Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / EntitySqlException.cs / 1 / EntitySqlException.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- //#define CLEAN_INTERNALS_FROM_STACK namespace System.Data { using System; using System.IO; using System.Data.Common.EntitySql; using System.Globalization; using System.Runtime.Serialization; using System.Security.Permissions; using System.Diagnostics; using System.Text; ////// Represents an eSql Query compilation exception; /// The class of exceptional conditions that may cause this exception to be raised are mainly: /// 1) Syntax Errors: raised during query text parsing and when a given query does not conform to eSql formal grammar; /// 2) Semantic Errors: raised when semantic rules of eSql language are not met such as metadata or schema information /// not accurate or not present, type validation errors, scoping rule violations, user of undefined variables, etc. /// For more information, see eSql Language Spec. /// [Serializable] public sealed class EntitySqlException : EntityException { #region Private Fields ////// represents the full error message containing the error description, the error context if available, line /// and column number if available. /// private string _message; ////// error message description. /// private string _errorDescription; ////// information about the context where the error occurred /// private string _errorContext; ////// error line number /// private int _line; ////// error column number /// private int _column; #endregion #region Public Constructors ////// initializes a new instance of EntityException with the generic error message /// public EntitySqlException() : this(System.Data.Entity.Strings.GeneralQueryError) { HResult = HResults.InvalidQuery; } ////// initializes a new instance of EntityException with a given message /// /// public EntitySqlException( string message ) { _message = message; HResult = HResults.InvalidQuery; } ////// initializes a new instance of EntityException with a given message and innerException instance /// /// /// public EntitySqlException( string message, Exception innerException ) : base(message,innerException) { _message = message; HResult = HResults.InvalidQuery; } ////// initializes a new instance EntityException with a given SerializationInfo and StreamingContext /// /// /// private EntitySqlException( SerializationInfo serializationInfo, StreamingContext streamingContext ) : base(serializationInfo, streamingContext) { HResult = HResults.InvalidQuery; } #endregion #region Internal Constructors ////// initializes a new instance EntityException with an ErrorContext instance and a given error message /// internal static EntitySqlException Create(ErrorContext errCtx, string errorMessage, Exception innerException) { return EntitySqlException.Create(errCtx.QueryText, errorMessage, errCtx.InputPosition, errCtx.ErrorContextInfo, errCtx.UseContextInfoAsResourceIdentifier, innerException); } ////// initializes a new instance EntityException with contextual information to allow detailed error feedback /// internal static EntitySqlException Create( string queryText, string errorDescription, int errorPosition, string errorContextInfo, bool loadErrorContextFromResource, Exception innerException) { string errorContext; int line; int column; string errorMessage; if (loadErrorContextFromResource) { errorContext = (null != errorContextInfo) ? EntityRes.GetString(errorContextInfo) : String.Empty; } else { errorContext = errorContextInfo; } errorMessage = FormatQueryError(queryText, errorDescription, errorPosition, errorContext, out line, out column); return new EntitySqlException(errorMessage, errorDescription, errorContext, line, column, innerException); } ////// core constructor /// private EntitySqlException( string message, string errorDescription, string errorContext, int line, int column, Exception innerException ) : base(message,innerException) { _message = message; _errorDescription = errorDescription; _errorContext = errorContext; _line = line; _column = column; HResult = HResults.InvalidQuery; } #endregion #region Public Properties ////// Gets the full error message containing the error description, the error context if available, line /// and column numbers if available /// public override string Message { get { return _message ?? String.Empty; } } ////// Gets the error description explaining the reason why the query was not accepted or an empty String.Empty /// public string ErrorDescription { get { return _errorDescription ?? String.Empty; } } ////// Gets the aproximate context where the error occurred if available. /// public string ErrorContext { get { return _errorContext ?? String.Empty; } } ////// Returns the the aproximate line number where the error occurred /// public int Line { get { return _line; } } ////// Returns the the aproximate column number where the error occurred /// public int Column { get { return _column; } } #endregion #region Private helpers ////// formats error message adding query context to exception message /// /// original query text /// error message /// error position in the input stream /// optional additional information /// aproximate line number where the error occurred /// aproximate column number where the error occurred ///string error message in the format: error such and such[,near [additional information,] line ddd, column ddd]. private static string FormatQueryError( string queryText, string errorMessage, int errPos, string additionalInfo, out int lineNumber, out int columnNumber ) { lineNumber = columnNumber = 0; if (null == queryText) { return (errorMessage ?? String.Empty); } Debug.Assert(errPos > -1, "position in input stream cannot be < 0"); Debug.Assert(errPos <= queryText.Length, "position in input stream cannot be greater than query text size"); // // replace control chars and newLines for single representation characters // StringBuilder sb = new StringBuilder(queryText.Length); for (int i = 0; i < queryText.Length; i++) { Char c = queryText[i]; if (CqlLexer.IsNewLine(c)) { c = '\n'; } else if ((Char.IsControl(c) || Char.IsWhiteSpace(c)) && ('\r' != c)) { c = ' '; } sb.Append(c); } queryText = sb.ToString().TrimEnd(new char[] {'\n' }); // // now compute line and column // int colNum = errPos; int linNum = 1; string[] queryLines = queryText.Split(new char[] {'\n' }, StringSplitOptions.None); for(int i=0; i< queryLines.Length; i++) { if (colNum < queryLines[i].Length) { break; } colNum -= (queryLines[i].Length + 1); linNum++; } colNum++; // // Message format: error such and such[,near [additional information,] line ddd, column ddd]. // sb = new Text.StringBuilder(); sb.Append(errorMessage); bool wasNearTermUsed = false; if ( !String.IsNullOrEmpty(additionalInfo)) { wasNearTermUsed = true; sb.AppendFormat(CultureInfo.CurrentCulture, ", {0}", System.Data.Entity.Strings.LocalizedNear); sb.AppendFormat(CultureInfo.CurrentCulture, " {0}", additionalInfo); } if (0 < errPos) { if (wasNearTermUsed) { sb.Append(","); } else { sb.AppendFormat(CultureInfo.CurrentCulture, ", {0}", System.Data.Entity.Strings.LocalizedNear); } sb.AppendFormat(CultureInfo.CurrentCulture, " {0} {1}, {2} {3}", System.Data.Entity.Strings.LocalizedLine, linNum, System.Data.Entity.Strings.LocalizedColumn, colNum); } lineNumber = linNum; columnNumber = colNum; return sb.Append(".").ToString(); } #endregion #region ISerializable implementation ////// sets the System.Runtime.Serialization.SerializationInfo /// with information about the exception. /// /// The System.Runtime.Serialization.SerializationInfo that holds the serialized /// object data about the exception being thrown. /// /// [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData( SerializationInfo info, StreamingContext context ) { base.GetObjectData(info, context); info.AddValue("Message", _message); info.AddValue("ErrorDescription", _errorDescription); info.AddValue("ErrorContext", _errorContext); info.AddValue("Line", _line); info.AddValue("Column", _column); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- //#define CLEAN_INTERNALS_FROM_STACK namespace System.Data { using System; using System.IO; using System.Data.Common.EntitySql; using System.Globalization; using System.Runtime.Serialization; using System.Security.Permissions; using System.Diagnostics; using System.Text; ////// Represents an eSql Query compilation exception; /// The class of exceptional conditions that may cause this exception to be raised are mainly: /// 1) Syntax Errors: raised during query text parsing and when a given query does not conform to eSql formal grammar; /// 2) Semantic Errors: raised when semantic rules of eSql language are not met such as metadata or schema information /// not accurate or not present, type validation errors, scoping rule violations, user of undefined variables, etc. /// For more information, see eSql Language Spec. /// [Serializable] public sealed class EntitySqlException : EntityException { #region Private Fields ////// represents the full error message containing the error description, the error context if available, line /// and column number if available. /// private string _message; ////// error message description. /// private string _errorDescription; ////// information about the context where the error occurred /// private string _errorContext; ////// error line number /// private int _line; ////// error column number /// private int _column; #endregion #region Public Constructors ////// initializes a new instance of EntityException with the generic error message /// public EntitySqlException() : this(System.Data.Entity.Strings.GeneralQueryError) { HResult = HResults.InvalidQuery; } ////// initializes a new instance of EntityException with a given message /// /// public EntitySqlException( string message ) { _message = message; HResult = HResults.InvalidQuery; } ////// initializes a new instance of EntityException with a given message and innerException instance /// /// /// public EntitySqlException( string message, Exception innerException ) : base(message,innerException) { _message = message; HResult = HResults.InvalidQuery; } ////// initializes a new instance EntityException with a given SerializationInfo and StreamingContext /// /// /// private EntitySqlException( SerializationInfo serializationInfo, StreamingContext streamingContext ) : base(serializationInfo, streamingContext) { HResult = HResults.InvalidQuery; } #endregion #region Internal Constructors ////// initializes a new instance EntityException with an ErrorContext instance and a given error message /// internal static EntitySqlException Create(ErrorContext errCtx, string errorMessage, Exception innerException) { return EntitySqlException.Create(errCtx.QueryText, errorMessage, errCtx.InputPosition, errCtx.ErrorContextInfo, errCtx.UseContextInfoAsResourceIdentifier, innerException); } ////// initializes a new instance EntityException with contextual information to allow detailed error feedback /// internal static EntitySqlException Create( string queryText, string errorDescription, int errorPosition, string errorContextInfo, bool loadErrorContextFromResource, Exception innerException) { string errorContext; int line; int column; string errorMessage; if (loadErrorContextFromResource) { errorContext = (null != errorContextInfo) ? EntityRes.GetString(errorContextInfo) : String.Empty; } else { errorContext = errorContextInfo; } errorMessage = FormatQueryError(queryText, errorDescription, errorPosition, errorContext, out line, out column); return new EntitySqlException(errorMessage, errorDescription, errorContext, line, column, innerException); } ////// core constructor /// private EntitySqlException( string message, string errorDescription, string errorContext, int line, int column, Exception innerException ) : base(message,innerException) { _message = message; _errorDescription = errorDescription; _errorContext = errorContext; _line = line; _column = column; HResult = HResults.InvalidQuery; } #endregion #region Public Properties ////// Gets the full error message containing the error description, the error context if available, line /// and column numbers if available /// public override string Message { get { return _message ?? String.Empty; } } ////// Gets the error description explaining the reason why the query was not accepted or an empty String.Empty /// public string ErrorDescription { get { return _errorDescription ?? String.Empty; } } ////// Gets the aproximate context where the error occurred if available. /// public string ErrorContext { get { return _errorContext ?? String.Empty; } } ////// Returns the the aproximate line number where the error occurred /// public int Line { get { return _line; } } ////// Returns the the aproximate column number where the error occurred /// public int Column { get { return _column; } } #endregion #region Private helpers ////// formats error message adding query context to exception message /// /// original query text /// error message /// error position in the input stream /// optional additional information /// aproximate line number where the error occurred /// aproximate column number where the error occurred ///string error message in the format: error such and such[,near [additional information,] line ddd, column ddd]. private static string FormatQueryError( string queryText, string errorMessage, int errPos, string additionalInfo, out int lineNumber, out int columnNumber ) { lineNumber = columnNumber = 0; if (null == queryText) { return (errorMessage ?? String.Empty); } Debug.Assert(errPos > -1, "position in input stream cannot be < 0"); Debug.Assert(errPos <= queryText.Length, "position in input stream cannot be greater than query text size"); // // replace control chars and newLines for single representation characters // StringBuilder sb = new StringBuilder(queryText.Length); for (int i = 0; i < queryText.Length; i++) { Char c = queryText[i]; if (CqlLexer.IsNewLine(c)) { c = '\n'; } else if ((Char.IsControl(c) || Char.IsWhiteSpace(c)) && ('\r' != c)) { c = ' '; } sb.Append(c); } queryText = sb.ToString().TrimEnd(new char[] {'\n' }); // // now compute line and column // int colNum = errPos; int linNum = 1; string[] queryLines = queryText.Split(new char[] {'\n' }, StringSplitOptions.None); for(int i=0; i< queryLines.Length; i++) { if (colNum < queryLines[i].Length) { break; } colNum -= (queryLines[i].Length + 1); linNum++; } colNum++; // // Message format: error such and such[,near [additional information,] line ddd, column ddd]. // sb = new Text.StringBuilder(); sb.Append(errorMessage); bool wasNearTermUsed = false; if ( !String.IsNullOrEmpty(additionalInfo)) { wasNearTermUsed = true; sb.AppendFormat(CultureInfo.CurrentCulture, ", {0}", System.Data.Entity.Strings.LocalizedNear); sb.AppendFormat(CultureInfo.CurrentCulture, " {0}", additionalInfo); } if (0 < errPos) { if (wasNearTermUsed) { sb.Append(","); } else { sb.AppendFormat(CultureInfo.CurrentCulture, ", {0}", System.Data.Entity.Strings.LocalizedNear); } sb.AppendFormat(CultureInfo.CurrentCulture, " {0} {1}, {2} {3}", System.Data.Entity.Strings.LocalizedLine, linNum, System.Data.Entity.Strings.LocalizedColumn, colNum); } lineNumber = linNum; columnNumber = colNum; return sb.Append(".").ToString(); } #endregion #region ISerializable implementation ////// sets the System.Runtime.Serialization.SerializationInfo /// with information about the exception. /// /// The System.Runtime.Serialization.SerializationInfo that holds the serialized /// object data about the exception being thrown. /// /// [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData( SerializationInfo info, StreamingContext context ) { base.GetObjectData(info, context); info.AddValue("Message", _message); info.AddValue("ErrorDescription", _errorDescription); info.AddValue("ErrorContext", _errorContext); info.AddValue("Line", _line); info.AddValue("Column", _column); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
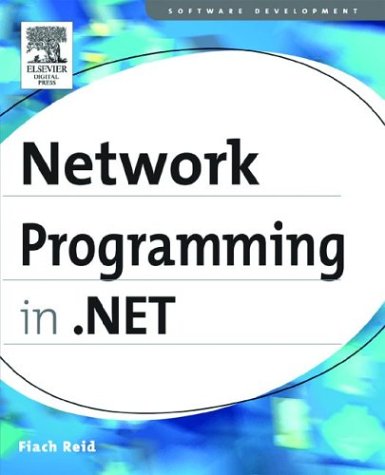
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpApplicationStateBase.cs
- BeginStoryboard.cs
- GroupJoinQueryOperator.cs
- LicenseManager.cs
- XmlSignatureManifest.cs
- RequestQueue.cs
- DataViewSetting.cs
- OdbcConnection.cs
- GetCardDetailsRequest.cs
- ComboBox.cs
- XmlDigitalSignatureProcessor.cs
- AnimationClock.cs
- ProviderBase.cs
- XmlSchemaCompilationSettings.cs
- SchemaMapping.cs
- MasterPageBuildProvider.cs
- DataGridViewComponentPropertyGridSite.cs
- CustomAttributeSerializer.cs
- CoordinationService.cs
- BadImageFormatException.cs
- DbQueryCommandTree.cs
- ColorTransform.cs
- MessageQueue.cs
- XmlSchemas.cs
- SelectionService.cs
- CancellationHandler.cs
- Crc32.cs
- ProfileManager.cs
- DocumentPageTextView.cs
- MetroSerializationManager.cs
- ExpandSegmentCollection.cs
- ScriptComponentDescriptor.cs
- InternalDispatchObject.cs
- EntityCommandDefinition.cs
- Context.cs
- SchemaCollectionPreprocessor.cs
- SamlConditions.cs
- RightsManagementInformation.cs
- SystemIPGlobalStatistics.cs
- SoapDocumentServiceAttribute.cs
- DataErrorValidationRule.cs
- ProtocolsConfiguration.cs
- Stopwatch.cs
- DelegateHelpers.Generated.cs
- CompilationUnit.cs
- ObjectSelectorEditor.cs
- DataShape.cs
- ErrorRuntimeConfig.cs
- InfoCardListRequest.cs
- LabelInfo.cs
- ItemsChangedEventArgs.cs
- XamlWriter.cs
- ViewBox.cs
- WorkflowDesignerMessageFilter.cs
- CodeStatement.cs
- TypographyProperties.cs
- translator.cs
- HyperLink.cs
- HtmlButton.cs
- ExtendedPropertiesHandler.cs
- ConstructorExpr.cs
- SecurityTokenAuthenticator.cs
- AutoResizedEvent.cs
- GlobalItem.cs
- DebugHandleTracker.cs
- EncodingNLS.cs
- RenamedEventArgs.cs
- CollectionBuilder.cs
- NamedObjectList.cs
- ScriptControlManager.cs
- ListItem.cs
- DataControlExtensions.cs
- FieldNameLookup.cs
- MultiPageTextView.cs
- FilteredAttributeCollection.cs
- RegistryExceptionHelper.cs
- SpeechDetectedEventArgs.cs
- ViewStateChangedEventArgs.cs
- ScriptDescriptor.cs
- WebHttpSecurityElement.cs
- OdbcRowUpdatingEvent.cs
- PropertyInformation.cs
- GroupQuery.cs
- MediaEntryAttribute.cs
- InvalidAsynchronousStateException.cs
- XslCompiledTransform.cs
- Point.cs
- ErrorInfoXmlDocument.cs
- ToolStripRenderEventArgs.cs
- XmlSchemaSimpleTypeUnion.cs
- Accessible.cs
- CodeGotoStatement.cs
- DecimalAnimationBase.cs
- PropertyRecord.cs
- ValueConversionAttribute.cs
- ApplicationManager.cs
- JpegBitmapDecoder.cs
- WorkflowServiceBehavior.cs
- CalendarDataBindingHandler.cs
- ButtonRenderer.cs