Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / NullableDecimalSumAggregationOperator.cs / 1305376 / NullableDecimalSumAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableDecimalSumAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for nullable decimals. /// internal sealed class NullableDecimalSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal NullableDecimalSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override decimal? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. decimal sum = 0.0m; while (enumerator.MoveNext()) { sum += enumerator.Current.GetValueOrDefault(); } return sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableDecimalSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableDecimalSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableDecimalSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref decimal? currentElement) { decimal? element = default(decimal?); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. decimal tempSum = 0.0m; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); tempSum += element.GetValueOrDefault(); } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = new decimal?(tempSum); return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableDecimalSumAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for nullable decimals. /// internal sealed class NullableDecimalSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal NullableDecimalSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override decimal? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. decimal sum = 0.0m; while (enumerator.MoveNext()) { sum += enumerator.Current.GetValueOrDefault(); } return sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableDecimalSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableDecimalSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableDecimalSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref decimal? currentElement) { decimal? element = default(decimal?); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. decimal tempSum = 0.0m; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); tempSum += element.GetValueOrDefault(); } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = new decimal?(tempSum); return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
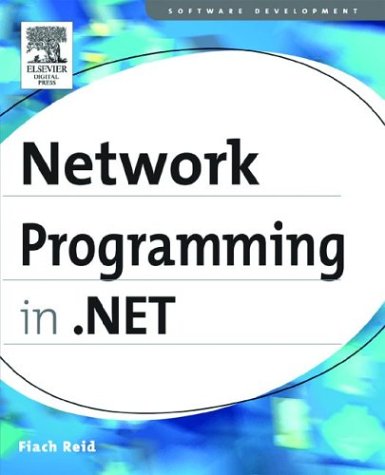
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeProcessHandle.cs
- ToolStripMenuItemDesigner.cs
- BaseValidatorDesigner.cs
- DefinitionUpdate.cs
- SoapIncludeAttribute.cs
- Panel.cs
- HttpServerProtocol.cs
- Comparer.cs
- LongSumAggregationOperator.cs
- TimelineGroup.cs
- ActivationArguments.cs
- SetIndexBinder.cs
- XMLUtil.cs
- CombinedTcpChannel.cs
- ExpandCollapseProviderWrapper.cs
- Quaternion.cs
- XmlWellformedWriter.cs
- SequenceRangeCollection.cs
- ScalarType.cs
- State.cs
- ListViewDataItem.cs
- CellLabel.cs
- BrowserCapabilitiesCompiler.cs
- ReadOnlyDataSource.cs
- Publisher.cs
- StyleSelector.cs
- SHA384Managed.cs
- RequestCache.cs
- TableRow.cs
- CodeCommentStatementCollection.cs
- HandleCollector.cs
- DataGridViewToolTip.cs
- Msec.cs
- StringArrayConverter.cs
- TemplateControl.cs
- ParameterBuilder.cs
- PrincipalPermission.cs
- ListSortDescriptionCollection.cs
- XPathDocumentBuilder.cs
- UpDownEvent.cs
- TypeDescriptor.cs
- Underline.cs
- Effect.cs
- FontCacheLogic.cs
- EFAssociationProvider.cs
- AttachmentCollection.cs
- ListItem.cs
- SiteMap.cs
- Blend.cs
- NavigatingCancelEventArgs.cs
- InstanceData.cs
- DrawingImage.cs
- ResourceBinder.cs
- FixedSOMPageConstructor.cs
- ActivityTypeCodeDomSerializer.cs
- SafeHandles.cs
- CodeIdentifier.cs
- FtpCachePolicyElement.cs
- XmlSerializerVersionAttribute.cs
- SqlAggregateChecker.cs
- ConfigurationHandlersInstallComponent.cs
- SourceFileBuildProvider.cs
- XD.cs
- ProvideValueServiceProvider.cs
- InvalidFilterCriteriaException.cs
- SQLInt32Storage.cs
- ErrorStyle.cs
- precedingquery.cs
- GlobalItem.cs
- ValueTypeIndexerReference.cs
- MimeReturn.cs
- QilScopedVisitor.cs
- DataServiceRequest.cs
- RecognizedPhrase.cs
- FrameworkTextComposition.cs
- CodeSpit.cs
- HttpRawResponse.cs
- GridViewCellAutomationPeer.cs
- xsdvalidator.cs
- ToolConsole.cs
- GridViewRowPresenter.cs
- DeviceOverridableAttribute.cs
- ZipIOLocalFileBlock.cs
- OrderByBuilder.cs
- RectangleGeometry.cs
- WindowsAuthenticationModule.cs
- ZipIOLocalFileBlock.cs
- BaseTypeViewSchema.cs
- DataGridItemCollection.cs
- OutputCacheSettings.cs
- invalidudtexception.cs
- LazyTextWriterCreator.cs
- KeyedCollection.cs
- cryptoapiTransform.cs
- SpanIndex.cs
- WebPartChrome.cs
- SoundPlayerAction.cs
- DefaultAuthorizationContext.cs
- WindowsSolidBrush.cs
- ManagedFilter.cs