Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / IdleTimeoutMonitor.cs / 1 / IdleTimeoutMonitor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // if (HostingEnvironment.BusyCount != 0) return; // if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // if (HostingEnvironment.BusyCount != 0) return; // if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
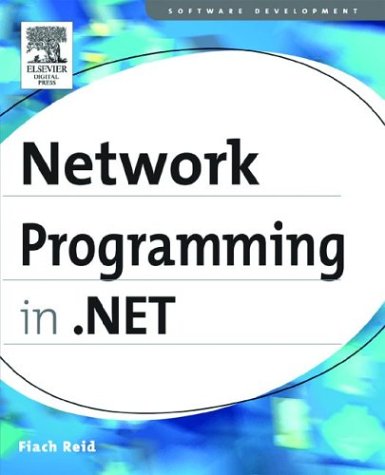
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataTableNewRowEvent.cs
- TreeNodeStyle.cs
- PathGradientBrush.cs
- FormViewPagerRow.cs
- Latin1Encoding.cs
- TokenFactoryFactory.cs
- altserialization.cs
- EntityDataSourceState.cs
- CacheDependency.cs
- BaseDataBoundControl.cs
- DayRenderEvent.cs
- FieldInfo.cs
- SizeF.cs
- ThemeDictionaryExtension.cs
- PkcsUtils.cs
- IODescriptionAttribute.cs
- SeparatorAutomationPeer.cs
- AppDomainUnloadedException.cs
- TypographyProperties.cs
- ListMarkerLine.cs
- BasicExpressionVisitor.cs
- ConnectionPoint.cs
- CodeComment.cs
- DependencyObjectValidator.cs
- categoryentry.cs
- ConfigurationValidatorBase.cs
- FieldDescriptor.cs
- CollectionViewSource.cs
- OperatingSystemVersionCheck.cs
- TypeConverterHelper.cs
- PropertyDescriptorCollection.cs
- RunInstallerAttribute.cs
- Rights.cs
- CannotUnloadAppDomainException.cs
- InfiniteIntConverter.cs
- AppDomainProtocolHandler.cs
- RequestQueue.cs
- BufferedOutputStream.cs
- Itemizer.cs
- Rectangle.cs
- XPathExpr.cs
- TextDecorationUnitValidation.cs
- Translator.cs
- InputMethodStateChangeEventArgs.cs
- DataGridTable.cs
- OleDbFactory.cs
- TagMapCollection.cs
- ConnectionStringsExpressionBuilder.cs
- MultiPropertyDescriptorGridEntry.cs
- PasswordRecovery.cs
- Int64.cs
- EncodingInfo.cs
- SiteMapPathDesigner.cs
- WebPartUserCapability.cs
- DesignerPerfEventProvider.cs
- HtmlMeta.cs
- EventLogPermission.cs
- XmlSiteMapProvider.cs
- DocumentPage.cs
- Control.cs
- ListView.cs
- EmbeddedMailObject.cs
- DataSpaceManager.cs
- WorkflowApplicationTerminatedException.cs
- CatalogPartCollection.cs
- RepeaterItemEventArgs.cs
- SurrogateChar.cs
- XamlTemplateSerializer.cs
- CheckBoxBaseAdapter.cs
- Constraint.cs
- XmlUnspecifiedAttribute.cs
- DrawingContextWalker.cs
- DataColumn.cs
- GeneralTransformGroup.cs
- StateRuntime.cs
- CodeMemberField.cs
- QilBinary.cs
- CustomPopupPlacement.cs
- PipeSecurity.cs
- SchemaImporterExtensionsSection.cs
- WsdlBuildProvider.cs
- CodeDelegateCreateExpression.cs
- RouteItem.cs
- HandlerBase.cs
- WrappedIUnknown.cs
- PersonalizationDictionary.cs
- WindowsGraphicsCacheManager.cs
- SamlAssertionKeyIdentifierClause.cs
- HyperLinkStyle.cs
- TextTreeTextElementNode.cs
- TCEAdapterGenerator.cs
- DataSourceProvider.cs
- MediaTimeline.cs
- TextPatternIdentifiers.cs
- DataGridBeginningEditEventArgs.cs
- HtmlFormAdapter.cs
- AnnotationElement.cs
- FeatureSupport.cs
- ErrorFormatterPage.cs
- CryptoHelper.cs