Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CellPartitioner.cs / 1 / CellPartitioner.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.Structures; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.Validation; using System.Text; using System.Data.Mapping.Update.Internal; using System.Collections.ObjectModel; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration { using CellGroup = Set; // This class is responsible for partitioning cells into groups of cells // that are related and for which view generation needs to be done together internal class CellPartitioner : InternalBase { #region Constructor // effects: Creates a partitioner for cells with extra information // about foreign key constraints internal CellPartitioner(IEnumerable | cells, IEnumerable | foreignKeyConstraints) { m_foreignKeyConstraints = foreignKeyConstraints; m_cells = cells; } #endregion #region Fields private IEnumerable m_cells; private IEnumerable | m_foreignKeyConstraints; #endregion #region Available Methods // effects: Given a list of cells, segments them into multiple // "groups" such that view generation (including validation) of one // group can be done independently of another group. Returns the // groups as a list (uses the foreign key information as well) internal List GroupRelatedCells() { // If two cells share the same C or S, we place them in the same group // For each cell, determine the Cis and Sis that it refers // to. For every Ci (Si), keep track of the cells that Ci is // contained in. At the end, run through the Cis and Sis and do a // "connected components" algorithm to determine partitions // Now form a graph between different cells -- then compute the connected // components in it UndirectedGraph graph = new UndirectedGraph | (EqualityComparer | .Default); List | alreadyAddedCells = new List | (); // For each extent, add an edge between it and all previously // added extents with which it overlaps foreach (Cell cell in m_cells) { graph.AddVertex(cell); // Add an edge from this cell to the already added cells EntitySetBase firstCExtent = cell.CQuery.Extent; EntitySetBase firstSExtent = cell.SQuery.Extent; foreach (Cell existingCell in alreadyAddedCells) { EntitySetBase secondCExtent = existingCell.CQuery.Extent; EntitySetBase secondSExtent = existingCell.SQuery.Extent; // Add an edge between cell and existingCell if // * They have the same C or S extent // * They are linked via a foreign key between the S extents // * They are linked via a relationship bool sameExtent = secondCExtent.Equals(firstCExtent) || secondSExtent.Equals(firstSExtent); bool linkViaForeignKey = OverlapViaForeignKeys(cell, existingCell); bool linkViaRelationship = AreCellsConnectedViaRelationship(cell, existingCell); if (sameExtent || linkViaForeignKey || linkViaRelationship) { graph.AddEdge(existingCell, cell); } } alreadyAddedCells.Add(cell); } // Now determine the connected components of this graph List | result = GenerateConnectedComponents(graph); return result; } #endregion #region Private Methods // effects: Returns true iff cell1 is an extent at the end of cell2's // relationship set or vice versa private static bool AreCellsConnectedViaRelationship(Cell cell1, Cell cell2) { AssociationSet cRelationSet1 = cell1.CQuery.Extent as AssociationSet; AssociationSet cRelationSet2 = cell2.CQuery.Extent as AssociationSet; if (cRelationSet1 != null && MetadataHelper.IsExtentAtSomeRelationshipEnd(cRelationSet1, cell2.CQuery.Extent)) { return true; } if (cRelationSet2 != null && MetadataHelper.IsExtentAtSomeRelationshipEnd(cRelationSet2, cell1.CQuery.Extent)) { return true; } return false; } // effects: Given a graph of cell groups, returns a list of cellgroup // such that each cellgroup contains all the cells that are in the // same connected component private static List GenerateConnectedComponents(UndirectedGraph graph) { KeyToListMap | groupMap = graph.GenerateConnectedComponents(); // Run through the list of groups and generate the merged groups List result = new List (); foreach (int setNum in groupMap.Keys) { ReadOnlyCollection cellsInComponent = groupMap.ListForKey(setNum); CellGroup component = new CellGroup(cellsInComponent); result.Add(component); } return result; } // effects: Returns true iff there is a foreign key constraint // between cell1 and cell2's S extents private bool OverlapViaForeignKeys(Cell cell1, Cell cell2) { EntitySetBase sExtent1 = cell1.SQuery.Extent; EntitySetBase sExtent2 = cell2.SQuery.Extent; foreach (ForeignConstraint constraint in m_foreignKeyConstraints) { if (sExtent1.Equals(constraint.ParentTable) && sExtent2.Equals(constraint.ChildTable) || sExtent2.Equals(constraint.ParentTable) && sExtent1.Equals(constraint.ChildTable)) { return true; } } return false; } #endregion internal override void ToCompactString(StringBuilder builder) { Cell.CellsToBuilder(builder, m_cells); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // | // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.Structures; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.Validation; using System.Text; using System.Data.Mapping.Update.Internal; using System.Collections.ObjectModel; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration { using CellGroup = Set; // This class is responsible for partitioning cells into groups of cells // that are related and for which view generation needs to be done together internal class CellPartitioner : InternalBase { #region Constructor // effects: Creates a partitioner for cells with extra information // about foreign key constraints internal CellPartitioner(IEnumerable | cells, IEnumerable | foreignKeyConstraints) { m_foreignKeyConstraints = foreignKeyConstraints; m_cells = cells; } #endregion #region Fields private IEnumerable m_cells; private IEnumerable | m_foreignKeyConstraints; #endregion #region Available Methods // effects: Given a list of cells, segments them into multiple // "groups" such that view generation (including validation) of one // group can be done independently of another group. Returns the // groups as a list (uses the foreign key information as well) internal List GroupRelatedCells() { // If two cells share the same C or S, we place them in the same group // For each cell, determine the Cis and Sis that it refers // to. For every Ci (Si), keep track of the cells that Ci is // contained in. At the end, run through the Cis and Sis and do a // "connected components" algorithm to determine partitions // Now form a graph between different cells -- then compute the connected // components in it UndirectedGraph graph = new UndirectedGraph | (EqualityComparer | .Default); List | alreadyAddedCells = new List | (); // For each extent, add an edge between it and all previously // added extents with which it overlaps foreach (Cell cell in m_cells) { graph.AddVertex(cell); // Add an edge from this cell to the already added cells EntitySetBase firstCExtent = cell.CQuery.Extent; EntitySetBase firstSExtent = cell.SQuery.Extent; foreach (Cell existingCell in alreadyAddedCells) { EntitySetBase secondCExtent = existingCell.CQuery.Extent; EntitySetBase secondSExtent = existingCell.SQuery.Extent; // Add an edge between cell and existingCell if // * They have the same C or S extent // * They are linked via a foreign key between the S extents // * They are linked via a relationship bool sameExtent = secondCExtent.Equals(firstCExtent) || secondSExtent.Equals(firstSExtent); bool linkViaForeignKey = OverlapViaForeignKeys(cell, existingCell); bool linkViaRelationship = AreCellsConnectedViaRelationship(cell, existingCell); if (sameExtent || linkViaForeignKey || linkViaRelationship) { graph.AddEdge(existingCell, cell); } } alreadyAddedCells.Add(cell); } // Now determine the connected components of this graph List | result = GenerateConnectedComponents(graph); return result; } #endregion #region Private Methods // effects: Returns true iff cell1 is an extent at the end of cell2's // relationship set or vice versa private static bool AreCellsConnectedViaRelationship(Cell cell1, Cell cell2) { AssociationSet cRelationSet1 = cell1.CQuery.Extent as AssociationSet; AssociationSet cRelationSet2 = cell2.CQuery.Extent as AssociationSet; if (cRelationSet1 != null && MetadataHelper.IsExtentAtSomeRelationshipEnd(cRelationSet1, cell2.CQuery.Extent)) { return true; } if (cRelationSet2 != null && MetadataHelper.IsExtentAtSomeRelationshipEnd(cRelationSet2, cell1.CQuery.Extent)) { return true; } return false; } // effects: Given a graph of cell groups, returns a list of cellgroup // such that each cellgroup contains all the cells that are in the // same connected component private static List GenerateConnectedComponents(UndirectedGraph graph) { KeyToListMap | groupMap = graph.GenerateConnectedComponents(); // Run through the list of groups and generate the merged groups List result = new List (); foreach (int setNum in groupMap.Keys) { ReadOnlyCollection cellsInComponent = groupMap.ListForKey(setNum); CellGroup component = new CellGroup(cellsInComponent); result.Add(component); } return result; } // effects: Returns true iff there is a foreign key constraint // between cell1 and cell2's S extents private bool OverlapViaForeignKeys(Cell cell1, Cell cell2) { EntitySetBase sExtent1 = cell1.SQuery.Extent; EntitySetBase sExtent2 = cell2.SQuery.Extent; foreach (ForeignConstraint constraint in m_foreignKeyConstraints) { if (sExtent1.Equals(constraint.ParentTable) && sExtent2.Equals(constraint.ChildTable) || sExtent2.Equals(constraint.ParentTable) && sExtent1.Equals(constraint.ChildTable)) { return true; } } return false; } #endregion internal override void ToCompactString(StringBuilder builder) { Cell.CellsToBuilder(builder, m_cells); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. |
Link Menu
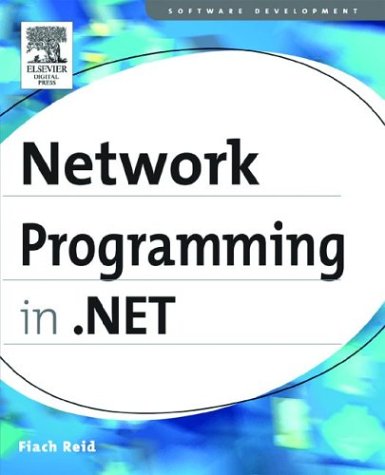
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CultureTable.cs
- TrustVersion.cs
- HttpPostedFileWrapper.cs
- TdsValueSetter.cs
- Encoding.cs
- InputProcessorProfiles.cs
- errorpatternmatcher.cs
- DesignRelationCollection.cs
- Hashtable.cs
- ModulesEntry.cs
- Ipv6Element.cs
- FilterableData.cs
- WebPartDisplayModeCancelEventArgs.cs
- PenThread.cs
- TargetFrameworkUtil.cs
- DataChangedEventManager.cs
- IxmlLineInfo.cs
- Page.cs
- ZoneMembershipCondition.cs
- login.cs
- DataFieldConverter.cs
- WebControlAdapter.cs
- PolyBezierSegmentFigureLogic.cs
- WindowsFormsHostPropertyMap.cs
- RemotingException.cs
- TimelineCollection.cs
- SQLByteStorage.cs
- Int16.cs
- Literal.cs
- WindowsEditBox.cs
- DocumentGridContextMenu.cs
- AsyncOperation.cs
- MessageFormatterConverter.cs
- Animatable.cs
- RuntimeConfigurationRecord.cs
- PageParser.cs
- LocalizationComments.cs
- MessageSecurityProtocol.cs
- PostBackTrigger.cs
- CodeMemberEvent.cs
- UnsafeNativeMethods.cs
- ServiceDebugBehavior.cs
- COM2PropertyBuilderUITypeEditor.cs
- IsolatedStorageFile.cs
- EventBuilder.cs
- UrlMapping.cs
- ScrollProviderWrapper.cs
- Model3DGroup.cs
- TableAutomationPeer.cs
- DecimalStorage.cs
- WriteableBitmap.cs
- ArgumentValidation.cs
- SharedStatics.cs
- HorizontalAlignConverter.cs
- DataStorage.cs
- FixedBufferAttribute.cs
- ByteStack.cs
- ManualResetEvent.cs
- Bidi.cs
- CompiledRegexRunner.cs
- RightsManagementEncryptedStream.cs
- BaseConfigurationRecord.cs
- ProxyGenerator.cs
- COM2ExtendedUITypeEditor.cs
- CodeThrowExceptionStatement.cs
- FullTextLine.cs
- BinarySerializer.cs
- StorageSetMapping.cs
- BookmarkUndoUnit.cs
- SqlNamer.cs
- BitVector32.cs
- SqlRemoveConstantOrderBy.cs
- InfoCardKeyedHashAlgorithm.cs
- FontNamesConverter.cs
- MasterPage.cs
- OledbConnectionStringbuilder.cs
- UIPermission.cs
- ConfigXmlElement.cs
- InputLanguageManager.cs
- Trigger.cs
- SelfIssuedTokenFactoryCredential.cs
- InertiaRotationBehavior.cs
- SymLanguageVendor.cs
- XPathException.cs
- SatelliteContractVersionAttribute.cs
- SafeNativeMemoryHandle.cs
- FileAccessException.cs
- PolyBezierSegment.cs
- ContainerFilterService.cs
- TransactionFlowBindingElement.cs
- UriScheme.cs
- ClockGroup.cs
- TableLayoutStyleCollection.cs
- RTTrackingProfile.cs
- MediaElementAutomationPeer.cs
- GroupQuery.cs
- SystemIcons.cs
- ProjectionCamera.cs
- FactoryGenerator.cs
- ClientRuntimeConfig.cs