Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Reflection / Emit / EventBuilder.cs / 1 / EventBuilder.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: EventBuilder ** ** ** Eventbuilder is for client to define eevnts for a class ** ** ===========================================================*/ namespace System.Reflection.Emit { using System; using System.Reflection; using System.Security.Permissions; using System.Runtime.InteropServices; // // A EventBuilder is always associated with a TypeBuilder. The TypeBuilder.DefineEvent // method will return a new EventBuilder to a client. // [HostProtection(MayLeakOnAbort = true)] [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_EventBuilder))] [System.Runtime.InteropServices.ComVisible(true)] public sealed class EventBuilder : _EventBuilder { // Make a private constructor so these cannot be constructed externally. private EventBuilder() {} // Constructs a EventBuilder. // internal EventBuilder( Module mod, // the module containing this EventBuilder String name, // Event name EventAttributes attr, // event attribute such as Public, Private, and Protected defined above int eventType, // event type TypeBuilder type, // containing type EventToken evToken) { m_name = name; m_module = mod; m_attributes = attr; m_evToken = evToken; m_type = type; } // Return the Token for this event within the TypeBuilder that the // event is defined within. public EventToken GetEventToken() { return m_evToken; } public void SetAddOnMethod(MethodBuilder mdBuilder) { if (mdBuilder == null) { throw new ArgumentNullException("mdBuilder"); } m_type.ThrowIfCreated(); TypeBuilder.InternalDefineMethodSemantics( m_module, m_evToken.Token, MethodSemanticsAttributes.AddOn, mdBuilder.GetToken().Token); } public void SetRemoveOnMethod(MethodBuilder mdBuilder) { if (mdBuilder == null) { throw new ArgumentNullException("mdBuilder"); } m_type.ThrowIfCreated(); TypeBuilder.InternalDefineMethodSemantics( m_module, m_evToken.Token, MethodSemanticsAttributes.RemoveOn, mdBuilder.GetToken().Token); } public void SetRaiseMethod(MethodBuilder mdBuilder) { if (mdBuilder == null) { throw new ArgumentNullException("mdBuilder"); } m_type.ThrowIfCreated(); TypeBuilder.InternalDefineMethodSemantics( m_module, m_evToken.Token, MethodSemanticsAttributes.Fire, mdBuilder.GetToken().Token); } public void AddOtherMethod(MethodBuilder mdBuilder) { if (mdBuilder == null) { throw new ArgumentNullException("mdBuilder"); } m_type.ThrowIfCreated(); TypeBuilder.InternalDefineMethodSemantics( m_module, m_evToken.Token, MethodSemanticsAttributes.Other, mdBuilder.GetToken().Token); } // Use this function if client decides to form the custom attribute blob themselves [System.Runtime.InteropServices.ComVisible(true)] public void SetCustomAttribute(ConstructorInfo con, byte[] binaryAttribute) { if (con == null) throw new ArgumentNullException("con"); if (binaryAttribute == null) throw new ArgumentNullException("binaryAttribute"); m_type.ThrowIfCreated(); TypeBuilder.InternalCreateCustomAttribute( m_evToken.Token, ((ModuleBuilder )m_module).GetConstructorToken(con).Token, binaryAttribute, m_module, false); } // Use this function if client wishes to build CustomAttribute using CustomAttributeBuilder public void SetCustomAttribute(CustomAttributeBuilder customBuilder) { if (customBuilder == null) { throw new ArgumentNullException("customBuilder"); } m_type.ThrowIfCreated(); customBuilder.CreateCustomAttribute((ModuleBuilder)m_module, m_evToken.Token); } void _EventBuilder.GetTypeInfoCount(out uint pcTInfo) { throw new NotImplementedException(); } void _EventBuilder.GetTypeInfo(uint iTInfo, uint lcid, IntPtr ppTInfo) { throw new NotImplementedException(); } void _EventBuilder.GetIDsOfNames([In] ref Guid riid, IntPtr rgszNames, uint cNames, uint lcid, IntPtr rgDispId) { throw new NotImplementedException(); } void _EventBuilder.Invoke(uint dispIdMember, [In] ref Guid riid, uint lcid, short wFlags, IntPtr pDispParams, IntPtr pVarResult, IntPtr pExcepInfo, IntPtr puArgErr) { throw new NotImplementedException(); } // These are package private so that TypeBuilder can access them. private String m_name; // The name of the event private EventToken m_evToken; // The token of this event private Module m_module; private EventAttributes m_attributes; private TypeBuilder m_type; } }
Link Menu
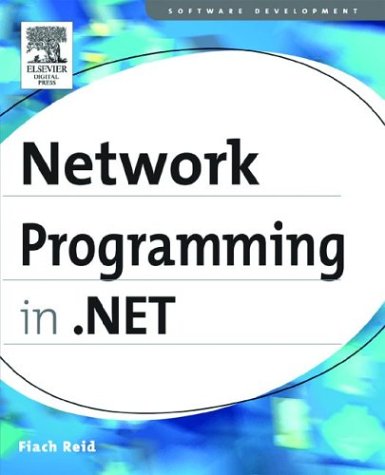
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- M3DUtil.cs
- FontEmbeddingManager.cs
- XmlNamedNodeMap.cs
- COM2ColorConverter.cs
- KeyFrames.cs
- QueryPageSettingsEventArgs.cs
- DataGridViewCellStyleChangedEventArgs.cs
- ProjectionPlan.cs
- TagMapCollection.cs
- Activity.cs
- KeyManager.cs
- TimeZoneInfo.cs
- SingleAnimation.cs
- PrefixQName.cs
- ParallelDesigner.cs
- ShapingWorkspace.cs
- RenderData.cs
- Accessors.cs
- WindowsListViewItemCheckBox.cs
- QuadraticBezierSegment.cs
- PngBitmapDecoder.cs
- MenuItemBindingCollection.cs
- ChildrenQuery.cs
- MimeWriter.cs
- ManagedFilter.cs
- CfgSemanticTag.cs
- SchemaExporter.cs
- HtmlSelect.cs
- GroupItemAutomationPeer.cs
- TextModifier.cs
- FlowDocumentFormatter.cs
- DeferredRunTextReference.cs
- LayoutEditorPart.cs
- DbProviderConfigurationHandler.cs
- BaseParagraph.cs
- UniformGrid.cs
- Encoding.cs
- UnsafeNativeMethodsPenimc.cs
- SettingsPropertyCollection.cs
- StringCollection.cs
- DbProviderFactories.cs
- DiffuseMaterial.cs
- KeyedHashAlgorithm.cs
- GlyphRunDrawing.cs
- HTTPNotFoundHandler.cs
- ColorInterpolationModeValidation.cs
- XmlArrayItemAttributes.cs
- BaseAppDomainProtocolHandler.cs
- ConfigUtil.cs
- TraceContext.cs
- StatusBarItem.cs
- objectresult_tresulttype.cs
- EntityWrapperFactory.cs
- DeclarativeCatalogPart.cs
- ChannelManagerBase.cs
- HttpHostedTransportConfiguration.cs
- namescope.cs
- TableRow.cs
- HtmlHistory.cs
- IisTraceListener.cs
- RemotingServices.cs
- Exception.cs
- DataGridAddNewRow.cs
- DataKey.cs
- StateDesigner.Helpers.cs
- XsltContext.cs
- HostedTransportConfigurationBase.cs
- MetaData.cs
- SeverityFilter.cs
- ellipse.cs
- CodeCatchClauseCollection.cs
- ProgressBar.cs
- RuleProcessor.cs
- Viewport3DAutomationPeer.cs
- GeneralTransform2DTo3DTo2D.cs
- AmbientLight.cs
- ObjectCloneHelper.cs
- TextServicesDisplayAttribute.cs
- PageOrientation.cs
- FormatSettings.cs
- MetadataSet.cs
- ToolStripSplitButton.cs
- StringInfo.cs
- GridViewSelectEventArgs.cs
- XhtmlMobileTextWriter.cs
- oledbmetadatacollectionnames.cs
- StructuredType.cs
- ApplicationContext.cs
- AsymmetricKeyExchangeDeformatter.cs
- VectorCollectionValueSerializer.cs
- GridViewRow.cs
- Drawing.cs
- XmlQuerySequence.cs
- ISCIIEncoding.cs
- BufferedOutputStream.cs
- X509ClientCertificateCredentialsElement.cs
- TreeNodeBindingCollection.cs
- DiscardableAttribute.cs
- WebBrowserNavigatingEventHandler.cs
- PerformanceCounterPermissionEntry.cs