Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / DataBoundLiteralControl.cs / 1305376 / DataBoundLiteralControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Control that holds databinding expressions and literals * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.IO; using System.Text; using System.Security.Permissions; using System.Web.Util; internal class DataBoundLiteralControlBuilder : ControlBuilder { internal DataBoundLiteralControlBuilder() { } internal void AddLiteralString(string s) { Debug.Assert(!InDesigner, "!InDesigner"); // Make sure strings and databinding expressions alternate object lastBuilder = GetLastBuilder(); if (lastBuilder != null && lastBuilder is string) { AddSubBuilder(null); } AddSubBuilder(s); } internal void AddDataBindingExpression(CodeBlockBuilder codeBlockBuilder) { Debug.Assert(!InDesigner, "!InDesigner"); // Make sure strings and databinding expressions alternate object lastBuilder = GetLastBuilder(); if (lastBuilder == null || lastBuilder is CodeBlockBuilder) { AddSubBuilder(null); } AddSubBuilder(codeBlockBuilder); } internal int GetStaticLiteralsCount() { // it's divided by 2 because half are strings and half are databinding // expressions). '+1' because we always start with a literal string. return (SubBuilders.Count+1) / 2; } internal int GetDataBoundLiteralCount() { // it's divided by 2 because half are strings and half are databinding // expressions) return SubBuilders.Count / 2; } } ////// [ ToolboxItem(false) ] public sealed class DataBoundLiteralControl : Control, ITextControl { private string[] _staticLiterals; private string[] _dataBoundLiteral; private bool _hasDataBoundStrings; ///Defines the properties and methods of the DataBoundLiteralControl class. ///public DataBoundLiteralControl(int staticLiteralsCount, int dataBoundLiteralCount) { _staticLiterals = new string[staticLiteralsCount]; _dataBoundLiteral = new string[dataBoundLiteralCount]; PreventAutoID(); } /// public void SetStaticString(int index, string s) { _staticLiterals[index] = s; } /// public void SetDataBoundString(int index, string s) { _dataBoundLiteral[index] = s; _hasDataBoundStrings = true; } /// /// public string Text { get { StringBuilder sb = new StringBuilder(); int dataBoundLiteralCount = _dataBoundLiteral.Length; // Append literal and databound strings alternatively for (int i=0; i<_staticLiterals.Length; i++) { if (_staticLiterals[i] != null) sb.Append(_staticLiterals[i]); // Could be null if DataBind() was not called if (i < dataBoundLiteralCount && _dataBoundLiteral[i] != null) sb.Append(_dataBoundLiteral[i]); } return sb.ToString(); } } ///Gets the text content of the data-bound literal control. ///protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } /// /// /// protected override void LoadViewState(object savedState) { if (savedState != null) { _dataBoundLiteral = (string[]) savedState; _hasDataBoundStrings = true; } } ///Loads the previously saved state. Overridden to synchronize Text property with /// LiteralContent. ////// /// protected override object SaveViewState() { // Return null if we didn't get any databound strings if (!_hasDataBoundStrings) return null; // Only save the databound literals to the view state return _dataBoundLiteral; } ///The object that contains the state changes. ///protected internal override void Render(HtmlTextWriter output) { int dataBoundLiteralCount = _dataBoundLiteral.Length; // Render literal and databound strings alternatively for (int i=0; i<_staticLiterals.Length; i++) { if (_staticLiterals[i] != null) output.Write(_staticLiterals[i]); // Could be null if DataBind() was not called if (i < dataBoundLiteralCount && _dataBoundLiteral[i] != null) output.Write(_dataBoundLiteral[i]); } } /// /// /// string ITextControl.Text { get { return Text; } set { throw new NotSupportedException(); } } } ///Implementation of TextControl.Text property. ////// [ DataBindingHandler("System.Web.UI.Design.TextDataBindingHandler, " + AssemblyRef.SystemDesign), ToolboxItem(false) ] public sealed class DesignerDataBoundLiteralControl : Control { private string _text; public DesignerDataBoundLiteralControl() { PreventAutoID(); } ///Simpler version of DataBoundLiteralControlBuilder, used at design time. ////// public string Text { get { return _text; } set { _text = (value != null) ? value : String.Empty; } } protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } ///Gets or sets the text content of the data-bound literal control. ////// protected override void LoadViewState(object savedState) { if (savedState != null) _text = (string) savedState; } ///Loads the previously saved state. Overridden to synchronize Text property with /// LiteralContent. ////// protected internal override void Render(HtmlTextWriter output) { output.Write(_text); } ///Saves any state that was modified after the control began monitoring state changes. ////// protected override object SaveViewState() { return _text; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //The object that contains the state changes. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Control that holds databinding expressions and literals * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.IO; using System.Text; using System.Security.Permissions; using System.Web.Util; internal class DataBoundLiteralControlBuilder : ControlBuilder { internal DataBoundLiteralControlBuilder() { } internal void AddLiteralString(string s) { Debug.Assert(!InDesigner, "!InDesigner"); // Make sure strings and databinding expressions alternate object lastBuilder = GetLastBuilder(); if (lastBuilder != null && lastBuilder is string) { AddSubBuilder(null); } AddSubBuilder(s); } internal void AddDataBindingExpression(CodeBlockBuilder codeBlockBuilder) { Debug.Assert(!InDesigner, "!InDesigner"); // Make sure strings and databinding expressions alternate object lastBuilder = GetLastBuilder(); if (lastBuilder == null || lastBuilder is CodeBlockBuilder) { AddSubBuilder(null); } AddSubBuilder(codeBlockBuilder); } internal int GetStaticLiteralsCount() { // it's divided by 2 because half are strings and half are databinding // expressions). '+1' because we always start with a literal string. return (SubBuilders.Count+1) / 2; } internal int GetDataBoundLiteralCount() { // it's divided by 2 because half are strings and half are databinding // expressions) return SubBuilders.Count / 2; } } ////// [ ToolboxItem(false) ] public sealed class DataBoundLiteralControl : Control, ITextControl { private string[] _staticLiterals; private string[] _dataBoundLiteral; private bool _hasDataBoundStrings; ///Defines the properties and methods of the DataBoundLiteralControl class. ///public DataBoundLiteralControl(int staticLiteralsCount, int dataBoundLiteralCount) { _staticLiterals = new string[staticLiteralsCount]; _dataBoundLiteral = new string[dataBoundLiteralCount]; PreventAutoID(); } /// public void SetStaticString(int index, string s) { _staticLiterals[index] = s; } /// public void SetDataBoundString(int index, string s) { _dataBoundLiteral[index] = s; _hasDataBoundStrings = true; } /// /// public string Text { get { StringBuilder sb = new StringBuilder(); int dataBoundLiteralCount = _dataBoundLiteral.Length; // Append literal and databound strings alternatively for (int i=0; i<_staticLiterals.Length; i++) { if (_staticLiterals[i] != null) sb.Append(_staticLiterals[i]); // Could be null if DataBind() was not called if (i < dataBoundLiteralCount && _dataBoundLiteral[i] != null) sb.Append(_dataBoundLiteral[i]); } return sb.ToString(); } } ///Gets the text content of the data-bound literal control. ///protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } /// /// /// protected override void LoadViewState(object savedState) { if (savedState != null) { _dataBoundLiteral = (string[]) savedState; _hasDataBoundStrings = true; } } ///Loads the previously saved state. Overridden to synchronize Text property with /// LiteralContent. ////// /// protected override object SaveViewState() { // Return null if we didn't get any databound strings if (!_hasDataBoundStrings) return null; // Only save the databound literals to the view state return _dataBoundLiteral; } ///The object that contains the state changes. ///protected internal override void Render(HtmlTextWriter output) { int dataBoundLiteralCount = _dataBoundLiteral.Length; // Render literal and databound strings alternatively for (int i=0; i<_staticLiterals.Length; i++) { if (_staticLiterals[i] != null) output.Write(_staticLiterals[i]); // Could be null if DataBind() was not called if (i < dataBoundLiteralCount && _dataBoundLiteral[i] != null) output.Write(_dataBoundLiteral[i]); } } /// /// /// string ITextControl.Text { get { return Text; } set { throw new NotSupportedException(); } } } ///Implementation of TextControl.Text property. ////// [ DataBindingHandler("System.Web.UI.Design.TextDataBindingHandler, " + AssemblyRef.SystemDesign), ToolboxItem(false) ] public sealed class DesignerDataBoundLiteralControl : Control { private string _text; public DesignerDataBoundLiteralControl() { PreventAutoID(); } ///Simpler version of DataBoundLiteralControlBuilder, used at design time. ////// public string Text { get { return _text; } set { _text = (value != null) ? value : String.Empty; } } protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } ///Gets or sets the text content of the data-bound literal control. ////// protected override void LoadViewState(object savedState) { if (savedState != null) _text = (string) savedState; } ///Loads the previously saved state. Overridden to synchronize Text property with /// LiteralContent. ////// protected internal override void Render(HtmlTextWriter output) { output.Write(_text); } ///Saves any state that was modified after the control began monitoring state changes. ////// protected override object SaveViewState() { return _text; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.The object that contains the state changes. ///
Link Menu
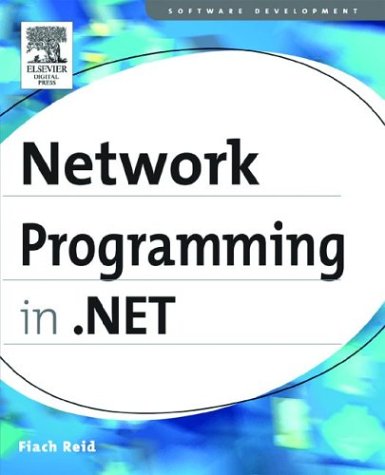
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WorkflowMessageEventHandler.cs
- TransactedBatchingBehavior.cs
- StylusShape.cs
- NavigationHelper.cs
- LinqDataSourceValidationException.cs
- TextAdaptor.cs
- HtmlSelect.cs
- PermissionListSet.cs
- DynamicDiscoveryDocument.cs
- DisplayToken.cs
- MsmqReceiveHelper.cs
- FloaterBaseParagraph.cs
- DeferredSelectedIndexReference.cs
- ComponentConverter.cs
- ParallelEnumerableWrapper.cs
- BaseCollection.cs
- CodeAccessPermission.cs
- WindowsTreeView.cs
- IChannel.cs
- SplitContainer.cs
- UIElement3D.cs
- PagePropertiesChangingEventArgs.cs
- RecognizedPhrase.cs
- CodeCompiler.cs
- SerializationAttributes.cs
- SplineKeyFrames.cs
- ObjectTag.cs
- Fault.cs
- EventPropertyMap.cs
- SqlCacheDependency.cs
- RoleGroup.cs
- StorageAssociationTypeMapping.cs
- BinaryConverter.cs
- CompressEmulationStream.cs
- WindowsToolbarItemAsMenuItem.cs
- CompiledXpathExpr.cs
- XpsPackagingPolicy.cs
- CommandSet.cs
- ToggleButtonAutomationPeer.cs
- ResXResourceWriter.cs
- InvalidProgramException.cs
- RelationshipType.cs
- OrderedDictionary.cs
- CompilerState.cs
- MetadataConversionError.cs
- OdbcError.cs
- UriScheme.cs
- UnmanagedBitmapWrapper.cs
- SQLCharsStorage.cs
- BroadcastEventHelper.cs
- InheritanceContextHelper.cs
- SqlDataAdapter.cs
- IntegerValidatorAttribute.cs
- EntitySetBaseCollection.cs
- AxHostDesigner.cs
- SizeAnimationBase.cs
- VersionedStream.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- XmlSerializationGeneratedCode.cs
- RoleService.cs
- DbMetaDataColumnNames.cs
- ReflectionUtil.cs
- ProcessInputEventArgs.cs
- LinkLabelLinkClickedEvent.cs
- XDeferredAxisSource.cs
- RewritingPass.cs
- XmlElement.cs
- PageRequestManager.cs
- ProxyAttribute.cs
- Zone.cs
- WebPartConnection.cs
- ContainerCodeDomSerializer.cs
- PagedDataSource.cs
- WebResponse.cs
- Point3DCollection.cs
- SettingsPropertyIsReadOnlyException.cs
- UIElement.cs
- XMLSyntaxException.cs
- SkipStoryboardToFill.cs
- SortableBindingList.cs
- XmlUrlEditor.cs
- ElapsedEventArgs.cs
- PriorityRange.cs
- listitem.cs
- EventMap.cs
- StretchValidation.cs
- TemplateBindingExtensionConverter.cs
- InvalidOperationException.cs
- ControlAdapter.cs
- SafeNativeMethodsCLR.cs
- SharedUtils.cs
- GenericPrincipal.cs
- SubtreeProcessor.cs
- DefaultTraceListener.cs
- OleDbRowUpdatingEvent.cs
- SkewTransform.cs
- SimpleType.cs
- DetailsViewPageEventArgs.cs
- StringConcat.cs
- DataBindingList.cs