Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / Automation / ElementProxy.cs / 1 / ElementProxy.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: UIAutomation/Visual bridge // // History: // 07/15/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; using System.Diagnostics; using System.Windows.Input; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; using MS.Win32; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Automation { // Automation Proxy for WCP elements - exposes WCP tree // and properties to Automation. // // Currently builds tree based on Visual tree; many later incorporate // parts of logical tree. // // Currently exposes just BoundingRectangle, ClassName, IsEnabled // and IsKeyboardFocused properties. internal class ElementProxy: IRawElementProviderFragmentRoot, IRawElementProviderAdviseEvents { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // private ctor - the Wrap() pseudo-ctor is used instead. private ElementProxy(AutomationPeer peer) { _peer = peer; } #endregion Constructors //------------------------------------------------------ // // Interface IRawElementProviderFragmentRoot // //------------ ------------------------------------------ #region Interface IRawElementProviderFragmentRoot // Implementation of the interface that exposes this // to UIAutomation. // // Interface IRawElementProviderFragmentRoot 'derives' from // IRawElementProviderSimple and IRawElementProviderFragment, // methods from those appear first below. // // Most of the interface methods on this interface need // to get onto the Visual's context before they can access it, // so use Dispatcher.Invoke to call a private method (in the // private methods section of this class) that does the real work. // IRawElementProviderSimple methods... public object GetPatternProvider ( int pattern ) { return ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextGetPatternProvider ), pattern); } public object GetPropertyValue(int property) { return ElementUtil.Invoke(_peer, new DispatcherOperationCallback( InContextGetPropertyValue ), property); } public ProviderOptions ProviderOptions { get { return (ProviderOptions)ElementUtil.Invoke(_peer, new DispatcherOperationCallback( InContextGetProviderOptions ), null); } } public IRawElementProviderSimple HostRawElementProvider { get { IRawElementProviderSimple host = null; HostedWindowWrapper hwndWrapper = null; hwndWrapper = (HostedWindowWrapper)ElementUtil.Invoke( _peer, new DispatcherOperationCallback(InContextGetHostRawElementProvider), null); if(hwndWrapper != null) host = GetHostHelper(hwndWrapper); return host; } } ////// Critical - Calls critical HostedWindowWrapper.Handle. /// TreatAsSafe - Critical data is used internally and not explosed /// [SecurityCritical, SecurityTreatAsSafe] private IRawElementProviderSimple GetHostHelper(HostedWindowWrapper hwndWrapper) { return AutomationInteropProvider.HostProviderFromHandle(hwndWrapper.Handle); } // IRawElementProviderFragment methods... public IRawElementProviderFragment Navigate( NavigateDirection direction ) { return (IRawElementProviderFragment)ElementUtil.Invoke(_peer, new DispatcherOperationCallback(InContextNavigate), direction); } public int [ ] GetRuntimeId() { return (int []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextGetRuntimeId ), null ); } public Rect BoundingRectangle { get { return (Rect)ElementUtil.Invoke(_peer, new DispatcherOperationCallback(InContextBoundingRectangle), null); } } public IRawElementProviderSimple [] GetEmbeddedFragmentRoots() { return null; } public void SetFocus() { ElementUtil.Invoke(_peer, new DispatcherOperationCallback( InContextSetFocus ), null); } public IRawElementProviderFragmentRoot FragmentRoot { get { return (IRawElementProviderFragmentRoot) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextFragmentRoot ), null ); } } // IRawElementProviderFragmentRoot methods.. public IRawElementProviderFragment ElementProviderFromPoint( double x, double y ) { return (IRawElementProviderFragment) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextElementProviderFromPoint ), new Point( x, y ) ); } public IRawElementProviderFragment GetFocus() { return (IRawElementProviderFragment) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextGetFocus ), null ); } // Event support... public void AdviseEventAdded(int eventID, int[] properties) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextAdviseEventAdded ), new object [] { eventID, properties } ); } public void AdviseEventRemoved(int eventID, int[] properties) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextAdviseEventRemoved ), new object [] { eventID, properties } ); } #endregion Interface IRawElementProviderFragmentRoot //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods // Wrap the found peer before shipping it over process boundary. private ElementProxy Wrap(AutomationPeer peer) { //_peer is well-connected, since UIA is asking us using it, //so it's ok to pass it as the referencePeer to StaticWrap return StaticWrap(peer, _peer); } // Wrap the found peer before shipping it over process boundary - static version for use outside ElementProxy. internal static ElementProxy StaticWrap(AutomationPeer peer, AutomationPeer referencePeer) { ElementProxy result = null; if (peer != null) { //referencePeer is well-connected, since UIA is asking us using it. //However we are trying to return the peer that is possibly just created on some //element in the middle of un-walked tree and we need to make sure it is fully //"connected" or initialized before we return it to UIA. //This method ensures it has the right _parent and other hookup. peer = peer.ValidateConnected(referencePeer); if (peer != null) { result = new ElementProxy(peer); } } return result; } // Needed to enable access from AutomationPeer.PeerFromProvider internal AutomationPeer Peer { get { return _peer; } } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods // The signature of most of the folling methods is "object func( object arg )", // since that's what the Conmtext.Invoke delegate requires. // Return the element at specified coords. private object InContextElementProviderFromPoint( object arg ) { Point point = (Point)arg; AutomationPeer peer = _peer.GetPeerFromPoint(point); return Wrap(peer); } // Return proxy representing currently focused element (if any) private object InContextGetFocus( object unused ) { // AutomationPeer peer = AutomationPeer.AutomationPeerFromInputElement(Keyboard.FocusedElement); return Wrap(peer); } // redirect to AutomationPeer private object InContextGetPatternProvider(object arg) { return _peer.GetWrappedPattern((int)arg); } // Return proxy representing element in specified direction (parent/next/firstchild/etc.) private object InContextNavigate( object arg ) { NavigateDirection direction = (NavigateDirection) arg; AutomationPeer dest; switch( direction ) { case NavigateDirection.Parent: dest = _peer.GetParent(); break; case NavigateDirection.FirstChild: if (!_peer.IsInteropPeer) { dest = _peer.GetFirstChild(); } else { return _peer.GetInteropChild(); } break; case NavigateDirection.LastChild: if (!_peer.IsInteropPeer) { dest = _peer.GetLastChild(); } else { return _peer.GetInteropChild(); } break; case NavigateDirection.NextSibling: dest = _peer.GetNextSibling(); break; case NavigateDirection.PreviousSibling: dest = _peer.GetPreviousSibling(); break; default: dest = null; break; } return Wrap(dest); } // Return value for specified property; or null if not supported private object InContextGetProviderOptions( object arg ) { ProviderOptions options = ProviderOptions.ServerSideProvider; if(_peer.IsHwndHost) options |= ProviderOptions.OverrideProvider; return options; } // Return value for specified property; or null if not supported private object InContextGetPropertyValue ( object arg ) { return _peer.GetPropertyValue((int)arg); } /// Returns whether this is the Root of the WCP tree or not private object InContextGetHostRawElementProvider( object unused ) { return _peer.GetHostRawElementProvider(); } // Return unique ID for this element... private object InContextGetRuntimeId( object unused ) { return _peer.GetRuntimeId(); } // Return bounding rectangle (screen coords) for this element... private object InContextBoundingRectangle(object unused) { return _peer.GetBoundingRectangle(); } // Set focus to this element... private object InContextSetFocus( object unused ) { _peer.SetFocus(); return null; } // Return proxy representing the root of this WCP tree... private object InContextFragmentRoot( object unused ) { AutomationPeer root = _peer; while(true) { AutomationPeer parent = root.GetParent(); if(parent == null) break; root = parent; } return Wrap(root); } private object InContextAdviseEventAdded(object arg) { object [] args = (object [])arg; int eventId = (int)args[0]; EventMap.AddEvent(eventId); return null; } private object InContextAdviseEventRemoved(object arg) { object [] args = (object [])arg; int eventId = (int)args[0]; EventMap.RemoveEvent(eventId); return null; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: UIAutomation/Visual bridge // // History: // 07/15/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; using System.Diagnostics; using System.Windows.Input; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; using MS.Win32; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Automation { // Automation Proxy for WCP elements - exposes WCP tree // and properties to Automation. // // Currently builds tree based on Visual tree; many later incorporate // parts of logical tree. // // Currently exposes just BoundingRectangle, ClassName, IsEnabled // and IsKeyboardFocused properties. internal class ElementProxy: IRawElementProviderFragmentRoot, IRawElementProviderAdviseEvents { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // private ctor - the Wrap() pseudo-ctor is used instead. private ElementProxy(AutomationPeer peer) { _peer = peer; } #endregion Constructors //------------------------------------------------------ // // Interface IRawElementProviderFragmentRoot // //------------ ------------------------------------------ #region Interface IRawElementProviderFragmentRoot // Implementation of the interface that exposes this // to UIAutomation. // // Interface IRawElementProviderFragmentRoot 'derives' from // IRawElementProviderSimple and IRawElementProviderFragment, // methods from those appear first below. // // Most of the interface methods on this interface need // to get onto the Visual's context before they can access it, // so use Dispatcher.Invoke to call a private method (in the // private methods section of this class) that does the real work. // IRawElementProviderSimple methods... public object GetPatternProvider ( int pattern ) { return ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextGetPatternProvider ), pattern); } public object GetPropertyValue(int property) { return ElementUtil.Invoke(_peer, new DispatcherOperationCallback( InContextGetPropertyValue ), property); } public ProviderOptions ProviderOptions { get { return (ProviderOptions)ElementUtil.Invoke(_peer, new DispatcherOperationCallback( InContextGetProviderOptions ), null); } } public IRawElementProviderSimple HostRawElementProvider { get { IRawElementProviderSimple host = null; HostedWindowWrapper hwndWrapper = null; hwndWrapper = (HostedWindowWrapper)ElementUtil.Invoke( _peer, new DispatcherOperationCallback(InContextGetHostRawElementProvider), null); if(hwndWrapper != null) host = GetHostHelper(hwndWrapper); return host; } } ////// Critical - Calls critical HostedWindowWrapper.Handle. /// TreatAsSafe - Critical data is used internally and not explosed /// [SecurityCritical, SecurityTreatAsSafe] private IRawElementProviderSimple GetHostHelper(HostedWindowWrapper hwndWrapper) { return AutomationInteropProvider.HostProviderFromHandle(hwndWrapper.Handle); } // IRawElementProviderFragment methods... public IRawElementProviderFragment Navigate( NavigateDirection direction ) { return (IRawElementProviderFragment)ElementUtil.Invoke(_peer, new DispatcherOperationCallback(InContextNavigate), direction); } public int [ ] GetRuntimeId() { return (int []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextGetRuntimeId ), null ); } public Rect BoundingRectangle { get { return (Rect)ElementUtil.Invoke(_peer, new DispatcherOperationCallback(InContextBoundingRectangle), null); } } public IRawElementProviderSimple [] GetEmbeddedFragmentRoots() { return null; } public void SetFocus() { ElementUtil.Invoke(_peer, new DispatcherOperationCallback( InContextSetFocus ), null); } public IRawElementProviderFragmentRoot FragmentRoot { get { return (IRawElementProviderFragmentRoot) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextFragmentRoot ), null ); } } // IRawElementProviderFragmentRoot methods.. public IRawElementProviderFragment ElementProviderFromPoint( double x, double y ) { return (IRawElementProviderFragment) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextElementProviderFromPoint ), new Point( x, y ) ); } public IRawElementProviderFragment GetFocus() { return (IRawElementProviderFragment) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextGetFocus ), null ); } // Event support... public void AdviseEventAdded(int eventID, int[] properties) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextAdviseEventAdded ), new object [] { eventID, properties } ); } public void AdviseEventRemoved(int eventID, int[] properties) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( InContextAdviseEventRemoved ), new object [] { eventID, properties } ); } #endregion Interface IRawElementProviderFragmentRoot //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods // Wrap the found peer before shipping it over process boundary. private ElementProxy Wrap(AutomationPeer peer) { //_peer is well-connected, since UIA is asking us using it, //so it's ok to pass it as the referencePeer to StaticWrap return StaticWrap(peer, _peer); } // Wrap the found peer before shipping it over process boundary - static version for use outside ElementProxy. internal static ElementProxy StaticWrap(AutomationPeer peer, AutomationPeer referencePeer) { ElementProxy result = null; if (peer != null) { //referencePeer is well-connected, since UIA is asking us using it. //However we are trying to return the peer that is possibly just created on some //element in the middle of un-walked tree and we need to make sure it is fully //"connected" or initialized before we return it to UIA. //This method ensures it has the right _parent and other hookup. peer = peer.ValidateConnected(referencePeer); if (peer != null) { result = new ElementProxy(peer); } } return result; } // Needed to enable access from AutomationPeer.PeerFromProvider internal AutomationPeer Peer { get { return _peer; } } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods // The signature of most of the folling methods is "object func( object arg )", // since that's what the Conmtext.Invoke delegate requires. // Return the element at specified coords. private object InContextElementProviderFromPoint( object arg ) { Point point = (Point)arg; AutomationPeer peer = _peer.GetPeerFromPoint(point); return Wrap(peer); } // Return proxy representing currently focused element (if any) private object InContextGetFocus( object unused ) { // AutomationPeer peer = AutomationPeer.AutomationPeerFromInputElement(Keyboard.FocusedElement); return Wrap(peer); } // redirect to AutomationPeer private object InContextGetPatternProvider(object arg) { return _peer.GetWrappedPattern((int)arg); } // Return proxy representing element in specified direction (parent/next/firstchild/etc.) private object InContextNavigate( object arg ) { NavigateDirection direction = (NavigateDirection) arg; AutomationPeer dest; switch( direction ) { case NavigateDirection.Parent: dest = _peer.GetParent(); break; case NavigateDirection.FirstChild: if (!_peer.IsInteropPeer) { dest = _peer.GetFirstChild(); } else { return _peer.GetInteropChild(); } break; case NavigateDirection.LastChild: if (!_peer.IsInteropPeer) { dest = _peer.GetLastChild(); } else { return _peer.GetInteropChild(); } break; case NavigateDirection.NextSibling: dest = _peer.GetNextSibling(); break; case NavigateDirection.PreviousSibling: dest = _peer.GetPreviousSibling(); break; default: dest = null; break; } return Wrap(dest); } // Return value for specified property; or null if not supported private object InContextGetProviderOptions( object arg ) { ProviderOptions options = ProviderOptions.ServerSideProvider; if(_peer.IsHwndHost) options |= ProviderOptions.OverrideProvider; return options; } // Return value for specified property; or null if not supported private object InContextGetPropertyValue ( object arg ) { return _peer.GetPropertyValue((int)arg); } /// Returns whether this is the Root of the WCP tree or not private object InContextGetHostRawElementProvider( object unused ) { return _peer.GetHostRawElementProvider(); } // Return unique ID for this element... private object InContextGetRuntimeId( object unused ) { return _peer.GetRuntimeId(); } // Return bounding rectangle (screen coords) for this element... private object InContextBoundingRectangle(object unused) { return _peer.GetBoundingRectangle(); } // Set focus to this element... private object InContextSetFocus( object unused ) { _peer.SetFocus(); return null; } // Return proxy representing the root of this WCP tree... private object InContextFragmentRoot( object unused ) { AutomationPeer root = _peer; while(true) { AutomationPeer parent = root.GetParent(); if(parent == null) break; root = parent; } return Wrap(root); } private object InContextAdviseEventAdded(object arg) { object [] args = (object [])arg; int eventId = (int)args[0]; EventMap.AddEvent(eventId); return null; } private object InContextAdviseEventRemoved(object arg) { object [] args = (object [])arg; int eventId = (int)args[0]; EventMap.RemoveEvent(eventId); return null; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
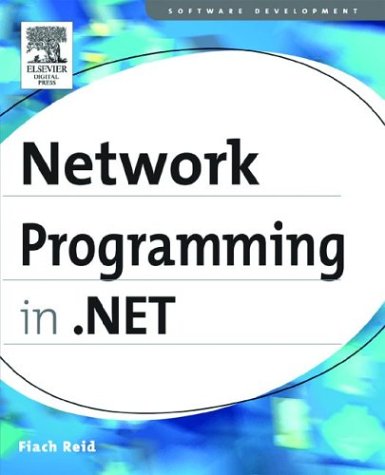
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyValidationContext.cs
- PingOptions.cs
- Geometry.cs
- QueryGenerator.cs
- oledbmetadatacollectionnames.cs
- AdditionalEntityFunctions.cs
- AppDomain.cs
- HttpHeaderCollection.cs
- OutputCacheSection.cs
- DataGridColumnEventArgs.cs
- XsltContext.cs
- DocumentStatusResources.cs
- SecurityProtocolFactory.cs
- SelectionPattern.cs
- ExpandCollapseProviderWrapper.cs
- ThrowHelper.cs
- MethodToken.cs
- WindowsScroll.cs
- SHA384.cs
- ValidationUtility.cs
- PointHitTestParameters.cs
- ViewStateModeByIdAttribute.cs
- PresentationAppDomainManager.cs
- XmlWhitespace.cs
- TrackingStringDictionary.cs
- EmbeddedMailObject.cs
- UIServiceHelper.cs
- QilInvokeLateBound.cs
- DefaultPrintController.cs
- InfoCardSymmetricCrypto.cs
- ListenerChannelContext.cs
- EntityDataSourceReferenceGroup.cs
- TrackPointCollection.cs
- XPathEmptyIterator.cs
- DATA_BLOB.cs
- X509CertificateCollection.cs
- NamedPipeProcessProtocolHandler.cs
- JsonStringDataContract.cs
- SecurityKeyType.cs
- RangeContentEnumerator.cs
- MapPathBasedVirtualPathProvider.cs
- WebEventCodes.cs
- SmiGettersStream.cs
- XmlResolver.cs
- InputQueue.cs
- DispatcherFrame.cs
- DataRelationPropertyDescriptor.cs
- ListControlActionList.cs
- ArraySubsetEnumerator.cs
- MutexSecurity.cs
- UrlMappingsModule.cs
- FamilyMap.cs
- NetworkInformationPermission.cs
- RTTypeWrapper.cs
- TextOnlyOutput.cs
- DispatcherExceptionFilterEventArgs.cs
- EntityStoreSchemaGenerator.cs
- DefaultMemberAttribute.cs
- RepeatButton.cs
- smtpconnection.cs
- TreeBuilder.cs
- ConfigurationSchemaErrors.cs
- VerticalConnector.xaml.cs
- AssemblyNameProxy.cs
- PathFigure.cs
- ListViewGroup.cs
- IndexedString.cs
- StateDesignerConnector.cs
- FormClosedEvent.cs
- DEREncoding.cs
- StylusPointCollection.cs
- DefaultMemberAttribute.cs
- IgnoreFlushAndCloseStream.cs
- SoapHeaders.cs
- RadioButton.cs
- PropertyGroupDescription.cs
- WeakReferenceList.cs
- ResourceBinder.cs
- SocketException.cs
- ProfileGroupSettingsCollection.cs
- WindowsPen.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- QuarticEase.cs
- Update.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- SqlBinder.cs
- StorageSetMapping.cs
- RequiredFieldValidator.cs
- PolicyStatement.cs
- TranslateTransform3D.cs
- XmlDataCollection.cs
- SimpleApplicationHost.cs
- ExpressionNormalizer.cs
- BoolExpression.cs
- FileInfo.cs
- EmissiveMaterial.cs
- SystemColors.cs
- PropertyToken.cs
- SequenceQuery.cs
- SecurityToken.cs