Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Utils / TypeExtensions.cs / 1305376 / TypeExtensions.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Diagnostics; using System.Reflection; using System.Reflection.Emit; using System.Text; namespace System.Dynamic.Utils { // Extensions on System.Type and friends internal static class TypeExtensions { ////// Creates an open delegate for the given (dynamic)method. /// internal static Delegate CreateDelegate(this MethodInfo methodInfo, Type delegateType) { Debug.Assert(methodInfo != null && delegateType != null); var dm = methodInfo as DynamicMethod; if (dm != null) { return dm.CreateDelegate(delegateType); } else { return Delegate.CreateDelegate(delegateType, methodInfo); } } ////// Creates a closed delegate for the given (dynamic)method. /// internal static Delegate CreateDelegate(this MethodInfo methodInfo, Type delegateType, object target) { Debug.Assert(methodInfo != null && delegateType != null); var dm = methodInfo as DynamicMethod; if (dm != null) { return dm.CreateDelegate(delegateType, target); } else { return Delegate.CreateDelegate(delegateType, target, methodInfo); } } internal static Type GetReturnType(this MethodBase mi) { return (mi.IsConstructor) ? mi.DeclaringType : ((MethodInfo)mi).ReturnType; } private static readonly CacheDict_ParamInfoCache = new CacheDict (75); internal static ParameterInfo[] GetParametersCached(this MethodBase method) { ParameterInfo[] pis; lock (_ParamInfoCache) { if (!_ParamInfoCache.TryGetValue(method, out pis)) { pis = method.GetParameters(); Type t = method.DeclaringType; if (t != null && TypeUtils.CanCache(t)) { _ParamInfoCache[method] = pis; } } } return pis; } // Expression trees/compiler just use IsByRef, why do we need this? // (see LambdaCompiler.EmitArguments for usage in the compiler) internal static bool IsByRefParameter(this ParameterInfo pi) { // not using IsIn/IsOut properties as they are not available in Silverlight: if (pi.ParameterType.IsByRef) return true; return (pi.Attributes & (ParameterAttributes.Out)) == ParameterAttributes.Out; } // Returns the matching method if the parameter types are reference // assignable from the provided type arguments, otherwise null. internal static MethodInfo GetMethodValidated( this Type type, string name, BindingFlags bindingAttr, Binder binder, Type[] types, ParameterModifier[] modifiers) { var method = type.GetMethod(name, bindingAttr, binder, types, modifiers); return method.MatchesArgumentTypes(types) ? method : null; } /// /// Returns true if the method's parameter types are reference assignable from /// the argument types, otherwise false. /// /// An example that can make the method return false is that /// typeof(double).GetMethod("op_Equality", ..., new[] { typeof(double), typeof(int) }) /// returns a method with two double parameters, which doesn't match the provided /// argument types. /// ///private static bool MatchesArgumentTypes(this MethodInfo mi, Type[] argTypes) { if (mi == null || argTypes == null) { return false; } var ps = mi.GetParameters(); if (ps.Length != argTypes.Length) { return false; } for (int i = 0; i < ps.Length; i++) { if (!TypeUtils.AreReferenceAssignable(ps[i].ParameterType, argTypes[i])) { return false; } } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Diagnostics; using System.Reflection; using System.Reflection.Emit; using System.Text; namespace System.Dynamic.Utils { // Extensions on System.Type and friends internal static class TypeExtensions { /// /// Creates an open delegate for the given (dynamic)method. /// internal static Delegate CreateDelegate(this MethodInfo methodInfo, Type delegateType) { Debug.Assert(methodInfo != null && delegateType != null); var dm = methodInfo as DynamicMethod; if (dm != null) { return dm.CreateDelegate(delegateType); } else { return Delegate.CreateDelegate(delegateType, methodInfo); } } ////// Creates a closed delegate for the given (dynamic)method. /// internal static Delegate CreateDelegate(this MethodInfo methodInfo, Type delegateType, object target) { Debug.Assert(methodInfo != null && delegateType != null); var dm = methodInfo as DynamicMethod; if (dm != null) { return dm.CreateDelegate(delegateType, target); } else { return Delegate.CreateDelegate(delegateType, target, methodInfo); } } internal static Type GetReturnType(this MethodBase mi) { return (mi.IsConstructor) ? mi.DeclaringType : ((MethodInfo)mi).ReturnType; } private static readonly CacheDict_ParamInfoCache = new CacheDict (75); internal static ParameterInfo[] GetParametersCached(this MethodBase method) { ParameterInfo[] pis; lock (_ParamInfoCache) { if (!_ParamInfoCache.TryGetValue(method, out pis)) { pis = method.GetParameters(); Type t = method.DeclaringType; if (t != null && TypeUtils.CanCache(t)) { _ParamInfoCache[method] = pis; } } } return pis; } // Expression trees/compiler just use IsByRef, why do we need this? // (see LambdaCompiler.EmitArguments for usage in the compiler) internal static bool IsByRefParameter(this ParameterInfo pi) { // not using IsIn/IsOut properties as they are not available in Silverlight: if (pi.ParameterType.IsByRef) return true; return (pi.Attributes & (ParameterAttributes.Out)) == ParameterAttributes.Out; } // Returns the matching method if the parameter types are reference // assignable from the provided type arguments, otherwise null. internal static MethodInfo GetMethodValidated( this Type type, string name, BindingFlags bindingAttr, Binder binder, Type[] types, ParameterModifier[] modifiers) { var method = type.GetMethod(name, bindingAttr, binder, types, modifiers); return method.MatchesArgumentTypes(types) ? method : null; } /// /// Returns true if the method's parameter types are reference assignable from /// the argument types, otherwise false. /// /// An example that can make the method return false is that /// typeof(double).GetMethod("op_Equality", ..., new[] { typeof(double), typeof(int) }) /// returns a method with two double parameters, which doesn't match the provided /// argument types. /// ///private static bool MatchesArgumentTypes(this MethodInfo mi, Type[] argTypes) { if (mi == null || argTypes == null) { return false; } var ps = mi.GetParameters(); if (ps.Length != argTypes.Length) { return false; } for (int i = 0; i < ps.Length; i++) { if (!TypeUtils.AreReferenceAssignable(ps[i].ParameterType, argTypes[i])) { return false; } } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
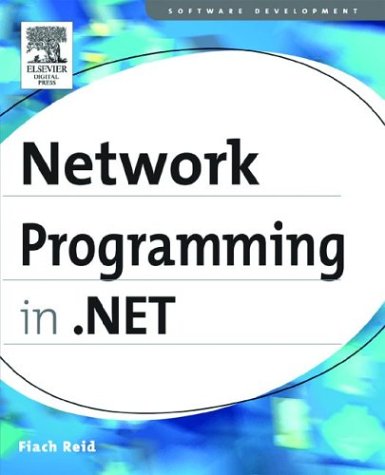
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XPathParser.cs
- TextPointer.cs
- QilStrConcat.cs
- SoapFault.cs
- Variant.cs
- WbmpConverter.cs
- IncrementalReadDecoders.cs
- ContractAdapter.cs
- ViewKeyConstraint.cs
- SizeAnimationUsingKeyFrames.cs
- Renderer.cs
- OracleCommandSet.cs
- TextOutput.cs
- TransformationRules.cs
- Assembly.cs
- RegexCompilationInfo.cs
- Configuration.cs
- PropertyBuilder.cs
- DecoderReplacementFallback.cs
- UxThemeWrapper.cs
- FileDialogCustomPlacesCollection.cs
- SQLConvert.cs
- HwndAppCommandInputProvider.cs
- DescriptionAttribute.cs
- FollowerQueueCreator.cs
- ValidationError.cs
- FormViewPagerRow.cs
- DataTemplate.cs
- ComponentGlyph.cs
- CodeNamespace.cs
- XmlSchemaInfo.cs
- ComponentDispatcher.cs
- HasCopySemanticsAttribute.cs
- Bezier.cs
- XmlTextReaderImplHelpers.cs
- GridViewSelectEventArgs.cs
- EdmProperty.cs
- Soap12ServerProtocol.cs
- UnsafeCollabNativeMethods.cs
- DataGridTextBox.cs
- ChtmlTextWriter.cs
- MasterPage.cs
- ETagAttribute.cs
- GcSettings.cs
- OverrideMode.cs
- BamlBinaryWriter.cs
- Dump.cs
- AttributeUsageAttribute.cs
- TreeViewAutomationPeer.cs
- WebPartZoneAutoFormat.cs
- PassportAuthenticationModule.cs
- Repeater.cs
- DelegatingTypeDescriptionProvider.cs
- CacheAxisQuery.cs
- ClientUrlResolverWrapper.cs
- BitmapFrame.cs
- HashRepartitionEnumerator.cs
- BlurEffect.cs
- SqlProcedureAttribute.cs
- DataGridCellEditEndingEventArgs.cs
- ToolboxItemFilterAttribute.cs
- XmlSchemaAny.cs
- ImpersonationContext.cs
- ResourcePool.cs
- WorkflowQueueInfo.cs
- ResXDataNode.cs
- ServiceModelPerformanceCounters.cs
- ButtonBase.cs
- DrawingServices.cs
- Message.cs
- RoleManagerEventArgs.cs
- SmiContext.cs
- documentsequencetextview.cs
- Screen.cs
- PassportAuthenticationEventArgs.cs
- ProcessModule.cs
- TreeIterators.cs
- RootContext.cs
- Repeater.cs
- PageContentAsyncResult.cs
- PingReply.cs
- DataServiceHostFactory.cs
- ZoomPercentageConverter.cs
- CellTreeNode.cs
- TrackBar.cs
- SiblingIterators.cs
- SvcMapFileLoader.cs
- SafeHandles.cs
- ObjectDataSourceStatusEventArgs.cs
- PassportIdentity.cs
- MultiBindingExpression.cs
- ExceptionRoutedEventArgs.cs
- FtpWebResponse.cs
- CodeTypeOfExpression.cs
- InstanceDescriptor.cs
- NotImplementedException.cs
- SecurityTokenAuthenticator.cs
- TypeExtensions.cs
- InfoCardService.cs
- SchemaMapping.cs