Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / IntSecurity.cs / 1 / IntSecurity.cs
namespace System.Web { using System.Web; using System.Web.Util; using System.Security; using System.Security.Permissions; internal static class InternalSecurityPermissions { private static IStackWalk _unrestricted; private static IStackWalk _unmanagedCode; #if UNUSED_CODE private static IStackWalk _sensitiveInformation; #endif private static IStackWalk _controlPrincipal; #if UNUSED_CODE private static IStackWalk _controlEvidence; #endif private static IStackWalk _reflection; private static IStackWalk _appPathDiscovery; private static IStackWalk _controlThread; #if UNUSED_CODE private static IStackWalk _levelMinimal; #endif private static IStackWalk _levelLow; private static IStackWalk _levelMedium; private static IStackWalk _levelHigh; #if UNUSED_CODE private static IStackWalk _levelUnrestricted; #endif // // Static permissions as properties, created on demand // internal static IStackWalk Unrestricted { get { if (_unrestricted == null) _unrestricted = new PermissionSet(PermissionState.Unrestricted); Debug.Trace("Permissions", "Unrestricted Set"); return _unrestricted; } } internal static IStackWalk UnmanagedCode { get { if (_unmanagedCode == null) _unmanagedCode = new SecurityPermission(SecurityPermissionFlag.UnmanagedCode); Debug.Trace("Permissions", "UnmanagedCode"); return _unmanagedCode; } } #if UNUSED_CODE internal static IStackWalk SensitiveInformation { get { if (_sensitiveInformation == null) _sensitiveInformation = new EnvironmentPermission(PermissionState.Unrestricted); Debug.Trace("Permissions", "SensitiveInformation"); return _sensitiveInformation; } } #endif internal static IStackWalk ControlPrincipal { get { if (_controlPrincipal == null) _controlPrincipal = new SecurityPermission(SecurityPermissionFlag.ControlPrincipal); Debug.Trace("Permissions", "ControlPrincipal"); return _controlPrincipal; } } #if UNUSED_CODE internal static IStackWalk ControlEvidence { get { if (_controlEvidence == null) _controlEvidence = new SecurityPermission(SecurityPermissionFlag.ControlEvidence); Debug.Trace("Permissions", "ControlEvidence"); return _controlEvidence; } } #endif internal static IStackWalk Reflection { get { if (_reflection == null) _reflection = new ReflectionPermission(ReflectionPermissionFlag.MemberAccess); Debug.Trace("Permissions", "Reflection"); return _reflection; } } internal static IStackWalk AppPathDiscovery { get { if (_appPathDiscovery == null) _appPathDiscovery = new FileIOPermission(FileIOPermissionAccess.PathDiscovery, HttpRuntime.AppDomainAppPathInternal); Debug.Trace("Permissions", "AppPathDiscovery"); return _appPathDiscovery; } } internal static IStackWalk ControlThread { get { if (_controlThread == null) _controlThread = new SecurityPermission(SecurityPermissionFlag.ControlThread); Debug.Trace("Permissions", "ControlThread"); return _controlThread; } } #if UNUSED_CODE internal static IStackWalk AspNetHostingPermissionLevelMinimal { get { if (_levelMinimal == null) _levelMinimal = new AspNetHostingPermission(AspNetHostingPermissionLevel.Minimal); Debug.Trace("Permissions", "AspNetHostingPermissionLevelMinimal"); return _levelMinimal; } } #endif internal static IStackWalk AspNetHostingPermissionLevelLow { get { if (_levelLow == null) _levelLow = new AspNetHostingPermission(AspNetHostingPermissionLevel.Low); Debug.Trace("Permissions", "AspNetHostingPermissionLevelLow"); return _levelLow; } } internal static IStackWalk AspNetHostingPermissionLevelMedium { get { if (_levelMedium == null) _levelMedium = new AspNetHostingPermission(AspNetHostingPermissionLevel.Medium); Debug.Trace("Permissions", "AspNetHostingPermissionLevelMedium"); return _levelMedium; } } internal static IStackWalk AspNetHostingPermissionLevelHigh { get { if (_levelHigh == null) _levelHigh = new AspNetHostingPermission(AspNetHostingPermissionLevel.High); Debug.Trace("Permissions", "AspNetHostingPermissionLevelHigh"); return _levelHigh; } } #if UNUSED_CODE internal static IStackWalk AspNetHostingPermissionLevelUnrestricted { get { if (_levelUnrestricted == null) _levelUnrestricted = new AspNetHostingPermission(AspNetHostingPermissionLevel.Unrestricted); Debug.Trace("Permissions", "AspNetHostingPermissionLevelUnrestricted"); return _levelUnrestricted; } } #endif // Parameterized permissions internal static IStackWalk FileReadAccess(String filename) { Debug.Trace("Permissions", "FileReadAccess(" + filename + ")"); return new FileIOPermission(FileIOPermissionAccess.Read, filename); } internal static IStackWalk PathDiscovery(String path) { Debug.Trace("Permissions", "PathDiscovery(" + path + ")"); return new FileIOPermission(FileIOPermissionAccess.PathDiscovery, path); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Web { using System.Web; using System.Web.Util; using System.Security; using System.Security.Permissions; internal static class InternalSecurityPermissions { private static IStackWalk _unrestricted; private static IStackWalk _unmanagedCode; #if UNUSED_CODE private static IStackWalk _sensitiveInformation; #endif private static IStackWalk _controlPrincipal; #if UNUSED_CODE private static IStackWalk _controlEvidence; #endif private static IStackWalk _reflection; private static IStackWalk _appPathDiscovery; private static IStackWalk _controlThread; #if UNUSED_CODE private static IStackWalk _levelMinimal; #endif private static IStackWalk _levelLow; private static IStackWalk _levelMedium; private static IStackWalk _levelHigh; #if UNUSED_CODE private static IStackWalk _levelUnrestricted; #endif // // Static permissions as properties, created on demand // internal static IStackWalk Unrestricted { get { if (_unrestricted == null) _unrestricted = new PermissionSet(PermissionState.Unrestricted); Debug.Trace("Permissions", "Unrestricted Set"); return _unrestricted; } } internal static IStackWalk UnmanagedCode { get { if (_unmanagedCode == null) _unmanagedCode = new SecurityPermission(SecurityPermissionFlag.UnmanagedCode); Debug.Trace("Permissions", "UnmanagedCode"); return _unmanagedCode; } } #if UNUSED_CODE internal static IStackWalk SensitiveInformation { get { if (_sensitiveInformation == null) _sensitiveInformation = new EnvironmentPermission(PermissionState.Unrestricted); Debug.Trace("Permissions", "SensitiveInformation"); return _sensitiveInformation; } } #endif internal static IStackWalk ControlPrincipal { get { if (_controlPrincipal == null) _controlPrincipal = new SecurityPermission(SecurityPermissionFlag.ControlPrincipal); Debug.Trace("Permissions", "ControlPrincipal"); return _controlPrincipal; } } #if UNUSED_CODE internal static IStackWalk ControlEvidence { get { if (_controlEvidence == null) _controlEvidence = new SecurityPermission(SecurityPermissionFlag.ControlEvidence); Debug.Trace("Permissions", "ControlEvidence"); return _controlEvidence; } } #endif internal static IStackWalk Reflection { get { if (_reflection == null) _reflection = new ReflectionPermission(ReflectionPermissionFlag.MemberAccess); Debug.Trace("Permissions", "Reflection"); return _reflection; } } internal static IStackWalk AppPathDiscovery { get { if (_appPathDiscovery == null) _appPathDiscovery = new FileIOPermission(FileIOPermissionAccess.PathDiscovery, HttpRuntime.AppDomainAppPathInternal); Debug.Trace("Permissions", "AppPathDiscovery"); return _appPathDiscovery; } } internal static IStackWalk ControlThread { get { if (_controlThread == null) _controlThread = new SecurityPermission(SecurityPermissionFlag.ControlThread); Debug.Trace("Permissions", "ControlThread"); return _controlThread; } } #if UNUSED_CODE internal static IStackWalk AspNetHostingPermissionLevelMinimal { get { if (_levelMinimal == null) _levelMinimal = new AspNetHostingPermission(AspNetHostingPermissionLevel.Minimal); Debug.Trace("Permissions", "AspNetHostingPermissionLevelMinimal"); return _levelMinimal; } } #endif internal static IStackWalk AspNetHostingPermissionLevelLow { get { if (_levelLow == null) _levelLow = new AspNetHostingPermission(AspNetHostingPermissionLevel.Low); Debug.Trace("Permissions", "AspNetHostingPermissionLevelLow"); return _levelLow; } } internal static IStackWalk AspNetHostingPermissionLevelMedium { get { if (_levelMedium == null) _levelMedium = new AspNetHostingPermission(AspNetHostingPermissionLevel.Medium); Debug.Trace("Permissions", "AspNetHostingPermissionLevelMedium"); return _levelMedium; } } internal static IStackWalk AspNetHostingPermissionLevelHigh { get { if (_levelHigh == null) _levelHigh = new AspNetHostingPermission(AspNetHostingPermissionLevel.High); Debug.Trace("Permissions", "AspNetHostingPermissionLevelHigh"); return _levelHigh; } } #if UNUSED_CODE internal static IStackWalk AspNetHostingPermissionLevelUnrestricted { get { if (_levelUnrestricted == null) _levelUnrestricted = new AspNetHostingPermission(AspNetHostingPermissionLevel.Unrestricted); Debug.Trace("Permissions", "AspNetHostingPermissionLevelUnrestricted"); return _levelUnrestricted; } } #endif // Parameterized permissions internal static IStackWalk FileReadAccess(String filename) { Debug.Trace("Permissions", "FileReadAccess(" + filename + ")"); return new FileIOPermission(FileIOPermissionAccess.Read, filename); } internal static IStackWalk PathDiscovery(String path) { Debug.Trace("Permissions", "PathDiscovery(" + path + ")"); return new FileIOPermission(FileIOPermissionAccess.PathDiscovery, path); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
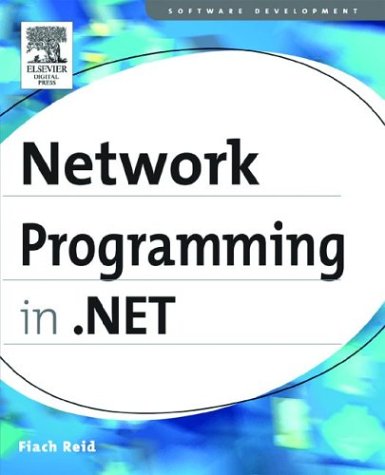
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpanderAutomationPeer.cs
- PartEditor.cs
- WebBrowsableAttribute.cs
- returneventsaver.cs
- XmlILOptimizerVisitor.cs
- DbDataAdapter.cs
- TdsParserSafeHandles.cs
- ToolStripRendererSwitcher.cs
- X509Chain.cs
- BooleanExpr.cs
- CultureInfoConverter.cs
- AdapterUtil.cs
- RemotingConfigParser.cs
- DesignTimeParseData.cs
- WinEventTracker.cs
- Propagator.JoinPropagator.cs
- StatusBar.cs
- AspNetHostingPermission.cs
- objectquery_tresulttype.cs
- OleDbConnectionFactory.cs
- GCHandleCookieTable.cs
- CompositeActivityValidator.cs
- SynchronizingStream.cs
- OdbcParameterCollection.cs
- SelectorAutomationPeer.cs
- safesecurityhelperavalon.cs
- CollectionContainer.cs
- initElementDictionary.cs
- SecurityContextTokenCache.cs
- MaskedTextBox.cs
- DockProviderWrapper.cs
- OperatorExpressions.cs
- CodeTypeDeclaration.cs
- HighContrastHelper.cs
- ParentUndoUnit.cs
- DeriveBytes.cs
- PropertyBuilder.cs
- DataControlButton.cs
- GlobalDataBindingHandler.cs
- AbsoluteQuery.cs
- XmlUrlResolver.cs
- SpecialNameAttribute.cs
- NativeMethods.cs
- ToolZone.cs
- CalendarDay.cs
- LocalsItemDescription.cs
- ObjectCacheSettings.cs
- SweepDirectionValidation.cs
- ReflectionUtil.cs
- QilCloneVisitor.cs
- AssemblyNameProxy.cs
- DeleteCardRequest.cs
- DataGridViewImageColumn.cs
- GAC.cs
- FontCacheLogic.cs
- XmlQueryType.cs
- EventLogTraceListener.cs
- TemplatedControlDesigner.cs
- StdValidatorsAndConverters.cs
- ScaleTransform.cs
- AssemblyBuilder.cs
- HostedHttpTransportManager.cs
- CodeSnippetExpression.cs
- Point.cs
- PairComparer.cs
- MemberInitExpression.cs
- ConfigurationFileMap.cs
- CngUIPolicy.cs
- MatrixTransform.cs
- GenericWebPart.cs
- WindowsIdentity.cs
- LassoSelectionBehavior.cs
- TimersDescriptionAttribute.cs
- ParameterModifier.cs
- XmlSignificantWhitespace.cs
- ITextView.cs
- ConfigXmlDocument.cs
- CacheForPrimitiveTypes.cs
- StrongName.cs
- Verify.cs
- ServicesUtilities.cs
- FirstQueryOperator.cs
- AnchoredBlock.cs
- DynamicQueryableWrapper.cs
- HTMLTagNameToTypeMapper.cs
- Dynamic.cs
- CommandConverter.cs
- WebPartConnection.cs
- XDRSchema.cs
- ResourcePermissionBase.cs
- Brushes.cs
- PublishLicense.cs
- IntermediatePolicyValidator.cs
- XmlSchemaSimpleTypeUnion.cs
- thaishape.cs
- SrgsNameValueTag.cs
- ComplexPropertyEntry.cs
- UnhandledExceptionEventArgs.cs
- DefaultProxySection.cs
- HttpValueCollection.cs