Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / FontUnit.cs / 2 / FontUnit.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; using System.Web.Util; ////// [ TypeConverterAttribute(typeof(FontUnitConverter)) ] [Serializable] public struct FontUnit { ///Respresent the font unit. ////// public static readonly FontUnit Empty = new FontUnit(); ///Specifies an empty ///. This field is read only. /// public static readonly FontUnit Smaller = new FontUnit(FontSize.Smaller); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit Larger = new FontUnit(FontSize.Larger); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit XXSmall = new FontUnit(FontSize.XXSmall); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit XSmall = new FontUnit(FontSize.XSmall); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit Small = new FontUnit(FontSize.Small); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit Medium = new FontUnit(FontSize.Medium); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit Large = new FontUnit(FontSize.Large); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit XLarge = new FontUnit(FontSize.XLarge); ///Specifies a ///with /// font. This field is read only. /// Specifies a public static readonly FontUnit XXLarge = new FontUnit(FontSize.XXLarge); private readonly FontSize type; private readonly Unit value; ///with /// font. This field is read only. /// /// public FontUnit(FontSize type) { if (type < FontSize.NotSet || type > FontSize.XXLarge) { throw new ArgumentOutOfRangeException("type"); } this.type = type; if (this.type == FontSize.AsUnit) { value = Unit.Point(10); } else { value = Unit.Empty; } } ///Initializes a new instance of the ///class with a . /// public FontUnit(Unit value) { this.type = FontSize.NotSet; if (value.IsEmpty == false) { this.type = FontSize.AsUnit; this.value = value; } else { this.value = Unit.Empty; } } ///Initializes a new instance of the ///class with a . /// public FontUnit(int value) { this.type = FontSize.AsUnit; this.value = Unit.Point(value); } ///Initializes a new instance of the ///class with an integer value. /// public FontUnit(double value) : this(new Unit(value, UnitType.Point)) { } ///Initializes a new instance of the ///class with a double value. /// public FontUnit(double value, UnitType type) : this(new Unit(value, type)) { } ///Initializes a new instance of the ///class with a double value. /// public FontUnit(string value) : this(value, CultureInfo.CurrentCulture) { } ///Initializes a new instance of the ///class with a string. /// public FontUnit(string value, CultureInfo culture) { this.type = FontSize.NotSet; this.value = Unit.Empty; if (!String.IsNullOrEmpty(value)) { // This is invariant because it acts like an enum with a number together. // The enum part is invariant, but the number uses current culture. char firstChar = Char.ToLower(value[0], CultureInfo.InvariantCulture); if (firstChar == 'x') { if (String.Equals(value, "xx-small", StringComparison.OrdinalIgnoreCase) || String.Equals(value, "xxsmall", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.XXSmall; return; } else if (String.Equals(value, "x-small", StringComparison.OrdinalIgnoreCase) || String.Equals(value, "xsmall", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.XSmall; return; } else if (String.Equals(value, "x-large", StringComparison.OrdinalIgnoreCase) || String.Equals(value, "xlarge", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.XLarge; return; } else if (String.Equals(value, "xx-large", StringComparison.OrdinalIgnoreCase) || String.Equals(value, "xxlarge", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.XXLarge; return; } } else if (firstChar == 's') { if (String.Equals(value, "small", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Small; return; } else if (String.Equals(value, "smaller", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Smaller; return; } } else if (firstChar == 'l') { if (String.Equals(value, "large", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Large; return; } if (String.Equals(value, "larger", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Larger; return; } } else if ((firstChar == 'm') && String.Equals(value, "medium", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Medium; return; } this.value = new Unit(value, culture, UnitType.Point); this.type = FontSize.AsUnit; } } ///[To be supplied.] ////// public bool IsEmpty { get { return type == FontSize.NotSet; } } ///Indicates whether the font size has been set. ////// public FontSize Type { get { return type; } } ///Indicates the font size by type. ////// public Unit Unit { get { return value; } } ///Indicates the font size by ///. /// public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(type.GetHashCode(), value.GetHashCode()); } ///[To be supplied.] ////// public override bool Equals(object obj) { if (obj == null || !(obj is FontUnit)) return false; FontUnit f = (FontUnit)obj; if ((f.type == type) && (f.value == value)) { return true; } return false; } ///Determines if the specified ///is equivilent to the represented by this instance. /// public static bool operator ==(FontUnit left, FontUnit right) { return ((left.type == right.type) && (left.value == right.value)); } ///Compares two ///objects for equality. /// public static bool operator !=(FontUnit left, FontUnit right) { return ((left.type != right.type) || (left.value != right.value)); } ///Compares two ///objects /// for inequality. /// public static FontUnit Parse(string s) { return new FontUnit(s, CultureInfo.InvariantCulture); } ///[To be supplied.] ////// public static FontUnit Parse(string s, CultureInfo culture) { return new FontUnit(s, culture); } ///[To be supplied.] ////// public static FontUnit Point(int n) { return new FontUnit(n); } ///Creates a ///of type Point from an integer value. /// public override string ToString() { return ToString((IFormatProvider)CultureInfo.CurrentCulture); } public string ToString(CultureInfo culture) { return ToString((IFormatProvider)culture); } public string ToString(IFormatProvider formatProvider) { string s = String.Empty; if (IsEmpty) return s; switch (type) { case FontSize.AsUnit: s = value.ToString(formatProvider); break; case FontSize.XXSmall: s = "XX-Small"; break; case FontSize.XSmall: s = "X-Small"; break; case FontSize.XLarge: s = "X-Large"; break; case FontSize.XXLarge: s = "XX-Large"; break; default: s = PropertyConverter.EnumToString(typeof(FontSize), type); break; } return s; } ///Convert a ///to a string. /// public static implicit operator FontUnit(int n) { return FontUnit.Point(n); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //Implicitly creates a ///of type Point from an integer value. // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; using System.Web.Util; ////// [ TypeConverterAttribute(typeof(FontUnitConverter)) ] [Serializable] public struct FontUnit { ///Respresent the font unit. ////// public static readonly FontUnit Empty = new FontUnit(); ///Specifies an empty ///. This field is read only. /// public static readonly FontUnit Smaller = new FontUnit(FontSize.Smaller); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit Larger = new FontUnit(FontSize.Larger); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit XXSmall = new FontUnit(FontSize.XXSmall); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit XSmall = new FontUnit(FontSize.XSmall); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit Small = new FontUnit(FontSize.Small); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit Medium = new FontUnit(FontSize.Medium); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit Large = new FontUnit(FontSize.Large); ///Specifies a ///with /// font. This field is read only. /// public static readonly FontUnit XLarge = new FontUnit(FontSize.XLarge); ///Specifies a ///with /// font. This field is read only. /// Specifies a public static readonly FontUnit XXLarge = new FontUnit(FontSize.XXLarge); private readonly FontSize type; private readonly Unit value; ///with /// font. This field is read only. /// /// public FontUnit(FontSize type) { if (type < FontSize.NotSet || type > FontSize.XXLarge) { throw new ArgumentOutOfRangeException("type"); } this.type = type; if (this.type == FontSize.AsUnit) { value = Unit.Point(10); } else { value = Unit.Empty; } } ///Initializes a new instance of the ///class with a . /// public FontUnit(Unit value) { this.type = FontSize.NotSet; if (value.IsEmpty == false) { this.type = FontSize.AsUnit; this.value = value; } else { this.value = Unit.Empty; } } ///Initializes a new instance of the ///class with a . /// public FontUnit(int value) { this.type = FontSize.AsUnit; this.value = Unit.Point(value); } ///Initializes a new instance of the ///class with an integer value. /// public FontUnit(double value) : this(new Unit(value, UnitType.Point)) { } ///Initializes a new instance of the ///class with a double value. /// public FontUnit(double value, UnitType type) : this(new Unit(value, type)) { } ///Initializes a new instance of the ///class with a double value. /// public FontUnit(string value) : this(value, CultureInfo.CurrentCulture) { } ///Initializes a new instance of the ///class with a string. /// public FontUnit(string value, CultureInfo culture) { this.type = FontSize.NotSet; this.value = Unit.Empty; if (!String.IsNullOrEmpty(value)) { // This is invariant because it acts like an enum with a number together. // The enum part is invariant, but the number uses current culture. char firstChar = Char.ToLower(value[0], CultureInfo.InvariantCulture); if (firstChar == 'x') { if (String.Equals(value, "xx-small", StringComparison.OrdinalIgnoreCase) || String.Equals(value, "xxsmall", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.XXSmall; return; } else if (String.Equals(value, "x-small", StringComparison.OrdinalIgnoreCase) || String.Equals(value, "xsmall", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.XSmall; return; } else if (String.Equals(value, "x-large", StringComparison.OrdinalIgnoreCase) || String.Equals(value, "xlarge", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.XLarge; return; } else if (String.Equals(value, "xx-large", StringComparison.OrdinalIgnoreCase) || String.Equals(value, "xxlarge", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.XXLarge; return; } } else if (firstChar == 's') { if (String.Equals(value, "small", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Small; return; } else if (String.Equals(value, "smaller", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Smaller; return; } } else if (firstChar == 'l') { if (String.Equals(value, "large", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Large; return; } if (String.Equals(value, "larger", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Larger; return; } } else if ((firstChar == 'm') && String.Equals(value, "medium", StringComparison.OrdinalIgnoreCase)) { this.type = FontSize.Medium; return; } this.value = new Unit(value, culture, UnitType.Point); this.type = FontSize.AsUnit; } } ///[To be supplied.] ////// public bool IsEmpty { get { return type == FontSize.NotSet; } } ///Indicates whether the font size has been set. ////// public FontSize Type { get { return type; } } ///Indicates the font size by type. ////// public Unit Unit { get { return value; } } ///Indicates the font size by ///. /// public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(type.GetHashCode(), value.GetHashCode()); } ///[To be supplied.] ////// public override bool Equals(object obj) { if (obj == null || !(obj is FontUnit)) return false; FontUnit f = (FontUnit)obj; if ((f.type == type) && (f.value == value)) { return true; } return false; } ///Determines if the specified ///is equivilent to the represented by this instance. /// public static bool operator ==(FontUnit left, FontUnit right) { return ((left.type == right.type) && (left.value == right.value)); } ///Compares two ///objects for equality. /// public static bool operator !=(FontUnit left, FontUnit right) { return ((left.type != right.type) || (left.value != right.value)); } ///Compares two ///objects /// for inequality. /// public static FontUnit Parse(string s) { return new FontUnit(s, CultureInfo.InvariantCulture); } ///[To be supplied.] ////// public static FontUnit Parse(string s, CultureInfo culture) { return new FontUnit(s, culture); } ///[To be supplied.] ////// public static FontUnit Point(int n) { return new FontUnit(n); } ///Creates a ///of type Point from an integer value. /// public override string ToString() { return ToString((IFormatProvider)CultureInfo.CurrentCulture); } public string ToString(CultureInfo culture) { return ToString((IFormatProvider)culture); } public string ToString(IFormatProvider formatProvider) { string s = String.Empty; if (IsEmpty) return s; switch (type) { case FontSize.AsUnit: s = value.ToString(formatProvider); break; case FontSize.XXSmall: s = "XX-Small"; break; case FontSize.XSmall: s = "X-Small"; break; case FontSize.XLarge: s = "X-Large"; break; case FontSize.XXLarge: s = "XX-Large"; break; default: s = PropertyConverter.EnumToString(typeof(FontSize), type); break; } return s; } ///Convert a ///to a string. /// public static implicit operator FontUnit(int n) { return FontUnit.Point(n); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Implicitly creates a ///of type Point from an integer value.
Link Menu
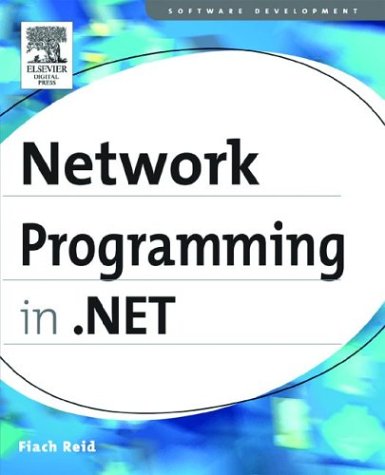
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmptyEnumerator.cs
- IndentTextWriter.cs
- DLinqDataModelProvider.cs
- DataObjectMethodAttribute.cs
- GridItemProviderWrapper.cs
- RtfToXamlLexer.cs
- CommentEmitter.cs
- WS2007FederationHttpBindingCollectionElement.cs
- DataGridViewComboBoxColumn.cs
- ValidationSummary.cs
- SqlProviderUtilities.cs
- ListViewItemEventArgs.cs
- Regex.cs
- XPathSingletonIterator.cs
- TextCollapsingProperties.cs
- BufferedGraphicsManager.cs
- PenContext.cs
- LowerCaseStringConverter.cs
- Rect.cs
- URLMembershipCondition.cs
- NavigationExpr.cs
- CorrelationTokenInvalidatedHandler.cs
- RowParagraph.cs
- TemplateBindingExpressionConverter.cs
- DriveNotFoundException.cs
- CoreSwitches.cs
- UndirectedGraph.cs
- DataGridViewCellConverter.cs
- XmlSchemas.cs
- ParseChildrenAsPropertiesAttribute.cs
- AdapterUtil.cs
- ToolboxItemCollection.cs
- SmiGettersStream.cs
- Literal.cs
- DynamicActionMessageFilter.cs
- AssociationSetEnd.cs
- SqlProviderUtilities.cs
- HttpException.cs
- TextServicesDisplayAttribute.cs
- ListViewUpdatedEventArgs.cs
- QueryOutputWriter.cs
- Completion.cs
- ThemeDictionaryExtension.cs
- BrowserDefinition.cs
- Helpers.cs
- ToolStripDropDownClosingEventArgs.cs
- TableLayoutCellPaintEventArgs.cs
- Span.cs
- PassportAuthenticationEventArgs.cs
- LoginUtil.cs
- GetIsBrowserClientRequest.cs
- Help.cs
- CodeLinePragma.cs
- SoapCommonClasses.cs
- ObjectNavigationPropertyMapping.cs
- NodeFunctions.cs
- OverflowException.cs
- InvalidComObjectException.cs
- CasesDictionary.cs
- PropertyToken.cs
- ComponentChangingEvent.cs
- SchemaCollectionPreprocessor.cs
- UnsignedPublishLicense.cs
- XmlName.cs
- AnnotationHighlightLayer.cs
- TemplateControl.cs
- SoapException.cs
- BamlRecordWriter.cs
- StreamDocument.cs
- QuaternionAnimationUsingKeyFrames.cs
- DataGridViewSortCompareEventArgs.cs
- AnnotationResourceCollection.cs
- ContentControl.cs
- MarginsConverter.cs
- WindowsEditBoxRange.cs
- FastPropertyAccessor.cs
- selecteditemcollection.cs
- AspNetSynchronizationContext.cs
- CommandTreeTypeHelper.cs
- ProfessionalColors.cs
- GeneralTransform3DGroup.cs
- GridItem.cs
- MimePart.cs
- GenericsInstances.cs
- HttpRuntimeSection.cs
- SqlMethodAttribute.cs
- BmpBitmapDecoder.cs
- ProfileGroupSettings.cs
- TimelineGroup.cs
- RelationshipNavigation.cs
- RSAOAEPKeyExchangeFormatter.cs
- ListViewSelectEventArgs.cs
- DynamicRenderer.cs
- StrongNameUtility.cs
- AsymmetricKeyExchangeFormatter.cs
- TemplateInstanceAttribute.cs
- Table.cs
- ArglessEventHandlerProxy.cs
- TextRange.cs
- StylusPointPropertyUnit.cs