Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / VisualStateGroup.cs / 1458001 / VisualStateGroup.cs
// -------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All Rights Reserved. // ------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows; using System.Windows.Controls; using System.Windows.Markup; using System.Windows.Media.Animation; namespace System.Windows { ////// A group of mutually exclusive visual states. /// [ContentProperty("States")] [RuntimeNameProperty("Name")] public class VisualStateGroup : DependencyObject { ////// The Name of the VisualStateGroup. /// public string Name { get; set; } ////// VisualStates in the group. /// public IList States { get { if (_states == null) { _states = new FreezableCollection(); } return _states; } } /// /// Transitions between VisualStates in the group. /// public IList Transitions { get { if (_transitions == null) { _transitions = new FreezableCollection(); } return _transitions; } } /// /// VisualState that is currently applied. /// public VisualState CurrentState { get; internal set; } internal VisualState GetState(string stateName) { for (int stateIndex = 0; stateIndex < States.Count; ++stateIndex) { VisualState state = (VisualState)States[stateIndex]; if (state.Name == stateName) { return state; } } return null; } internal CollectionCurrentStoryboards { get { if (_currentStoryboards == null) { _currentStoryboards = new Collection (); } return _currentStoryboards; } } internal void StartNewThenStopOld(FrameworkElement element, params Storyboard[] newStoryboards) { // Remove the old Storyboards. Remove is delayed until the next TimeManager tick, so the // handoff to the new storyboard is unaffected. for (int index = 0; index < CurrentStoryboards.Count; ++index) { if (CurrentStoryboards[index] == null) { continue; } CurrentStoryboards[index].Remove(element); } CurrentStoryboards.Clear(); // Start the new Storyboards for (int index = 0; index < newStoryboards.Length; ++index) { if (newStoryboards[index] == null) { continue; } newStoryboards[index].Begin(element, HandoffBehavior.SnapshotAndReplace, true); // Hold on to the running Storyboards CurrentStoryboards.Add(newStoryboards[index]); // Silverlight had an issue where initially, a checked CheckBox would not show the check mark // until the second frame. They chose to do a Seek(0) at this point, which this line // is supposed to mimic. It does not seem to be equivalent, though, and WPF ends up // with some odd animation behavior. I haven't seen the CheckBox issue on WPF, so // commenting this out for now. // newStoryboards[index].SeekAlignedToLastTick(element, TimeSpan.Zero, TimeSeekOrigin.BeginTime); } } internal void RaiseCurrentStateChanging(FrameworkElement stateGroupsRoot, VisualState oldState, VisualState newState, FrameworkElement control) { if (CurrentStateChanging != null) { CurrentStateChanging(stateGroupsRoot, new VisualStateChangedEventArgs(oldState, newState, control, stateGroupsRoot)); } } internal void RaiseCurrentStateChanged(FrameworkElement stateGroupsRoot, VisualState oldState, VisualState newState, FrameworkElement control) { if (CurrentStateChanged != null) { CurrentStateChanged(stateGroupsRoot, new VisualStateChangedEventArgs(oldState, newState, control, stateGroupsRoot)); } } /// /// Raised when transition begins /// public event EventHandlerCurrentStateChanged; /// /// Raised when transition ends and new state storyboard begins. /// public event EventHandlerCurrentStateChanging; private Collection _currentStoryboards; private FreezableCollection _states; private FreezableCollection _transitions; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // -------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All Rights Reserved. // ------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows; using System.Windows.Controls; using System.Windows.Markup; using System.Windows.Media.Animation; namespace System.Windows { /// /// A group of mutually exclusive visual states. /// [ContentProperty("States")] [RuntimeNameProperty("Name")] public class VisualStateGroup : DependencyObject { ////// The Name of the VisualStateGroup. /// public string Name { get; set; } ////// VisualStates in the group. /// public IList States { get { if (_states == null) { _states = new FreezableCollection(); } return _states; } } /// /// Transitions between VisualStates in the group. /// public IList Transitions { get { if (_transitions == null) { _transitions = new FreezableCollection(); } return _transitions; } } /// /// VisualState that is currently applied. /// public VisualState CurrentState { get; internal set; } internal VisualState GetState(string stateName) { for (int stateIndex = 0; stateIndex < States.Count; ++stateIndex) { VisualState state = (VisualState)States[stateIndex]; if (state.Name == stateName) { return state; } } return null; } internal CollectionCurrentStoryboards { get { if (_currentStoryboards == null) { _currentStoryboards = new Collection (); } return _currentStoryboards; } } internal void StartNewThenStopOld(FrameworkElement element, params Storyboard[] newStoryboards) { // Remove the old Storyboards. Remove is delayed until the next TimeManager tick, so the // handoff to the new storyboard is unaffected. for (int index = 0; index < CurrentStoryboards.Count; ++index) { if (CurrentStoryboards[index] == null) { continue; } CurrentStoryboards[index].Remove(element); } CurrentStoryboards.Clear(); // Start the new Storyboards for (int index = 0; index < newStoryboards.Length; ++index) { if (newStoryboards[index] == null) { continue; } newStoryboards[index].Begin(element, HandoffBehavior.SnapshotAndReplace, true); // Hold on to the running Storyboards CurrentStoryboards.Add(newStoryboards[index]); // Silverlight had an issue where initially, a checked CheckBox would not show the check mark // until the second frame. They chose to do a Seek(0) at this point, which this line // is supposed to mimic. It does not seem to be equivalent, though, and WPF ends up // with some odd animation behavior. I haven't seen the CheckBox issue on WPF, so // commenting this out for now. // newStoryboards[index].SeekAlignedToLastTick(element, TimeSpan.Zero, TimeSeekOrigin.BeginTime); } } internal void RaiseCurrentStateChanging(FrameworkElement stateGroupsRoot, VisualState oldState, VisualState newState, FrameworkElement control) { if (CurrentStateChanging != null) { CurrentStateChanging(stateGroupsRoot, new VisualStateChangedEventArgs(oldState, newState, control, stateGroupsRoot)); } } internal void RaiseCurrentStateChanged(FrameworkElement stateGroupsRoot, VisualState oldState, VisualState newState, FrameworkElement control) { if (CurrentStateChanged != null) { CurrentStateChanged(stateGroupsRoot, new VisualStateChangedEventArgs(oldState, newState, control, stateGroupsRoot)); } } /// /// Raised when transition begins /// public event EventHandlerCurrentStateChanged; /// /// Raised when transition ends and new state storyboard begins. /// public event EventHandlerCurrentStateChanging; private Collection _currentStoryboards; private FreezableCollection _states; private FreezableCollection _transitions; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
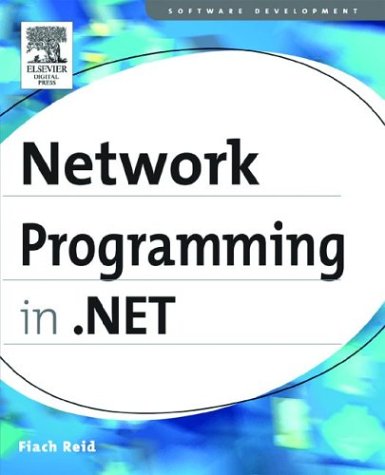
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceQueryOfT.cs
- BitmapEffectGroup.cs
- MetadataItem_Static.cs
- IDispatchConstantAttribute.cs
- sqlmetadatafactory.cs
- Int64Converter.cs
- UndoEngine.cs
- CompilationLock.cs
- IApplicationTrustManager.cs
- Attributes.cs
- FormsIdentity.cs
- MailAddress.cs
- SizeAnimationBase.cs
- RegexMatch.cs
- WindowsTreeView.cs
- DirectoryGroupQuery.cs
- BooleanAnimationBase.cs
- SerializationException.cs
- PageParserFilter.cs
- ErrorWrapper.cs
- PageRanges.cs
- CollectionViewProxy.cs
- TreeViewItem.cs
- WebPartTransformerCollection.cs
- AstNode.cs
- TextParagraphProperties.cs
- ObjectTokenCategory.cs
- WebRequest.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- TextHintingModeValidation.cs
- XmlSchemaSimpleContentExtension.cs
- AssemblyResourceLoader.cs
- BinHexDecoder.cs
- CheckBoxStandardAdapter.cs
- SynthesizerStateChangedEventArgs.cs
- ListMarkerLine.cs
- Region.cs
- DataBindingList.cs
- ObjectConverter.cs
- BooleanSwitch.cs
- FormatterConverter.cs
- PriorityRange.cs
- TextViewSelectionProcessor.cs
- CachedTypeface.cs
- ListViewContainer.cs
- ExeConfigurationFileMap.cs
- JumpItem.cs
- Schema.cs
- selecteditemcollection.cs
- CompilerGeneratedAttribute.cs
- SoapMessage.cs
- EntityKeyElement.cs
- CustomLineCap.cs
- SettingsPropertyCollection.cs
- XmlQualifiedNameTest.cs
- HttpCacheParams.cs
- StylusDownEventArgs.cs
- KoreanLunisolarCalendar.cs
- GACIdentityPermission.cs
- DynamicActionMessageFilter.cs
- ToolboxItemFilterAttribute.cs
- BitmapEffectInput.cs
- MenuAutoFormat.cs
- RegistrationServices.cs
- New.cs
- AutomationProperties.cs
- OutputCacheSection.cs
- DataSetFieldSchema.cs
- _FixedSizeReader.cs
- SqlComparer.cs
- StylusButtonCollection.cs
- SerializableAttribute.cs
- GridEntry.cs
- AmbiguousMatchException.cs
- WebPartTransformer.cs
- ExtendedPropertyCollection.cs
- ContentTextAutomationPeer.cs
- TableSectionStyle.cs
- HtmlValidatorAdapter.cs
- FixedSOMTable.cs
- Utilities.cs
- TemplateField.cs
- ArgumentNullException.cs
- EmbeddedMailObject.cs
- MemberListBinding.cs
- Atom10FormatterFactory.cs
- PersonalizationAdministration.cs
- Keywords.cs
- NamespaceEmitter.cs
- ClientFormsAuthenticationCredentials.cs
- DataControlFieldCell.cs
- SafeSecurityHandles.cs
- InternalPolicyElement.cs
- WebDisplayNameAttribute.cs
- MenuItemStyle.cs
- FrameworkContentElement.cs
- Vector3DConverter.cs
- ErrorHandler.cs
- BrowserCapabilitiesFactoryBase.cs
- WriteableBitmap.cs