Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / AddIn / AddIn / System / Addin / Pipeline / ContractAdapter.cs / 1305376 / ContractAdapter.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ContractAdapter ** ===========================================================*/ using System; using System.Collections.Generic; using System.Globalization; using System.AddIn.Contract; using System.AddIn; using System.Security; using System.Security.Permissions; using System.AddIn.Hosting; using System.Reflection; using System.AddIn.MiniReflection; using System.Diagnostics.Contracts; namespace System.AddIn.Pipeline { public static class ContractAdapter { public static ContractHandle ViewToContractAdapter(Object view) { if (view == null) throw new ArgumentNullException("view"); System.Diagnostics.Contracts.Contract.EndContractBlock(); AddInController controller = AddInController.GetAddInController(view); if (controller != null) { return new ContractHandle(controller.AddInControllerImpl.GetContract()); } return null; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1004:GenericMethodsShouldProvideTypeParameter", Justification = "Factory Method")] public static TView ContractToViewAdapter(ContractHandle contract, PipelineStoreLocation location) { if (location != PipelineStoreLocation.ApplicationBase) throw new ArgumentException(Res.InvalidPipelineStoreLocation, "location"); System.Diagnostics.Contracts.Contract.EndContractBlock(); String appBase = AddInStore.GetAppBase(); return ContractToViewAdapterImpl (contract, appBase, false); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1004:GenericMethodsShouldProvideTypeParameter", Justification = "Factory Method")] public static TView ContractToViewAdapter (ContractHandle contract, string pipelineRoot) { return ContractToViewAdapterImpl (contract, pipelineRoot, true); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability","CA2004:RemoveCallsToGCKeepAlive", Justification="The message is about SafeHandles")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1004:GenericMethodsShouldProvideTypeParameter", Justification="Factory Method")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security","CA2103:ReviewImperativeSecurity")] private static TView ContractToViewAdapterImpl (ContractHandle contract, String pipelineRoot, bool demand) { if (contract == null) throw new ArgumentNullException("contract"); if (pipelineRoot == null) throw new ArgumentNullException("pipelineRoot"); if (String.IsNullOrEmpty(pipelineRoot)) throw new ArgumentException(Res.PathCantBeEmpty); System.Diagnostics.Contracts.Contract.EndContractBlock(); if (demand) new FileIOPermission(FileIOPermissionAccess.Read, pipelineRoot).Demand(); Type havType = typeof(TView); TypeInfo havTypeInfo = new TypeInfo(havType); List partialTokens = AddInStore.GetPartialTokens(pipelineRoot); foreach (PartialToken partialToken in partialTokens) { if (AddInStore.Contains(partialToken.HostAdapter.HostAddinViews, havTypeInfo)) { partialToken.PipelineRootDirectory = pipelineRoot; //Ask for something that can implement the contract in this partial token. The result will //either be null, the addin adapter itself, or another addin adapter IContract subcontract = contract.Contract.QueryContract(partialToken._contract.TypeInfo.AssemblyQualifiedName); if (subcontract != null) { //Instantiate the adapter and pass in the addin to its constructor TView hav = AddInActivator.ActivateHostAdapter (partialToken, subcontract); return hav; } } } // Don't let the ref count go to zero too soon, before we increment it in ActivateHostAdapter // This is important when QueryContract returns the addIn adapter itself. A GC at that point // may collect the ContractHandle and decrement the ref count to zero before we have a chance to increment it System.GC.KeepAlive(contract); // return null. Compiler makes us return default(TView), which will be null return default(TView); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
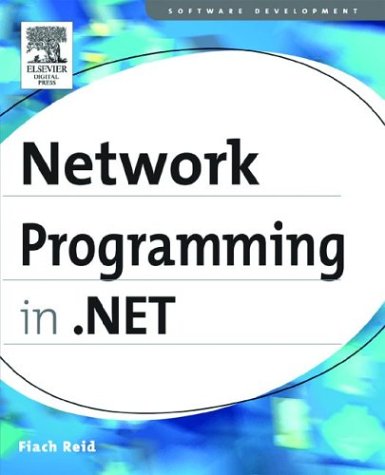
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SelectedDatesCollection.cs
- StreamProxy.cs
- TakeQueryOptionExpression.cs
- XmlProcessingInstruction.cs
- ElementUtil.cs
- SelectionPattern.cs
- BindingList.cs
- DecimalAnimationUsingKeyFrames.cs
- GeometryGroup.cs
- TimersDescriptionAttribute.cs
- PenContexts.cs
- ByteStack.cs
- SignedXmlDebugLog.cs
- WebPartConnection.cs
- TranslateTransform3D.cs
- CompareValidator.cs
- TextTreeObjectNode.cs
- NextPreviousPagerField.cs
- TransformedBitmap.cs
- DocumentPageTextView.cs
- TrackingProvider.cs
- AmbientProperties.cs
- ClientTarget.cs
- ListChangedEventArgs.cs
- ProgressBarBrushConverter.cs
- BitmapSourceSafeMILHandle.cs
- CqlGenerator.cs
- ADConnectionHelper.cs
- SafeReversePInvokeHandle.cs
- DbSourceParameterCollection.cs
- FigureParaClient.cs
- DurableServiceAttribute.cs
- SwitchLevelAttribute.cs
- RenderingEventArgs.cs
- ContentOperations.cs
- OutputChannelBinder.cs
- hresults.cs
- RowCache.cs
- HMACRIPEMD160.cs
- WindowPatternIdentifiers.cs
- SQLStringStorage.cs
- COMException.cs
- ProxyWebPartManager.cs
- HttpValueCollection.cs
- ImageDrawing.cs
- LayoutManager.cs
- SmtpReplyReaderFactory.cs
- CompensationHandlingFilter.cs
- BufferedGraphicsManager.cs
- ASCIIEncoding.cs
- ResourcePart.cs
- TextEditorCharacters.cs
- XmlEnumAttribute.cs
- SharedUtils.cs
- BreadCrumbTextConverter.cs
- DataGridViewCheckBoxCell.cs
- PathFigure.cs
- SeverityFilter.cs
- TextWriter.cs
- Color.cs
- PageFunction.cs
- Metafile.cs
- ArcSegment.cs
- EnvironmentPermission.cs
- DataRecordInfo.cs
- DataViewManager.cs
- IntersectQueryOperator.cs
- TdsParserSessionPool.cs
- DoubleKeyFrameCollection.cs
- SqlOuterApplyReducer.cs
- UseAttributeSetsAction.cs
- UrlMappingCollection.cs
- ClassDataContract.cs
- XmlSchemaAttributeGroupRef.cs
- RegionIterator.cs
- HtmlControl.cs
- ProcessingInstructionAction.cs
- TdsRecordBufferSetter.cs
- InboundActivityHelper.cs
- SimpleLine.cs
- AttributeEmitter.cs
- SimpleFileLog.cs
- XmlNamedNodeMap.cs
- XmlRawWriterWrapper.cs
- Input.cs
- StateItem.cs
- Rectangle.cs
- XmlName.cs
- ObjectTokenCategory.cs
- mda.cs
- Header.cs
- Pair.cs
- NoneExcludedImageIndexConverter.cs
- localization.cs
- IdentityValidationException.cs
- WindowsTooltip.cs
- SqlDataReader.cs
- EventWaitHandleSecurity.cs
- EventMappingSettings.cs
- ForEach.cs