Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Bridge / Configuration / ProtocolElementCollection.cs / 1 / ProtocolElementCollection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Bridge.Configuration { using System; using System.Configuration; using System.Xml; [ConfigurationCollection(typeof(ProtocolElement))] internal sealed partial class ProtocolElementCollection : ConfigurationElementCollection { const string wstxProtocolType10 = ("Microsoft.Transactions.Wsat.Protocol.PluggableProtocol10, "+ "Microsoft.Transactions.Bridge, "+ "Version=" +ThisAssembly.Version + ", " + "Culture=neutral, "+ "PublicKeyToken="+AssemblyRef.MicrosoftPublicKey); const string wstxProtocolType11 = ("Microsoft.Transactions.Wsat.Protocol.PluggableProtocol11, "+ "Microsoft.Transactions.Bridge, "+ "Version=" +ThisAssembly.Version + ", " + "Culture=neutral, "+ "PublicKeyToken="+AssemblyRef.MicrosoftPublicKey); public ProtocolElementCollection() { } internal void SetDefaults() { ProtocolElement element1 = new ProtocolElement(); element1.Type = wstxProtocolType10; ProtocolElement element2 = new ProtocolElement(); element2.Type = wstxProtocolType11; this.Add(element1); this.Add(element2); this.ResetModified(); } public ProtocolElement this[int index] { get { return (ProtocolElement)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index,value); } } public void AssertBothWsatProtocolVersions() { bool found10 = false; bool found11 = false; foreach (ProtocolElement protocol in this) { if(protocol.Type == wstxProtocolType10) found10 = true; else if(protocol.Type == wstxProtocolType11) found11 =true; } if((!found10 & found11) || (found10 & !found11)) { string protocolStarted; if(found10) { protocolStarted = " Wsat 1.0 "; } else { protocolStarted = " Wsat 1.1 "; } DiagnosticUtility.FailFast("Both Wsat protocol versions should be configured to start up. Only" + protocolStarted + "is started."); } } public void Add(ProtocolElement element) { BaseAdd(element); } public void Clear() { BaseClear(); } protected override ConfigurationElement CreateNewElement() { return new ProtocolElement(); } protected override Object GetElementKey(ConfigurationElement element) { return ((ProtocolElement)element).Type; } public int IndexOf(ProtocolElement element) { return BaseIndexOf(element); } public void Remove(ProtocolElement element) { BaseRemove(element.Type); } public void Remove(string name) { BaseRemove(name); } public void RemoveAt(int index) { BaseRemoveAt(index); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
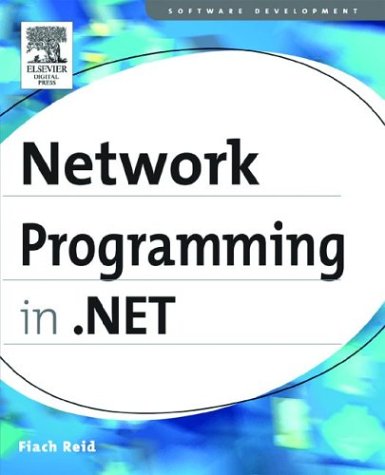
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QueryPageSettingsEventArgs.cs
- InputScopeManager.cs
- TimeSpanValidator.cs
- arc.cs
- MemberRelationshipService.cs
- querybuilder.cs
- DataGridViewColumnCollection.cs
- ColumnTypeConverter.cs
- CodeObjectCreateExpression.cs
- Dictionary.cs
- PaintEvent.cs
- OleDbSchemaGuid.cs
- SEHException.cs
- TableProvider.cs
- MouseGesture.cs
- AssemblyBuilderData.cs
- DesignerAdapterUtil.cs
- IntPtr.cs
- DependencyObjectValidator.cs
- SafeTimerHandle.cs
- GridViewDeleteEventArgs.cs
- SelectionBorderGlyph.cs
- UnSafeCharBuffer.cs
- TraceSection.cs
- ServiceCredentials.cs
- Native.cs
- ProtocolInformationReader.cs
- FileDetails.cs
- SvcMapFileLoader.cs
- ConfigXmlElement.cs
- MobileDeviceCapabilitiesSectionHandler.cs
- MemberPath.cs
- XmlIlTypeHelper.cs
- HttpListenerElement.cs
- StickyNoteHelper.cs
- XmlCountingReader.cs
- XmlAnyElementAttributes.cs
- updatecommandorderer.cs
- Accessors.cs
- DataServiceStreamProviderWrapper.cs
- WbemProvider.cs
- Stroke2.cs
- Help.cs
- ScrollPattern.cs
- WindowsRichEditRange.cs
- sitestring.cs
- EditorZone.cs
- GiveFeedbackEventArgs.cs
- Freezable.cs
- Stopwatch.cs
- CodeAttributeDeclarationCollection.cs
- TrustSection.cs
- SortedSetDebugView.cs
- EntityDataSourceQueryBuilder.cs
- HuffmanTree.cs
- WebPartVerbCollection.cs
- UnconditionalPolicy.cs
- ColorMatrix.cs
- PrimitiveXmlSerializers.cs
- DynamicValidatorEventArgs.cs
- OdbcStatementHandle.cs
- PartialCachingControl.cs
- SqlFlattener.cs
- PropertyGrid.cs
- DataServiceQueryException.cs
- ListItemConverter.cs
- XmlWellformedWriter.cs
- IncrementalReadDecoders.cs
- StateMachine.cs
- SqlException.cs
- ColorAnimationBase.cs
- RectAnimation.cs
- FontStyle.cs
- ThreadInterruptedException.cs
- jithelpers.cs
- IndicCharClassifier.cs
- HandlerFactoryCache.cs
- FrameDimension.cs
- PermissionToken.cs
- OleDbException.cs
- AutomationTextAttribute.cs
- WindowsContainer.cs
- _KerberosClient.cs
- CurrencyManager.cs
- X509IssuerSerialKeyIdentifierClause.cs
- SqlUserDefinedTypeAttribute.cs
- TreeWalkHelper.cs
- CellTreeNode.cs
- XmlAtomicValue.cs
- FilterInvalidBodyAccessException.cs
- documentation.cs
- UnauthorizedAccessException.cs
- CryptoHelper.cs
- XmlBoundElement.cs
- EntityClientCacheKey.cs
- ListItem.cs
- SmiMetaData.cs
- AuthenticationConfig.cs
- DateTimeValueSerializerContext.cs
- BitmapEffectState.cs