Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Drawing.cs / 1 / Drawing.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Drawing is the base Drawing class that defines the standard // interface which Drawing's must implement. // // History: // 2003/07/16 : adsmith - Created it. // 2003/07/28 : adsmith - Renamed it and hooked it up to the unmanaged code. // 2004/11/17 : timothyc - Repurposed Drawing from being a Changeable // implementation that contains render data into the base // class for the enumerable & modifiable Drawing subclasses. // 2005/01/26 : timothyc - Made Drawing abstract & removed legacy Drawing // support, including .Open() & IAddChild. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Resources; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Markup; using System.Windows.Threading; namespace System.Windows.Media { ////// Base class for the enumerable and modifiable Drawing subclasses. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public abstract partial class Drawing : Animatable, IDrawingContent, DUCE.IResource { #region Constructors ////// Constructor for Drawing /// ////// This constructor is internal to prevent external subclassing. /// internal Drawing() { } #endregion Constructors #region Public Properties ////// Bounds - the axis-aligned bounds of this Drawing. /// ////// Rect - the axis-aligned bounds of this Drawing. /// public Rect Bounds { get { ReadPreamble(); return GetBounds(); } } #endregion Public Properties #region Internal Methods ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { return true; } ////// Called when an object needs to Update it's realizations. /// Currently, GlyphRun is the only MIL object that needs realization /// updates. /// internal abstract void UpdateRealizations(RealizationContext realizationContext); ////// Calls methods on the DrawingContext that are equivalent to the /// Drawing with the Drawing's current value. /// internal abstract void WalkCurrentValue(DrawingContextWalker ctx); #endregion Internal Methods #region IDrawingContent implementation void IDrawingContent.PrecomputeContent() { PrecomputeCore(); } bool IDrawingContent.ContentRequiresRealizationUpdates { get { return this.RequiresRealizationUpdates; } } ////// True if this content can introduce graphness /// bool IDrawingContent.ContentIntroducesGraphness { get { return CanIntroduceGraphness(); } } #endregion IDrawingContent implementation ////// Precompute updates cached data on the Drawings. Currently it is only caching the /// realization flag. /// internal override void Precompute() { PrecomputeCore(); } ////// Derived classes implement the caching of the RequiresRealizationUpdates flag /// in this method. /// ////// internal abstract void PrecomputeCore(); /// /// True if and only if the Drawing requires realization updates. /// internal override bool RequiresRealizationUpdates { get { return m_requiresRealizationUpdates; } set { m_requiresRealizationUpdates = value; } } ////// Returns the bounding box occupied by the content /// ////// Bounding box occupied by the content /// Rect IDrawingContent.GetContentBounds(BoundsDrawingContextWalker ctx) { Debug.Assert(ctx != null); WalkCurrentValue(ctx); return ctx.Bounds; } ////// Forward the current value of the content to the DrawingContextWalker /// methods. /// /// DrawingContextWalker to forward content to. void IDrawingContent.WalkContent(DrawingContextWalker ctx) { ((Drawing)this).WalkCurrentValue(ctx); } ////// Determines whether or not a point exists within the content /// /// Point to hit-test for. ////// 'true' if the point exists within the content, 'false' otherwise /// bool IDrawingContent.HitTestPoint(Point point) { return DrawingServices.HitTestPoint(this, point); } ////// Hit-tests a geometry against this content /// /// PathGeometry to hit-test for. ////// IntersectionDetail describing the result of the hit-test /// IntersectionDetail IDrawingContent.HitTestGeometry(PathGeometry geometry) { return DrawingServices.HitTestGeometry(this, geometry); } ////// Propagates an event handler to Freezables referenced by /// the content. /// /// Event handler to propagate /// 'true' to add the handler, 'false' to remove it void IDrawingContent.PropagateChangedHandler(EventHandler handler, bool adding) { if (!IsFrozen) { if (adding) { ((Drawing)this).Changed += handler; } else { ((Drawing)this).Changed -= handler; } } } ////// Calls UpdatesRealization on GlyphRun's referenced by the content /// /// Context to create realization updates for void IDrawingContent.UpdateRealizations(RealizationContext realizationContext) { ((Drawing)this).UpdateRealizations(realizationContext); } ////// GetBounds to calculate the bounds of this drawing. /// internal Rect GetBounds() { BoundsDrawingContextWalker ctx = new BoundsDrawingContextWalker(); WalkCurrentValue(ctx); return ctx.Bounds; } // private bool m_requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Drawing is the base Drawing class that defines the standard // interface which Drawing's must implement. // // History: // 2003/07/16 : adsmith - Created it. // 2003/07/28 : adsmith - Renamed it and hooked it up to the unmanaged code. // 2004/11/17 : timothyc - Repurposed Drawing from being a Changeable // implementation that contains render data into the base // class for the enumerable & modifiable Drawing subclasses. // 2005/01/26 : timothyc - Made Drawing abstract & removed legacy Drawing // support, including .Open() & IAddChild. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Resources; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Markup; using System.Windows.Threading; namespace System.Windows.Media { ////// Base class for the enumerable and modifiable Drawing subclasses. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public abstract partial class Drawing : Animatable, IDrawingContent, DUCE.IResource { #region Constructors ////// Constructor for Drawing /// ////// This constructor is internal to prevent external subclassing. /// internal Drawing() { } #endregion Constructors #region Public Properties ////// Bounds - the axis-aligned bounds of this Drawing. /// ////// Rect - the axis-aligned bounds of this Drawing. /// public Rect Bounds { get { ReadPreamble(); return GetBounds(); } } #endregion Public Properties #region Internal Methods ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { return true; } ////// Called when an object needs to Update it's realizations. /// Currently, GlyphRun is the only MIL object that needs realization /// updates. /// internal abstract void UpdateRealizations(RealizationContext realizationContext); ////// Calls methods on the DrawingContext that are equivalent to the /// Drawing with the Drawing's current value. /// internal abstract void WalkCurrentValue(DrawingContextWalker ctx); #endregion Internal Methods #region IDrawingContent implementation void IDrawingContent.PrecomputeContent() { PrecomputeCore(); } bool IDrawingContent.ContentRequiresRealizationUpdates { get { return this.RequiresRealizationUpdates; } } ////// True if this content can introduce graphness /// bool IDrawingContent.ContentIntroducesGraphness { get { return CanIntroduceGraphness(); } } #endregion IDrawingContent implementation ////// Precompute updates cached data on the Drawings. Currently it is only caching the /// realization flag. /// internal override void Precompute() { PrecomputeCore(); } ////// Derived classes implement the caching of the RequiresRealizationUpdates flag /// in this method. /// ////// internal abstract void PrecomputeCore(); /// /// True if and only if the Drawing requires realization updates. /// internal override bool RequiresRealizationUpdates { get { return m_requiresRealizationUpdates; } set { m_requiresRealizationUpdates = value; } } ////// Returns the bounding box occupied by the content /// ////// Bounding box occupied by the content /// Rect IDrawingContent.GetContentBounds(BoundsDrawingContextWalker ctx) { Debug.Assert(ctx != null); WalkCurrentValue(ctx); return ctx.Bounds; } ////// Forward the current value of the content to the DrawingContextWalker /// methods. /// /// DrawingContextWalker to forward content to. void IDrawingContent.WalkContent(DrawingContextWalker ctx) { ((Drawing)this).WalkCurrentValue(ctx); } ////// Determines whether or not a point exists within the content /// /// Point to hit-test for. ////// 'true' if the point exists within the content, 'false' otherwise /// bool IDrawingContent.HitTestPoint(Point point) { return DrawingServices.HitTestPoint(this, point); } ////// Hit-tests a geometry against this content /// /// PathGeometry to hit-test for. ////// IntersectionDetail describing the result of the hit-test /// IntersectionDetail IDrawingContent.HitTestGeometry(PathGeometry geometry) { return DrawingServices.HitTestGeometry(this, geometry); } ////// Propagates an event handler to Freezables referenced by /// the content. /// /// Event handler to propagate /// 'true' to add the handler, 'false' to remove it void IDrawingContent.PropagateChangedHandler(EventHandler handler, bool adding) { if (!IsFrozen) { if (adding) { ((Drawing)this).Changed += handler; } else { ((Drawing)this).Changed -= handler; } } } ////// Calls UpdatesRealization on GlyphRun's referenced by the content /// /// Context to create realization updates for void IDrawingContent.UpdateRealizations(RealizationContext realizationContext) { ((Drawing)this).UpdateRealizations(realizationContext); } ////// GetBounds to calculate the bounds of this drawing. /// internal Rect GetBounds() { BoundsDrawingContextWalker ctx = new BoundsDrawingContextWalker(); WalkCurrentValue(ctx); return ctx.Bounds; } // private bool m_requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
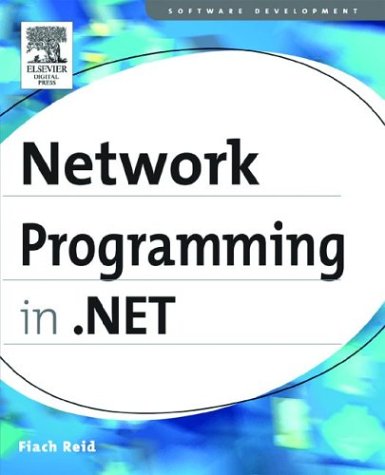
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HostedElements.cs
- RemoteWebConfigurationHostStream.cs
- QilScopedVisitor.cs
- TextBoxBase.cs
- CompatibleIComparer.cs
- HttpModuleCollection.cs
- WindowsToolbarAsMenu.cs
- DataRecordInternal.cs
- DataFormat.cs
- BitConverter.cs
- httpserverutility.cs
- UnmanagedMarshal.cs
- ToolStripDropTargetManager.cs
- DataObjectMethodAttribute.cs
- ApplicationBuildProvider.cs
- TransformPatternIdentifiers.cs
- ZeroOpNode.cs
- ColorInterpolationModeValidation.cs
- TrustManagerMoreInformation.cs
- SponsorHelper.cs
- RowParagraph.cs
- AuthorizationRule.cs
- TokenCreationParameter.cs
- DynamicDataManager.cs
- LassoSelectionBehavior.cs
- TimeoutException.cs
- XmlSignificantWhitespace.cs
- EncryptedReference.cs
- MimeReflector.cs
- NumericPagerField.cs
- DataGridViewButtonColumn.cs
- CrossSiteScriptingValidation.cs
- XmlCompatibilityReader.cs
- Models.cs
- FloatUtil.cs
- WebPartRestoreVerb.cs
- FrameworkContentElement.cs
- ItemCheckEvent.cs
- IndexExpression.cs
- NaturalLanguageHyphenator.cs
- MessageDecoder.cs
- HwndHostAutomationPeer.cs
- TextTreeFixupNode.cs
- MailAddressParser.cs
- LexicalChunk.cs
- ImageSource.cs
- CopyCodeAction.cs
- WebPartCatalogAddVerb.cs
- ExtractedStateEntry.cs
- Main.cs
- ConsoleKeyInfo.cs
- ResourceAttributes.cs
- ValueSerializer.cs
- RightNameExpirationInfoPair.cs
- OptimalBreakSession.cs
- VerticalAlignConverter.cs
- XmlAttributeCollection.cs
- RenderTargetBitmap.cs
- WorkItem.cs
- DbParameterHelper.cs
- InputMethodStateChangeEventArgs.cs
- ObjectCloneHelper.cs
- SystemIPv6InterfaceProperties.cs
- HybridDictionary.cs
- Error.cs
- DataGridTableCollection.cs
- Utilities.cs
- DurableEnlistmentState.cs
- ClientClassGenerator.cs
- CalendarTable.cs
- SimpleNameService.cs
- ActivitySurrogate.cs
- RegexRunnerFactory.cs
- ContextStaticAttribute.cs
- AutomationElementIdentifiers.cs
- DirectoryObjectSecurity.cs
- EventArgs.cs
- HttpListenerException.cs
- ToolStripKeyboardHandlingService.cs
- Stack.cs
- Select.cs
- XsltConvert.cs
- _FtpDataStream.cs
- ListControl.cs
- FilterableAttribute.cs
- WSSecurityTokenSerializer.cs
- ItemType.cs
- DocumentViewerConstants.cs
- XmlWrappingReader.cs
- GridPatternIdentifiers.cs
- ExtendedProperty.cs
- UriGenerator.cs
- MemoryPressure.cs
- PublisherIdentityPermission.cs
- MenuItem.cs
- OleDbStruct.cs
- Int64Storage.cs
- XmlReaderSettings.cs
- Stroke.cs
- odbcmetadatacolumnnames.cs