Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Runtime / Serialization / Formatters / SoapFault.cs / 1 / SoapFault.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SoapFault ** **Author: Peter de Jong ([....]) ** ** Purpose: Specifies information for a Soap Fault ** ** Date: June 27, 2000 ** ===========================================================*/ namespace System.Runtime.Serialization.Formatters { using System; using System.Runtime.Serialization; using System.Runtime.Remoting; using System.Runtime.Remoting.Metadata; using System.Globalization; using System.Security.Permissions; //* Class holds soap fault information [Serializable, SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class SoapFault : ISerializable { String faultCode; String faultString; String faultActor; [SoapField(Embedded=true)] Object detail; public SoapFault() { } public SoapFault(String faultCode, String faultString, String faultActor, ServerFault serverFault) { this.faultCode = faultCode; this.faultString = faultString; this.faultActor = faultActor; this.detail = serverFault; } internal SoapFault(SerializationInfo info, StreamingContext context) { SerializationInfoEnumerator siEnum = info.GetEnumerator(); while(siEnum.MoveNext()) { String name = siEnum.Name; Object value = siEnum.Value; SerTrace.Log(this, "SetObjectData enum ",name," value ",value); if (String.Compare(name, "faultCode", true, CultureInfo.InvariantCulture) == 0) { int index = ((String)value).IndexOf(':'); if (index > -1) faultCode = ((String)value).Substring(++index); else faultCode = (String)value; } else if (String.Compare(name, "faultString", true, CultureInfo.InvariantCulture) == 0) faultString = (String)value; else if (String.Compare(name, "faultActor", true, CultureInfo.InvariantCulture) == 0) faultActor = (String)value; else if (String.Compare(name, "detail", true, CultureInfo.InvariantCulture) == 0) detail = value; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public void GetObjectData(SerializationInfo info, StreamingContext context) { info.AddValue("faultcode", "SOAP-ENV:"+faultCode); info.AddValue("faultstring", faultString); if (faultActor != null) info.AddValue("faultactor", faultActor); info.AddValue("detail", detail, typeof(Object)); } public String FaultCode { get {return faultCode;} set { faultCode = value;} } public String FaultString { get {return faultString;} set { faultString = value;} } public String FaultActor { get {return faultActor;} set { faultActor = value;} } public Object Detail { get {return detail;} set {detail = value;} } } [Serializable, SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class ServerFault { String exceptionType; String message; String stackTrace; Exception exception; internal ServerFault(Exception exception) { this.exception = exception; //this.exceptionType = exception.GetType().AssemblyQualifiedName; //this.message = exception.Message; } public ServerFault(String exceptionType, String message, String stackTrace) { this.exceptionType = exceptionType; this.message = message; this.stackTrace = stackTrace; } public String ExceptionType { get {return exceptionType;} set { exceptionType = value;} } public String ExceptionMessage { get {return message;} set { message = value;} } public String StackTrace { get {return stackTrace;} set {stackTrace = value;} } internal Exception Exception { get {return exception;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SoapFault ** **Author: Peter de Jong ([....]) ** ** Purpose: Specifies information for a Soap Fault ** ** Date: June 27, 2000 ** ===========================================================*/ namespace System.Runtime.Serialization.Formatters { using System; using System.Runtime.Serialization; using System.Runtime.Remoting; using System.Runtime.Remoting.Metadata; using System.Globalization; using System.Security.Permissions; //* Class holds soap fault information [Serializable, SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class SoapFault : ISerializable { String faultCode; String faultString; String faultActor; [SoapField(Embedded=true)] Object detail; public SoapFault() { } public SoapFault(String faultCode, String faultString, String faultActor, ServerFault serverFault) { this.faultCode = faultCode; this.faultString = faultString; this.faultActor = faultActor; this.detail = serverFault; } internal SoapFault(SerializationInfo info, StreamingContext context) { SerializationInfoEnumerator siEnum = info.GetEnumerator(); while(siEnum.MoveNext()) { String name = siEnum.Name; Object value = siEnum.Value; SerTrace.Log(this, "SetObjectData enum ",name," value ",value); if (String.Compare(name, "faultCode", true, CultureInfo.InvariantCulture) == 0) { int index = ((String)value).IndexOf(':'); if (index > -1) faultCode = ((String)value).Substring(++index); else faultCode = (String)value; } else if (String.Compare(name, "faultString", true, CultureInfo.InvariantCulture) == 0) faultString = (String)value; else if (String.Compare(name, "faultActor", true, CultureInfo.InvariantCulture) == 0) faultActor = (String)value; else if (String.Compare(name, "detail", true, CultureInfo.InvariantCulture) == 0) detail = value; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public void GetObjectData(SerializationInfo info, StreamingContext context) { info.AddValue("faultcode", "SOAP-ENV:"+faultCode); info.AddValue("faultstring", faultString); if (faultActor != null) info.AddValue("faultactor", faultActor); info.AddValue("detail", detail, typeof(Object)); } public String FaultCode { get {return faultCode;} set { faultCode = value;} } public String FaultString { get {return faultString;} set { faultString = value;} } public String FaultActor { get {return faultActor;} set { faultActor = value;} } public Object Detail { get {return detail;} set {detail = value;} } } [Serializable, SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class ServerFault { String exceptionType; String message; String stackTrace; Exception exception; internal ServerFault(Exception exception) { this.exception = exception; //this.exceptionType = exception.GetType().AssemblyQualifiedName; //this.message = exception.Message; } public ServerFault(String exceptionType, String message, String stackTrace) { this.exceptionType = exceptionType; this.message = message; this.stackTrace = stackTrace; } public String ExceptionType { get {return exceptionType;} set { exceptionType = value;} } public String ExceptionMessage { get {return message;} set { message = value;} } public String StackTrace { get {return stackTrace;} set {stackTrace = value;} } internal Exception Exception { get {return exception;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
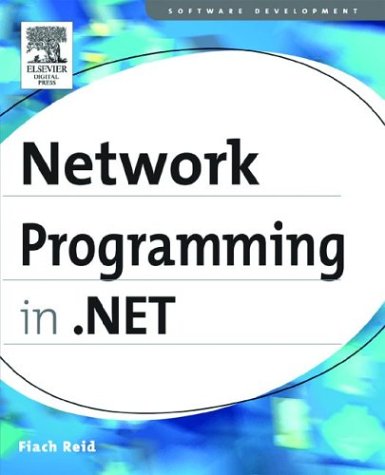
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Form.cs
- EnumValidator.cs
- IUnknownConstantAttribute.cs
- NullableDoubleMinMaxAggregationOperator.cs
- ErrorHandlingReceiver.cs
- QilBinary.cs
- BitmapEffect.cs
- PaperSource.cs
- PortCache.cs
- InputDevice.cs
- DataGridViewControlCollection.cs
- BackgroundFormatInfo.cs
- TextRangeEditTables.cs
- XmlSchemaChoice.cs
- DescendantBaseQuery.cs
- PathSegmentCollection.cs
- WorkflowDispatchContext.cs
- SchemaImporterExtension.cs
- DataGridViewRowsRemovedEventArgs.cs
- IntegerValidatorAttribute.cs
- XmlReaderSettings.cs
- ToolboxItemLoader.cs
- RequiredFieldValidator.cs
- EventMappingSettingsCollection.cs
- FlowLayoutPanel.cs
- StreamHelper.cs
- _NetworkingPerfCounters.cs
- TextMetrics.cs
- CompilerScope.cs
- CqlLexer.cs
- ConditionalAttribute.cs
- DnsEndpointIdentity.cs
- UnsettableComboBox.cs
- HtmlGenericControl.cs
- MaterialGroup.cs
- DrawingAttributes.cs
- MaskInputRejectedEventArgs.cs
- SQLMoneyStorage.cs
- InternalRelationshipCollection.cs
- UInt32.cs
- Encoder.cs
- GCHandleCookieTable.cs
- ActivityExecutorDelegateInfo.cs
- PeerResolverSettings.cs
- LineInfo.cs
- Utils.cs
- TreeView.cs
- HelloOperationCD1AsyncResult.cs
- Renderer.cs
- ListControl.cs
- PointAnimationUsingKeyFrames.cs
- NameSpaceExtractor.cs
- Metafile.cs
- SizeLimitedCache.cs
- DeploymentSection.cs
- DataPagerFieldCommandEventArgs.cs
- SignalGate.cs
- ExtenderProviderService.cs
- unsafeIndexingFilterStream.cs
- ColumnBinding.cs
- XsdValidatingReader.cs
- StreamInfo.cs
- StorageTypeMapping.cs
- PriorityBinding.cs
- Emitter.cs
- DataRowExtensions.cs
- TextTreeExtractElementUndoUnit.cs
- StringWriter.cs
- CallSiteOps.cs
- CustomError.cs
- XamlInterfaces.cs
- DeflateStream.cs
- MLangCodePageEncoding.cs
- TextElement.cs
- WindowsListViewSubItem.cs
- ThicknessKeyFrameCollection.cs
- InputProcessorProfilesLoader.cs
- DataGridViewRowStateChangedEventArgs.cs
- PropertyGridView.cs
- StylusPlugInCollection.cs
- FrameworkElementFactory.cs
- IntAverageAggregationOperator.cs
- CollectionBuilder.cs
- IgnoreFileBuildProvider.cs
- TypePropertyEditor.cs
- EnvelopedPkcs7.cs
- InvalidPropValue.cs
- WebBrowserNavigatedEventHandler.cs
- ToolStripDesigner.cs
- RawStylusInputReport.cs
- CompilerError.cs
- FtpCachePolicyElement.cs
- ToolStripContentPanel.cs
- ContractType.cs
- DateTimeFormatInfoScanner.cs
- RuleSet.cs
- ValidationHelper.cs
- XmlSerializerAssemblyAttribute.cs
- XmlUtil.cs
- CannotUnloadAppDomainException.cs