Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Serializers / AtomServiceDocumentSerializer.cs / 1305376 / AtomServiceDocumentSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a serializer for the Atom Service Document format. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System; using System.Data.Services.Providers; using System.Diagnostics; using System.IO; using System.Text; using System.Xml; ////// Provides support for serializing service models as /// a Service Document. /// ////// For more information, see http://tools.ietf.org/html/rfc5023#section-8. /// [DebuggerDisplay("AtomServiceDocumentSerializer={baseUri}")] internal sealed class AtomServiceDocumentSerializer : XmlDocumentSerializer { ///XML prefix for the Atom Publishing Protocol namespace. private const string AppNamespacePrefix = "app"; ///XML prefix for the Atom namespace. private const string AtomNamespacePrefix = "atom"; ////// Initializes a new AtomServiceDocumentSerializer, ready to write /// out the Service Document for a data provider. /// /// Stream to which output should be sent. /// Base URI from which resources should be resolved. /// Data provider from which metadata should be gathered. /// Text encoding for the response. internal AtomServiceDocumentSerializer( Stream output, Uri baseUri, DataServiceProviderWrapper provider, Encoding encoding) : base(output, baseUri, provider, encoding) { } ///Writes the Service Document to the output stream. /// Data service instance. internal override void WriteRequest(IDataService service) { try { this.Writer.WriteStartElement(XmlConstants.AtomPublishingServiceElementName, XmlConstants.AppNamespace); this.IncludeCommonNamespaces(); this.Writer.WriteStartElement("", XmlConstants.AtomPublishingWorkspaceElementName, XmlConstants.AppNamespace); this.Writer.WriteStartElement(XmlConstants.AtomTitleElementName, XmlConstants.AtomNamespace); this.Writer.WriteString(XmlConstants.AtomPublishingWorkspaceDefaultValue); this.Writer.WriteEndElement(); foreach (ResourceSetWrapper container in this.Provider.ResourceSets) { this.Writer.WriteStartElement("", XmlConstants.AtomPublishingCollectionElementName, XmlConstants.AppNamespace); this.Writer.WriteAttributeString(XmlConstants.AtomHRefAttributeName, container.Name); this.Writer.WriteStartElement(XmlConstants.AtomTitleElementName, XmlConstants.AtomNamespace); this.Writer.WriteString(container.Name); this.Writer.WriteEndElement(); // Close 'title' element. this.Writer.WriteEndElement(); // Close 'collection' element. } this.Writer.WriteEndElement(); // Close 'workspace' element. this.Writer.WriteEndElement(); // Close 'service' element. } finally { this.Writer.Close(); } } ///Ensures that common namespaces are included in the topmost tag. ////// This method should be called by any method that may write a /// topmost element tag. /// private void IncludeCommonNamespaces() { Debug.Assert( this.Writer.WriteState == WriteState.Element, "this.writer.WriteState == WriteState.Element - otherwise, not called at beginning - " + this.Writer.WriteState); this.Writer.WriteAttributeString(XmlConstants.XmlNamespacePrefix, XmlConstants.XmlBaseAttributeName, null, this.BaseUri.AbsoluteUri); this.Writer.WriteAttributeString(XmlConstants.XmlnsNamespacePrefix, AtomNamespacePrefix, null, XmlConstants.AtomNamespace); this.Writer.WriteAttributeString(XmlConstants.XmlnsNamespacePrefix, AppNamespacePrefix, null, XmlConstants.AppNamespace); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
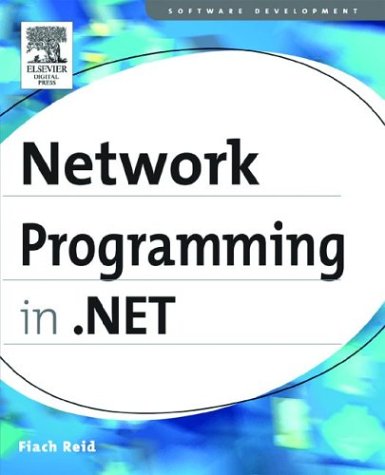
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigXmlWhitespace.cs
- SystemPens.cs
- DataServiceHost.cs
- DataGridHeaderBorder.cs
- Gdiplus.cs
- HighlightComponent.cs
- RSAPKCS1KeyExchangeFormatter.cs
- PointLightBase.cs
- FontFamily.cs
- AppDomainFactory.cs
- StylusCollection.cs
- HijriCalendar.cs
- HttpWebRequestElement.cs
- NonBatchDirectoryCompiler.cs
- BinaryFormatter.cs
- FrameworkReadOnlyPropertyMetadata.cs
- WizardStepBase.cs
- TextTrailingWordEllipsis.cs
- SynchronizedDispatch.cs
- FilterException.cs
- UshortList2.cs
- sqlser.cs
- PersistenceContextEnlistment.cs
- SemaphoreFullException.cs
- CommonObjectSecurity.cs
- GraphicsContext.cs
- IOException.cs
- ProfilePropertyNameValidator.cs
- ContentValidator.cs
- GridViewHeaderRowPresenter.cs
- TextContainerChangedEventArgs.cs
- TransformerTypeCollection.cs
- BordersPage.cs
- DocumentOrderQuery.cs
- WebSysDescriptionAttribute.cs
- SqlBulkCopyColumnMapping.cs
- ReadOnlyHierarchicalDataSourceView.cs
- NeedSkipTokenVisitor.cs
- NumberEdit.cs
- XmlBinaryReader.cs
- LOSFormatter.cs
- behaviorssection.cs
- EastAsianLunisolarCalendar.cs
- EnumDataContract.cs
- CheckBox.cs
- QuerySettings.cs
- IOException.cs
- UIPermission.cs
- HexParser.cs
- InvalidFilterCriteriaException.cs
- QilFactory.cs
- MSAAWinEventWrap.cs
- BufferedGenericXmlSecurityToken.cs
- SecurityDescriptor.cs
- ProviderCollection.cs
- WebException.cs
- DataGridRowClipboardEventArgs.cs
- ObjectComplexPropertyMapping.cs
- ControlFilterExpression.cs
- SqlResolver.cs
- CryptoApi.cs
- ClrProviderManifest.cs
- Assert.cs
- EntityCommandCompilationException.cs
- Single.cs
- Canvas.cs
- SqlFunctionAttribute.cs
- HtmlSelect.cs
- ProfileBuildProvider.cs
- StringFreezingAttribute.cs
- BindingCompleteEventArgs.cs
- KeyValueSerializer.cs
- URLMembershipCondition.cs
- UnlockInstanceCommand.cs
- LicenseException.cs
- LinqExpressionNormalizer.cs
- TabletDevice.cs
- InputBinding.cs
- ConditionalExpression.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- FrameworkContentElement.cs
- CfgParser.cs
- Slider.cs
- ClientScriptItemCollection.cs
- Composition.cs
- EditingScope.cs
- DataGridToolTip.cs
- RelationshipEnd.cs
- EntityDataSourceDataSelection.cs
- Error.cs
- CultureMapper.cs
- RegexParser.cs
- SystemIcmpV4Statistics.cs
- LineServices.cs
- LocalizeDesigner.cs
- Solver.cs
- MimeMapping.cs
- UserControlDesigner.cs
- xsdvalidator.cs
- StatusBarDesigner.cs