Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / Windows / Markup / ValueSerializer.cs / 1 / ValueSerializer.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2005 // // File: ValueSerializer.cs // // Contents: Service for providing and finding custom serialization for // value and value like types. // // Created: 04/28/2005 [....] // //----------------------------------------------------------------------- using System.ComponentModel; using System.Collections; using System.Collections.Generic; using MS.Internal.Serialization; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Markup { ////// ValueSerializer allows a type to declare a serializer to control how the type is serializer to and from string /// If a TypeConverter is declared for a type that converts to and from a string, a default value serializer will /// be created for the type. The string values must be loss-less (i.e. converting to and from a string doesn't loose /// data) and must be stable (i.e. returns the same string for the same value). If a type converter doesn't meet /// these requirements, a custom ValueSerializer must be declared that meet the requirements or associate a null /// ValueSerializer with the type to indicate the type converter should be ignored. Implementation of ValueSerializer /// should avoid throwing exceptions. Any exceptions thrown could possibly terminate serialization. /// public abstract class ValueSerializer { ////// Constructor for a ValueSerializer /// protected ValueSerializer() { } ////// Returns true if the given value can be converted to a string. /// /// Value to convert /// Context information ///Whether or not the value can be converted to a string public virtual bool CanConvertToString(object value, IValueSerializerContext context) { return false; } ////// Returns true if the given value can be converted from a string. /// /// The string to convert /// Context information ///Whether or not the value can be converted from a string public virtual bool CanConvertFromString(string value, IValueSerializerContext context) { return false; } ////// Converts the given value to a string for use in serialization. This method should only be /// called if CanConvertToString returns true for the given value. /// /// The value to convert to a string /// Context information ///A string representation of value public virtual string ConvertToString(object value, IValueSerializerContext context) { throw GetConvertToException(value, typeof(string)); } ////// Convert a string to an object. This method should only be called if CanConvertFromString /// returns true for the given string. /// /// The string value to convert /// Context information ///An object corresponding to the string value public virtual object ConvertFromString(string value, IValueSerializerContext context) { throw GetConvertFromException(value); } static ListEmpty = new List (); /// /// Returns an enumeration of the types referenced by the value serializer. If the value serializer asks for /// a value serializer for System.Type, any types it asks to convert should be supplied in the returned /// enumeration. This allows a serializer to ensure a de-serializer has enough information about the types /// this serializer converts. /// /// Since a value serializer doesn't exist by default, it is important the value serializer be requested from /// the IValueSerializerContext, not ValueSerializer.GetSerializerFor. This allows a serializer to encode /// context information (such as xmlns definitions) to the System.Type converter (for example, which prefix /// to generate). /// /// The value being serialized /// Context information ///An enumeration of the types converted by this serializer public virtual IEnumerableTypeReferences(object value, IValueSerializerContext context) { return Empty; } /// /// Get the value serializer declared for the given type. /// /// The value type to serialize ///The value serializer associated with the given type public static ValueSerializer GetSerializerFor(Type type) { if (type == null) throw new ArgumentNullException("type"); object value = _valueSerializers[type]; if (value != null) // This uses _valueSerializersLock's instance as a sentinal for null (as opposed to not attempted yet). return value == _valueSerializersLock ? null : value as ValueSerializer; AttributeCollection attributes = TypeDescriptor.GetAttributes(type); ValueSerializerAttribute attribute = attributes[typeof(ValueSerializerAttribute)] as ValueSerializerAttribute; ValueSerializer result = null; if (attribute != null) result = (ValueSerializer)Activator.CreateInstance(attribute.ValueSerializerType); if (result == null) { if (type == typeof(string)) { result = new StringValueSerializer(); } else { // Try to use the type converter TypeConverter converter = TypeConverterHelper.GetTypeConverter(type); // DateTime is a special-case. We can't use the DateTimeConverter, because it doesn't // support anything other than user culture and invariant culture, and we need to specify // en-us culture. if (converter.GetType() == typeof(DateTimeConverter2)) { result = new DateTimeValueSerializer(); } else if (converter.CanConvertTo(typeof(string)) && converter.CanConvertFrom(typeof(string)) && !(converter is ReferenceConverter)) { result = new TypeConverterValueSerializer(converter); } } } lock (_valueSerializersLock) { // This uses _valueSerializersLock's instance as a sentinal for null (as opposed to not attempted yet). _valueSerializers[type] = result == null ? _valueSerializersLock : result; } return result; } ////// Get the value serializer declared for the given property. ValueSerializer can be overriden by an attribute /// on the property declaration. /// /// PropertyDescriptor for the property to be serialized ///A value serializer associated with the given property public static ValueSerializer GetSerializerFor(PropertyDescriptor descriptor) { // ValueSerializer result; if (descriptor == null) { throw new ArgumentNullException("descriptor"); } #pragma warning suppress 6506 // descriptor is obviously not null ValueSerializerAttribute serializerAttribute = descriptor.Attributes[typeof(ValueSerializerAttribute)] as ValueSerializerAttribute; if (serializerAttribute != null) { result = (ValueSerializer)Activator.CreateInstance(serializerAttribute.ValueSerializerType); } else { result = GetSerializerFor(descriptor.PropertyType); if (result == null || result is TypeConverterValueSerializer) { TypeConverter converter = descriptor.Converter; if (converter!=null && converter.CanConvertTo(typeof(string)) && converter.CanConvertFrom(typeof(string)) && !(converter is ReferenceConverter)) result = new TypeConverterValueSerializer(converter); } } return result; } ////// Get the value serializer declared for the given type. This version should be called whenever the caller /// has a IValueSerializerContext to ensure that the correct value serializer is returned for the given /// context. /// /// The value type to serialize /// Context information ///The value serializer associated with the given type public static ValueSerializer GetSerializerFor(Type type, IValueSerializerContext context) { if (context != null) { ValueSerializer result = context.GetValueSerializerFor(type); if (result != null) return result; } return GetSerializerFor(type); } ////// Get the value serializer declared for the given property. ValueSerializer can be overriden by an attribute /// on the property declaration. This version should be called whenever the caller has a /// IValueSerializerContext to ensure that the correct value serializer is returned for the given context. /// /// PropertyDescriptor for the property to be serialized /// Context information ///A value serializer associated with the given property public static ValueSerializer GetSerializerFor(PropertyDescriptor descriptor, IValueSerializerContext context) { if (context != null) { ValueSerializer result = context.GetValueSerializerFor(descriptor); if (result != null) return result; } return GetSerializerFor(descriptor); } ////// Return a exception to throw if the value cannot be converted /// protected Exception GetConvertToException(object value, Type destinationType) { string text; if (value == null) { text = SR.Get(SRID.ToStringNull); } else { text = value.GetType().FullName; } return new NotSupportedException(SR.Get(SRID.ConvertToException, base.GetType().Name, text, destinationType.FullName)); } ////// Return a exception to throw if the string cannot be converted /// protected Exception GetConvertFromException(object value) { string text; if (value == null) { text = SR.Get(SRID.ToStringNull); } else { text = value.GetType().FullName; } return new NotSupportedException(SR.Get(SRID.ConvertFromException, base.GetType().Name, text)); } private static void TypeDescriptorRefreshed(RefreshEventArgs args) { _valueSerializers = new Hashtable(); } static ValueSerializer() { TypeDescriptor.Refreshed += TypeDescriptorRefreshed; } private static object _valueSerializersLock = new object(); private static Hashtable _valueSerializers = new Hashtable(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2005 // // File: ValueSerializer.cs // // Contents: Service for providing and finding custom serialization for // value and value like types. // // Created: 04/28/2005 [....] // //----------------------------------------------------------------------- using System.ComponentModel; using System.Collections; using System.Collections.Generic; using MS.Internal.Serialization; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Markup { ////// ValueSerializer allows a type to declare a serializer to control how the type is serializer to and from string /// If a TypeConverter is declared for a type that converts to and from a string, a default value serializer will /// be created for the type. The string values must be loss-less (i.e. converting to and from a string doesn't loose /// data) and must be stable (i.e. returns the same string for the same value). If a type converter doesn't meet /// these requirements, a custom ValueSerializer must be declared that meet the requirements or associate a null /// ValueSerializer with the type to indicate the type converter should be ignored. Implementation of ValueSerializer /// should avoid throwing exceptions. Any exceptions thrown could possibly terminate serialization. /// public abstract class ValueSerializer { ////// Constructor for a ValueSerializer /// protected ValueSerializer() { } ////// Returns true if the given value can be converted to a string. /// /// Value to convert /// Context information ///Whether or not the value can be converted to a string public virtual bool CanConvertToString(object value, IValueSerializerContext context) { return false; } ////// Returns true if the given value can be converted from a string. /// /// The string to convert /// Context information ///Whether or not the value can be converted from a string public virtual bool CanConvertFromString(string value, IValueSerializerContext context) { return false; } ////// Converts the given value to a string for use in serialization. This method should only be /// called if CanConvertToString returns true for the given value. /// /// The value to convert to a string /// Context information ///A string representation of value public virtual string ConvertToString(object value, IValueSerializerContext context) { throw GetConvertToException(value, typeof(string)); } ////// Convert a string to an object. This method should only be called if CanConvertFromString /// returns true for the given string. /// /// The string value to convert /// Context information ///An object corresponding to the string value public virtual object ConvertFromString(string value, IValueSerializerContext context) { throw GetConvertFromException(value); } static ListEmpty = new List (); /// /// Returns an enumeration of the types referenced by the value serializer. If the value serializer asks for /// a value serializer for System.Type, any types it asks to convert should be supplied in the returned /// enumeration. This allows a serializer to ensure a de-serializer has enough information about the types /// this serializer converts. /// /// Since a value serializer doesn't exist by default, it is important the value serializer be requested from /// the IValueSerializerContext, not ValueSerializer.GetSerializerFor. This allows a serializer to encode /// context information (such as xmlns definitions) to the System.Type converter (for example, which prefix /// to generate). /// /// The value being serialized /// Context information ///An enumeration of the types converted by this serializer public virtual IEnumerableTypeReferences(object value, IValueSerializerContext context) { return Empty; } /// /// Get the value serializer declared for the given type. /// /// The value type to serialize ///The value serializer associated with the given type public static ValueSerializer GetSerializerFor(Type type) { if (type == null) throw new ArgumentNullException("type"); object value = _valueSerializers[type]; if (value != null) // This uses _valueSerializersLock's instance as a sentinal for null (as opposed to not attempted yet). return value == _valueSerializersLock ? null : value as ValueSerializer; AttributeCollection attributes = TypeDescriptor.GetAttributes(type); ValueSerializerAttribute attribute = attributes[typeof(ValueSerializerAttribute)] as ValueSerializerAttribute; ValueSerializer result = null; if (attribute != null) result = (ValueSerializer)Activator.CreateInstance(attribute.ValueSerializerType); if (result == null) { if (type == typeof(string)) { result = new StringValueSerializer(); } else { // Try to use the type converter TypeConverter converter = TypeConverterHelper.GetTypeConverter(type); // DateTime is a special-case. We can't use the DateTimeConverter, because it doesn't // support anything other than user culture and invariant culture, and we need to specify // en-us culture. if (converter.GetType() == typeof(DateTimeConverter2)) { result = new DateTimeValueSerializer(); } else if (converter.CanConvertTo(typeof(string)) && converter.CanConvertFrom(typeof(string)) && !(converter is ReferenceConverter)) { result = new TypeConverterValueSerializer(converter); } } } lock (_valueSerializersLock) { // This uses _valueSerializersLock's instance as a sentinal for null (as opposed to not attempted yet). _valueSerializers[type] = result == null ? _valueSerializersLock : result; } return result; } ////// Get the value serializer declared for the given property. ValueSerializer can be overriden by an attribute /// on the property declaration. /// /// PropertyDescriptor for the property to be serialized ///A value serializer associated with the given property public static ValueSerializer GetSerializerFor(PropertyDescriptor descriptor) { // ValueSerializer result; if (descriptor == null) { throw new ArgumentNullException("descriptor"); } #pragma warning suppress 6506 // descriptor is obviously not null ValueSerializerAttribute serializerAttribute = descriptor.Attributes[typeof(ValueSerializerAttribute)] as ValueSerializerAttribute; if (serializerAttribute != null) { result = (ValueSerializer)Activator.CreateInstance(serializerAttribute.ValueSerializerType); } else { result = GetSerializerFor(descriptor.PropertyType); if (result == null || result is TypeConverterValueSerializer) { TypeConverter converter = descriptor.Converter; if (converter!=null && converter.CanConvertTo(typeof(string)) && converter.CanConvertFrom(typeof(string)) && !(converter is ReferenceConverter)) result = new TypeConverterValueSerializer(converter); } } return result; } ////// Get the value serializer declared for the given type. This version should be called whenever the caller /// has a IValueSerializerContext to ensure that the correct value serializer is returned for the given /// context. /// /// The value type to serialize /// Context information ///The value serializer associated with the given type public static ValueSerializer GetSerializerFor(Type type, IValueSerializerContext context) { if (context != null) { ValueSerializer result = context.GetValueSerializerFor(type); if (result != null) return result; } return GetSerializerFor(type); } ////// Get the value serializer declared for the given property. ValueSerializer can be overriden by an attribute /// on the property declaration. This version should be called whenever the caller has a /// IValueSerializerContext to ensure that the correct value serializer is returned for the given context. /// /// PropertyDescriptor for the property to be serialized /// Context information ///A value serializer associated with the given property public static ValueSerializer GetSerializerFor(PropertyDescriptor descriptor, IValueSerializerContext context) { if (context != null) { ValueSerializer result = context.GetValueSerializerFor(descriptor); if (result != null) return result; } return GetSerializerFor(descriptor); } ////// Return a exception to throw if the value cannot be converted /// protected Exception GetConvertToException(object value, Type destinationType) { string text; if (value == null) { text = SR.Get(SRID.ToStringNull); } else { text = value.GetType().FullName; } return new NotSupportedException(SR.Get(SRID.ConvertToException, base.GetType().Name, text, destinationType.FullName)); } ////// Return a exception to throw if the string cannot be converted /// protected Exception GetConvertFromException(object value) { string text; if (value == null) { text = SR.Get(SRID.ToStringNull); } else { text = value.GetType().FullName; } return new NotSupportedException(SR.Get(SRID.ConvertFromException, base.GetType().Name, text)); } private static void TypeDescriptorRefreshed(RefreshEventArgs args) { _valueSerializers = new Hashtable(); } static ValueSerializer() { TypeDescriptor.Refreshed += TypeDescriptorRefreshed; } private static object _valueSerializersLock = new object(); private static Hashtable _valueSerializers = new Hashtable(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
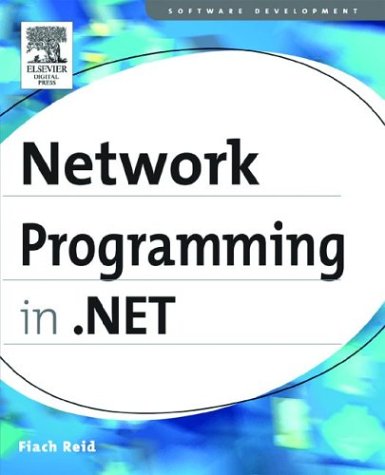
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeTypeConverter.cs
- PermissionListSet.cs
- DataGridColumnCollection.cs
- DataStreamFromComStream.cs
- XmlCustomFormatter.cs
- RegexCapture.cs
- DebugManager.cs
- StoragePropertyMapping.cs
- AmbientValueAttribute.cs
- SoapIgnoreAttribute.cs
- IndexOutOfRangeException.cs
- ErrorLog.cs
- SmiEventSink_DeferedProcessing.cs
- JsonReaderDelegator.cs
- Underline.cs
- EventLogRecord.cs
- ColorContextHelper.cs
- SystemInfo.cs
- NTAccount.cs
- ModelItemDictionary.cs
- TableLayoutStyleCollection.cs
- BevelBitmapEffect.cs
- MethodExpression.cs
- RootAction.cs
- RectKeyFrameCollection.cs
- TriggerCollection.cs
- WriteTimeStream.cs
- PeerNodeTraceRecord.cs
- WorkflowItemPresenter.cs
- CheckBox.cs
- SetterTriggerConditionValueConverter.cs
- BitmapEffectDrawing.cs
- XmlSchemaImport.cs
- MenuItemStyle.cs
- SocketManager.cs
- MergeLocalizationDirectives.cs
- WebPartConnectVerb.cs
- TextSimpleMarkerProperties.cs
- RuntimeConfigurationRecord.cs
- EventListenerClientSide.cs
- GradientSpreadMethodValidation.cs
- XmlSerializationReader.cs
- DefaultDialogButtons.cs
- prompt.cs
- EncryptedKeyIdentifierClause.cs
- BindingManagerDataErrorEventArgs.cs
- VersionedStreamOwner.cs
- ListBox.cs
- Cursors.cs
- ProgressBar.cs
- Line.cs
- cookie.cs
- Logging.cs
- RuleSettings.cs
- GenericsInstances.cs
- ActivityDesignerLayoutSerializers.cs
- TypeGeneratedEventArgs.cs
- DataControlFieldHeaderCell.cs
- SchemaElementDecl.cs
- LayoutTableCell.cs
- XNodeNavigator.cs
- DrawingAttributesDefaultValueFactory.cs
- TypeEnumerableViewSchema.cs
- ObjectDataSource.cs
- RuntimeWrappedException.cs
- DecimalAnimation.cs
- ServiceNameCollection.cs
- SQLDouble.cs
- ClientSettingsStore.cs
- SoapMessage.cs
- Int16Converter.cs
- SizeAnimationBase.cs
- OdbcDataReader.cs
- XmlSchemaInferenceException.cs
- Rect3DConverter.cs
- ErrorTableItemStyle.cs
- ManagedIStream.cs
- EntityDataSourceSelectedEventArgs.cs
- EUCJPEncoding.cs
- BitmapSource.cs
- InstanceNotReadyException.cs
- EntityCommandExecutionException.cs
- Expander.cs
- ReferentialConstraint.cs
- ContextStaticAttribute.cs
- OdbcCommand.cs
- RowSpanVector.cs
- ElementUtil.cs
- DomNameTable.cs
- TemplateKey.cs
- GACMembershipCondition.cs
- UntrustedRecipientException.cs
- CollectionBuilder.cs
- BinHexDecoder.cs
- UnsafeNativeMethods.cs
- TabItem.cs
- RegistrationServices.cs
- DynamicRenderer.cs
- DecimalConstantAttribute.cs
- XmlSequenceWriter.cs