Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / SqlClient / SqlGen / SqlSelectClauseBuilder.cs / 1305376 / SqlSelectClauseBuilder.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; namespace System.Data.SqlClient.SqlGen { ////// This class is used for building the SELECT clause of a Sql Statement /// It is used to gather information about required and optional columns /// and whether TOP and DISTINCT should be specified. /// /// The underlying SqlBuilder is used for gathering the required columns. /// /// The list of OptionalColumns is used for gathering the optional columns. /// Whether a given OptionalColumn should be written is known only after the entire /// command tree has been processed. /// /// The IsDistinct property indicates that we want distinct columns. /// This is given out of band, since the input expression to the select clause /// may already have some columns projected out, and we use append-only SqlBuilders. /// The DISTINCT is inserted when we finally write the object into a string. /// /// Also, we have a Top property, which is non-null if the number of results should /// be limited to certain number. It is given out of band for the same reasons as DISTINCT. /// /// internal class SqlSelectClauseBuilder : SqlBuilder { #region Fields and Properties private Listm_optionalColumns; internal void AddOptionalColumn(OptionalColumn column) { if (m_optionalColumns == null) { m_optionalColumns = new List (); } m_optionalColumns.Add(column); } private TopClause m_top; internal TopClause Top { get { return m_top; } set { Debug.Assert(m_top == null, "SqlSelectStatement.Top has already been set"); m_top = value; } } /// /// Do we need to add a DISTINCT at the beginning of the SELECT /// internal bool IsDistinct { get; set; } ////// Whether any columns have been specified. /// public override bool IsEmpty { get { return (base.IsEmpty) && (this.m_optionalColumns == null || this.m_optionalColumns.Count == 0); } } private readonly Funcm_isPartOfTopMostStatement; #endregion #region Constructor internal SqlSelectClauseBuilder(Func isPartOfTopMostStatement) { this.m_isPartOfTopMostStatement = isPartOfTopMostStatement; } #endregion #region ISqlFragment Members /// /// Writes the string representing the Select statement: /// /// SELECT (DISTINCT) (TOP topClause) (optionalColumns) (requiredColumns) /// /// If Distinct is specified or this is part of a top most statement /// all optional columns are marked as used. /// /// Optional columns are only written if marked as used. /// In addition, if no required columns are specified and no optional columns are /// marked as used, the first optional column is written. /// /// /// /// public override void WriteSql(SqlWriter writer, SqlGenerator sqlGenerator) { writer.Write("SELECT "); if (IsDistinct) { writer.Write("DISTINCT "); } if (this.Top != null) { this.Top.WriteSql(writer, sqlGenerator); } if (this.IsEmpty) { Debug.Assert(false); // we have removed all possibilities of SELECT *. writer.Write("*"); } else { //Print the optional columns if any bool printedAny = WriteOptionalColumns(writer, sqlGenerator); if (!base.IsEmpty) { if (printedAny) { writer.Write(", "); } base.WriteSql(writer, sqlGenerator); } //If no optional columns were printed and there were no other columns, // print at least the first optional column else if (!printedAny) { this.m_optionalColumns[0].MarkAsUsed(); m_optionalColumns[0].WriteSqlIfUsed(writer, sqlGenerator, ""); } } } #endregion #region Private Helper Methods ////// Writes the optional columns that are used. /// If this is the topmost statement or distict is specifed as part of the same statement /// all optoinal columns are written. /// /// /// ///Whether at least one column got written private bool WriteOptionalColumns(SqlWriter writer, SqlGenerator sqlGenerator) { if (this.m_optionalColumns == null) { return false; } if (m_isPartOfTopMostStatement() || IsDistinct) { foreach (OptionalColumn column in this.m_optionalColumns) { column.MarkAsUsed(); } } string separator = ""; bool printedAny = false; foreach (OptionalColumn column in this.m_optionalColumns) { if (column.WriteSqlIfUsed(writer, sqlGenerator, separator)) { printedAny = true; separator = ", "; } } return printedAny; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
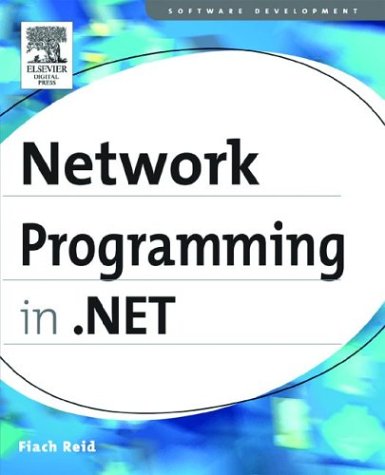
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigsHelper.cs
- FormViewRow.cs
- ActiveDocumentEvent.cs
- SimpleHandlerBuildProvider.cs
- XmlEnumAttribute.cs
- Visual3DCollection.cs
- XmlAttributes.cs
- TypeConverter.cs
- EventArgs.cs
- FixUp.cs
- WorkflowMarkupSerializationProvider.cs
- EntityDataSourceChangedEventArgs.cs
- SubqueryRules.cs
- NamedPipeTransportElement.cs
- Button.cs
- WebControlAdapter.cs
- MemoryPressure.cs
- IntSecurity.cs
- ConstraintManager.cs
- HatchBrush.cs
- GridViewPageEventArgs.cs
- PropertyGridEditorPart.cs
- BitmapEffectvisualstate.cs
- SemanticKeyElement.cs
- ProtocolElementCollection.cs
- GridViewUpdateEventArgs.cs
- ResolveMatchesMessageCD1.cs
- Annotation.cs
- DynamicContractTypeBuilder.cs
- FixedPageStructure.cs
- XmlSchemaExporter.cs
- HwndSource.cs
- XmlUtil.cs
- VolatileResourceManager.cs
- XmlComment.cs
- UInt64.cs
- GridViewColumn.cs
- SolidColorBrush.cs
- ObjectDataSourceEventArgs.cs
- ToolboxDataAttribute.cs
- ComplexTypeEmitter.cs
- BrowserDefinition.cs
- BitmapImage.cs
- LambdaCompiler.Logical.cs
- TrustLevelCollection.cs
- PartitionResolver.cs
- Util.cs
- WindowsTitleBar.cs
- DoubleCollectionConverter.cs
- Gdiplus.cs
- MembershipPasswordException.cs
- CollectionBuilder.cs
- TaiwanLunisolarCalendar.cs
- GroupItem.cs
- DbDataReader.cs
- VectorAnimationBase.cs
- PopupControlService.cs
- HttpCookie.cs
- SQLSingle.cs
- InputScope.cs
- UrlMappingsSection.cs
- CryptoKeySecurity.cs
- ChildDocumentBlock.cs
- StrokeNodeOperations.cs
- Int32Animation.cs
- CSharpCodeProvider.cs
- Model3DGroup.cs
- TimerEventSubscription.cs
- LogAppendAsyncResult.cs
- BigIntegerStorage.cs
- TimerElapsedEvenArgs.cs
- SessionStateUtil.cs
- OutputCacheProfile.cs
- ECDiffieHellmanPublicKey.cs
- CounterCreationData.cs
- CustomPopupPlacement.cs
- StaticDataManager.cs
- NonBatchDirectoryCompiler.cs
- JsonSerializer.cs
- ModelPropertyCollectionImpl.cs
- ThemeConfigurationDialog.cs
- StylusSystemGestureEventArgs.cs
- ConditionalAttribute.cs
- ObjectMemberMapping.cs
- SRGSCompiler.cs
- NonVisualControlAttribute.cs
- BigInt.cs
- Setter.cs
- Point.cs
- IdentifierService.cs
- QueryIntervalOp.cs
- EncodingFallbackAwareXmlTextWriter.cs
- messageonlyhwndwrapper.cs
- TreeNode.cs
- AnimationTimeline.cs
- TrackingMemoryStreamFactory.cs
- Table.cs
- WindowsScrollBarBits.cs
- XPathExpr.cs
- X509SubjectKeyIdentifierClause.cs