Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Expressions / New.cs / 1305376 / New.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Expressions { using System.Activities; using System.Collections.ObjectModel; using System.Diagnostics.CodeAnalysis; using System.Reflection; using System.Runtime; using System.Runtime.Collections; using System.Windows.Markup; [SuppressMessage(FxCop.Category.Naming, FxCop.Rule.IdentifiersShouldNotMatchKeywords, Justification = "Optimizing for XAML naming. VB imperative users will [] qualify (e.g. New [New])")] [SuppressMessage(FxCop.Category.Naming, FxCop.Rule.IdentifiersShouldNotHaveIncorrectSuffix, Justification = "Optimizing for XAML naming.")] [ContentProperty("Arguments")] public sealed class New: CodeActivity { Collection arguments; ConstructorInfo constructorInfo; public New() : base() { } [SuppressMessage(FxCop.Category.Naming, FxCop.Rule.PropertyNamesShouldNotMatchGetMethods, Justification = "Optimizing for XAML naming.")] public Collection Arguments { get { if (this.arguments == null) { this.arguments = new ValidatingCollection { // disallow null values OnAddValidationCallback = item => { if (item == null) { throw FxTrace.Exception.ArgumentNull("item"); } } }; } return this.arguments; } } protected override void CacheMetadata(CodeActivityMetadata metadata) { bool foundError = false; // Loop through each argument, validate it, and if validation // passed expose it to the metadata Type[] types = new Type[this.Arguments.Count]; for (int i = 0; i < this.Arguments.Count; i++) { Argument argument = this.Arguments[i]; if (argument == null || argument.Expression == null) { metadata.AddValidationError(SR.ArgumentRequired("Arguments", typeof(New ))); foundError = true; } else { RuntimeArgument runtimeArgument = new RuntimeArgument("Argument" + i, this.arguments[i].ArgumentType, this.arguments[i].Direction, true); metadata.Bind(this.arguments[i], runtimeArgument); metadata.AddArgument(runtimeArgument); types[i] = this.Arguments[i].Direction == ArgumentDirection.In ? this.Arguments[i].ArgumentType : this.Arguments[i].ArgumentType.MakeByRefType(); } } // If we didn't find any errors in the arguments then // we can look for an appropriate constructor. if (!foundError) { this.constructorInfo = typeof(TResult).GetConstructor(types); if (this.constructorInfo == null && (!typeof(TResult).IsValueType || types.Length > 0)) { metadata.AddValidationError(SR.ConstructorInfoNotFound(typeof(TResult).Name)); } } } protected override TResult Execute(CodeActivityContext context) { object[] objects = new object[this.Arguments.Count]; for (int i = 0; i < this.Arguments.Count; i++) { objects[i] = this.Arguments[i].Get(context); } TResult result; if (this.constructorInfo != null) { result = (TResult)this.constructorInfo.Invoke(objects); } else { Fx.Assert(typeof(TResult).IsValueType, "The type of '" + typeof(TResult).Name + "' must be a value type."); Fx.Assert(this.Arguments.Count == 0, "The number of argument must be zero."); result = (TResult)Activator.CreateInstance(typeof(TResult)); } for (int i = 0; i < this.Arguments.Count; i++) { Argument argument = this.Arguments[i]; if (argument.Direction == ArgumentDirection.InOut || argument.Direction == ArgumentDirection.Out) { argument.Set(context, objects[i]); } } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
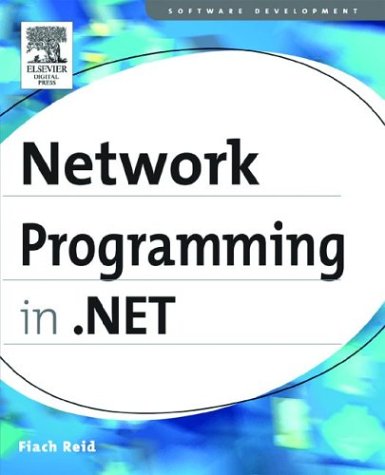
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GeometryGroup.cs
- CheckoutException.cs
- DeferrableContent.cs
- UserNameSecurityToken.cs
- CodeDesigner.cs
- TreeNode.cs
- DesignerRegion.cs
- SQLBoolean.cs
- ProtocolsConfigurationEntry.cs
- FileDialog_Vista.cs
- SoapAttributes.cs
- WeakReference.cs
- BindStream.cs
- EventProviderWriter.cs
- DeferredReference.cs
- PropertyMetadata.cs
- TextElement.cs
- ScriptHandlerFactory.cs
- StringSorter.cs
- DerivedKeySecurityTokenStub.cs
- SkipStoryboardToFill.cs
- WebPartConnectionsCloseVerb.cs
- AudioFormatConverter.cs
- WmlPanelAdapter.cs
- TextFormatterImp.cs
- MultiSelectRootGridEntry.cs
- ParenthesizePropertyNameAttribute.cs
- SystemException.cs
- SequentialActivityDesigner.cs
- PeerNode.cs
- RelatedImageListAttribute.cs
- BitmapEffectInput.cs
- SendKeys.cs
- MarkupObject.cs
- TraceShell.cs
- WebPartVerbsEventArgs.cs
- CompositeTypefaceMetrics.cs
- DragEventArgs.cs
- Variant.cs
- PathTooLongException.cs
- OdbcConnectionFactory.cs
- Win32SafeHandles.cs
- HatchBrush.cs
- ToolStripControlHost.cs
- DropDownList.cs
- DependencyObjectType.cs
- DependencyObjectType.cs
- ProfileManager.cs
- FontFamily.cs
- RepeaterItemCollection.cs
- XmlSchemas.cs
- ArgumentElement.cs
- WebPartConnectionsCloseVerb.cs
- InkCanvasAutomationPeer.cs
- HitTestFilterBehavior.cs
- odbcmetadatacolumnnames.cs
- ScriptReferenceBase.cs
- DataTableReader.cs
- ValueTypeFixupInfo.cs
- GACIdentityPermission.cs
- ComponentEditorPage.cs
- CounterSampleCalculator.cs
- ObjectCacheSettings.cs
- ResXDataNode.cs
- PositiveTimeSpanValidator.cs
- PathGeometry.cs
- InvalidProgramException.cs
- UnauthorizedAccessException.cs
- MsmqMessageSerializationFormat.cs
- Funcletizer.cs
- ConnectionPoint.cs
- FileLoadException.cs
- ConfigurationConverterBase.cs
- GridViewDeletedEventArgs.cs
- ObjectIDGenerator.cs
- Int16KeyFrameCollection.cs
- DefaultAssemblyResolver.cs
- followingquery.cs
- TextParagraphProperties.cs
- Transactions.cs
- DataKey.cs
- SoapSchemaExporter.cs
- DataGridViewComboBoxEditingControl.cs
- TextContainerHelper.cs
- PropertyInfoSet.cs
- TextSerializer.cs
- QuadraticBezierSegment.cs
- RuntimeHelpers.cs
- StringFunctions.cs
- Timeline.cs
- SoapAttributeOverrides.cs
- PrivilegeNotHeldException.cs
- Int64Animation.cs
- ObjectDataSourceSelectingEventArgs.cs
- PeerContact.cs
- TreeView.cs
- _ChunkParse.cs
- AuthenticatingEventArgs.cs
- SchemaNames.cs
- UdpConstants.cs