Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / AppModel / returneventsaver.cs / 1305600 / returneventsaver.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class provides a convenient way to persist/depersist the events on a PageFunction // // By calling the _Detach() method on a pagefunction, // this class will build a list of the class & methods on that Pagefunction, // as well as removing the current listener on the class when it's done. // // By passing in a pagefunction on the _Attach method, the class will reattach the // saved list to the calling pagefunction // // History: // 06/11/03: marka created // //--------------------------------------------------------------------------- using System; using System.Windows.Navigation; using System.Windows; using System.Diagnostics; using System.Collections; using System.Reflection; using System.IO; using System.Security.Permissions; using System.Security; namespace MS.Internal.AppModel { [Serializable] internal struct ReturnEventSaverInfo { internal ReturnEventSaverInfo(string delegateTypeName, string targetTypeName, string delegateMethodName, bool fSamePf) { _delegateTypeName = delegateTypeName; _targetTypeName = targetTypeName; _delegateMethodName = delegateMethodName; _delegateInSamePF = fSamePf; } internal String _delegateTypeName; internal String _targetTypeName; internal String _delegateMethodName; internal bool _delegateInSamePF; // Return Event handler comes from the same pagefunction, this is for non-generic workaround. } [Serializable] internal class ReturnEventSaver { internal ReturnEventSaver() { } ////// Critical - sets the critical _returnList. /// TreatAsSafe - _returnList is not exposed in any way. /// [SecurityCritical, SecurityTreatAsSafe] internal void _Detach(PageFunctionBase pf) { if (pf._Return != null && pf._Saver == null) { ReturnEventSaverInfo[] list = null; Delegate[] delegates = null; delegates = (pf._Return).GetInvocationList(); list = _returnList = new ReturnEventSaverInfo[delegates.Length]; for (int i = 0; i < delegates.Length; i++) { Delegate returnDelegate = delegates[i]; bool bSamePf = false; if (returnDelegate.Target == pf) { // This is the Event Handler implemented by the same PF, use for NonGeneric handling. bSamePf = true; } MethodInfo m = returnDelegate.Method; ReturnEventSaverInfo info = new ReturnEventSaverInfo( returnDelegate.GetType().AssemblyQualifiedName, returnDelegate.Target.GetType().AssemblyQualifiedName, m.Name, bSamePf); list[i] = info; } // // only save if there were delegates already attached. // note that there will be cases where the Saver has already been pre-populated from a Load // but no delegates have been created yet ( as the PF hasn`t called finish as yet) // // By only storing the saver once there are delegates - we avoid the problem of // wiping out any newly restored saver pf._Saver = this; } pf._DetachEvents(); } // // Attach the stored events to the supplied pagefunction. // // caller - the Calling Page's root element. We will reattach events *from* this page root element *to* the child // // child - the child PageFunction. Caller was originally attached to child, we're now reattaching *to* the child // ////// Critical - Asserts ReflectionPermission to be able re-create delegate to private method. /// TreatAsSafe - The delegate created is identical to the one that _Detach() received from /// the application and saved. This is ensured by matching the type of the original target /// object against the type of the new target. Thus we know that the application was able /// to create a delegate over the exact method, and even if that method had a LinkDemand, /// it was satisfied by the application. /// [SecurityCritical, SecurityTreatAsSafe] internal void _Attach(Object caller, PageFunctionBase child) { ReturnEventSaverInfo[] list = null; list = _returnList; if (list != null) { Debug.Assert(caller != null, "Caller should not be null"); for (int i = 0; i < list.Length; i++) { // // if (string.Compare(_returnList[i]._targetTypeName, caller.GetType().AssemblyQualifiedName, StringComparison.Ordinal) != 0) { throw new NotSupportedException(SR.Get(SRID.ReturnEventHandlerMustBeOnParentPage)); } Delegate d; try { new ReflectionPermission(ReflectionPermissionFlag.MemberAccess).Assert(); // BlessedAssert d = Delegate.CreateDelegate( Type.GetType(_returnList[i]._delegateTypeName), caller, _returnList[i]._delegateMethodName); } catch (Exception ex) { throw new NotSupportedException(SR.Get(SRID.ReturnEventHandlerMustBeOnParentPage), ex); } finally { ReflectionPermission.RevertAssert(); } child._AddEventHandler(d); } } } ////// Critical: contains metadata for delegates created under elevation. /// [SecurityCritical] private ReturnEventSaverInfo[] _returnList; // The list of delegates we want to persist and return later } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class provides a convenient way to persist/depersist the events on a PageFunction // // By calling the _Detach() method on a pagefunction, // this class will build a list of the class & methods on that Pagefunction, // as well as removing the current listener on the class when it's done. // // By passing in a pagefunction on the _Attach method, the class will reattach the // saved list to the calling pagefunction // // History: // 06/11/03: marka created // //--------------------------------------------------------------------------- using System; using System.Windows.Navigation; using System.Windows; using System.Diagnostics; using System.Collections; using System.Reflection; using System.IO; using System.Security.Permissions; using System.Security; namespace MS.Internal.AppModel { [Serializable] internal struct ReturnEventSaverInfo { internal ReturnEventSaverInfo(string delegateTypeName, string targetTypeName, string delegateMethodName, bool fSamePf) { _delegateTypeName = delegateTypeName; _targetTypeName = targetTypeName; _delegateMethodName = delegateMethodName; _delegateInSamePF = fSamePf; } internal String _delegateTypeName; internal String _targetTypeName; internal String _delegateMethodName; internal bool _delegateInSamePF; // Return Event handler comes from the same pagefunction, this is for non-generic workaround. } [Serializable] internal class ReturnEventSaver { internal ReturnEventSaver() { } ////// Critical - sets the critical _returnList. /// TreatAsSafe - _returnList is not exposed in any way. /// [SecurityCritical, SecurityTreatAsSafe] internal void _Detach(PageFunctionBase pf) { if (pf._Return != null && pf._Saver == null) { ReturnEventSaverInfo[] list = null; Delegate[] delegates = null; delegates = (pf._Return).GetInvocationList(); list = _returnList = new ReturnEventSaverInfo[delegates.Length]; for (int i = 0; i < delegates.Length; i++) { Delegate returnDelegate = delegates[i]; bool bSamePf = false; if (returnDelegate.Target == pf) { // This is the Event Handler implemented by the same PF, use for NonGeneric handling. bSamePf = true; } MethodInfo m = returnDelegate.Method; ReturnEventSaverInfo info = new ReturnEventSaverInfo( returnDelegate.GetType().AssemblyQualifiedName, returnDelegate.Target.GetType().AssemblyQualifiedName, m.Name, bSamePf); list[i] = info; } // // only save if there were delegates already attached. // note that there will be cases where the Saver has already been pre-populated from a Load // but no delegates have been created yet ( as the PF hasn`t called finish as yet) // // By only storing the saver once there are delegates - we avoid the problem of // wiping out any newly restored saver pf._Saver = this; } pf._DetachEvents(); } // // Attach the stored events to the supplied pagefunction. // // caller - the Calling Page's root element. We will reattach events *from* this page root element *to* the child // // child - the child PageFunction. Caller was originally attached to child, we're now reattaching *to* the child // ////// Critical - Asserts ReflectionPermission to be able re-create delegate to private method. /// TreatAsSafe - The delegate created is identical to the one that _Detach() received from /// the application and saved. This is ensured by matching the type of the original target /// object against the type of the new target. Thus we know that the application was able /// to create a delegate over the exact method, and even if that method had a LinkDemand, /// it was satisfied by the application. /// [SecurityCritical, SecurityTreatAsSafe] internal void _Attach(Object caller, PageFunctionBase child) { ReturnEventSaverInfo[] list = null; list = _returnList; if (list != null) { Debug.Assert(caller != null, "Caller should not be null"); for (int i = 0; i < list.Length; i++) { // // if (string.Compare(_returnList[i]._targetTypeName, caller.GetType().AssemblyQualifiedName, StringComparison.Ordinal) != 0) { throw new NotSupportedException(SR.Get(SRID.ReturnEventHandlerMustBeOnParentPage)); } Delegate d; try { new ReflectionPermission(ReflectionPermissionFlag.MemberAccess).Assert(); // BlessedAssert d = Delegate.CreateDelegate( Type.GetType(_returnList[i]._delegateTypeName), caller, _returnList[i]._delegateMethodName); } catch (Exception ex) { throw new NotSupportedException(SR.Get(SRID.ReturnEventHandlerMustBeOnParentPage), ex); } finally { ReflectionPermission.RevertAssert(); } child._AddEventHandler(d); } } } ////// Critical: contains metadata for delegates created under elevation. /// [SecurityCritical] private ReturnEventSaverInfo[] _returnList; // The list of delegates we want to persist and return later } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
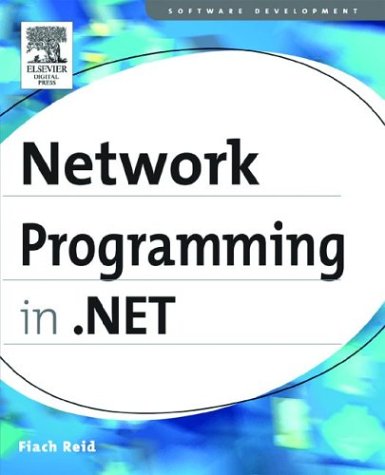
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NameSpaceExtractor.cs
- XmlReaderSettings.cs
- SecureStringHasher.cs
- TemplateBuilder.cs
- Matrix.cs
- MembershipSection.cs
- DataSourceControlBuilder.cs
- MetadataStore.cs
- Int64Storage.cs
- DynamicValueConverter.cs
- RelationshipDetailsRow.cs
- BypassElementCollection.cs
- FontCollection.cs
- XmlNodeReader.cs
- MenuItemAutomationPeer.cs
- indexingfiltermarshaler.cs
- X500Name.cs
- CmsInterop.cs
- FileIOPermission.cs
- Configuration.cs
- ToolboxDataAttribute.cs
- NameTable.cs
- TextEndOfParagraph.cs
- DrawingCollection.cs
- HttpCacheParams.cs
- VectorConverter.cs
- ColorTransform.cs
- BrowserTree.cs
- TagNameToTypeMapper.cs
- CookieParameter.cs
- ClrProviderManifest.cs
- ReadOnlyHierarchicalDataSourceView.cs
- RequestCachePolicy.cs
- XmlLinkedNode.cs
- XmlDomTextWriter.cs
- WindowsComboBox.cs
- WebPartHeaderCloseVerb.cs
- SqlDataSourceFilteringEventArgs.cs
- MessageEncoderFactory.cs
- PageSettings.cs
- DateTimeFormat.cs
- SmtpAuthenticationManager.cs
- DiagnosticsConfiguration.cs
- XmlParserContext.cs
- TreeNode.cs
- DependencyObjectProvider.cs
- DataPagerFieldItem.cs
- KeyGestureValueSerializer.cs
- ItemType.cs
- PerspectiveCamera.cs
- RubberbandSelector.cs
- VScrollBar.cs
- Preprocessor.cs
- ProtocolsConfiguration.cs
- CombinedGeometry.cs
- XmlWhitespace.cs
- DataObject.cs
- DataRelation.cs
- HijriCalendar.cs
- SqlEnums.cs
- BitmapFrame.cs
- Vector3DConverter.cs
- SelectionListComponentEditor.cs
- XmlSchemaFacet.cs
- DbException.cs
- CacheDependency.cs
- JsonEnumDataContract.cs
- EntitySetBase.cs
- JsonFormatReaderGenerator.cs
- TextBounds.cs
- UniqueSet.cs
- ToolStripPanelRenderEventArgs.cs
- MetabaseServerConfig.cs
- Descriptor.cs
- RadioButton.cs
- WithStatement.cs
- MultiDataTrigger.cs
- WebPartConnectionsCloseVerb.cs
- TemplateInstanceAttribute.cs
- DatatypeImplementation.cs
- SecurityToken.cs
- CharacterBuffer.cs
- CompatibleComparer.cs
- DbDataSourceEnumerator.cs
- BlurBitmapEffect.cs
- GuidelineSet.cs
- InvokeGenerator.cs
- CurrencyManager.cs
- GZipDecoder.cs
- UpdateCommand.cs
- PropertiesTab.cs
- DesignerView.xaml.cs
- TdsParser.cs
- IndexedString.cs
- TrackingMemoryStreamFactory.cs
- ICspAsymmetricAlgorithm.cs
- RadioButtonList.cs
- DynamicControlParameter.cs
- WindowsAuthenticationEventArgs.cs
- DoneReceivingAsyncResult.cs