Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ButtonInternal / CheckBoxStandardAdapter.cs / 1305376 / CheckBoxStandardAdapter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ButtonInternal { using System; using System.Diagnostics; using System.Drawing; using System.Drawing.Drawing2D; using System.Drawing.Imaging; using System.Drawing.Text; using System.Windows.Forms; using System.Windows.Forms.Layout; internal sealed class CheckBoxStandardAdapter : CheckBoxBaseAdapter { internal CheckBoxStandardAdapter(ButtonBase control) : base(control) {} internal override void PaintUp(PaintEventArgs e, CheckState state) { if (Control.Appearance == Appearance.Button) { ButtonAdapter.PaintUp(e, Control.CheckState); } else { ColorData colors = PaintRender(e.Graphics).Calculate(); LayoutData layout = Layout(e).Layout(); PaintButtonBackground(e, Control.ClientRectangle, null); //minor adjustment to make sure the appearance is exactly the same as Win32 app. int focusRectFixup = layout.focus.X & 0x1; // if it's odd, subtract one pixel for fixup. if (!Application.RenderWithVisualStyles) { focusRectFixup = 1 - focusRectFixup; } if (!layout.options.everettButtonCompat) { layout.textBounds.Offset(-1, -1); } layout.imageBounds.Offset(-1, -1); layout.focus.Offset(-(focusRectFixup+1), -2); layout.focus.Width = layout.textBounds.Width + layout.imageBounds.Width - 1; layout.focus.Intersect(layout.textBounds); if( layout.options.textAlign != LayoutUtils.AnyLeft && layout.options.useCompatibleTextRendering && layout.options.font.Italic) { // fixup for GDI+ text rendering. VSW#515164 layout.focus.Width += 2; } PaintImage(e, layout); DrawCheckBox(e, layout); PaintField(e, layout, colors, colors.windowText, true); } } internal override void PaintDown(PaintEventArgs e, CheckState state) { if (Control.Appearance == Appearance.Button) { ButtonAdapter.PaintDown(e, Control.CheckState); } else { PaintUp(e, state); } } internal override void PaintOver(PaintEventArgs e, CheckState state) { if (Control.Appearance == Appearance.Button) { ButtonAdapter.PaintOver(e, Control.CheckState); } else { PaintUp(e, state); } } internal override Size GetPreferredSizeCore(Size proposedSize) { if (Control.Appearance == Appearance.Button) { ButtonStandardAdapter adapter = new ButtonStandardAdapter(Control); return adapter.GetPreferredSizeCore(proposedSize); } else { using (Graphics measurementGraphics = WindowsFormsUtils.CreateMeasurementGraphics()) { using (PaintEventArgs pe = new PaintEventArgs(measurementGraphics, new Rectangle())) { LayoutOptions options = Layout(pe); return options.GetPreferredSizeCore(proposedSize); } } } } #region Layout private new ButtonStandardAdapter ButtonAdapter { get { return ((ButtonStandardAdapter)base.ButtonAdapter); } } protected override ButtonBaseAdapter CreateButtonAdapter() { return new ButtonStandardAdapter(Control); } protected override LayoutOptions Layout(PaintEventArgs e) { LayoutOptions layout = CommonLayout(); layout.checkPaddingSize = 1; layout.everettButtonCompat = !Application.RenderWithVisualStyles; // VSWhidbey 420870 if (Application.RenderWithVisualStyles) { using (Graphics g = WindowsFormsUtils.CreateMeasurementGraphics()) { layout.checkSize = CheckBoxRenderer.GetGlyphSize(g, CheckBoxRenderer.ConvertFromButtonState(GetState(), true, Control.MouseIsOver)).Width; } } // Dev10 bug 525537 else { layout.checkSize = (int)(layout.checkSize * GetDpiScaleRatio(e.Graphics)); } return layout; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ButtonInternal { using System; using System.Diagnostics; using System.Drawing; using System.Drawing.Drawing2D; using System.Drawing.Imaging; using System.Drawing.Text; using System.Windows.Forms; using System.Windows.Forms.Layout; internal sealed class CheckBoxStandardAdapter : CheckBoxBaseAdapter { internal CheckBoxStandardAdapter(ButtonBase control) : base(control) {} internal override void PaintUp(PaintEventArgs e, CheckState state) { if (Control.Appearance == Appearance.Button) { ButtonAdapter.PaintUp(e, Control.CheckState); } else { ColorData colors = PaintRender(e.Graphics).Calculate(); LayoutData layout = Layout(e).Layout(); PaintButtonBackground(e, Control.ClientRectangle, null); //minor adjustment to make sure the appearance is exactly the same as Win32 app. int focusRectFixup = layout.focus.X & 0x1; // if it's odd, subtract one pixel for fixup. if (!Application.RenderWithVisualStyles) { focusRectFixup = 1 - focusRectFixup; } if (!layout.options.everettButtonCompat) { layout.textBounds.Offset(-1, -1); } layout.imageBounds.Offset(-1, -1); layout.focus.Offset(-(focusRectFixup+1), -2); layout.focus.Width = layout.textBounds.Width + layout.imageBounds.Width - 1; layout.focus.Intersect(layout.textBounds); if( layout.options.textAlign != LayoutUtils.AnyLeft && layout.options.useCompatibleTextRendering && layout.options.font.Italic) { // fixup for GDI+ text rendering. VSW#515164 layout.focus.Width += 2; } PaintImage(e, layout); DrawCheckBox(e, layout); PaintField(e, layout, colors, colors.windowText, true); } } internal override void PaintDown(PaintEventArgs e, CheckState state) { if (Control.Appearance == Appearance.Button) { ButtonAdapter.PaintDown(e, Control.CheckState); } else { PaintUp(e, state); } } internal override void PaintOver(PaintEventArgs e, CheckState state) { if (Control.Appearance == Appearance.Button) { ButtonAdapter.PaintOver(e, Control.CheckState); } else { PaintUp(e, state); } } internal override Size GetPreferredSizeCore(Size proposedSize) { if (Control.Appearance == Appearance.Button) { ButtonStandardAdapter adapter = new ButtonStandardAdapter(Control); return adapter.GetPreferredSizeCore(proposedSize); } else { using (Graphics measurementGraphics = WindowsFormsUtils.CreateMeasurementGraphics()) { using (PaintEventArgs pe = new PaintEventArgs(measurementGraphics, new Rectangle())) { LayoutOptions options = Layout(pe); return options.GetPreferredSizeCore(proposedSize); } } } } #region Layout private new ButtonStandardAdapter ButtonAdapter { get { return ((ButtonStandardAdapter)base.ButtonAdapter); } } protected override ButtonBaseAdapter CreateButtonAdapter() { return new ButtonStandardAdapter(Control); } protected override LayoutOptions Layout(PaintEventArgs e) { LayoutOptions layout = CommonLayout(); layout.checkPaddingSize = 1; layout.everettButtonCompat = !Application.RenderWithVisualStyles; // VSWhidbey 420870 if (Application.RenderWithVisualStyles) { using (Graphics g = WindowsFormsUtils.CreateMeasurementGraphics()) { layout.checkSize = CheckBoxRenderer.GetGlyphSize(g, CheckBoxRenderer.ConvertFromButtonState(GetState(), true, Control.MouseIsOver)).Width; } } // Dev10 bug 525537 else { layout.checkSize = (int)(layout.checkSize * GetDpiScaleRatio(e.Graphics)); } return layout; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
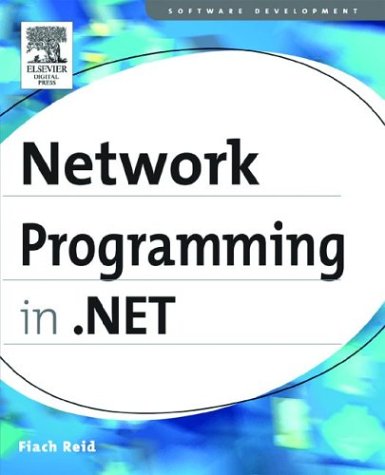
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Span.cs
- Point3DCollectionConverter.cs
- StrokeCollectionConverter.cs
- ScriptManagerProxy.cs
- XmlComment.cs
- WindowsAuthenticationModule.cs
- ConfigPathUtility.cs
- xamlnodes.cs
- FloatSumAggregationOperator.cs
- Stroke2.cs
- WebServiceFaultDesigner.cs
- SqlMetaData.cs
- FontResourceCache.cs
- ThicknessAnimationBase.cs
- InputEventArgs.cs
- XmlSchemaDocumentation.cs
- CommandBinding.cs
- GlobalEventManager.cs
- PasswordTextContainer.cs
- SurrogateDataContract.cs
- AdRotator.cs
- XPathNodePointer.cs
- Event.cs
- ExpressionsCollectionConverter.cs
- CodeTypeReferenceCollection.cs
- DefinitionUpdate.cs
- DeliveryStrategy.cs
- SQLBytesStorage.cs
- TagMapCollection.cs
- UnsafeNativeMethods.cs
- PointAnimationClockResource.cs
- Publisher.cs
- Baml2006Reader.cs
- SharedPerformanceCounter.cs
- HttpListenerException.cs
- Subtree.cs
- PerformanceCounterCategory.cs
- TimeSpanMinutesConverter.cs
- XmlCollation.cs
- BamlResourceDeserializer.cs
- LicenseException.cs
- RectAnimation.cs
- DbConnectionPoolGroup.cs
- ErrorProvider.cs
- StructuredTypeEmitter.cs
- RectKeyFrameCollection.cs
- ParagraphResult.cs
- WebBaseEventKeyComparer.cs
- RtType.cs
- DynamicObjectAccessor.cs
- PropertyNames.cs
- CharKeyFrameCollection.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- AdornerPresentationContext.cs
- Stack.cs
- ProviderSettings.cs
- WebPartZoneCollection.cs
- DictionaryEntry.cs
- TreeNodeStyleCollection.cs
- DispatcherSynchronizationContext.cs
- CopyCodeAction.cs
- ExportException.cs
- InteropExecutor.cs
- CTreeGenerator.cs
- WebPartsSection.cs
- TextCharacters.cs
- ProbeDuplex11AsyncResult.cs
- DataGridAddNewRow.cs
- RepeatBehaviorConverter.cs
- WebBrowsableAttribute.cs
- AuthenticationServiceManager.cs
- RuntimeHelpers.cs
- DataControlFieldCollection.cs
- IconConverter.cs
- ExternalException.cs
- HttpListenerPrefixCollection.cs
- IdentifierService.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- TdsValueSetter.cs
- DetailsViewActionList.cs
- PartManifestEntry.cs
- IndexedEnumerable.cs
- ListViewSelectEventArgs.cs
- SingleQueryOperator.cs
- HttpCapabilitiesSectionHandler.cs
- WebHttpBindingCollectionElement.cs
- DocumentXmlWriter.cs
- MouseEventArgs.cs
- QueryServiceConfigHandle.cs
- LogEntryHeaderv1Deserializer.cs
- SymbolTable.cs
- DataGridViewAccessibleObject.cs
- MTConfigUtil.cs
- MessageDecoder.cs
- CoTaskMemHandle.cs
- GPRECT.cs
- MailAddress.cs
- LocalizableAttribute.cs
- CapabilitiesSection.cs
- EditingScopeUndoUnit.cs