Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Advanced / MetafileHeader.cs / 1305376 / MetafileHeader.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Configuration.Assemblies; using System.Diagnostics; using System; using System.Drawing; using System.Runtime.InteropServices; using System.Drawing.Internal; ////// /// Contains attributes of an /// associated [StructLayout(LayoutKind.Sequential)] public sealed class MetafileHeader { // determine which to use by nullity internal MetafileHeaderWmf wmf; internal MetafileHeaderEmf emf; internal MetafileHeader() { } ///. /// /// /// Gets the type of the associated public MetafileType Type { get { return IsWmf() ? wmf.type : emf.type; } } ///. /// /// /// public int MetafileSize { get { return IsWmf() ? wmf.size : emf.size; } } ////// Gets the size, in bytes, of the associated /// ///. /// /// /// Gets the version number of the associated /// public int Version { get { return IsWmf() ? wmf.version : emf.version; } } /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. private EmfPlusFlags EmfPlusFlags { get { return IsWmf() ? wmf.emfPlusFlags : emf.emfPlusFlags; } } */ ///. /// /// /// Gets the horizontal resolution, in /// dots-per-inch, of the associated public float DpiX { get { return IsWmf() ? wmf.dpiX : emf.dpiX; } } ///. /// /// /// Gets the vertical resolution, in /// dots-per-inch, of the associated public float DpiY { get { return IsWmf() ? wmf.dpiY : emf.dpiY; } } ///. /// /// /// Gets a public Rectangle Bounds { get { return IsWmf() ? new Rectangle(wmf.X, wmf.Y, wmf.Width, wmf.Height) : new Rectangle(emf.X, emf.Y, emf.Width, emf.Height); } } ///that bounds the associated /// . /// /// /// Returns a value indicating whether the /// associated public bool IsWmf() { if ((wmf == null) && (emf == null)) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); if ((wmf != null) && ((wmf.type == MetafileType.Wmf) || (wmf.type == MetafileType.WmfPlaceable))) return true; else return false; } ///is in the Windows metafile /// format. /// /// /// public bool IsWmfPlaceable() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((wmf != null) && (wmf.type == MetafileType.WmfPlaceable)); } ////// Returns a value indicating whether the /// associated ///is in the Windows Placeable metafile /// format. /// /// /// Returns a value indicating whether the /// associated public bool IsEmf() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type == MetafileType.Emf)); } ///is in the Windows enhanced metafile /// format. /// /// /// Returns a value indicating whether the /// associated public bool IsEmfOrEmfPlus() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type >= MetafileType.Emf)); } ///is in the Windows enhanced metafile /// format or the Windows enhanced metafile plus. /// /// /// Returns a value indicating whether the /// associated public bool IsEmfPlus() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type >= MetafileType.EmfPlusOnly)); } ///is in the Windows enhanced metafile /// plus format. /// /// /// Returns a value indicating whether the /// associated public bool IsEmfPlusDual() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type == MetafileType.EmfPlusDual)); } ///is in the Dual enhanced /// metafile format. This format supports both the enhanced and the enhanced /// plus format. /// /// /// public bool IsEmfPlusOnly() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type == MetafileType.EmfPlusOnly)); } ////// Returns a value indicating whether the associated ///supports only the Windows /// enhanced metafile plus format. /// /// /// public bool IsDisplay() { return IsEmfPlus() && ((((int)emf.emfPlusFlags) & ((int)EmfPlusFlags.Display)) != 0); } ////// Returns a value indicating whether the associated ///is device-dependent. /// /// /// Gets the WMF header file for the associated /// public MetaHeader WmfHeader { get { if (wmf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return wmf.WmfHeader; } } ///. /// /// /// /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. internal SafeNativeMethods.ENHMETAHEADER EmfHeader { get { if (emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return emf.EmfHeader; } } */ ////// Gets the WMF header file for the associated ///. /// /// /// Gets the size, in bytes, of the /// enhanced metafile plus header file. /// public int EmfPlusHeaderSize { get { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return IsWmf() ? wmf.EmfPlusHeaderSize : emf.EmfPlusHeaderSize; } } ////// /// public int LogicalDpiX { get { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return IsWmf() ? wmf.LogicalDpiX : emf.LogicalDpiX; } } ////// Gets the logical horizontal resolution, in /// dots-per-inch, of the associated ///. /// /// /// Gets the logical vertical resolution, in /// dots-per-inch, of the associated public int LogicalDpiY { get { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return IsWmf() ? wmf.LogicalDpiY : emf.LogicalDpiX; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Configuration.Assemblies; using System.Diagnostics; using System; using System.Drawing; using System.Runtime.InteropServices; using System.Drawing.Internal; ////// /// Contains attributes of an /// associated [StructLayout(LayoutKind.Sequential)] public sealed class MetafileHeader { // determine which to use by nullity internal MetafileHeaderWmf wmf; internal MetafileHeaderEmf emf; internal MetafileHeader() { } ///. /// /// /// Gets the type of the associated public MetafileType Type { get { return IsWmf() ? wmf.type : emf.type; } } ///. /// /// /// public int MetafileSize { get { return IsWmf() ? wmf.size : emf.size; } } ////// Gets the size, in bytes, of the associated /// ///. /// /// /// Gets the version number of the associated /// public int Version { get { return IsWmf() ? wmf.version : emf.version; } } /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. private EmfPlusFlags EmfPlusFlags { get { return IsWmf() ? wmf.emfPlusFlags : emf.emfPlusFlags; } } */ ///. /// /// /// Gets the horizontal resolution, in /// dots-per-inch, of the associated public float DpiX { get { return IsWmf() ? wmf.dpiX : emf.dpiX; } } ///. /// /// /// Gets the vertical resolution, in /// dots-per-inch, of the associated public float DpiY { get { return IsWmf() ? wmf.dpiY : emf.dpiY; } } ///. /// /// /// Gets a public Rectangle Bounds { get { return IsWmf() ? new Rectangle(wmf.X, wmf.Y, wmf.Width, wmf.Height) : new Rectangle(emf.X, emf.Y, emf.Width, emf.Height); } } ///that bounds the associated /// . /// /// /// Returns a value indicating whether the /// associated public bool IsWmf() { if ((wmf == null) && (emf == null)) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); if ((wmf != null) && ((wmf.type == MetafileType.Wmf) || (wmf.type == MetafileType.WmfPlaceable))) return true; else return false; } ///is in the Windows metafile /// format. /// /// /// public bool IsWmfPlaceable() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((wmf != null) && (wmf.type == MetafileType.WmfPlaceable)); } ////// Returns a value indicating whether the /// associated ///is in the Windows Placeable metafile /// format. /// /// /// Returns a value indicating whether the /// associated public bool IsEmf() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type == MetafileType.Emf)); } ///is in the Windows enhanced metafile /// format. /// /// /// Returns a value indicating whether the /// associated public bool IsEmfOrEmfPlus() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type >= MetafileType.Emf)); } ///is in the Windows enhanced metafile /// format or the Windows enhanced metafile plus. /// /// /// Returns a value indicating whether the /// associated public bool IsEmfPlus() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type >= MetafileType.EmfPlusOnly)); } ///is in the Windows enhanced metafile /// plus format. /// /// /// Returns a value indicating whether the /// associated public bool IsEmfPlusDual() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type == MetafileType.EmfPlusDual)); } ///is in the Dual enhanced /// metafile format. This format supports both the enhanced and the enhanced /// plus format. /// /// /// public bool IsEmfPlusOnly() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type == MetafileType.EmfPlusOnly)); } ////// Returns a value indicating whether the associated ///supports only the Windows /// enhanced metafile plus format. /// /// /// public bool IsDisplay() { return IsEmfPlus() && ((((int)emf.emfPlusFlags) & ((int)EmfPlusFlags.Display)) != 0); } ////// Returns a value indicating whether the associated ///is device-dependent. /// /// /// Gets the WMF header file for the associated /// public MetaHeader WmfHeader { get { if (wmf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return wmf.WmfHeader; } } ///. /// /// /// /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. internal SafeNativeMethods.ENHMETAHEADER EmfHeader { get { if (emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return emf.EmfHeader; } } */ ////// Gets the WMF header file for the associated ///. /// /// /// Gets the size, in bytes, of the /// enhanced metafile plus header file. /// public int EmfPlusHeaderSize { get { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return IsWmf() ? wmf.EmfPlusHeaderSize : emf.EmfPlusHeaderSize; } } ////// /// public int LogicalDpiX { get { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return IsWmf() ? wmf.LogicalDpiX : emf.LogicalDpiX; } } ////// Gets the logical horizontal resolution, in /// dots-per-inch, of the associated ///. /// /// /// Gets the logical vertical resolution, in /// dots-per-inch, of the associated public int LogicalDpiY { get { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return IsWmf() ? wmf.LogicalDpiY : emf.LogicalDpiX; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.. ///
Link Menu
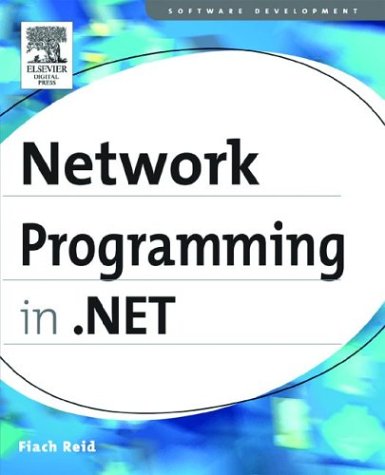
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ApplicationFileCodeDomTreeGenerator.cs
- XhtmlBasicObjectListAdapter.cs
- DiagnosticTrace.cs
- SourceSwitch.cs
- EncoderParameter.cs
- ItemCheckEvent.cs
- QilVisitor.cs
- AcceptorSessionSymmetricMessageSecurityProtocol.cs
- ColorConverter.cs
- KeyInfo.cs
- PropertyGridCommands.cs
- SQLRoleProvider.cs
- SelectedDatesCollection.cs
- RbTree.cs
- InputDevice.cs
- MailMessageEventArgs.cs
- _ConnectionGroup.cs
- MessageQueueCriteria.cs
- StorageModelBuildProvider.cs
- ClientRolePrincipal.cs
- StringAnimationBase.cs
- WmfPlaceableFileHeader.cs
- ContainerControl.cs
- ObjectStateManager.cs
- WsatConfiguration.cs
- TextComposition.cs
- SerializationException.cs
- SizeAnimationUsingKeyFrames.cs
- RequestCacheValidator.cs
- Config.cs
- SqlError.cs
- ToolStripItemCollection.cs
- MemoryResponseElement.cs
- SpinWait.cs
- safemediahandle.cs
- SafeProcessHandle.cs
- ConfigXmlSignificantWhitespace.cs
- EpmSyndicationContentDeSerializer.cs
- Win32.cs
- Inline.cs
- ExceptionUtility.cs
- PointAnimationUsingPath.cs
- DragDrop.cs
- CookielessData.cs
- AttributeParameterInfo.cs
- NCryptSafeHandles.cs
- TabItemAutomationPeer.cs
- XpsFont.cs
- TargetInvocationException.cs
- InvalidCommandTreeException.cs
- DesignerActionHeaderItem.cs
- Point3DCollectionValueSerializer.cs
- ToolStripDesignerUtils.cs
- ImageAnimator.cs
- BuildManager.cs
- DependencyPropertyKind.cs
- DocumentScope.cs
- DBPropSet.cs
- Parsers.cs
- TriggerBase.cs
- ConfigurationValidatorBase.cs
- DesignerVerbCollection.cs
- XPathBuilder.cs
- SourceItem.cs
- Timeline.cs
- MouseButtonEventArgs.cs
- DataGridCell.cs
- ReferenceSchema.cs
- LinkClickEvent.cs
- CompilationUnit.cs
- SchemaCollectionCompiler.cs
- DataConnectionHelper.cs
- BitStack.cs
- ListView.cs
- XPathAxisIterator.cs
- SimpleBitVector32.cs
- ByteAnimation.cs
- HttpClientCertificate.cs
- LinkArea.cs
- SqlDataSourceQueryEditorForm.cs
- AttachmentCollection.cs
- AnimationLayer.cs
- AffineTransform3D.cs
- MediaTimeline.cs
- PointAnimationUsingKeyFrames.cs
- ActivityPreviewDesigner.cs
- PnrpPeerResolver.cs
- FilterElement.cs
- RelationshipNavigation.cs
- SqlDataSourceSelectingEventArgs.cs
- WindowsTitleBar.cs
- WindowsListViewItem.cs
- ClientScriptManager.cs
- SafeSecurityHelper.cs
- ImageListUtils.cs
- OleDbRowUpdatedEvent.cs
- BaseDataList.cs
- MachineKeyConverter.cs
- StateRuntime.cs
- ColumnCollection.cs