTemplate", resourceCulture); } } ////// Looks up a localized string similar to A ProgressTemplate must be specified on UpdateProgress control with ID '{0}'.. /// internal static string UpdateProgress_TemplateRequired { get { return ResourceManager.GetString("UpdateProgress_TemplateRequired", resourceCulture); } } ////// Looks up a localized string similar to You must log on to call this method.. /// internal static string UserIsNotAuthenticated { get { return ResourceManager.GetString("UserIsNotAuthenticated", resourceCulture); } } ////// Looks up a localized string similar to Assembly '{0}' does not contain a Web resource with name '{1}'. Setting the ScriptReference.ScriptMode property to ScriptMode.Auto or ScriptMode.Release will cause the release script to be used.. /// internal static string WebResourceUtil_AssemblyDoesNotContainDebugWebResource { get { return ResourceManager.GetString("WebResourceUtil_AssemblyDoesNotContainDebugWebResource", resourceCulture); } } ////// Looks up a localized string similar to Assembly '{0}' contains a Web resource with name '{1}', but does not contain an embedded resource with name '{1}'.. /// internal static string WebResourceUtil_AssemblyDoesNotContainEmbeddedResource { get { return ResourceManager.GetString("WebResourceUtil_AssemblyDoesNotContainEmbeddedResource", resourceCulture); } } ////// Looks up a localized string similar to Assembly '{0}' does not contain a Web resource with name '{1}'.. /// internal static string WebResourceUtil_AssemblyDoesNotContainReleaseWebResource { get { return ResourceManager.GetString("WebResourceUtil_AssemblyDoesNotContainReleaseWebResource", resourceCulture); } } ////// Looks up a localized string similar to There was an error processing the request.. /// internal static string WebService_Error { get { return ResourceManager.GetString("WebService_Error", resourceCulture); } } ////// Looks up a localized string similar to Using the GenerateScriptTypes attribute is not supported for types in the following categories: primitive types; DateTime; generic types taking more than one parameter; types implementing IEnumerable or IDictionary; interfaces; Abstract classes; classes without a public default constructor.. /// internal static string WebService_InvalidGenerateScriptType { get { return ResourceManager.GetString("WebService_InvalidGenerateScriptType", resourceCulture); } } ////// Looks up a localized string similar to The path "{0}" is not supported. When InlineScript=true, the path should be a relative path pointing to the same web application as the current page.. /// internal static string WebService_InvalidInlineVirtualPath { get { return ResourceManager.GetString("WebService_InvalidInlineVirtualPath", resourceCulture); } } ////// Looks up a localized string similar to An attempt was made to call the method '{0}' using a {1} request, which is not allowed.. /// internal static string WebService_InvalidVerbRequest { get { return ResourceManager.GetString("WebService_InvalidVerbRequest", resourceCulture); } } ////// Looks up a localized string similar to Invalid web service call, expected path info of /js/<Method>.. /// internal static string WebService_InvalidWebServiceCall { get { return ResourceManager.GetString("WebService_InvalidWebServiceCall", resourceCulture); } } ////// Looks up a localized string similar to The method '{0}' returns a value of type '{1}', which cannot be serialized as Xml. Original error: {2}. /// internal static string WebService_InvalidXmlReturnType { get { return ResourceManager.GetString("WebService_InvalidXmlReturnType", resourceCulture); } } ////// Looks up a localized string similar to Invalid web service call, missing value for parameter: '{0}'.. /// internal static string WebService_MissingArg { get { return ResourceManager.GetString("WebService_MissingArg", resourceCulture); } } ////// Looks up a localized string similar to Only Web services with a [ScriptService] attribute on the class definition can be called from script.. /// internal static string WebService_NoScriptServiceAttribute { get { return ResourceManager.GetString("WebService_NoScriptServiceAttribute", resourceCulture); } } ////// Looks up a localized string similar to No web service found at: {0}.. /// internal static string WebService_NoWebServiceData { get { return ResourceManager.GetString("WebService_NoWebServiceData", resourceCulture); } } ////// Looks up a localized string similar to No web service found at: {0}. This error can occur if a ServiceReference to a WCF service has InlineScript set to 'true'. For WCF services InlineScript should be 'false'.. /// internal static string WebService_NoWebServiceDataInlineScript { get { return ResourceManager.GetString("WebService_NoWebServiceDataInlineScript", resourceCulture); } } ////// Looks up a localized string similar to Authentication failed.. /// internal static string WebService_RedirectError { get { return ResourceManager.GetString("WebService_RedirectError", resourceCulture); } } ////// Looks up a localized string similar to Unknown web method {0}.. /// internal static string WebService_UnknownWebMethod { get { return ResourceManager.GetString("WebService_UnknownWebMethod", resourceCulture); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// This code was generated by a tool. // Runtime Version:2.0.50727.1433 // // Changes to this file may cause incorrect behavior and will be lost if // the code is regenerated. // //----------------------------------------------------------------------------- namespace System.Web.Resources { using System; ////// A strongly-typed resource class, for looking up localized strings, etc. /// // This class was auto-generated by the StronglyTypedResourceBuilder // class via a tool like ResGen or Visual Studio. // To add or remove a member, edit your .ResX file then rerun ResGen // with the /str option, or rebuild your VS project. [global::System.CodeDom.Compiler.GeneratedCodeAttribute("System.Resources.Tools.StronglyTypedResourceBuilder", "2.0.0.0")] [global::System.Diagnostics.DebuggerNonUserCodeAttribute()] [global::System.Runtime.CompilerServices.CompilerGeneratedAttribute()] internal class AtlasWeb { private static global::System.Resources.ResourceManager resourceMan; private static global::System.Globalization.CultureInfo resourceCulture; [global::System.Diagnostics.CodeAnalysis.SuppressMessageAttribute("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal AtlasWeb() { } ////// Returns the cached ResourceManager instance used by this class. /// [global::System.ComponentModel.EditorBrowsableAttribute(global::System.ComponentModel.EditorBrowsableState.Advanced)] internal static global::System.Resources.ResourceManager ResourceManager { get { if (object.ReferenceEquals(resourceMan, null)) { global::System.Resources.ResourceManager temp = new global::System.Resources.ResourceManager("System.Web.Resources.AtlasWeb", typeof(AtlasWeb).Assembly); resourceMan = temp; } return resourceMan; } } ////// Overrides the current thread's CurrentUICulture property for all /// resource lookups using this strongly typed resource class. /// [global::System.ComponentModel.EditorBrowsableAttribute(global::System.ComponentModel.EditorBrowsableState.Advanced)] internal static global::System.Globalization.CultureInfo Culture { get { return resourceCulture; } set { resourceCulture = value; } } ////// Looks up a localized string similar to Specifies the path to the web service.. /// internal static string ApplicationServiceManager_Path { get { return ResourceManager.GetString("ApplicationServiceManager_Path", resourceCulture); } } ////// Looks up a localized string similar to {0} is disabled.. /// internal static string AppService_Disabled { get { return ResourceManager.GetString("AppService_Disabled", resourceCulture); } } ////// Looks up a localized string similar to Cannot specify more than one unique path.. /// internal static string AppService_MultiplePaths { get { return ResourceManager.GetString("AppService_MultiplePaths", resourceCulture); } } ////// Looks up a localized string similar to SSL is required for this operation.. /// internal static string AppService_RequiredSSL { get { return ResourceManager.GetString("AppService_RequiredSSL", resourceCulture); } } ////// Looks up a localized string similar to Unknown profile property '{0}'.. /// internal static string AppService_UnknownProfileProperty { get { return ResourceManager.GetString("AppService_UnknownProfileProperty", resourceCulture); } } ////// Looks up a localized string similar to Argument must be null, empty or same as the current user.. /// internal static string ArgumentMustBeCurrentUser { get { return ResourceManager.GetString("ArgumentMustBeCurrentUser", resourceCulture); } } ////// Looks up a localized string similar to Argument must be null or empty.. /// internal static string ArgumentMustBeNull { get { return ResourceManager.GetString("ArgumentMustBeNull", resourceCulture); } } ////// Looks up a localized string similar to Could not find an event named '{0}' on associated control '{1}' for the trigger in UpdatePanel '{2}'.. /// internal static string AsyncPostBackTrigger_CannotFindEvent { get { return ResourceManager.GetString("AsyncPostBackTrigger_CannotFindEvent", resourceCulture); } } ////// Looks up a localized string similar to The event that the trigger will hook up to determine whether to refresh the UpdatePanel. If the property is not set then the UpdatePanel will be refreshed only if the postback was initiated by the target control.. /// internal static string AsyncPostBackTrigger_EventName { get { return ResourceManager.GetString("AsyncPostBackTrigger_EventName", resourceCulture); } } ////// Looks up a localized string similar to The '{0}' event on associated control '{1}' for the trigger in UpdatePanel '{2}' does not match the standard event handler signature.. /// internal static string AsyncPostBackTrigger_InvalidEvent { get { return ResourceManager.GetString("AsyncPostBackTrigger_InvalidEvent", resourceCulture); } } ////// Looks up a localized string similar to The following configuration attribute was not recognized: '{0}'. /// internal static string AttributeNotRecognized { get { return ResourceManager.GetString("AttributeNotRecognized", resourceCulture); } } ////// Looks up a localized string similar to Sorting. /// internal static string Category_Sorting { get { return ResourceManager.GetString("Category_Sorting", resourceCulture); } } ////// Looks up a localized string similar to The server method returned invalid data.. /// internal static string ClientService_BadJsonResponse { get { return ResourceManager.GetString("ClientService_BadJsonResponse", resourceCulture); } } ////// Looks up a localized string similar to Value must be of type '{0}'.. /// internal static string Common_ArgumentInvalidType { get { return ResourceManager.GetString("Common_ArgumentInvalidType", resourceCulture); } } ////// Looks up a localized string similar to Value must be greater than or equal to 0.. /// internal static string Common_GreaterThanOrEqualToZero { get { return ResourceManager.GetString("Common_GreaterThanOrEqualToZero", resourceCulture); } } ////// Looks up a localized string similar to Value must be greater than or equal to 0 and less than or equal to 1.. /// internal static string Common_GreaterThanOrEqualToZeroAndLessThanOrEqualToOne { get { return ResourceManager.GetString("Common_GreaterThanOrEqualToZeroAndLessThanOrEqualToOne", resourceCulture); } } ////// Looks up a localized string similar to Value cannot be null or empty.. /// internal static string Common_NullOrEmpty { get { return ResourceManager.GetString("Common_NullOrEmpty", resourceCulture); } } ////// Looks up a localized string similar to Page cannot be null. Please ensure that this operation is being performed in the context of an ASP.NET request.. /// internal static string Common_PageCannotBeNull { get { return ResourceManager.GetString("Common_PageCannotBeNull", resourceCulture); } } ////// Looks up a localized string similar to The control with ID '{0}' requires a ScriptManager on the page. The ScriptManager must appear before any controls that need it.. /// internal static string Common_ScriptManagerRequired { get { return ResourceManager.GetString("Common_ScriptManagerRequired", resourceCulture); } } ////// Looks up a localized string similar to A collection of script references that the CompositeScriptReference should include in the page.. /// internal static string CompositeScriptReference_Scripts { get { return ResourceManager.GetString("CompositeScriptReference_Scripts", resourceCulture); } } ////// Looks up a localized string similar to Type: '{0}' does not inherits from JavaScriptConverter.. /// internal static string ConvertersCollection_NotJavaScriptConverter { get { return ResourceManager.GetString("ConvertersCollection_NotJavaScriptConverter", resourceCulture); } } ////// Looks up a localized string similar to Type: '{0}' cannot be found.. /// internal static string ConvertersCollection_UnknownType { get { return ResourceManager.GetString("ConvertersCollection_UnknownType", resourceCulture); } } ////// Looks up a localized string similar to The {0} control '{1}' does not have a naming container. Ensure that the control is added to the page before calling DataBind.. /// internal static string DataBoundControlHelper_NoNamingContainer { get { return ResourceManager.GetString("DataBoundControlHelper_NoNamingContainer", resourceCulture); } } ////// Looks up a localized string similar to Control '{0}' does not implement IPageableItemContainer.. /// internal static string DataPager_ControlIsntPageable { get { return ResourceManager.GetString("DataPager_ControlIsntPageable", resourceCulture); } } ////// Looks up a localized string similar to The collection of DataPagerFields.. /// internal static string DataPager_Fields { get { return ResourceManager.GetString("DataPager_Fields", resourceCulture); } } ////// Looks up a localized string similar to The DataPager control '{0}' does not have a naming container. Ensure that the DataPager is added to the page before calling DataBind.. /// internal static string DataPager_NoNamingContainer { get { return ResourceManager.GetString("DataPager_NoNamingContainer", resourceCulture); } } ////// Looks up a localized string similar to No IPageableItemContainer was found. Verify that either the DataPager is inside an IPageableItemContainer or PagedControlID is set to the control ID of an IPageableItemContainer.. /// internal static string DataPager_NoPageableItemContainer { get { return ResourceManager.GetString("DataPager_NoPageableItemContainer", resourceCulture); } } ////// Looks up a localized string similar to IPageableItemContainer '{0}' not found.. /// internal static string DataPager_PageableItemContainerNotFound { get { return ResourceManager.GetString("DataPager_PageableItemContainerNotFound", resourceCulture); } } ////// Looks up a localized string similar to The ID of the control this DataPager should page.. /// internal static string DataPager_PagedControlID { get { return ResourceManager.GetString("DataPager_PagedControlID", resourceCulture); } } ////// Looks up a localized string similar to Page properties cannot be set because no IPageableItemContainer has been found.. /// internal static string DataPager_PagePropertiesCannotBeSet { get { return ResourceManager.GetString("DataPager_PagePropertiesCannotBeSet", resourceCulture); } } ////// Looks up a localized string similar to The number of records displayed in a page by the paged control.. /// internal static string DataPager_PageSize { get { return ResourceManager.GetString("DataPager_PageSize", resourceCulture); } } ////// Looks up a localized string similar to The name of the query string field for the current page index. The pager will use the query string when this property is set.. /// internal static string DataPager_QueryStringField { get { return ResourceManager.GetString("DataPager_QueryStringField", resourceCulture); } } ////// Looks up a localized string similar to Whether the data pager field is visible.. /// internal static string DataPagerField_Visible { get { return ResourceManager.GetString("DataPagerField_Visible", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the field value should be converted to a null reference.. /// internal static string DynamicControlBase_ConvertEmptyStringToNull { get { return ResourceManager.GetString("DynamicControlBase_ConvertEmptyStringToNull", resourceCulture); } } ////// Looks up a localized string similar to Specifies the name of the data field to which the DynamicControl will bind.. /// internal static string DynamicControlBase_DataField { get { return ResourceManager.GetString("DynamicControlBase_DataField", resourceCulture); } } ////// Looks up a localized string similar to Specifies the display format for the field value.. /// internal static string DynamicControlBase_DataFormatString { get { return ResourceManager.GetString("DynamicControlBase_DataFormatString", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the field value is HTML-encoded before it is displayed.. /// internal static string DynamicControlBase_HtmlEncode { get { return ResourceManager.GetString("DynamicControlBase_HtmlEncode", resourceCulture); } } ////// Looks up a localized string similar to Specifies the caption displayed when the field value is null.. /// internal static string DynamicControlBase_NullDisplayText { get { return ResourceManager.GetString("DynamicControlBase_NullDisplayText", resourceCulture); } } ////// Looks up a localized string similar to Specifies the user control with which the field should be rendered.. /// internal static string DynamicControlBase_UIHint { get { return ResourceManager.GetString("DynamicControlBase_UIHint", resourceCulture); } } ////// Looks up a localized string similar to Specifies the name of the validation group to which validation controls in the DynamicControl belong.. /// internal static string DynamicControlBase_ValidationGroup { get { return ResourceManager.GetString("DynamicControlBase_ValidationGroup", resourceCulture); } } ////// Looks up a localized string similar to The ID of the DynamicFilter control that exists in the ItemTemplate.. /// internal static string DynamicFilterRepeater_DynamicFilterContainerId { get { return ResourceManager.GetString("DynamicFilterRepeater_DynamicFilterContainerId", resourceCulture); } } ////// Looks up a localized string similar to No accessible tables found. Make sure scaffolds are enabled or custom templates exist for your model.. /// internal static string DynamicNavigatorDataSource_NoAccessibleTablesFound { get { return ResourceManager.GetString("DynamicNavigatorDataSource_NoAccessibleTablesFound", resourceCulture); } } ////// Looks up a localized string similar to No data models have been registered.. /// internal static string DynamicNavigatorDataSource_NoModelsRegistered { get { return ResourceManager.GetString("DynamicNavigatorDataSource_NoModelsRegistered", resourceCulture); } } ////// Looks up a localized string similar to There are no tables defined in the registered data models.. /// internal static string DynamicNavigatorDataSource_NoTablesInModels { get { return ResourceManager.GetString("DynamicNavigatorDataSource_NoTablesInModels", resourceCulture); } } ////// Looks up a localized string similar to Ambiguous invocation of '{0}' constructor. /// internal static string ExpressionParser_AmbiguousConstructorInvocation { get { return ResourceManager.GetString("ExpressionParser_AmbiguousConstructorInvocation", resourceCulture); } } ////// Looks up a localized string similar to Ambiguous invocation of indexer in type '{0}'. /// internal static string ExpressionParser_AmbiguousIndexerInvocation { get { return ResourceManager.GetString("ExpressionParser_AmbiguousIndexerInvocation", resourceCulture); } } ////// Looks up a localized string similar to Ambiguous invocation of method '{0}' in type '{1}'. /// internal static string ExpressionParser_AmbiguousMethodInvocation { get { return ResourceManager.GetString("ExpressionParser_AmbiguousMethodInvocation", resourceCulture); } } ////// Looks up a localized string similar to Argument list incompatible with lambda expression. /// internal static string ExpressionParser_ArgsIncompatibleWithLambda { get { return ResourceManager.GetString("ExpressionParser_ArgsIncompatibleWithLambda", resourceCulture); } } ////// Looks up a localized string similar to Both of the types '{0}' and '{1}' convert to the other. /// internal static string ExpressionParser_BothTypesConvertToOther { get { return ResourceManager.GetString("ExpressionParser_BothTypesConvertToOther", resourceCulture); } } ////// Looks up a localized string similar to A value of type '{0}' cannot be converted to type '{1}'. /// internal static string ExpressionParser_CannotConvertValue { get { return ResourceManager.GetString("ExpressionParser_CannotConvertValue", resourceCulture); } } ////// Looks up a localized string similar to Indexing of multiple-dimensional arrays is not supported. /// internal static string ExpressionParser_CannotIndexMultipleDimensionalArray { get { return ResourceManager.GetString("ExpressionParser_CannotIndexMultipleDimensionalArray", resourceCulture); } } ////// Looks up a localized string similar to ']' or ',' expected. /// internal static string ExpressionParser_CloseBracketOrCommaExpected { get { return ResourceManager.GetString("ExpressionParser_CloseBracketOrCommaExpected", resourceCulture); } } ////// Looks up a localized string similar to ')' or ',' expected. /// internal static string ExpressionParser_CloseParenOrCommaExpected { get { return ResourceManager.GetString("ExpressionParser_CloseParenOrCommaExpected", resourceCulture); } } ////// Looks up a localized string similar to ')' or operator expected. /// internal static string ExpressionParser_CloseParenOrOperatorExpected { get { return ResourceManager.GetString("ExpressionParser_CloseParenOrOperatorExpected", resourceCulture); } } ////// Looks up a localized string similar to ':' expected. /// internal static string ExpressionParser_ColonExpected { get { return ResourceManager.GetString("ExpressionParser_ColonExpected", resourceCulture); } } ////// Looks up a localized string similar to Digit expected. /// internal static string ExpressionParser_DigitExpected { get { return ResourceManager.GetString("ExpressionParser_DigitExpected", resourceCulture); } } ////// Looks up a localized string similar to '.' or '(' expected. /// internal static string ExpressionParser_DotOrOpenParenExpected { get { return ResourceManager.GetString("ExpressionParser_DotOrOpenParenExpected", resourceCulture); } } ////// Looks up a localized string similar to The identifier '{0}' was defined more than once. /// internal static string ExpressionParser_DuplicateIdentifier { get { return ResourceManager.GetString("ExpressionParser_DuplicateIdentifier", resourceCulture); } } ////// Looks up a localized string similar to Expression expected. /// internal static string ExpressionParser_ExpressionExpected { get { return ResourceManager.GetString("ExpressionParser_ExpressionExpected", resourceCulture); } } ////// Looks up a localized string similar to Expression of type '{0}' expected. /// internal static string ExpressionParser_ExpressionTypeMismatch { get { return ResourceManager.GetString("ExpressionParser_ExpressionTypeMismatch", resourceCulture); } } ////// Looks up a localized string similar to The first expression must be of type 'Boolean'. /// internal static string ExpressionParser_FirstExprMustBeBool { get { return ResourceManager.GetString("ExpressionParser_FirstExprMustBeBool", resourceCulture); } } ////// Looks up a localized string similar to Identifier expected. /// internal static string ExpressionParser_IdentifierExpected { get { return ResourceManager.GetString("ExpressionParser_IdentifierExpected", resourceCulture); } } ////// Looks up a localized string similar to The 'iif' function requires three arguments. /// internal static string ExpressionParser_IifRequiresThreeArgs { get { return ResourceManager.GetString("ExpressionParser_IifRequiresThreeArgs", resourceCulture); } } ////// Looks up a localized string similar to Operator '{0}' incompatible with operand type '{1}'. /// internal static string ExpressionParser_IncompatibleOperand { get { return ResourceManager.GetString("ExpressionParser_IncompatibleOperand", resourceCulture); } } ////// Looks up a localized string similar to Operator '{0}' incompatible with operand types '{1}' and '{2}'. /// internal static string ExpressionParser_IncompatibleOperands { get { return ResourceManager.GetString("ExpressionParser_IncompatibleOperands", resourceCulture); } } ////// Looks up a localized string similar to Syntax error '{0}'. /// internal static string ExpressionParser_InvalidCharacter { get { return ResourceManager.GetString("ExpressionParser_InvalidCharacter", resourceCulture); } } ////// Looks up a localized string similar to Character literal must contain exactly one character. /// internal static string ExpressionParser_InvalidCharacterLiteral { get { return ResourceManager.GetString("ExpressionParser_InvalidCharacterLiteral", resourceCulture); } } ////// Looks up a localized string similar to Array index must be an integer expression. /// internal static string ExpressionParser_InvalidIndex { get { return ResourceManager.GetString("ExpressionParser_InvalidIndex", resourceCulture); } } ////// Looks up a localized string similar to Invalid integer literal '{0}'. /// internal static string ExpressionParser_InvalidIntegerLiteral { get { return ResourceManager.GetString("ExpressionParser_InvalidIntegerLiteral", resourceCulture); } } ////// Looks up a localized string similar to Invalid real literal '{0}'. /// internal static string ExpressionParser_InvalidRealLiteral { get { return ResourceManager.GetString("ExpressionParser_InvalidRealLiteral", resourceCulture); } } ////// Looks up a localized string similar to Method '{0}' in type '{1}' does not return a value. /// internal static string ExpressionParser_MethodIsVoid { get { return ResourceManager.GetString("ExpressionParser_MethodIsVoid", resourceCulture); } } ////// Looks up a localized string similar to Methods on type '{0}' are not accessible. /// internal static string ExpressionParser_MethodsAreInaccessible { get { return ResourceManager.GetString("ExpressionParser_MethodsAreInaccessible", resourceCulture); } } ////// Looks up a localized string similar to Expression is missing an 'as' clause. /// internal static string ExpressionParser_MissingAsClause { get { return ResourceManager.GetString("ExpressionParser_MissingAsClause", resourceCulture); } } ////// Looks up a localized string similar to Neither of the types '{0}' and '{1}' converts to the other. /// internal static string ExpressionParser_NeitherTypeConvertsToOther { get { return ResourceManager.GetString("ExpressionParser_NeitherTypeConvertsToOther", resourceCulture); } } ////// Looks up a localized string similar to No applicable aggregate method '{0}' exists. /// internal static string ExpressionParser_NoApplicableAggregate { get { return ResourceManager.GetString("ExpressionParser_NoApplicableAggregate", resourceCulture); } } ////// Looks up a localized string similar to No applicable indexer exists in type '{0}'. /// internal static string ExpressionParser_NoApplicableIndexer { get { return ResourceManager.GetString("ExpressionParser_NoApplicableIndexer", resourceCulture); } } ////// Looks up a localized string similar to No applicable method '{0}' exists in type '{1}'. /// internal static string ExpressionParser_NoApplicableMethod { get { return ResourceManager.GetString("ExpressionParser_NoApplicableMethod", resourceCulture); } } ////// Looks up a localized string similar to No 'it' is in scope. /// internal static string ExpressionParser_NoItInScope { get { return ResourceManager.GetString("ExpressionParser_NoItInScope", resourceCulture); } } ////// Looks up a localized string similar to No matching constructor in type '{0}'. /// internal static string ExpressionParser_NoMatchingConstructor { get { return ResourceManager.GetString("ExpressionParser_NoMatchingConstructor", resourceCulture); } } ////// Looks up a localized string similar to '[' expected. /// internal static string ExpressionParser_OpenBracketExpected { get { return ResourceManager.GetString("ExpressionParser_OpenBracketExpected", resourceCulture); } } ////// Looks up a localized string similar to '(' expected. /// internal static string ExpressionParser_OpenParenExpected { get { return ResourceManager.GetString("ExpressionParser_OpenParenExpected", resourceCulture); } } ////// Looks up a localized string similar to Syntax error. /// internal static string ExpressionParser_SyntaxError { get { return ResourceManager.GetString("ExpressionParser_SyntaxError", resourceCulture); } } ////// Looks up a localized string similar to {0} expected. /// internal static string ExpressionParser_TokenExpected { get { return ResourceManager.GetString("ExpressionParser_TokenExpected", resourceCulture); } } ////// Looks up a localized string similar to Type '{0}' has no nullable form. /// internal static string ExpressionParser_TypeHasNoNullableForm { get { return ResourceManager.GetString("ExpressionParser_TypeHasNoNullableForm", resourceCulture); } } ////// Looks up a localized string similar to Unknown identifier '{0}'. /// internal static string ExpressionParser_UnknownIdentifier { get { return ResourceManager.GetString("ExpressionParser_UnknownIdentifier", resourceCulture); } } ////// Looks up a localized string similar to No property or field '{0}' exists in type '{1}'. /// internal static string ExpressionParser_UnknownPropertyOrField { get { return ResourceManager.GetString("ExpressionParser_UnknownPropertyOrField", resourceCulture); } } ////// Looks up a localized string similar to Unterminated string literal. /// internal static string ExpressionParser_UnterminatedStringLiteral { get { return ResourceManager.GetString("ExpressionParser_UnterminatedStringLiteral", resourceCulture); } } ////// Looks up a localized string similar to An extender can't be in a different UpdatePanel than the control it extends.. /// internal static string ExtenderControl_TargetControlDifferentUpdatePanel { get { return ResourceManager.GetString("ExtenderControl_TargetControlDifferentUpdatePanel", resourceCulture); } } ////// Looks up a localized string similar to Identifies the control to extend.. /// internal static string ExtenderControl_TargetControlID { get { return ResourceManager.GetString("ExtenderControl_TargetControlID", resourceCulture); } } ////// Looks up a localized string similar to The TargetControlID of '{0}' is not valid. The value cannot be null or empty.. /// internal static string ExtenderControl_TargetControlIDEmpty { get { return ResourceManager.GetString("ExtenderControl_TargetControlIDEmpty", resourceCulture); } } ////// Looks up a localized string similar to The TargetControlID of '{0}' is not valid. A control with ID '{1}' could not be found.. /// internal static string ExtenderControl_TargetControlIDInvalid { get { return ResourceManager.GetString("ExtenderControl_TargetControlIDInvalid", resourceCulture); } } ////// Looks up a localized string similar to Specifies an override for the table name used by the FilterRepeater. By default the table is inferred from the page URL.. /// internal static string FilterRepeater_TableName { get { return ResourceManager.GetString("FilterRepeater_TableName", resourceCulture); } } ////// Looks up a localized string similar to Type '{0}' is not supported for deserialization of an array.. /// internal static string JSON_ArrayTypeNotSupported { get { return ResourceManager.GetString("JSON_ArrayTypeNotSupported", resourceCulture); } } ////// Looks up a localized string similar to Unrecognized escape sequence.. /// internal static string JSON_BadEscape { get { return ResourceManager.GetString("JSON_BadEscape", resourceCulture); } } ////// Looks up a localized string similar to Cannot convert object of type '{0}' to type '{1}'. /// internal static string JSON_CannotConvertObjectToType { get { return ResourceManager.GetString("JSON_CannotConvertObjectToType", resourceCulture); } } ////// Looks up a localized string similar to Cannot create instance of {0}.. /// internal static string JSON_CannotCreateListType { get { return ResourceManager.GetString("JSON_CannotCreateListType", resourceCulture); } } ////// Looks up a localized string similar to A circular reference was detected while serializing an object of type '{0}'.. /// internal static string JSON_CircularReference { get { return ResourceManager.GetString("JSON_CircularReference", resourceCulture); } } ////// Looks up a localized string similar to RecursionLimit exceeded.. /// internal static string JSON_DepthLimitExceeded { get { return ResourceManager.GetString("JSON_DepthLimitExceeded", resourceCulture); } } ////// Looks up a localized string similar to Cannot deserialize object graph into type of '{0}'.. /// internal static string JSON_DeserializerTypeMismatch { get { return ResourceManager.GetString("JSON_DeserializerTypeMismatch", resourceCulture); } } ////// Looks up a localized string similar to Type '{0}' is not supported for serialization/deserialization of a dictionary, keys must be strings or objects.. /// internal static string JSON_DictionaryTypeNotSupported { get { return ResourceManager.GetString("JSON_DictionaryTypeNotSupported", resourceCulture); } } ////// Looks up a localized string similar to Invalid object passed in, '{' expected.. /// internal static string JSON_ExpectedOpenBrace { get { return ResourceManager.GetString("JSON_ExpectedOpenBrace", resourceCulture); } } ////// Looks up a localized string similar to Invalid JSON primitive: {0}.. /// internal static string JSON_IllegalPrimitive { get { return ResourceManager.GetString("JSON_IllegalPrimitive", resourceCulture); } } ////// Looks up a localized string similar to Invalid array passed in, ']' expected.. /// internal static string JSON_InvalidArrayEnd { get { return ResourceManager.GetString("JSON_InvalidArrayEnd", resourceCulture); } } ////// Looks up a localized string similar to Invalid array passed in, ',' expected.. /// internal static string JSON_InvalidArrayExpectComma { get { return ResourceManager.GetString("JSON_InvalidArrayExpectComma", resourceCulture); } } ////// Looks up a localized string similar to Invalid array passed in, extra trailing ','.. /// internal static string JSON_InvalidArrayExtraComma { get { return ResourceManager.GetString("JSON_InvalidArrayExtraComma", resourceCulture); } } ////// Looks up a localized string similar to Invalid array passed in, '[' expected.. /// internal static string JSON_InvalidArrayStart { get { return ResourceManager.GetString("JSON_InvalidArrayStart", resourceCulture); } } ////// Looks up a localized string similar to Enums based on System.Int64 or System.UInt64 are not JSON-serializable because JavaScript does not support the necessary precision.. /// internal static string JSON_InvalidEnumType { get { return ResourceManager.GetString("JSON_InvalidEnumType", resourceCulture); } } ////// Looks up a localized string similar to Value must be a positive integer.. /// internal static string JSON_InvalidMaxJsonLength { get { return ResourceManager.GetString("JSON_InvalidMaxJsonLength", resourceCulture); } } ////// Looks up a localized string similar to Invalid object passed in, member name expected.. /// internal static string JSON_InvalidMemberName { get { return ResourceManager.GetString("JSON_InvalidMemberName", resourceCulture); } } ////// Looks up a localized string similar to Invalid object passed in, ':' or '}' expected.. /// internal static string JSON_InvalidObject { get { return ResourceManager.GetString("JSON_InvalidObject", resourceCulture); } } ////// Looks up a localized string similar to RecursionLimit must be a positive integer.. /// internal static string JSON_InvalidRecursionLimit { get { return ResourceManager.GetString("JSON_InvalidRecursionLimit", resourceCulture); } } ////// Looks up a localized string similar to Error during serialization or deserialization using the JSON JavaScriptSerializer. The length of the string exceeds the value set on the maxJsonLength property.. /// internal static string JSON_MaxJsonLengthExceeded { get { return ResourceManager.GetString("JSON_MaxJsonLengthExceeded", resourceCulture); } } ////// Looks up a localized string similar to No parameterless constructor defined for type of '{0}'.. /// internal static string JSON_NoConstructor { get { return ResourceManager.GetString("JSON_NoConstructor", resourceCulture); } } ////// Looks up a localized string similar to Invalid string passed in, '\"' expected.. /// internal static string JSON_StringNotQuoted { get { return ResourceManager.GetString("JSON_StringNotQuoted", resourceCulture); } } ////// Looks up a localized string similar to Unterminated string passed in.. /// internal static string JSON_UnterminatedString { get { return ResourceManager.GetString("JSON_UnterminatedString", resourceCulture); } } ////// Looks up a localized string similar to Cannot convert null to a value type.. /// internal static string JSON_ValueTypeCannotBeNull { get { return ResourceManager.GetString("JSON_ValueTypeCannotBeNull", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether to automatically generate the OrderBy clause from the OrderByParameters.. /// internal static string LinqDataSource_AutoGenerateOrderByClause { get { return ResourceManager.GetString("LinqDataSource_AutoGenerateOrderByClause", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether to automatically generate the Where clause from the WhereParameters.. /// internal static string LinqDataSource_AutoGenerateWhereClause { get { return ResourceManager.GetString("LinqDataSource_AutoGenerateWhereClause", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether data is automatically paged.. /// internal static string LinqDataSource_AutoPage { get { return ResourceManager.GetString("LinqDataSource_AutoPage", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether data is automatically sorted.. /// internal static string LinqDataSource_AutoSort { get { return ResourceManager.GetString("LinqDataSource_AutoSort", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the context is created unless a query result is specified during the Selecting event.. /// internal static string LinqDataSource_ContextCreated { get { return ResourceManager.GetString("LinqDataSource_ContextCreated", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the context is created unless a query result is specified during the Selecting event.. /// internal static string LinqDataSource_ContextCreating { get { return ResourceManager.GetString("LinqDataSource_ContextCreating", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the context is disposed.. /// internal static string LinqDataSource_ContextDisposing { get { return ResourceManager.GetString("LinqDataSource_ContextDisposing", resourceCulture); } } ////// Looks up a localized string similar to The data context type that contains the table property.. /// internal static string LinqDataSource_ContextTypeName { get { return ResourceManager.GetString("LinqDataSource_ContextTypeName", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the Delete operation is completed.. /// internal static string LinqDataSource_Deleted { get { return ResourceManager.GetString("LinqDataSource_Deleted", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used during the Delete operation. These parameters are merged with the parameters provided by data-bound controls.. /// internal static string LinqDataSource_DeleteParameters { get { return ResourceManager.GetString("LinqDataSource_DeleteParameters", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the Delete operation is executed.. /// internal static string LinqDataSource_Deleting { get { return ResourceManager.GetString("LinqDataSource_Deleting", resourceCulture); } } ////// Looks up a localized string similar to Use LINQ to connect to a DataContext or object in the Bin or App_Code directory for the application.. /// internal static string LinqDataSource_Description { get { return ResourceManager.GetString("LinqDataSource_Description", resourceCulture); } } ////// Looks up a localized string similar to LINQ. /// internal static string LinqDataSource_DisplayName { get { return ResourceManager.GetString("LinqDataSource_DisplayName", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the Delete operation is enabled.. /// internal static string LinqDataSource_EnableDelete { get { return ResourceManager.GetString("LinqDataSource_EnableDelete", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the Insert operation is enabled.. /// internal static string LinqDataSource_EnableInsert { get { return ResourceManager.GetString("LinqDataSource_EnableInsert", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether ObjectTracking should be disabled on read-only Linq to SQL data contexts.. /// internal static string LinqDataSource_EnableObjectTracking { get { return ResourceManager.GetString("LinqDataSource_EnableObjectTracking", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether the Update operation is enabled.. /// internal static string LinqDataSource_EnableUpdate { get { return ResourceManager.GetString("LinqDataSource_EnableUpdate", resourceCulture); } } ////// Looks up a localized string similar to The expression passed to the GroupBy operator during the Select query.. /// internal static string LinqDataSource_GroupBy { get { return ResourceManager.GetString("LinqDataSource_GroupBy", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used for the GroupBy operator during the Select query.. /// internal static string LinqDataSource_GroupByParameters { get { return ResourceManager.GetString("LinqDataSource_GroupByParameters", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the Insert operation is completed.. /// internal static string LinqDataSource_Inserted { get { return ResourceManager.GetString("LinqDataSource_Inserted", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the Insert operation is executed.. /// internal static string LinqDataSource_Inserting { get { return ResourceManager.GetString("LinqDataSource_Inserting", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used during the Insert operation. These parameters are merged with the parameters provided by data-bound controls.. /// internal static string LinqDataSource_InsertParameters { get { return ResourceManager.GetString("LinqDataSource_InsertParameters", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' only supports a single view named '{1}'. You may also leave the view name empty for the default view to be chosen.. /// internal static string LinqDataSource_InvalidViewName { get { return ResourceManager.GetString("LinqDataSource_InvalidViewName", resourceCulture); } } ////// Looks up a localized string similar to The expression passed to the OrderBy operator during the Select query.. /// internal static string LinqDataSource_OrderBy { get { return ResourceManager.GetString("LinqDataSource_OrderBy", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used for the OrderBy operator during the Select query.. /// internal static string LinqDataSource_OrderByParameters { get { return ResourceManager.GetString("LinqDataSource_OrderByParameters", resourceCulture); } } ////// Looks up a localized string similar to The expression passed to the OrderBy operator used for ordering groups after a GroupBy has been performed during the Select query.. /// internal static string LinqDataSource_OrderGroupsBy { get { return ResourceManager.GetString("LinqDataSource_OrderGroupsBy", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used for the OrderGroupsBy operator during the Select query.. /// internal static string LinqDataSource_OrderGroupsByParameters { get { return ResourceManager.GetString("LinqDataSource_OrderGroupsByParameters", resourceCulture); } } ////// Looks up a localized string similar to The expression defining a projection used during the Select query.. /// internal static string LinqDataSource_Select { get { return ResourceManager.GetString("LinqDataSource_Select", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the Select operation is completed.. /// internal static string LinqDataSource_Selected { get { return ResourceManager.GetString("LinqDataSource_Selected", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the Select operation is executed.. /// internal static string LinqDataSource_Selecting { get { return ResourceManager.GetString("LinqDataSource_Selecting", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used in the projection during the Select query.. /// internal static string LinqDataSource_SelectParameters { get { return ResourceManager.GetString("LinqDataSource_SelectParameters", resourceCulture); } } ////// Looks up a localized string similar to Specifies whether to store original data values in ViewState. This property is used for conflict detection during Update and Delete operations.. /// internal static string LinqDataSource_StoreOriginalValuesInViewState { get { return ResourceManager.GetString("LinqDataSource_StoreOriginalValuesInViewState", resourceCulture); } } ////// Looks up a localized string similar to The name of the table property on the data context.. /// internal static string LinqDataSource_TableName { get { return ResourceManager.GetString("LinqDataSource_TableName", resourceCulture); } } ////// Looks up a localized string similar to Event raised after the Update operation is completed.. /// internal static string LinqDataSource_Updated { get { return ResourceManager.GetString("LinqDataSource_Updated", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used during the Update operation. These parameters are merged with the parameters provided by data-bound controls.. /// internal static string LinqDataSource_UpdateParameters { get { return ResourceManager.GetString("LinqDataSource_UpdateParameters", resourceCulture); } } ////// Looks up a localized string similar to Event raised before the Update operation is executed.. /// internal static string LinqDataSource_Updating { get { return ResourceManager.GetString("LinqDataSource_Updating", resourceCulture); } } ////// Looks up a localized string similar to The expression passed to the Where operator during the Select query.. /// internal static string LinqDataSource_Where { get { return ResourceManager.GetString("LinqDataSource_Where", resourceCulture); } } ////// Looks up a localized string similar to Collection of parameters used for the Where operator during the Select query.. /// internal static string LinqDataSource_WhereParameters { get { return ResourceManager.GetString("LinqDataSource_WhereParameters", resourceCulture); } } ////// Looks up a localized string similar to Failed to set one or more properties on the data object. Ensure that the input values are valid and can be converted to the corresponding property types.. /// internal static string LinqDataSourceValidationException_ValidationFailed { get { return ResourceManager.GetString("LinqDataSourceValidationException_ValidationFailed", resourceCulture); } } ////// Looks up a localized string similar to Cannot convert value of parameter '{0}' from '{1}' to '{2}'.. /// internal static string LinqDataSourceView_CannotConvertType { get { return ResourceManager.GetString("LinqDataSourceView_CannotConvertType", resourceCulture); } } ////// Looks up a localized string similar to The ContextTypeName property of LinqDataSource '{0}' cannot be changed after the data context has been created.. /// internal static string LinqDataSourceView_ContextTypeNameChanged { get { return ResourceManager.GetString("LinqDataSourceView_ContextTypeNameChanged", resourceCulture); } } ////// Looks up a localized string similar to Could not find the type specified in the ContextTypeName property of LinqDataSource '{0}'.. /// internal static string LinqDataSourceView_ContextTypeNameNotFound { get { return ResourceManager.GetString("LinqDataSourceView_ContextTypeNameNotFound", resourceCulture); } } ////// Looks up a localized string similar to The ContextTypeName property of LinqDataSource '{0}' must specify a data context type.. /// internal static string LinqDataSourceView_ContextTypeNameNotSpecified { get { return ResourceManager.GetString("LinqDataSourceView_ContextTypeNameNotSpecified", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Delete operation unless EnableDelete is true.. /// internal static string LinqDataSourceView_DeleteNotSupported { get { return ResourceManager.GetString("LinqDataSourceView_DeleteNotSupported", resourceCulture); } } ////// Looks up a localized string similar to The EnableObjectTracking property of LinqDataSource '{0}' cannot be changed after the data context has been created.. /// internal static string LinqDataSourceView_EnableObjectTrackingChanged { get { return ResourceManager.GetString("LinqDataSourceView_EnableObjectTrackingChanged", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the GroupBy property when the Delete, Insert or Update operations are enabled.. /// internal static string LinqDataSourceView_GroupByNotSupportedOnEdit { get { return ResourceManager.GetString("LinqDataSourceView_GroupByNotSupportedOnEdit", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Insert operation unless EnableInsert is true.. /// internal static string LinqDataSourceView_InsertNotSupported { get { return ResourceManager.GetString("LinqDataSourceView_InsertNotSupported", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' has no values to insert. Check that the 'values' dictionary contains values.. /// internal static string LinqDataSourceView_InsertRequiresValues { get { return ResourceManager.GetString("LinqDataSourceView_InsertRequiresValues", resourceCulture); } } ////// Looks up a localized string similar to The data context used by LinqDataSource '{0}' must extend DataContext when the Delete, Insert or Update operations are enabled.. /// internal static string LinqDataSourceView_InvalidContextType { get { return ResourceManager.GetString("LinqDataSourceView_InvalidContextType", resourceCulture); } } ////// Looks up a localized string similar to The value '{0}' for parameter '{1}' is not a valid OrderBy field name.. /// internal static string LinqDataSourceView_InvalidOrderByFieldName { get { return ResourceManager.GetString("LinqDataSourceView_InvalidOrderByFieldName", resourceCulture); } } ////// Looks up a localized string similar to The name for parameter '{0}' on LinqDataSource '{1}' is not a valid identifier name.. /// internal static string LinqDataSourceView_InvalidParameterName { get { return ResourceManager.GetString("LinqDataSourceView_InvalidParameterName", resourceCulture); } } ////// Looks up a localized string similar to The table property used by LinqDataSource '{0}' must extend Table<T> when the Delete, Insert or Update operations are enabled.. /// internal static string LinqDataSourceView_InvalidTablePropertyType { get { return ResourceManager.GetString("LinqDataSourceView_InvalidTablePropertyType", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the OrderBy property when AutoGenerateOrderByClause is true.. /// internal static string LinqDataSourceView_OrderByAlreadySpecified { get { return ResourceManager.GetString("LinqDataSourceView_OrderByAlreadySpecified", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the OrderGroupsBy property when the GroupsBy property has not been set.. /// internal static string LinqDataSourceView_OrderGroupsByRequiresGroupBy { get { return ResourceManager.GetString("LinqDataSourceView_OrderGroupsByRequiresGroupBy", resourceCulture); } } ////// Looks up a localized string similar to Could not find a row that matches the given keys in the original values stored in ViewState. Ensure that the 'keys' dictionary contains unique key values that correspond to a row returned from the previous Select operation.. /// internal static string LinqDataSourceView_OriginalValuesNotFound { get { return ResourceManager.GetString("LinqDataSourceView_OriginalValuesNotFound", resourceCulture); } } ////// Looks up a localized string similar to AutoPage is disabled on LinqDataSource {0} but paging has not been handled. Ensure you have set the LinqDataSourceSelectArguments.Arguments.TotalRowCount property to the total number of rows.. /// internal static string LinqDataSourceView_PagingNotHandled { get { return ResourceManager.GetString("LinqDataSourceView_PagingNotHandled", resourceCulture); } } ////// Looks up a localized string similar to Parameters for LinqDataSource '{0}' that are not used for AutoGenerateOrderBy must be named.. /// internal static string LinqDataSourceView_ParametersMustBeNamed { get { return ResourceManager.GetString("LinqDataSourceView_ParametersMustBeNamed", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Select property when the Delete, Insert or Update operations are enabled.. /// internal static string LinqDataSourceView_SelectNewNotSupportedOnEdit { get { return ResourceManager.GetString("LinqDataSourceView_SelectNewNotSupportedOnEdit", resourceCulture); } } ////// Looks up a localized string similar to Member '{0}' on the data context type '{1}' of LinqDataSource '{2}' is not a valid table. For Insert, Update and Delete the table must not be a static member.. /// internal static string LinqDataSourceView_TableCannotBeStatic { get { return ResourceManager.GetString("LinqDataSourceView_TableCannotBeStatic", resourceCulture); } } ////// Looks up a localized string similar to The TableName property of LinqDataSource '{0}' cannot be changed after the data context has been created.. /// internal static string LinqDataSourceView_TableNameChanged { get { return ResourceManager.GetString("LinqDataSourceView_TableNameChanged", resourceCulture); } } ////// Looks up a localized string similar to Could not find a property or field called '{0}' on the data context type '{1}' of LinqDataSource '{2}'.. /// internal static string LinqDataSourceView_TableNameNotFound { get { return ResourceManager.GetString("LinqDataSourceView_TableNameNotFound", resourceCulture); } } ////// Looks up a localized string similar to The TableName property of LinqDataSource '{0}' must specify a table property or field on the data context type.. /// internal static string LinqDataSourceView_TableNameNotSpecified { get { return ResourceManager.GetString("LinqDataSourceView_TableNameNotSpecified", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Update operation unless EnableUpdate is true.. /// internal static string LinqDataSourceView_UpdateNotSupported { get { return ResourceManager.GetString("LinqDataSourceView_UpdateNotSupported", resourceCulture); } } ////// Looks up a localized string similar to Failed to set one or more properties on type {0}. {1}. /// internal static string LinqDataSourceView_ValidationFailed { get { return ResourceManager.GetString("LinqDataSourceView_ValidationFailed", resourceCulture); } } ////// Looks up a localized string similar to LinqDataSource '{0}' does not support the Where property when AutoGenerateWhereClause is true.. /// internal static string LinqDataSourceView_WhereAlreadySpecified { get { return ResourceManager.GetString("LinqDataSourceView_WhereAlreadySpecified", resourceCulture); } } ////// Looks up a localized string similar to The template used for alternating items.. /// internal static string ListView_AlternatingItemTemplate { get { return ResourceManager.GetString("ListView_AlternatingItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to The value of {0} must not be empty.. /// internal static string ListView_ContainerNameMustNotBeEmpty { get { return ResourceManager.GetString("ListView_ContainerNameMustNotBeEmpty", resourceCulture); } } ////// Looks up a localized string similar to Whether the ListView treats empty strings as null when the value is extracted from the item.. /// internal static string ListView_ConvertEmptyStringToNull { get { return ResourceManager.GetString("ListView_ConvertEmptyStringToNull", resourceCulture); } } ////// Looks up a localized string similar to A comma-separated list of key fields in the data source.. /// internal static string ListView_DataKeyNames { get { return ResourceManager.GetString("ListView_DataKeyNames", resourceCulture); } } ////// Looks up a localized string similar to Data keys must be specified on ListView '{0}' before the selected data keys can be retrieved. Use the DataKeyNames property to specify data keys.. /// internal static string ListView_DataKeyNamesMustBeSpecified { get { return ResourceManager.GetString("ListView_DataKeyNamesMustBeSpecified", resourceCulture); } } ////// Looks up a localized string similar to The collection of data key field values.. /// internal static string ListView_DataKeys { get { return ResourceManager.GetString("ListView_DataKeys", resourceCulture); } } ////// Looks up a localized string similar to The data source '{0}' does not support server-side paging and it returned non-ICollection. /// internal static string ListView_DataSourceDoesntSupportPaging { get { return ResourceManager.GetString("ListView_DataSourceDoesntSupportPaging", resourceCulture); } } ////// Looks up a localized string similar to Data source must implement ICollection when calling CreateChildControls with dataBinding=false.. /// internal static string ListView_DataSourceMustBeCollectionWhenNotDataBinding { get { return ResourceManager.GetString("ListView_DataSourceMustBeCollectionWhenNotDataBinding", resourceCulture); } } ////// Looks up a localized string similar to The index of the item shown in edit mode.. /// internal static string ListView_EditIndex { get { return ResourceManager.GetString("ListView_EditIndex", resourceCulture); } } ////// Looks up a localized string similar to The ListViewItem that is currently being edited.. /// internal static string ListView_EditItem { get { return ResourceManager.GetString("ListView_EditItem", resourceCulture); } } ////// Looks up a localized string similar to The template used for items in edit mode.. /// internal static string ListView_EditItemTemplate { get { return ResourceManager.GetString("ListView_EditItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to The template used when no data is returned from the data source. This template replaces the LayoutTemplate when used.. /// internal static string ListView_EmptyDataTemplate { get { return ResourceManager.GetString("ListView_EmptyDataTemplate", resourceCulture); } } ////// Looks up a localized string similar to The template used in the GroupTemplate when the number of remaining data items is less than the GroupItemCount.. /// internal static string ListView_EmptyItemTemplate { get { return ResourceManager.GetString("ListView_EmptyItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to Whether the data bound control will register itself with a data bound control manager on the page.. /// internal static string ListView_EnableDataBoundControlManager { get { return ResourceManager.GetString("ListView_EnableDataBoundControlManager", resourceCulture); } } ////// Looks up a localized string similar to Whether page validation will be performed after validation is done in the model.. /// internal static string ListView_EnableModelValidation { get { return ResourceManager.GetString("ListView_EnableModelValidation", resourceCulture); } } ////// Looks up a localized string similar to The ID of the server control that will be replaced with instances of the GroupTemplate.. /// internal static string ListView_GroupContainerID { get { return ResourceManager.GetString("ListView_GroupContainerID", resourceCulture); } } ////// Looks up a localized string similar to The number of items that are rendered inside the GroupTemplate.. /// internal static string ListView_GroupItemCount { get { return ResourceManager.GetString("ListView_GroupItemCount", resourceCulture); } } ////// Looks up a localized string similar to ListView '{0}' has a GroupItemCount specified on it but no GroupTemplate. A GroupTemplate must be present for ListView to render groups.. /// internal static string ListView_GroupItemCountNoGroupTemplate { get { return ResourceManager.GetString("ListView_GroupItemCountNoGroupTemplate", resourceCulture); } } ////// Looks up a localized string similar to The template used for group separators between GroupTemplates.. /// internal static string ListView_GroupSeparatorTemplate { get { return ResourceManager.GetString("ListView_GroupSeparatorTemplate", resourceCulture); } } ////// Looks up a localized string similar to The template used for item groups.. /// internal static string ListView_GroupTemplate { get { return ResourceManager.GetString("ListView_GroupTemplate", resourceCulture); } } ////// Looks up a localized string similar to The ListViewItem that is currently being inserted.. /// internal static string ListView_InsertItem { get { return ResourceManager.GetString("ListView_InsertItem", resourceCulture); } } ////// Looks up a localized string similar to The position of the insert item within the ListView.. /// internal static string ListView_InsertItemPosition { get { return ResourceManager.GetString("ListView_InsertItemPosition", resourceCulture); } } ////// Looks up a localized string similar to The template used for items in insert mode.. /// internal static string ListView_InsertItemTemplate { get { return ResourceManager.GetString("ListView_InsertItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to An InsertItemTemplate must be defined on ListView '{0}' if InsertItemPosition is set to FirstItem or LastItem.. /// internal static string ListView_InsertTemplateRequired { get { return ResourceManager.GetString("ListView_InsertTemplateRequired", resourceCulture); } } ////// Looks up a localized string similar to Cancel can only be called from the currently-edited record or an insert item.. /// internal static string ListView_InvalidCancel { get { return ResourceManager.GetString("ListView_InvalidCancel", resourceCulture); } } ////// Looks up a localized string similar to Delete can only be called on a valid data item.. /// internal static string ListView_InvalidDelete { get { return ResourceManager.GetString("ListView_InvalidDelete", resourceCulture); } } ////// Looks up a localized string similar to Edit can only be called on a valid data item.. /// internal static string ListView_InvalidEdit { get { return ResourceManager.GetString("ListView_InvalidEdit", resourceCulture); } } ////// Looks up a localized string similar to Insert can only be called on an insert item. Ensure only the InsertTemplate has a button with CommandName=Insert.. /// internal static string ListView_InvalidInsert { get { return ResourceManager.GetString("ListView_InvalidInsert", resourceCulture); } } ////// Looks up a localized string similar to Select can only be called on a valid data item.. /// internal static string ListView_InvalidSelect { get { return ResourceManager.GetString("ListView_InvalidSelect", resourceCulture); } } ////// Looks up a localized string similar to Update can only be called on a valid data item.. /// internal static string ListView_InvalidUpdate { get { return ResourceManager.GetString("ListView_InvalidUpdate", resourceCulture); } } ////// Looks up a localized string similar to The ID of the server control that will be replaced with instances of the ItemTemplate.. /// internal static string ListView_ItemPlaceholderID { get { return ResourceManager.GetString("ListView_ItemPlaceholderID", resourceCulture); } } ////// Looks up a localized string similar to The collection of visible items.. /// internal static string ListView_Items { get { return ResourceManager.GetString("ListView_Items", resourceCulture); } } ////// Looks up a localized string similar to The template used for separator items.. /// internal static string ListView_ItemSeparatorTemplate { get { return ResourceManager.GetString("ListView_ItemSeparatorTemplate", resourceCulture); } } ////// Looks up a localized string similar to ListViewItems that have type DataItem must be of type ListViewDataItem.. /// internal static string ListView_ItemsNotDataItems { get { return ResourceManager.GetString("ListView_ItemsNotDataItems", resourceCulture); } } ////// Looks up a localized string similar to The template used for items.. /// internal static string ListView_ItemTemplate { get { return ResourceManager.GetString("ListView_ItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to An ItemTemplate must be defined on ListView '{0}'.. /// internal static string ListView_ItemTemplateRequired { get { return ResourceManager.GetString("ListView_ItemTemplateRequired", resourceCulture); } } ////// Looks up a localized string similar to The template used for the ListView layout.. /// internal static string ListView_LayoutTemplate { get { return ResourceManager.GetString("ListView_LayoutTemplate", resourceCulture); } } ////// Looks up a localized string similar to ListView with id '{0}' must have a data source that either implements ICollection or can perform data source paging if AllowPaging is true.. /// internal static string ListView_Missing_VirtualItemCount { get { return ResourceManager.GetString("ListView_Missing_VirtualItemCount", resourceCulture); } } ////// Looks up a localized string similar to If a data source does not return ICollection and cannot return the total row count, it cannot be used by the {0} to implement server-side paging.. /// internal static string ListView_NeedICollectionOrTotalRowCount { get { return ResourceManager.GetString("ListView_NeedICollectionOrTotalRowCount", resourceCulture); } } ////// Looks up a localized string similar to A group placeholder must be specified on ListView '{0}' when the GroupTemplate is defined. Specify a group placeholder by setting its ID property to "{1}". The group placeholder control must also specify runat="server".. /// internal static string ListView_NoGroupPlaceholder { get { return ResourceManager.GetString("ListView_NoGroupPlaceholder", resourceCulture); } } ////// Looks up a localized string similar to An insert item wasn't found.. /// internal static string ListView_NoInsertItem { get { return ResourceManager.GetString("ListView_NoInsertItem", resourceCulture); } } ////// Looks up a localized string similar to An item placeholder must be specified on ListView '{0}'. Specify an item placeholder by setting a control's ID property to "{1}". The item placeholder control must also specify runat="server".. /// internal static string ListView_NoItemPlaceholder { get { return ResourceManager.GetString("ListView_NoItemPlaceholder", resourceCulture); } } ////// Looks up a localized string similar to The data source retrieved by '{0}' returned a null DataSourceView.. /// internal static string ListView_NullView { get { return ResourceManager.GetString("ListView_NullView", resourceCulture); } } ////// Looks up a localized string similar to Fires when a Cancel event is generated within the ListView.. /// internal static string ListView_OnItemCanceling { get { return ResourceManager.GetString("ListView_OnItemCanceling", resourceCulture); } } ////// Looks up a localized string similar to Fires when an event is generated within the ListView.. /// internal static string ListView_OnItemCommand { get { return ResourceManager.GetString("ListView_OnItemCommand", resourceCulture); } } ////// Looks up a localized string similar to Fires when an item is created.. /// internal static string ListView_OnItemCreated { get { return ResourceManager.GetString("ListView_OnItemCreated", resourceCulture); } } ////// Looks up a localized string similar to Fires after an item has been data-bound.. /// internal static string ListView_OnItemDataBound { get { return ResourceManager.GetString("ListView_OnItemDataBound", resourceCulture); } } ////// Looks up a localized string similar to Fires after a Delete Command is executed on the data source.. /// internal static string ListView_OnItemDeleted { get { return ResourceManager.GetString("ListView_OnItemDeleted", resourceCulture); } } ////// Looks up a localized string similar to Fires before a Delete Command is executed on the data source.. /// internal static string ListView_OnItemDeleting { get { return ResourceManager.GetString("ListView_OnItemDeleting", resourceCulture); } } ////// Looks up a localized string similar to Fires when an Edit event is generated within the ListView.. /// internal static string ListView_OnItemEditing { get { return ResourceManager.GetString("ListView_OnItemEditing", resourceCulture); } } ////// Looks up a localized string similar to Fires after an Insert Command is executed on the data source.. /// internal static string ListView_OnItemInserted { get { return ResourceManager.GetString("ListView_OnItemInserted", resourceCulture); } } ////// Looks up a localized string similar to Fires before an Insert Command is executed on the data source.. /// internal static string ListView_OnItemInserting { get { return ResourceManager.GetString("ListView_OnItemInserting", resourceCulture); } } ////// Looks up a localized string similar to Fires after an Update Command is executed on the data source.. /// internal static string ListView_OnItemUpdated { get { return ResourceManager.GetString("ListView_OnItemUpdated", resourceCulture); } } ////// Looks up a localized string similar to Fires before an Update Command is executed on the data source.. /// internal static string ListView_OnItemUpdating { get { return ResourceManager.GetString("ListView_OnItemUpdating", resourceCulture); } } ////// Looks up a localized string similar to Fires when the ListView's layout is created.. /// internal static string ListView_OnLayoutCreated { get { return ResourceManager.GetString("ListView_OnLayoutCreated", resourceCulture); } } ////// Looks up a localized string similar to Fires when the ListView's paging properties have changed.. /// internal static string ListView_OnPagePropertiesChanged { get { return ResourceManager.GetString("ListView_OnPagePropertiesChanged", resourceCulture); } } ////// Looks up a localized string similar to Fires when the ListView's paging properties are changing.. /// internal static string ListView_OnPagePropertiesChanging { get { return ResourceManager.GetString("ListView_OnPagePropertiesChanging", resourceCulture); } } ////// Looks up a localized string similar to Fires when an item is selected in the ListView, after the selection is complete.. /// internal static string ListView_OnSelectedIndexChanged { get { return ResourceManager.GetString("ListView_OnSelectedIndexChanged", resourceCulture); } } ////// Looks up a localized string similar to Fires when an item is selected in the ListView, before the item is selected.. /// internal static string ListView_OnSelectedIndexChanging { get { return ResourceManager.GetString("ListView_OnSelectedIndexChanging", resourceCulture); } } ////// Looks up a localized string similar to Fires when a field is sorted in the ListView, after the sort is complete.. /// internal static string ListView_OnSorted { get { return ResourceManager.GetString("ListView_OnSorted", resourceCulture); } } ////// Looks up a localized string similar to Fires when a field is sorted in the ListView, before the sort occurs.. /// internal static string ListView_OnSorting { get { return ResourceManager.GetString("ListView_OnSorting", resourceCulture); } } ////// Looks up a localized string similar to The index of the currently selected item.. /// internal static string ListView_SelectedIndex { get { return ResourceManager.GetString("ListView_SelectedIndex", resourceCulture); } } ////// Looks up a localized string similar to The template used for the currently selected item.. /// internal static string ListView_SelectedItemTemplate { get { return ResourceManager.GetString("ListView_SelectedItemTemplate", resourceCulture); } } ////// Looks up a localized string similar to The direction in which to sort the field.. /// internal static string ListView_SortDirection { get { return ResourceManager.GetString("ListView_SortDirection", resourceCulture); } } ////// Looks up a localized string similar to Sort expression used to sort the data source to which the ListView is binding.. /// internal static string ListView_SortExpression { get { return ResourceManager.GetString("ListView_SortExpression", resourceCulture); } } ////// Looks up a localized string similar to Style properties are not supported on ListView.. /// internal static string ListView_StyleNotSupported { get { return ResourceManager.GetString("ListView_StyleNotSupported", resourceCulture); } } ////// Looks up a localized string similar to Style properties are not supported on ListView. Apply styling or CSS classes to the elements inside ListView's templates.. /// internal static string ListView_StylePropertiesNotSupported { get { return ResourceManager.GetString("ListView_StylePropertiesNotSupported", resourceCulture); } } ////// Looks up a localized string similar to The ListView '{0}' raised event {1} which wasn't handled.. /// internal static string ListView_UnhandledEvent { get { return ResourceManager.GetString("ListView_UnhandledEvent", resourceCulture); } } ////// Looks up a localized string similar to Cannot compute Count for a data source that does not implement ICollection.. /// internal static string ListViewPagedDataSource_CannotGetCount { get { return ResourceManager.GetString("ListViewPagedDataSource_CannotGetCount", resourceCulture); } } ////// Looks up a localized string similar to You must call MoveNext on IEnumerator before accessing the Current property.. /// internal static string ListViewPagedDataSource_EnumeratorMoveNextNotCalled { get { return ResourceManager.GetString("ListViewPagedDataSource_EnumeratorMoveNextNotCalled", resourceCulture); } } ////// Looks up a localized string similar to The CSS class applied to the next and previous buttons.. /// internal static string NextPreviousPagerField_ButtonCssClass { get { return ResourceManager.GetString("NextPreviousPagerField_ButtonCssClass", resourceCulture); } } ////// Looks up a localized string similar to The type of button contained within the pager field.. /// internal static string NextPreviousPagerField_ButtonType { get { return ResourceManager.GetString("NextPreviousPagerField_ButtonType", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the first page button if the ButtonType is Image.. /// internal static string NextPreviousPagerField_FirstPageImageUrl { get { return ResourceManager.GetString("NextPreviousPagerField_FirstPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the first page button.. /// internal static string NextPreviousPagerField_FirstPageText { get { return ResourceManager.GetString("NextPreviousPagerField_FirstPageText", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the last page button if the ButtonType is Image.. /// internal static string NextPreviousPagerField_LastPageImageUrl { get { return ResourceManager.GetString("NextPreviousPagerField_LastPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the last page button.. /// internal static string NextPreviousPagerField_LastPageText { get { return ResourceManager.GetString("NextPreviousPagerField_LastPageText", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the next page button if the ButtonType is Image.. /// internal static string NextPreviousPagerField_NextPageImageUrl { get { return ResourceManager.GetString("NextPreviousPagerField_NextPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the next page button.. /// internal static string NextPreviousPagerField_NextPageText { get { return ResourceManager.GetString("NextPreviousPagerField_NextPageText", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the previous page button if the ButtonType is Image.. /// internal static string NextPreviousPagerField_PreviousPageImageUrl { get { return ResourceManager.GetString("NextPreviousPagerField_PreviousPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the previous page button.. /// internal static string NextPreviousPagerField_PreviousPageText { get { return ResourceManager.GetString("NextPreviousPagerField_PreviousPageText", resourceCulture); } } ////// Looks up a localized string similar to Whether disabled pager links should be rendered as labels rather than buttons.. /// internal static string NextPreviousPagerField_RenderDisabledButtonsAsLabels { get { return ResourceManager.GetString("NextPreviousPagerField_RenderDisabledButtonsAsLabels", resourceCulture); } } ////// Looks up a localized string similar to Whether non-breaking spaces should be rendered between pager controls.. /// internal static string NextPreviousPagerField_RenderNonBreakingSpacesBetweenControls { get { return ResourceManager.GetString("NextPreviousPagerField_RenderNonBreakingSpacesBetweenControls", resourceCulture); } } ////// Looks up a localized string similar to Whether the pager field should display the first page button.. /// internal static string NextPreviousPagerField_ShowFirstPageButton { get { return ResourceManager.GetString("NextPreviousPagerField_ShowFirstPageButton", resourceCulture); } } ////// Looks up a localized string similar to Whether the pager field should display the last page button.. /// internal static string NextPreviousPagerField_ShowLastPageButton { get { return ResourceManager.GetString("NextPreviousPagerField_ShowLastPageButton", resourceCulture); } } ////// Looks up a localized string similar to Whether the pager field should display the next page button.. /// internal static string NextPreviousPagerField_ShowNextPageButton { get { return ResourceManager.GetString("NextPreviousPagerField_ShowNextPageButton", resourceCulture); } } ////// Looks up a localized string similar to Whether the pager field should display the previous page button.. /// internal static string NextPreviousPagerField_ShowPreviousPageButton { get { return ResourceManager.GetString("NextPreviousPagerField_ShowPreviousPageButton", resourceCulture); } } ////// Looks up a localized string similar to First. /// internal static string NextPrevPagerField_DefaultFirstPageText { get { return ResourceManager.GetString("NextPrevPagerField_DefaultFirstPageText", resourceCulture); } } ////// Looks up a localized string similar to Last. /// internal static string NextPrevPagerField_DefaultLastPageText { get { return ResourceManager.GetString("NextPrevPagerField_DefaultLastPageText", resourceCulture); } } ////// Looks up a localized string similar to Next. /// internal static string NextPrevPagerField_DefaultNextPageText { get { return ResourceManager.GetString("NextPrevPagerField_DefaultNextPageText", resourceCulture); } } ////// Looks up a localized string similar to Previous. /// internal static string NextPrevPagerField_DefaultPreviousPageText { get { return ResourceManager.GetString("NextPrevPagerField_DefaultPreviousPageText", resourceCulture); } } ////// Looks up a localized string similar to The maximum number of page number buttons that can be displayed by the pager field.. /// internal static string NumericPagerField_ButtonCount { get { return ResourceManager.GetString("NumericPagerField_ButtonCount", resourceCulture); } } ////// Looks up a localized string similar to The type of button contained within the pager field.. /// internal static string NumericPagerField_ButtonType { get { return ResourceManager.GetString("NumericPagerField_ButtonType", resourceCulture); } } ////// Looks up a localized string similar to The CSS class applied to the label containing the current page number.. /// internal static string NumericPagerField_CurrentPageLabelCssClass { get { return ResourceManager.GetString("NumericPagerField_CurrentPageLabelCssClass", resourceCulture); } } ////// Looks up a localized string similar to .... /// internal static string NumericPagerField_DefaultNextPageText { get { return ResourceManager.GetString("NumericPagerField_DefaultNextPageText", resourceCulture); } } ////// Looks up a localized string similar to .... /// internal static string NumericPagerField_DefaultPreviousPageText { get { return ResourceManager.GetString("NumericPagerField_DefaultPreviousPageText", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the next page button if the ButtonType is Image.. /// internal static string NumericPagerField_NextPageImageUrl { get { return ResourceManager.GetString("NumericPagerField_NextPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the next page button.. /// internal static string NumericPagerField_NextPageText { get { return ResourceManager.GetString("NumericPagerField_NextPageText", resourceCulture); } } ////// Looks up a localized string similar to The CSS class applied to the next and previous buttons.. /// internal static string NumericPagerField_NextPreviousButtonCssClass { get { return ResourceManager.GetString("NumericPagerField_NextPreviousButtonCssClass", resourceCulture); } } ////// Looks up a localized string similar to The CSS class applied to the numeric pager buttons.. /// internal static string NumericPagerField_NumericButtonCssClass { get { return ResourceManager.GetString("NumericPagerField_NumericButtonCssClass", resourceCulture); } } ////// Looks up a localized string similar to The URL of the image of the previous page button if the ButtonType is Image.. /// internal static string NumericPagerField_PreviousPageImageUrl { get { return ResourceManager.GetString("NumericPagerField_PreviousPageImageUrl", resourceCulture); } } ////// Looks up a localized string similar to The text of the previous page button.. /// internal static string NumericPagerField_PreviousPageText { get { return ResourceManager.GetString("NumericPagerField_PreviousPageText", resourceCulture); } } ////// Looks up a localized string similar to Whether non-breaking spaces should be rendered between pager controls.. /// internal static string NumericPagerField_RenderNonBreakingSpacesBetweenControls { get { return ResourceManager.GetString("NumericPagerField_RenderNonBreakingSpacesBetweenControls", resourceCulture); } } ////// Looks up a localized string similar to RegisterDataItem can only be called during an async postback.. /// internal static string PageRequestManager_RegisterDataItemInNonAsyncRequest { get { return ResourceManager.GetString("PageRequestManager_RegisterDataItemInNonAsyncRequest", resourceCulture); } } ////// Looks up a localized string similar to The control '{0}' already has a data item registered.. /// internal static string PageRequestManager_RegisterDataItemTwice { get { return ResourceManager.GetString("PageRequestManager_RegisterDataItemTwice", resourceCulture); } } ////// Looks up a localized string similar to Object is not a DataPagerField.. /// internal static string PagerFieldCollection_InvalidType { get { return ResourceManager.GetString("PagerFieldCollection_InvalidType", resourceCulture); } } ////// Looks up a localized string similar to Type index is out of bounds.. /// internal static string PagerFieldCollection_InvalidTypeIndex { get { return ResourceManager.GetString("PagerFieldCollection_InvalidTypeIndex", resourceCulture); } } ////// Looks up a localized string similar to {0} (at index {1}). /// internal static string ParseException_ParseExceptionFormat { get { return ResourceManager.GetString("ParseException_ParseExceptionFormat", resourceCulture); } } ////// Looks up a localized string similar to The attribute 'LoadProperties' can only be used when using the default ProfileService.. /// internal static string ProfileServiceManager_LoadProperitesWithNonDefaultPath { get { return ResourceManager.GetString("ProfileServiceManager_LoadProperitesWithNonDefaultPath", resourceCulture); } } ////// Looks up a localized string similar to Specifies profile properties that should be rendered inline with the page.. /// internal static string ProfileServiceManager_LoadProperties { get { return ResourceManager.GetString("ProfileServiceManager_LoadProperties", resourceCulture); } } ////// Looks up a localized string similar to Type {0} is not supported.. /// internal static string ProxyGenerator_UnsupportedType { get { return ResourceManager.GetString("ProxyGenerator_UnsupportedType", resourceCulture); } } ////// Looks up a localized string similar to Error status code returned by the Web Service: {0}. Error details from service: {1}. /// internal static string ProxyHelper_BadStatusCode { get { return ResourceManager.GetString("ProxyHelper_BadStatusCode", resourceCulture); } } ////// Looks up a localized string similar to Role Provider could not be found.. /// internal static string RoleService_RoleProviderNotFound { get { return ResourceManager.GetString("RoleService_RoleProviderNotFound", resourceCulture); } } ////// Looks up a localized string similar to The Role Manager feature has not been enabled.. /// internal static string RoleService_RolesFeatureNotEnabled { get { return ResourceManager.GetString("RoleService_RolesFeatureNotEnabled", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether user roles are rendered inline with the page.. /// internal static string RoleServiceManager_LoadRoles { get { return ResourceManager.GetString("RoleServiceManager_LoadRoles", resourceCulture); } } ////// Looks up a localized string similar to For RoleService, 'loadRoles' property must be set to false when the 'path' property is set to a value different from the default value.. /// internal static string RoleServiceManager_LoadRolesWithNonDefaultPath { get { return ResourceManager.GetString("RoleServiceManager_LoadRolesWithNonDefaultPath", resourceCulture); } } ////// Looks up a localized string similar to The 'ID' property on ScriptControlDescriptor is not settable. The client ID of a script control is always equal to its element ID.. /// internal static string ScriptControlDescriptor_IDNotSettable { get { return ResourceManager.GetString("ScriptControlDescriptor_IDNotSettable", resourceCulture); } } ////// Looks up a localized string similar to Extender control '{0}' is not a registered extender control. Extender controls must be registered using RegisterExtenderControl() before calling RegisterScriptDescriptors().. /// internal static string ScriptControlManager_ExtenderControlNotRegistered { get { return ResourceManager.GetString("ScriptControlManager_ExtenderControlNotRegistered", resourceCulture); } } ////// Looks up a localized string similar to Extender control type '{0}' does not have any attributes of type '{1}'. Extender control types must have at least one attribute of type '{1}'.. /// internal static string ScriptControlManager_NoTargetControlTypes { get { return ResourceManager.GetString("ScriptControlManager_NoTargetControlTypes", resourceCulture); } } ////// Looks up a localized string similar to Extender controls may not be registered before PreRender.. /// internal static string ScriptControlManager_RegisterExtenderControlTooEarly { get { return ResourceManager.GetString("ScriptControlManager_RegisterExtenderControlTooEarly", resourceCulture); } } ////// Looks up a localized string similar to Extender controls may not be registered after PreRender.. /// internal static string ScriptControlManager_RegisterExtenderControlTooLate { get { return ResourceManager.GetString("ScriptControlManager_RegisterExtenderControlTooLate", resourceCulture); } } ////// Looks up a localized string similar to Script controls may not be registered before PreRender.. /// internal static string ScriptControlManager_RegisterScriptControlTooEarly { get { return ResourceManager.GetString("ScriptControlManager_RegisterScriptControlTooEarly", resourceCulture); } } ////// Looks up a localized string similar to Script controls may not be registered after PreRender.. /// internal static string ScriptControlManager_RegisterScriptControlTooLate { get { return ResourceManager.GetString("ScriptControlManager_RegisterScriptControlTooLate", resourceCulture); } } ////// Looks up a localized string similar to Script control '{0}' is not a registered script control. Script controls must be registered using RegisterScriptControl() before calling RegisterScriptDescriptors().. /// internal static string ScriptControlManager_ScriptControlNotRegistered { get { return ResourceManager.GetString("ScriptControlManager_ScriptControlNotRegistered", resourceCulture); } } ////// Looks up a localized string similar to Extender control '{0}' cannot extend '{1}'. Extender controls of type '{2}' cannot extend controls of type '{3}'.. /// internal static string ScriptControlManager_TargetControlTypeInvalid { get { return ResourceManager.GetString("ScriptControlManager_TargetControlTypeInvalid", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether custom error redirects will occur during an async postback.. /// internal static string ScriptManager_AllowCustomErrorsRedirect { get { return ResourceManager.GetString("ScriptManager_AllowCustomErrorsRedirect", resourceCulture); } } ////// Looks up a localized string similar to This event is raised to allow customization of the error message sent to the client during an async postback.. /// internal static string ScriptManager_AsyncPostBackError { get { return ResourceManager.GetString("ScriptManager_AsyncPostBackError", resourceCulture); } } ////// Looks up a localized string similar to The error message to be sent to the client when an unhandled exception occurs on the server. The property can be set declaratively in the page markup or during the ScriptManager's AsyncPostBackError event. If the value is empty the exception's message will be used.. /// internal static string ScriptManager_AsyncPostBackErrorMessage { get { return ResourceManager.GetString("ScriptManager_AsyncPostBackErrorMessage", resourceCulture); } } ////// Looks up a localized string similar to The page is performing an async postback but the ScriptManager.SupportsPartialRendering property is set to false. Ensure that the property is set to true during an async postback.. /// internal static string ScriptManager_AsyncPostBackNotInPartialRenderingMode { get { return ResourceManager.GetString("ScriptManager_AsyncPostBackNotInPartialRenderingMode", resourceCulture); } } ////// Looks up a localized string similar to The timeout period in seconds for async postbacks. A value of zero indicates no timeout.. /// internal static string ScriptManager_AsyncPostBackTimeout { get { return ResourceManager.GetString("ScriptManager_AsyncPostBackTimeout", resourceCulture); } } ////// Looks up a localized string similar to Contains preferences for the client side authentication service.. /// internal static string ScriptManager_AuthenticationService { get { return ResourceManager.GetString("ScriptManager_AuthenticationService", resourceCulture); } } ////// Looks up a localized string similar to A history point can only be created during an asynchronous postback.. /// internal static string ScriptManager_CannotAddHistoryPointOutsideOfAsyncPostBack { get { return ResourceManager.GetString("ScriptManager_CannotAddHistoryPointOutsideOfAsyncPostBack", resourceCulture); } } ////// Looks up a localized string similar to A history point can only be added if EnableHistory is set to true.. /// internal static string ScriptManager_CannotAddHistoryPointWithHistoryDisabled { get { return ResourceManager.GetString("ScriptManager_CannotAddHistoryPointWithHistoryDisabled", resourceCulture); } } ////// Looks up a localized string similar to The EnableHistory property cannot be changed after the Init event.. /// internal static string ScriptManager_CannotChangeEnableHistory { get { return ResourceManager.GetString("ScriptManager_CannotChangeEnableHistory", resourceCulture); } } ////// Looks up a localized string similar to The EnablePartialRendering property cannot be changed after the Init event.. /// internal static string ScriptManager_CannotChangeEnablePartialRendering { get { return ResourceManager.GetString("ScriptManager_CannotChangeEnablePartialRendering", resourceCulture); } } ////// Looks up a localized string similar to The EnableScriptGlobalization property cannot be changed during async postbacks or after the Init event.. /// internal static string ScriptManager_CannotChangeEnableScriptGlobalization { get { return ResourceManager.GetString("ScriptManager_CannotChangeEnableScriptGlobalization", resourceCulture); } } ////// Looks up a localized string similar to The SupportsPartialRendering property cannot be changed after the Init event.. /// internal static string ScriptManager_CannotChangeSupportsPartialRendering { get { return ResourceManager.GetString("ScriptManager_CannotChangeSupportsPartialRendering", resourceCulture); } } ////// Looks up a localized string similar to Control with ID '{0}' cannot be registered through both RegisterAsyncPostBackControl and RegisterPostBackControl. This can happen if you have conflicting triggers associated with the target control.. /// internal static string ScriptManager_CannotRegisterBothPostBacks { get { return ResourceManager.GetString("ScriptManager_CannotRegisterBothPostBacks", resourceCulture); } } ////// Looks up a localized string similar to A script reference cannot be included multiple times in composite script references.. /// internal static string ScriptManager_CannotRegisterScriptInMultipleCompositeReferences { get { return ResourceManager.GetString("ScriptManager_CannotRegisterScriptInMultipleCompositeReferences", resourceCulture); } } ////// Looks up a localized string similar to The SupportsPartialRendering property cannot be set when EnablePartialRendering is false.. /// internal static string ScriptManager_CannotSetSupportsPartialRenderingWhenDisabled { get { return ResourceManager.GetString("ScriptManager_CannotSetSupportsPartialRenderingWhenDisabled", resourceCulture); } } ////// Looks up a localized string similar to Specifies a client-side event handler name for the navigate event.. /// internal static string ScriptManager_ClientNavigateHandler { get { return ResourceManager.GetString("ScriptManager_ClientNavigateHandler", resourceCulture); } } ////// Looks up a localized string similar to Enables the composition of individual script references into one to minimize the number of requests to the server.. /// internal static string ScriptManager_CompositeScript { get { return ResourceManager.GetString("ScriptManager_CompositeScript", resourceCulture); } } ////// Looks up a localized string similar to The URL of an empty page that will be used to manage history on Internet Explorer. The script manager uses a built-in, resource-based page if this property is unspecified.. /// internal static string ScriptManager_EmptyPageUrl { get { return ResourceManager.GetString("ScriptManager_EmptyPageUrl", resourceCulture); } } ////// Looks up a localized string similar to Enables ScriptManager to manage browser history on supported browsers.. /// internal static string ScriptManager_EnableHistory { get { return ResourceManager.GetString("ScriptManager_EnableHistory", resourceCulture); } } ////// Looks up a localized string similar to Enables page methods.. /// internal static string ScriptManager_EnablePageMethods { get { return ResourceManager.GetString("ScriptManager_EnablePageMethods", resourceCulture); } } ////// Looks up a localized string similar to Enables asynchronous postbacks for the UpdatePanel control on supported browsers. To override the default browser support detection you can set the SupportsPartialRendering property.. /// internal static string ScriptManager_EnablePartialRendering { get { return ResourceManager.GetString("ScriptManager_EnablePartialRendering", resourceCulture); } } ////// Looks up a localized string similar to Enables ScriptManager to add client-side globalization information to the page for the current culture.. /// internal static string ScriptManager_EnableScriptGlobalization { get { return ResourceManager.GetString("ScriptManager_EnableScriptGlobalization", resourceCulture); } } ////// Looks up a localized string similar to Enables ScriptManager to generate localized versions of script files if they are available.. /// internal static string ScriptManager_EnableScriptLocalization { get { return ResourceManager.GetString("ScriptManager_EnableScriptLocalization", resourceCulture); } } ////// Looks up a localized string similar to When true, the server-side history state is hashed using the same settings as ViewState. When false, the server history state is a clear-text string dictionary that can be modified by the end user by modifying the url.. /// internal static string ScriptManager_EnableSecureHistoryState { get { return ResourceManager.GetString("ScriptManager_EnableSecureHistoryState", resourceCulture); } } ////// Looks up a localized string similar to ASP.NET Ajax client-side framework failed to load.. /// internal static string ScriptManager_FrameworkFailedToLoad { get { return ResourceManager.GetString("ScriptManager_FrameworkFailedToLoad", resourceCulture); } } ////// Looks up a localized string similar to Control with ID '{0}' being registered through RegisterAsyncPostBackControl or RegisterPostBackControl must implement either INamingContainer, IPostBackDataHandler, or IPostBackEventHandler.. /// internal static string ScriptManager_InvalidControlRegistration { get { return ResourceManager.GetString("ScriptManager_InvalidControlRegistration", resourceCulture); } } ////// Looks up a localized string similar to Specifies that script references should be loaded before the UI is rendered in the browser.. /// internal static string ScriptManager_LoadScriptsBeforeUI { get { return ResourceManager.GetString("ScriptManager_LoadScriptsBeforeUI", resourceCulture); } } ////// Looks up a localized string similar to This event is raised during asynchronous postbacks when the server-side history state changes.. /// internal static string ScriptManager_Navigate { get { return ResourceManager.GetString("ScriptManager_Navigate", resourceCulture); } } ////// Looks up a localized string similar to Only one instance of a ScriptManager can be added to the page.. /// internal static string ScriptManager_OnlyOneScriptManager { get { return ResourceManager.GetString("ScriptManager_OnlyOneScriptManager", resourceCulture); } } ////// Looks up a localized string similar to Untitled Page. /// internal static string ScriptManager_PageUntitled { get { return ResourceManager.GetString("ScriptManager_PageUntitled", resourceCulture); } } ////// Looks up a localized string similar to Contains preferences for the client side profile service.. /// internal static string ScriptManager_ProfileService { get { return ResourceManager.GetString("ScriptManager_ProfileService", resourceCulture); } } ////// Looks up a localized string similar to This event is raised to allow modifications to composite script references before they are rendered.. /// internal static string ScriptManager_ResolveCompositeScriptReference { get { return ResourceManager.GetString("ScriptManager_ResolveCompositeScriptReference", resourceCulture); } } ////// Looks up a localized string similar to This event is raised to allow modifications to script references before they are rendered.. /// internal static string ScriptManager_ResolveScriptReference { get { return ResourceManager.GetString("ScriptManager_ResolveScriptReference", resourceCulture); } } ////// Looks up a localized string similar to Contains preferences for the client side authentication service.. /// internal static string ScriptManager_RoleService { get { return ResourceManager.GetString("ScriptManager_RoleService", resourceCulture); } } ////// Looks up a localized string similar to Indicates the type of scripts to load when more than one type is available.. /// internal static string ScriptManager_ScriptMode { get { return ResourceManager.GetString("ScriptManager_ScriptMode", resourceCulture); } } ////// Looks up a localized string similar to Specifies that scripts should be loaded from this path instead of from assembly web resources.. /// internal static string ScriptManager_ScriptPath { get { return ResourceManager.GetString("ScriptManager_ScriptPath", resourceCulture); } } ////// Looks up a localized string similar to A collection of script references that the ScriptManager should include in the page. The Scripts collections on the ScriptManager and ScriptManagerProxy controls are merged at runtime.. /// internal static string ScriptManager_Scripts { get { return ResourceManager.GetString("ScriptManager_Scripts", resourceCulture); } } ////// Looks up a localized string similar to A collection of service references that the ScriptManager should include in the page. The Services collections on the ScriptManager and ScriptManagerProxy controls are merged at runtime.. /// internal static string ScriptManager_Services { get { return ResourceManager.GetString("ScriptManager_Services", resourceCulture); } } ////// Looks up a localized string similar to Cannot unregister UpdatePanel with ID '{0}' since it was not registered with the ScriptManager. This might occur if the UpdatePanel was removed from the control tree and later added again, which is not supported.. /// internal static string ScriptManager_UpdatePanelNotRegistered { get { return ResourceManager.GetString("ScriptManager_UpdatePanelNotRegistered", resourceCulture); } } ////// Looks up a localized string similar to The assembly that contains the script as a web resource.. /// internal static string ScriptReference_Assembly { get { return ResourceManager.GetString("ScriptReference_Assembly", resourceCulture); } } ////// Looks up a localized string similar to Assembly cannot be defined without Name.. /// internal static string ScriptReference_AssemblyRequiresName { get { return ResourceManager.GetString("ScriptReference_AssemblyRequiresName", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether this script reference should ignore the ScriptManager.ScriptPath property.. /// internal static string ScriptReference_IgnoreScriptPath { get { return ResourceManager.GetString("ScriptReference_IgnoreScriptPath", resourceCulture); } } ////// Looks up a localized string similar to '{0}' is not a valid script name. The name must end in '.js'.. /// internal static string ScriptReference_InvalidReleaseScriptName { get { return ResourceManager.GetString("ScriptReference_InvalidReleaseScriptName", resourceCulture); } } ////// Looks up a localized string similar to '{0}' is not a valid script path. The path must end in '.js'.. /// internal static string ScriptReference_InvalidReleaseScriptPath { get { return ResourceManager.GetString("ScriptReference_InvalidReleaseScriptPath", resourceCulture); } } ////// Looks up a localized string similar to The name of the web resource.. /// internal static string ScriptReference_Name { get { return ResourceManager.GetString("ScriptReference_Name", resourceCulture); } } ////// Looks up a localized string similar to Name and Path cannot both be empty.. /// internal static string ScriptReference_NameAndPathCannotBeEmpty { get { return ResourceManager.GetString("ScriptReference_NameAndPathCannotBeEmpty", resourceCulture); } } ////// Looks up a localized string similar to Specifies if the script resource loader should automatically append a script loaded notification statement.. /// internal static string ScriptReference_NotifyScriptLoaded { get { return ResourceManager.GetString("ScriptReference_NotifyScriptLoaded", resourceCulture); } } ////// Looks up a localized string similar to The path to the script.. /// internal static string ScriptReference_Path { get { return ResourceManager.GetString("ScriptReference_Path", resourceCulture); } } ////// Looks up a localized string similar to A comma-delimited string of valid UI cultures supported by the path. ResourceUICultures is only valid with Path.. /// internal static string ScriptReference_ResourceUICultures { get { return ResourceManager.GetString("ScriptReference_ResourceUICultures", resourceCulture); } } ////// Looks up a localized string similar to Specifies the algorithm for choosing between the debug and release scripts.. /// internal static string ScriptReference_ScriptMode { get { return ResourceManager.GetString("ScriptReference_ScriptMode", resourceCulture); } } ////// Looks up a localized string similar to The control must be in the control tree of a page.. /// internal static string ScriptRegistrationManager_ControlNotOnPage { get { return ResourceManager.GetString("ScriptRegistrationManager_ControlNotOnPage", resourceCulture); } } ////// Looks up a localized string similar to The script tag registered for type '{0}' and key '{1}' has invalid characters outside of the script tags: {2}. Only properly formatted script tags can be registered.. /// internal static string ScriptRegistrationManager_InvalidChars { get { return ResourceManager.GetString("ScriptRegistrationManager_InvalidChars", resourceCulture); } } ////// Looks up a localized string similar to The script tag registered for type '{0}' and key '{1}' is missing a matching close tag.. /// internal static string ScriptRegistrationManager_NoCloseTag { get { return ResourceManager.GetString("ScriptRegistrationManager_NoCloseTag", resourceCulture); } } ////// Looks up a localized string similar to The script tag registered for type '{0}' and key '{1}' does not contain any valid script tags.. /// internal static string ScriptRegistrationManager_NoTags { get { return ResourceManager.GetString("ScriptRegistrationManager_NoTags", resourceCulture); } } ////// Looks up a localized string similar to Script resource handler can only serve resources from file-based assemblies.. /// internal static string ScriptResourceHandler_AssemblyNotFileBased { get { return ResourceManager.GetString("ScriptResourceHandler_AssemblyNotFileBased", resourceCulture); } } ////// Looks up a localized string similar to More than one ScriptResourceAttribute points to script '{0}' in assembly '{1}'.. /// internal static string ScriptResourceHandler_DuplicateScriptResources { get { return ResourceManager.GetString("ScriptResourceHandler_DuplicateScriptResources", resourceCulture); } } ////// Looks up a localized string similar to This is an invalid script resource request.. /// internal static string ScriptResourceHandler_InvalidRequest { get { return ResourceManager.GetString("ScriptResourceHandler_InvalidRequest", resourceCulture); } } ////// Looks up a localized string similar to The resource URL cannot be longer than 1024 characters. If using a CompositeScriptReference, reduce the number of ScriptReferences it contains, or combine them into a single static file and set the Path property to the location of it.. /// internal static string ScriptResourceHandler_ResourceUrlLongerThan1024Characters { get { return ResourceManager.GetString("ScriptResourceHandler_ResourceUrlLongerThan1024Characters", resourceCulture); } } ////// Looks up a localized string similar to The type names for the debug and release versions of resource {0} don't match.. /// internal static string ScriptResourceHandler_TypeNameMismatch { get { return ResourceManager.GetString("ScriptResourceHandler_TypeNameMismatch", resourceCulture); } } ////// Looks up a localized string similar to Web resource '{0}' was not found.. /// internal static string ScriptResourceHandler_UnknownResource { get { return ResourceManager.GetString("ScriptResourceHandler_UnknownResource", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether this service reference should have its proxy script rendered inline in the page.. /// internal static string ServiceReference_InlineScript { get { return ResourceManager.GetString("ServiceReference_InlineScript", resourceCulture); } } ////// Looks up a localized string similar to The path to the service being referenced.. /// internal static string ServiceReference_Path { get { return ResourceManager.GetString("ServiceReference_Path", resourceCulture); } } ////// Looks up a localized string similar to Path cannot be empty.. /// internal static string ServiceReference_PathCannotBeEmpty { get { return ResourceManager.GetString("ServiceReference_PathCannotBeEmpty", resourceCulture); } } ////// Looks up a localized string similar to The serviceUri configuration setting was not found.. /// internal static string ServiceUriNotFound { get { return ResourceManager.GetString("ServiceUriNotFound", resourceCulture); } } ////// Looks up a localized string similar to Unable to connect to the Microsoft SQL Everywhere Service using the specified connection string. Make sure that Microsoft SQL Server Everywhere is correctly installed on this computer.. /// internal static string SqlHelper_SqlEverywhereNotInstalled { get { return ResourceManager.GetString("SqlHelper_SqlEverywhereNotInstalled", resourceCulture); } } ////// Looks up a localized string similar to Fires when an event is generated within the pager field.. /// internal static string TemplatePagerField_OnPagerCommand { get { return ResourceManager.GetString("TemplatePagerField_OnPagerCommand", resourceCulture); } } ////// Looks up a localized string similar to The template used in the pager field.. /// internal static string TemplatePagerField_PagerTemplate { get { return ResourceManager.GetString("TemplatePagerField_PagerTemplate", resourceCulture); } } ////// Looks up a localized string similar to The TemplatePagerField raised event {0} which wasn't handled.. /// internal static string TemplatePagerField_UnhandledEvent { get { return ResourceManager.GetString("TemplatePagerField_UnhandledEvent", resourceCulture); } } ////// Looks up a localized string similar to The interval must be greater than zero.. /// internal static string Timer_IntervalMustBeGreaterThanZero { get { return ResourceManager.GetString("Timer_IntervalMustBeGreaterThanZero", resourceCulture); } } ////// Looks up a localized string similar to Enables raising of Tick events.. /// internal static string Timer_TimerEnable { get { return ResourceManager.GetString("Timer_TimerEnable", resourceCulture); } } ////// Looks up a localized string similar to The duration between Tick events in milliseconds.. /// internal static string Timer_TimerInterval { get { return ResourceManager.GetString("Timer_TimerInterval", resourceCulture); } } ////// Looks up a localized string similar to Occurs whenever the specified interval time elapses.. /// internal static string Timer_TimerTick { get { return ResourceManager.GetString("Timer_TimerTick", resourceCulture); } } ////// Looks up a localized string similar to An unhandled exception has occurred.. /// internal static string UnhandledExceptionEventLogMessage { get { return ResourceManager.GetString("UnhandledExceptionEventLogMessage", resourceCulture); } } ////// Looks up a localized string similar to The Controls property of UpdatePanel with ID '{0}' cannot be modified directly. To change the contents of the UpdatePanel modify the child controls of the ContentTemplateContainer property.. /// internal static string UpdatePanel_CannotModifyControlCollection { get { return ResourceManager.GetString("UpdatePanel_CannotModifyControlCollection", resourceCulture); } } ////// Looks up a localized string similar to The ContentTemplate of UpdatePanel with ID '{0}' cannot be changed after it has been instantiated.. /// internal static string UpdatePanel_CannotSetContentTemplate { get { return ResourceManager.GetString("UpdatePanel_CannotSetContentTemplate", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether postbacks coming from the UpdatePanel's child controls will cause the UpdatePanel to refresh.. /// internal static string UpdatePanel_ChildrenAsTriggers { get { return ResourceManager.GetString("UpdatePanel_ChildrenAsTriggers", resourceCulture); } } ////// Looks up a localized string similar to ChildrenAsTriggers cannot be set to false when UpdateMode is set to Always on UpdatePanel '{0}'.. /// internal static string UpdatePanel_ChildrenTriggersAndUpdateAlways { get { return ResourceManager.GetString("UpdatePanel_ChildrenTriggersAndUpdateAlways", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether the UpdatePanel should render as a block tag (<div>) or an inline tag (<span>).. /// internal static string UpdatePanel_RenderMode { get { return ResourceManager.GetString("UpdatePanel_RenderMode", resourceCulture); } } ////// Looks up a localized string similar to SetPartialRenderingMode can only be called once.. /// internal static string UpdatePanel_SetPartialRenderingModeCalledOnce { get { return ResourceManager.GetString("UpdatePanel_SetPartialRenderingModeCalledOnce", resourceCulture); } } ////// Looks up a localized string similar to A collection of triggers that can cause the UpdatePanel to be updated.. /// internal static string UpdatePanel_Triggers { get { return ResourceManager.GetString("UpdatePanel_Triggers", resourceCulture); } } ////// Looks up a localized string similar to The Update method can only be called on UpdatePanel with ID '{0}' when UpdateMode is set to Conditional.. /// internal static string UpdatePanel_UpdateConditional { get { return ResourceManager.GetString("UpdatePanel_UpdateConditional", resourceCulture); } } ////// Looks up a localized string similar to Indicates whether the UpdatePanel will refresh on every asynchronous postback or only as the result of a specific action, such as a call to UpdatePanel.Update().. /// internal static string UpdatePanel_UpdateMode { get { return ResourceManager.GetString("UpdatePanel_UpdateMode", resourceCulture); } } ////// Looks up a localized string similar to The Update method can only be called on UpdatePanel with ID '{0}' before Render.. /// internal static string UpdatePanel_UpdateTooLate { get { return ResourceManager.GetString("UpdatePanel_UpdateTooLate", resourceCulture); } } ////// Looks up a localized string similar to The trigger's target control ID.. /// internal static string UpdatePanelControlTrigger_ControlID { get { return ResourceManager.GetString("UpdatePanelControlTrigger_ControlID", resourceCulture); } } ////// Looks up a localized string similar to A control with ID '{0}' could not be found for the trigger in UpdatePanel '{1}'.. /// internal static string UpdatePanelControlTrigger_ControlNotFound { get { return ResourceManager.GetString("UpdatePanelControlTrigger_ControlNotFound", resourceCulture); } } ////// Looks up a localized string similar to The ControlID property must be set on the trigger in UpdatePanel '{0}'.. /// internal static string UpdatePanelControlTrigger_NoControlID { get { return ResourceManager.GetString("UpdatePanelControlTrigger_NoControlID", resourceCulture); } } ////// Looks up a localized string similar to UpdatePanel that this UpdateProgress is associated with.. /// internal static string UpdateProgress_AssociatedUpdatePanelID { get { return ResourceManager.GetString("UpdateProgress_AssociatedUpdatePanelID", resourceCulture); } } ////// Looks up a localized string similar to Time in ms after which the ProgressTemplate is displayed.. /// internal static string UpdateProgress_DisplayAfter { get { return ResourceManager.GetString("UpdateProgress_DisplayAfter", resourceCulture); } } ////// Looks up a localized string similar to DisplayAfter must be a non negative integer.. /// internal static string UpdateProgress_DisplayAfterInvalid { get { return ResourceManager.GetString("UpdateProgress_DisplayAfterInvalid", resourceCulture); } } ////// Looks up a localized string similar to Determines whether the progress template is dynamically rendered.. /// internal static string UpdateProgress_DynamicLayout { get { return ResourceManager.GetString("UpdateProgress_DynamicLayout", resourceCulture); } } ////// Looks up a localized string similar to No UpdatePanel found for AssociatedUpdatePanelID '{0}'.. /// internal static string UpdateProgress_NoUpdatePanel { get { return ResourceManager.GetString("UpdateProgress_NoUpdatePanel", resourceCulture); } } ////// Looks up a localized string similar to ProgressTemplate which is displayed during async postbacks.. /// internal static string UpdateProgress_ProgressTemplate { get { return ResourceManager.GetString("UpdateProgress_ProgressTemplate", resourceCulture); } } ////// Looks up a localized string similar to A ProgressTemplate must be specified on UpdateProgress control with ID '{0}'.. /// internal static string UpdateProgress_TemplateRequired { get { return ResourceManager.GetString("UpdateProgress_TemplateRequired", resourceCulture); } } ////// Looks up a localized string similar to You must log on to call this method.. /// internal static string UserIsNotAuthenticated { get { return ResourceManager.GetString("UserIsNotAuthenticated", resourceCulture); } } ////// Looks up a localized string similar to Assembly '{0}' does not contain a Web resource with name '{1}'. Setting the ScriptReference.ScriptMode property to ScriptMode.Auto or ScriptMode.Release will cause the release script to be used.. /// internal static string WebResourceUtil_AssemblyDoesNotContainDebugWebResource { get { return ResourceManager.GetString("WebResourceUtil_AssemblyDoesNotContainDebugWebResource", resourceCulture); } } ////// Looks up a localized string similar to Assembly '{0}' contains a Web resource with name '{1}', but does not contain an embedded resource with name '{1}'.. /// internal static string WebResourceUtil_AssemblyDoesNotContainEmbeddedResource { get { return ResourceManager.GetString("WebResourceUtil_AssemblyDoesNotContainEmbeddedResource", resourceCulture); } } ////// Looks up a localized string similar to Assembly '{0}' does not contain a Web resource with name '{1}'.. /// internal static string WebResourceUtil_AssemblyDoesNotContainReleaseWebResource { get { return ResourceManager.GetString("WebResourceUtil_AssemblyDoesNotContainReleaseWebResource", resourceCulture); } } ////// Looks up a localized string similar to There was an error processing the request.. /// internal static string WebService_Error { get { return ResourceManager.GetString("WebService_Error", resourceCulture); } } ////// Looks up a localized string similar to Using the GenerateScriptTypes attribute is not supported for types in the following categories: primitive types; DateTime; generic types taking more than one parameter; types implementing IEnumerable or IDictionary; interfaces; Abstract classes; classes without a public default constructor.. /// internal static string WebService_InvalidGenerateScriptType { get { return ResourceManager.GetString("WebService_InvalidGenerateScriptType", resourceCulture); } } ////// Looks up a localized string similar to The path "{0}" is not supported. When InlineScript=true, the path should be a relative path pointing to the same web application as the current page.. /// internal static string WebService_InvalidInlineVirtualPath { get { return ResourceManager.GetString("WebService_InvalidInlineVirtualPath", resourceCulture); } } ////// Looks up a localized string similar to An attempt was made to call the method '{0}' using a {1} request, which is not allowed.. /// internal static string WebService_InvalidVerbRequest { get { return ResourceManager.GetString("WebService_InvalidVerbRequest", resourceCulture); } } ////// Looks up a localized string similar to Invalid web service call, expected path info of /js/<Method>.. /// internal static string WebService_InvalidWebServiceCall { get { return ResourceManager.GetString("WebService_InvalidWebServiceCall", resourceCulture); } } ////// Looks up a localized string similar to The method '{0}' returns a value of type '{1}', which cannot be serialized as Xml. Original error: {2}. /// internal static string WebService_InvalidXmlReturnType { get { return ResourceManager.GetString("WebService_InvalidXmlReturnType", resourceCulture); } } ////// Looks up a localized string similar to Invalid web service call, missing value for parameter: '{0}'.. /// internal static string WebService_MissingArg { get { return ResourceManager.GetString("WebService_MissingArg", resourceCulture); } } ////// Looks up a localized string similar to Only Web services with a [ScriptService] attribute on the class definition can be called from script.. /// internal static string WebService_NoScriptServiceAttribute { get { return ResourceManager.GetString("WebService_NoScriptServiceAttribute", resourceCulture); } } ////// Looks up a localized string similar to No web service found at: {0}.. /// internal static string WebService_NoWebServiceData { get { return ResourceManager.GetString("WebService_NoWebServiceData", resourceCulture); } } ////// Looks up a localized string similar to No web service found at: {0}. This error can occur if a ServiceReference to a WCF service has InlineScript set to 'true'. For WCF services InlineScript should be 'false'.. /// internal static string WebService_NoWebServiceDataInlineScript { get { return ResourceManager.GetString("WebService_NoWebServiceDataInlineScript", resourceCulture); } } ////// Looks up a localized string similar to Authentication failed.. /// internal static string WebService_RedirectError { get { return ResourceManager.GetString("WebService_RedirectError", resourceCulture); } } ////// Looks up a localized string similar to Unknown web method {0}.. /// internal static string WebService_UnknownWebMethod { get { return ResourceManager.GetString("WebService_UnknownWebMethod", resourceCulture); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
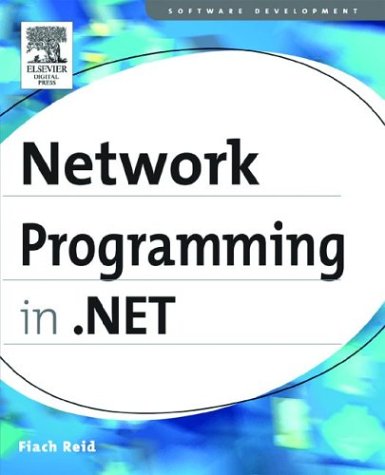
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TriggerCollection.cs
- XmlUnspecifiedAttribute.cs
- HandlerFactoryWrapper.cs
- Listbox.cs
- TrackingExtract.cs
- TypeDelegator.cs
- DataGridViewHitTestInfo.cs
- BindingContext.cs
- UrlPath.cs
- HttpDebugHandler.cs
- RuntimeCompatibilityAttribute.cs
- CellTreeNode.cs
- PenThread.cs
- BaseAppDomainProtocolHandler.cs
- cache.cs
- ServerIdentity.cs
- TransactionInterop.cs
- IfJoinedCondition.cs
- DataFormat.cs
- AssociationSetMetadata.cs
- CheckableControlBaseAdapter.cs
- IISMapPath.cs
- ProgressBar.cs
- ProfileBuildProvider.cs
- LocalizationCodeDomSerializer.cs
- XmlJsonReader.cs
- UdpMessageProperty.cs
- MessageQueueEnumerator.cs
- ServiceBehaviorAttribute.cs
- DBAsyncResult.cs
- ProfileSettingsCollection.cs
- TraceContextRecord.cs
- FtpWebRequest.cs
- Route.cs
- Renderer.cs
- PathSegmentCollection.cs
- PropertyChangedEventArgs.cs
- Helpers.cs
- Effect.cs
- XmlCDATASection.cs
- Int32Converter.cs
- WmfPlaceableFileHeader.cs
- PrivilegeNotHeldException.cs
- DataKeyArray.cs
- PhysicalFontFamily.cs
- ApplicationManager.cs
- TreeNodeStyle.cs
- PreviewPageInfo.cs
- ChameleonKey.cs
- AdRotator.cs
- FileDialog_Vista.cs
- AuthenticationException.cs
- FeatureSupport.cs
- DataGridParentRows.cs
- PropertyMetadata.cs
- EdmComplexPropertyAttribute.cs
- DriveInfo.cs
- LogicalMethodInfo.cs
- UnmanagedMemoryAccessor.cs
- KnownTypeAttribute.cs
- BulletDecorator.cs
- StreamHelper.cs
- SafeArrayRankMismatchException.cs
- ResourceDisplayNameAttribute.cs
- EventManager.cs
- SiblingIterators.cs
- NegationPusher.cs
- ProxyFragment.cs
- ACE.cs
- MgmtConfigurationRecord.cs
- WinCategoryAttribute.cs
- EditorBrowsableAttribute.cs
- SwitchAttribute.cs
- ControlBuilder.cs
- ButtonChrome.cs
- ValuePattern.cs
- DrawItemEvent.cs
- RepeaterItem.cs
- XamlInt32CollectionSerializer.cs
- EventSourceCreationData.cs
- AddInIpcChannel.cs
- ListViewPagedDataSource.cs
- DES.cs
- GZipObjectSerializer.cs
- CLRBindingWorker.cs
- StringBuilder.cs
- ToolStripMenuItem.cs
- DataControlField.cs
- ReadOnlyDictionary.cs
- RectAnimationBase.cs
- XmlAtomicValue.cs
- DataTableCollection.cs
- DataBoundLiteralControl.cs
- TableProviderWrapper.cs
- TaiwanLunisolarCalendar.cs
- SoapObjectReader.cs
- OutputCacheSection.cs
- StrokeCollectionDefaultValueFactory.cs
- MetadataHelper.cs
- DeclaredTypeValidator.cs