Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapFrame.cs / 1305600 / BitmapFrame.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation // // File: BitmapFrame.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using MS.Win32; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods = MS.Win32.PresentationCore.UnsafeNativeMethods; using System.Windows.Markup; using System.Net.Cache; namespace System.Windows.Media.Imaging { #region BitmapFrame ////// BitmapFrame abstract class /// public abstract class BitmapFrame : BitmapSource, IUriContext { #region Constructors ////// Constructor /// protected BitmapFrame() { } ////// Internal Constructor /// internal BitmapFrame(bool useVirtuals) : base(useVirtuals) { } ////// Create BitmapFrame from the uri or stream /// internal static BitmapFrame CreateFromUriOrStream( Uri baseUri, Uri uri, Stream stream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy uriCachePolicy ) { // Create a decoder and return the first frame if (uri != null) { Debug.Assert((stream == null), "Both stream and uri are non-null"); BitmapDecoder decoder = BitmapDecoder.CreateFromUriOrStream( baseUri, uri, null, createOptions, cacheOption, uriCachePolicy, true ); if (decoder.Frames.Count == 0) { throw new System.ArgumentException(SR.Get(SRID.Image_NoDecodeFrames), "uri"); } return decoder.Frames[0]; } else { Debug.Assert((stream != null), "Both stream and uri are null"); BitmapDecoder decoder = BitmapDecoder.Create( stream, createOptions, cacheOption ); if (decoder.Frames.Count == 0) { throw new System.ArgumentException(SR.Get(SRID.Image_NoDecodeFrames), "stream"); } return decoder.Frames[0]; } } ////// Create a BitmapFrame from a Uri using BitmapCreateOptions.None and /// BitmapCacheOption.Default /// /// Uri of the Bitmap public static BitmapFrame Create( Uri bitmapUri ) { return Create(bitmapUri, null); } ////// Create a BitmapFrame from a Uri using BitmapCreateOptions.None and /// BitmapCacheOption.Default /// /// Uri of the Bitmap /// Optional web request cache policy public static BitmapFrame Create( Uri bitmapUri, RequestCachePolicy uriCachePolicy ) { if (bitmapUri == null) { throw new ArgumentNullException("bitmapUri"); } return CreateFromUriOrStream( null, bitmapUri, null, BitmapCreateOptions.None, BitmapCacheOption.Default, uriCachePolicy ); } ////// Create a BitmapFrame from a Uri with the specified BitmapCreateOptions and /// BitmapCacheOption /// /// Uri of the Bitmap /// Creation options /// Caching option public static BitmapFrame Create( Uri bitmapUri, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption ) { return Create(bitmapUri, createOptions, cacheOption, null); } ////// Create a BitmapFrame from a Uri with the specified BitmapCreateOptions and /// BitmapCacheOption /// /// Uri of the Bitmap /// Creation options /// Caching option /// Optional web request cache policy public static BitmapFrame Create( Uri bitmapUri, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy uriCachePolicy ) { if (bitmapUri == null) { throw new ArgumentNullException("bitmapUri"); } return CreateFromUriOrStream( null, bitmapUri, null, createOptions, cacheOption, uriCachePolicy ); } ////// Create a BitmapFrame from a Stream using BitmapCreateOptions.None and /// BitmapCacheOption.Default /// /// Stream of the Bitmap public static BitmapFrame Create( Stream bitmapStream ) { if (bitmapStream == null) { throw new ArgumentNullException("bitmapStream"); } return CreateFromUriOrStream( null, null, bitmapStream, BitmapCreateOptions.None, BitmapCacheOption.Default, null ); } ////// Create a BitmapFrame from a Stream with the specified BitmapCreateOptions and /// BitmapCacheOption /// /// Stream of the Bitmap /// Creation options /// Caching option public static BitmapFrame Create( Stream bitmapStream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption ) { if (bitmapStream == null) { throw new ArgumentNullException("bitmapStream"); } return CreateFromUriOrStream( null, null, bitmapStream, createOptions, cacheOption, null ); } ////// Create a BitmapFrame from a BitmapSource /// /// Source input of the Bitmap public static BitmapFrame Create( BitmapSource source ) { if (source == null) { throw new ArgumentNullException("source"); } BitmapMetadata metadata = null; try { metadata = source.Metadata as BitmapMetadata; } catch (System.NotSupportedException) { //do not throw not support exception //just pass null } if (metadata != null) { metadata = metadata.Clone(); } return new BitmapFrameEncode(source, null, metadata, null); } ////// Create a BitmapFrame from a BitmapSource with the specified Thumbnail /// /// Source input of the Bitmap /// Thumbnail of the resulting Bitmap public static BitmapFrame Create( BitmapSource source, BitmapSource thumbnail ) { if (source == null) { throw new ArgumentNullException("source"); } BitmapMetadata metadata = null; try { metadata = source.Metadata as BitmapMetadata; } catch (System.NotSupportedException) { //do not throw not support exception //just pass null } if (metadata != null) { metadata = metadata.Clone(); } return BitmapFrame.Create(source, thumbnail, metadata, null); } ////// Create a BitmapFrame from a BitmapSource with the specified Thumbnail and metadata /// /// Source input of the Bitmap /// Thumbnail of the resulting Bitmap /// BitmapMetadata of the resulting Bitmap /// The ColorContexts for the resulting Bitmap public static BitmapFrame Create( BitmapSource source, BitmapSource thumbnail, BitmapMetadata metadata, ReadOnlyCollectioncolorContexts ) { if (source == null) { throw new ArgumentNullException("source"); } return new BitmapFrameEncode(source, thumbnail, metadata, colorContexts); } #endregion #region IUriContext /// /// Provides the base uri of the current context. /// public abstract Uri BaseUri { get; set; } #endregion #region Public Properties ////// Accesses the Thumbnail property for this BitmapFrame /// public abstract BitmapSource Thumbnail { get; } ////// Accesses the Decoder property for this BitmapFrame /// public abstract BitmapDecoder Decoder { get; } ////// Accesses the Decoder property for this BitmapFrame /// public abstract ReadOnlyCollectionColorContexts { get; } #endregion #region Public Properties /// /// Create an in-place bitmap metadata writer. /// public abstract InPlaceBitmapMetadataWriter CreateInPlaceBitmapMetadataWriter(); #endregion #region Internal Properties ////// Returns cached metadata and creates BitmapMetadata if it does not exist. /// This code will demand site of origin permissions. /// internal virtual BitmapMetadata InternalMetadata { get { return null; } set { throw new NotImplementedException(); } } #endregion #region Data Members /// Thumbnail internal BitmapSource _thumbnail = null; ////// Metadata /// ////// Critical - Access only granted if SiteOfOrigin demanded. /// [SecurityCritical] internal BitmapMetadata _metadata; /// ColorContexts collection internal ReadOnlyCollection_readOnlycolorContexts; #endregion } #endregion // BitmapFrame } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
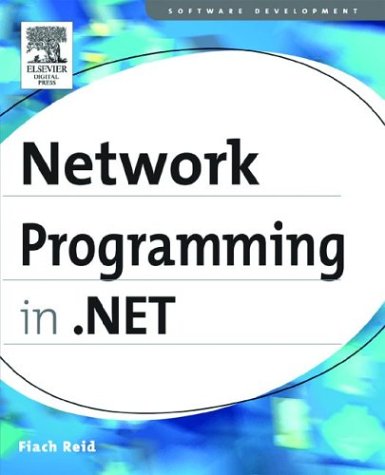
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLCharsStorage.cs
- UnsafeNativeMethods.cs
- TypeUnloadedException.cs
- TemplateEditingVerb.cs
- GlyphInfoList.cs
- SoapInteropTypes.cs
- TypeListConverter.cs
- PenContexts.cs
- AffineTransform3D.cs
- BufferedMessageData.cs
- AutomationPeer.cs
- FileSystemWatcher.cs
- FreezableOperations.cs
- ScriptControl.cs
- Setter.cs
- CredentialCache.cs
- DotExpr.cs
- TreeViewAutomationPeer.cs
- EntityConnectionStringBuilderItem.cs
- uribuilder.cs
- ServiceNotStartedException.cs
- UserControlParser.cs
- TCEAdapterGenerator.cs
- CaseExpr.cs
- PersistenceTypeAttribute.cs
- X509Utils.cs
- PersistenceProvider.cs
- EventProviderWriter.cs
- SettingsProperty.cs
- MouseActionConverter.cs
- ResourceExpressionBuilder.cs
- HostSecurityManager.cs
- DataGridColumnReorderingEventArgs.cs
- ImageClickEventArgs.cs
- ReaderWriterLockSlim.cs
- ProfilePropertySettingsCollection.cs
- Attachment.cs
- MsmqChannelListenerBase.cs
- ScopeCompiler.cs
- LookupNode.cs
- FilterEventArgs.cs
- ShaderEffect.cs
- PropertyTab.cs
- securitycriticaldataformultiplegetandset.cs
- DynamicExpression.cs
- PtsPage.cs
- StylusButtonEventArgs.cs
- MimeFormatter.cs
- ProbeMatchesMessage11.cs
- TrustManagerPromptUI.cs
- CodeVariableDeclarationStatement.cs
- FunctionMappingTranslator.cs
- ControlOperationBehavior.cs
- PerformanceCounter.cs
- ServiceDescriptionData.cs
- CodeAccessPermission.cs
- WebPartConnectionsCancelVerb.cs
- MetafileHeader.cs
- FtpCachePolicyElement.cs
- Compress.cs
- ItemsPresenter.cs
- SecurityCapabilities.cs
- IdnMapping.cs
- Operand.cs
- CodeMemberField.cs
- SQLInt32.cs
- ReturnEventArgs.cs
- CheckBoxPopupAdapter.cs
- ArrayWithOffset.cs
- FixedSOMTable.cs
- TextCompositionManager.cs
- ExeConfigurationFileMap.cs
- Schema.cs
- CategoryAttribute.cs
- TextEffect.cs
- MethodRental.cs
- KeyboardEventArgs.cs
- SafeNativeMethods.cs
- GenericAuthenticationEventArgs.cs
- XmlSchemaElement.cs
- UnsafeNativeMethodsTablet.cs
- TimelineClockCollection.cs
- Assembly.cs
- HtmlImage.cs
- CustomAssemblyResolver.cs
- RangeValidator.cs
- DtdParser.cs
- RealProxy.cs
- COM2IPerPropertyBrowsingHandler.cs
- ListViewItem.cs
- UxThemeWrapper.cs
- DataGridViewSelectedRowCollection.cs
- _HTTPDateParse.cs
- mda.cs
- MetadataArtifactLoaderCompositeResource.cs
- FormattedText.cs
- LayoutEvent.cs
- Win32KeyboardDevice.cs
- SqlBuilder.cs
- ResizeGrip.cs