Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / TextServicesDisplayAttribute.cs / 1305600 / TextServicesDisplayAttribute.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextServicesDisplayAttribute // // History: // 08/01/2003 : yutakas - Ported from dotnet tree. // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using System.Windows.Documents; using MS.Win32; using MS.Internal; using System; namespace System.Windows.Documents { //----------------------------------------------------- // // TextServicesDisplayAttribute class // //----------------------------------------------------- ////// The helper class to wrap TF_DISPLAYATTRIBUTE. /// internal class TextServicesDisplayAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- internal TextServicesDisplayAttribute(UnsafeNativeMethods.TF_DISPLAYATTRIBUTE attr) { _attr = attr; } //------------------------------------------------------ // // Internal Method // //------------------------------------------------------ ////// Check if this is empty. /// internal bool IsEmptyAttribute() { if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crLine.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) return false; return true; } ////// Apply the display attribute to to the given range. /// internal void Apply(ITextPointer start, ITextPointer end) { // // #if NOT_YET if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) { } #endif } ////// Convert TF_DA_COLOR to Color. /// internal static Color GetColor(UnsafeNativeMethods.TF_DA_COLOR dacolor, ITextPointer position) { if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_SYSCOLOR) { return GetSystemColor(dacolor.indexOrColorRef); } else if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_COLORREF) { int color = dacolor.indexOrColorRef; uint argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb & 0xff000000) >> 24), (byte)((argb & 0x00ff0000) >> 16), (byte)((argb & 0x0000ff00) >> 8), (byte)(argb & 0x000000ff)); } Invariant.Assert(position != null, "position can't be null"); return ((SolidColorBrush)position.GetValue(TextElement.ForegroundProperty)).Color; } ////// Line color of the composition line draw /// internal Color GetLineColor(ITextPointer position) { return GetColor(_attr.crLine, position); } ////// Line style of the composition line draw /// internal UnsafeNativeMethods.TF_DA_LINESTYLE LineStyle { get { return _attr.lsStyle; } } ////// Line bold of the composition line draw /// internal bool IsBoldLine { get { return _attr.fBoldLine; } } /** * Shift count and bit mask for A, R, G, B components */ private const int AlphaShift = 24; private const int RedShift = 16; private const int GreenShift = 8; private const int BlueShift = 0; private const int Win32RedShift = 0; private const int Win32GreenShift = 8; private const int Win32BlueShift = 16; private static int Encode(int alpha, int red, int green, int blue) { return red << RedShift | green << GreenShift | blue << BlueShift | alpha << AlphaShift; } private static int FromWin32Value(int value) { return Encode(255, (value >> Win32RedShift) & 0xFF, (value >> Win32GreenShift) & 0xFF, (value >> Win32BlueShift) & 0xFF); } ////// Query for system colors. /// /// Same parameter as Win32's GetSysColor ///The system color. private static Color GetSystemColor(int index) { uint argb; int color = SafeNativeMethods.GetSysColor(index); argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } //----------------------------------------------------- // // Private Field // //------------------------------------------------------ private UnsafeNativeMethods.TF_DISPLAYATTRIBUTE _attr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
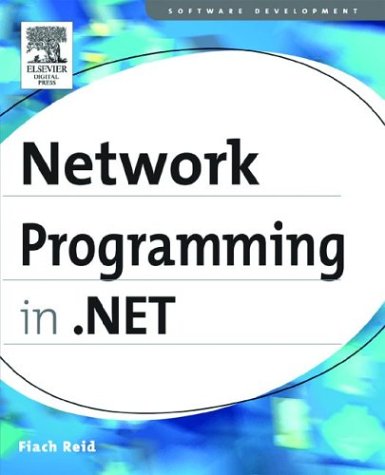
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _CommandStream.cs
- TdsParserSafeHandles.cs
- HttpConfigurationContext.cs
- SettingsSection.cs
- ScrollBarRenderer.cs
- Aes.cs
- SiteIdentityPermission.cs
- ValidationPropertyAttribute.cs
- BulletedList.cs
- MatchAttribute.cs
- CallContext.cs
- InternalResources.cs
- CompilationRelaxations.cs
- FixedTextSelectionProcessor.cs
- Brush.cs
- FontDifferentiator.cs
- FontFamilyConverter.cs
- ComponentEditorPage.cs
- SplitterCancelEvent.cs
- SoapServerProtocol.cs
- DesignerSelectionListAdapter.cs
- DrawingImage.cs
- ObjectListCommandsPage.cs
- TextDecorationCollection.cs
- DropTarget.cs
- PartialCachingControl.cs
- Scripts.cs
- CollectionBase.cs
- AndCondition.cs
- CheckBox.cs
- DataGridRow.cs
- RegexGroup.cs
- FormViewPagerRow.cs
- ValidationService.cs
- DataGridCellInfo.cs
- Expression.cs
- NotFiniteNumberException.cs
- GlyphInfoList.cs
- CustomAttribute.cs
- HostDesigntimeLicenseContext.cs
- DataGridViewCellParsingEventArgs.cs
- ConstrainedDataObject.cs
- IsolationInterop.cs
- BamlRecordHelper.cs
- PageAsyncTask.cs
- NameSpaceEvent.cs
- FileUtil.cs
- SessionState.cs
- ForeignKeyFactory.cs
- XmlRootAttribute.cs
- CompositeActivityMarkupSerializer.cs
- XmlEnumAttribute.cs
- XmlElementCollection.cs
- ListComponentEditorPage.cs
- RSAPKCS1SignatureFormatter.cs
- Keywords.cs
- XmlTextAttribute.cs
- WebServiceEnumData.cs
- HtmlContainerControl.cs
- BufferAllocator.cs
- DefaultObjectMappingItemCollection.cs
- safelink.cs
- MaterialCollection.cs
- FontCacheLogic.cs
- regiisutil.cs
- PolicyStatement.cs
- ActivityPreviewDesigner.cs
- Vector3DKeyFrameCollection.cs
- BoundColumn.cs
- TransformerConfigurationWizardBase.cs
- ObjectTypeMapping.cs
- ClientRolePrincipal.cs
- ToolboxItemSnapLineBehavior.cs
- CheckedPointers.cs
- BitmapMetadataEnumerator.cs
- CrossAppDomainChannel.cs
- XmlEntity.cs
- DataGridCell.cs
- ValueProviderWrapper.cs
- HttpCapabilitiesEvaluator.cs
- ControlIdConverter.cs
- HtmlCalendarAdapter.cs
- RealizationContext.cs
- SplitterCancelEvent.cs
- XmlNamespaceMappingCollection.cs
- IsolationInterop.cs
- _Win32.cs
- AutomationPatternInfo.cs
- MultipleViewProviderWrapper.cs
- ConfigXmlWhitespace.cs
- InkCollectionBehavior.cs
- ConsumerConnectionPointCollection.cs
- SolidColorBrush.cs
- TypeExtensionConverter.cs
- ChannelManager.cs
- TlsnegoTokenProvider.cs
- ClientTarget.cs
- WebSysDescriptionAttribute.cs
- ProcessInputEventArgs.cs
- ReadOnlyCollectionBase.cs