Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Enumerables / RangeEnumerable.cs / 1305376 / RangeEnumerable.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // RangeEnumerable.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; namespace System.Linq.Parallel { ////// A simple enumerable type that implements the range algorithm. It also supports /// partitioning of the indices by implementing an interface that PLINQ recognizes. /// internal class RangeEnumerable : ParallelQuery, IParallelPartitionable { private int m_from; // Lowest index to include. private int m_count; // Number of indices to include. //------------------------------------------------------------------------------------ // Constructs a new range enumerable object for the specified range. // internal RangeEnumerable(int from, int count) :base(QuerySettings.Empty) { // Transform the from and to indices into low and highs. m_from = from; m_count = count; } //----------------------------------------------------------------------------------- // Retrieves 'count' partitions, each of which uses a non-overlapping set of indices. // public QueryOperatorEnumerator [] GetPartitions(int partitionCount) { // Calculate a stride size, avoiding overflow if m_count is large int stride = m_count / partitionCount; int biggerPartitionCount = m_count % partitionCount; // Create individual partitions, carefully avoiding overflow int doneCount = 0; QueryOperatorEnumerator [] partitions = new QueryOperatorEnumerator [partitionCount]; for (int i = 0; i < partitionCount; i++) { int partitionSize = (i < biggerPartitionCount) ? stride + 1 : stride; partitions[i] = new RangeEnumerator( m_from + doneCount, partitionSize, doneCount); doneCount += partitionSize; } return partitions; } //----------------------------------------------------------------------------------- // Basic IEnumerator method implementations. // public override IEnumerator GetEnumerator() { return new RangeEnumerator(m_from, m_count, 0).AsClassicEnumerator(); } //----------------------------------------------------------------------------------- // The actual enumerator that walks over the specified range. // class RangeEnumerator : QueryOperatorEnumerator { private readonly int m_from; // The initial value. private readonly int m_count; // How many values to yield. private readonly int m_initialIndex; // The ordinal index of the first value in the range. private Shared m_currentCount; // The 0-based index of the current value. [allocate in moveNext to avoid false-sharing] //------------------------------------------------------------------------------------ // Creates a new enumerator. // internal RangeEnumerator(int from, int count, int initialIndex) { m_from = from; m_count = count; m_initialIndex = initialIndex; } //----------------------------------------------------------------------------------- // Basic enumeration method. This implements the logic to walk the desired // range, using the step specified at construction time. // internal override bool MoveNext(ref int currentElement, ref int currentKey) { if( m_currentCount == null) m_currentCount = new Shared (-1); // Calculate the next index and ensure it falls within our range. int nextCount = m_currentCount.Value + 1; if (nextCount < m_count) { m_currentCount.Value = nextCount; currentElement = nextCount + m_from; currentKey = nextCount + m_initialIndex; return true; } return false; } internal override void Reset() { // We set the current value such that the next addition of step // results in the 1st real value in the range. m_currentCount = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
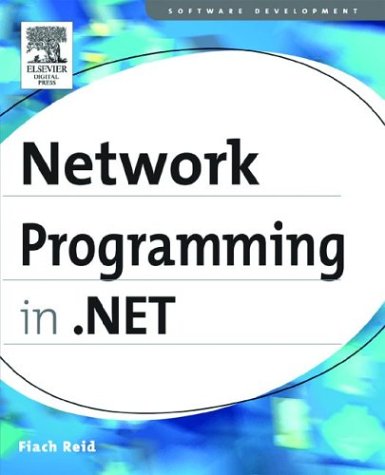
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewGroupConverter.cs
- PackagingUtilities.cs
- NetPipeSection.cs
- ContentPlaceHolder.cs
- DynamicPropertyHolder.cs
- ReferentialConstraint.cs
- OraclePermissionAttribute.cs
- WindowsAuthenticationEventArgs.cs
- MimeTypePropertyAttribute.cs
- HandleTable.cs
- Method.cs
- SafeArchiveContext.cs
- ListSourceHelper.cs
- WebBrowserContainer.cs
- ComEventsMethod.cs
- HtmlDocument.cs
- ConfigurationSettings.cs
- PropertyPath.cs
- TextClipboardData.cs
- XmlQueryRuntime.cs
- CodeSpit.cs
- SharedUtils.cs
- ServiceHostFactory.cs
- ErrorFormatterPage.cs
- Random.cs
- Underline.cs
- CodeGenHelper.cs
- CompensationHandlingFilter.cs
- TransportContext.cs
- XmlSchema.cs
- TogglePatternIdentifiers.cs
- EventSinkHelperWriter.cs
- _ProxyRegBlob.cs
- OdbcException.cs
- FlowDocumentScrollViewer.cs
- ThreadAbortException.cs
- OrderedDictionary.cs
- XmlDocumentFragment.cs
- UnsafeNativeMethods.cs
- HostedImpersonationContext.cs
- DescendantQuery.cs
- linebase.cs
- MarkupCompilePass2.cs
- XmlSchemaAnnotated.cs
- TranslateTransform3D.cs
- EntityDataSourceEntityTypeFilterItem.cs
- CatalogZoneBase.cs
- DrawingImage.cs
- PersistenceParticipant.cs
- SchemaImporter.cs
- DateTimeConverter.cs
- ImageCreator.cs
- SchemaElementDecl.cs
- SegmentInfo.cs
- RawStylusActions.cs
- FileAccessException.cs
- EntityParameter.cs
- Semaphore.cs
- QuaternionValueSerializer.cs
- FontInfo.cs
- WebBrowserSiteBase.cs
- HtmlEncodedRawTextWriter.cs
- CustomPeerResolverService.cs
- JobInputBins.cs
- CroppedBitmap.cs
- AbstractDataSvcMapFileLoader.cs
- StyleCollectionEditor.cs
- BezierSegment.cs
- QilInvokeEarlyBound.cs
- DataControlLinkButton.cs
- SmiEventSink_Default.cs
- PresentationAppDomainManager.cs
- OracleParameterCollection.cs
- FilterRepeater.cs
- ToolStripItem.cs
- PrinterSettings.cs
- WpfSharedBamlSchemaContext.cs
- SqlDataSourceCommandEventArgs.cs
- TokenizerHelper.cs
- SqlError.cs
- TrackingProfileSerializer.cs
- BigInt.cs
- NetTcpSecurity.cs
- TextPointerBase.cs
- HtmlInputFile.cs
- ManipulationVelocities.cs
- GridErrorDlg.cs
- TextChangedEventArgs.cs
- JournalEntryStack.cs
- InkCanvasSelectionAdorner.cs
- TabRenderer.cs
- TypeBrowserDialog.cs
- AudioException.cs
- ThrowOnMultipleAssignment.cs
- InheritedPropertyChangedEventArgs.cs
- CellPartitioner.cs
- JavaScriptObjectDeserializer.cs
- ReferenceList.cs
- StringComparer.cs
- Point3DIndependentAnimationStorage.cs