Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / Utils / Helpers.cs / 2 / Helpers.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Diagnostics; namespace System.Data.Common.Utils { // Miscellaneous helper routines internal static class Helpers { #region Trace methods // effects: Trace args according to the CLR format string with a new line internal static void FormatTraceLine(string format, params object[] args) { Trace.WriteLine(String.Format(CultureInfo.InvariantCulture, format, args)); } // effects: Trace the string with a new line internal static void StringTrace(string arg) { Trace.Write(arg); } // effects: Trace the string without adding a new line internal static void StringTraceLine(string arg) { Trace.WriteLine(arg); } #endregion #region Misc Helpers // effects: compares two sets using the given comparer - removes // duplicates if they exist internal static bool IsSetEqual(IEnumerable list1, IEnumerable list2, IEqualityComparer comparer) { Set set1 = new Set (list1, comparer); Set set2 = new Set (list2, comparer); return set1.SetEquals(set2); } // effects: Given a stream of values of type "SubType", returns a // stream of values of type "SuperType" where SuperType is a // superclass/supertype of SubType internal static IEnumerable AsSuperTypeList (IEnumerable values) where SubType : SuperType { foreach (SubType value in values) { yield return value; } } /// /// Builds a balanced binary tree with the specified nodes as leaves. /// Note that the current elements of ///MAY be overwritten /// as the leaves are combined to produce the tree. /// The type of each node in the tree /// The leaf nodes to combine into an balanced binary tree /// A function that produces a new node that is the combination of the two specified argument nodes ///The single node that is the root of the balanced binary tree internal static TNode BuildBalancedTreeInPlace(IList nodes, Func combinator) { EntityUtil.CheckArgumentNull(nodes, "nodes"); EntityUtil.CheckArgumentNull(combinator, "combinator"); Debug.Assert(nodes.Count > 0, "At least one node is required"); // If only one node is present, return the single node. if (nodes.Count == 1) { return nodes[0]; } // For the two-node case, simply combine the two nodes and return the result. if (nodes.Count == 2) { return combinator(nodes[0], nodes[1]); } // // Build the balanced tree in a bottom-up fashion. // On each iteration, an even number of nodes are paired off using the // combinator function, reducing the total number of available leaf nodes // by half each time. If the number of nodes in an iteration is not even, // the 'last' node in the set is omitted, then combined with the last pair // that is produced. // Nodes are collected from left to right with newly combined nodes overwriting // nodes from the previous iteration that have already been consumed (as can // be seen by 'writePos' lagging 'readPos' in the main statement of the loop below). // When a single available leaf node remains, this node is the root of the // balanced binary tree and can be returned to the caller. // int nodesToPair = nodes.Count; while (nodesToPair != 1) { bool combineModulo = ((nodesToPair & 0x1) == 1); if (combineModulo) { nodesToPair--; } int writePos = 0; for (int readPos = 0; readPos < nodesToPair; readPos += 2) { nodes[writePos++] = combinator(nodes[readPos], nodes[readPos + 1]); } if (combineModulo) { int updatePos = writePos - 1; nodes[updatePos] = combinator(nodes[updatePos], nodes[nodesToPair]); } nodesToPair /= 2; } return nodes[0]; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Diagnostics; namespace System.Data.Common.Utils { // Miscellaneous helper routines internal static class Helpers { #region Trace methods // effects: Trace args according to the CLR format string with a new line internal static void FormatTraceLine(string format, params object[] args) { Trace.WriteLine(String.Format(CultureInfo.InvariantCulture, format, args)); } // effects: Trace the string with a new line internal static void StringTrace(string arg) { Trace.Write(arg); } // effects: Trace the string without adding a new line internal static void StringTraceLine(string arg) { Trace.WriteLine(arg); } #endregion #region Misc Helpers // effects: compares two sets using the given comparer - removes // duplicates if they exist internal static bool IsSetEqual(IEnumerable list1, IEnumerable list2, IEqualityComparer comparer) { Set set1 = new Set (list1, comparer); Set set2 = new Set (list2, comparer); return set1.SetEquals(set2); } // effects: Given a stream of values of type "SubType", returns a // stream of values of type "SuperType" where SuperType is a // superclass/supertype of SubType internal static IEnumerable AsSuperTypeList (IEnumerable values) where SubType : SuperType { foreach (SubType value in values) { yield return value; } } /// /// Builds a balanced binary tree with the specified nodes as leaves. /// Note that the current elements of ///MAY be overwritten /// as the leaves are combined to produce the tree. /// The type of each node in the tree /// The leaf nodes to combine into an balanced binary tree /// A function that produces a new node that is the combination of the two specified argument nodes ///The single node that is the root of the balanced binary tree internal static TNode BuildBalancedTreeInPlace(IList nodes, Func combinator) { EntityUtil.CheckArgumentNull(nodes, "nodes"); EntityUtil.CheckArgumentNull(combinator, "combinator"); Debug.Assert(nodes.Count > 0, "At least one node is required"); // If only one node is present, return the single node. if (nodes.Count == 1) { return nodes[0]; } // For the two-node case, simply combine the two nodes and return the result. if (nodes.Count == 2) { return combinator(nodes[0], nodes[1]); } // // Build the balanced tree in a bottom-up fashion. // On each iteration, an even number of nodes are paired off using the // combinator function, reducing the total number of available leaf nodes // by half each time. If the number of nodes in an iteration is not even, // the 'last' node in the set is omitted, then combined with the last pair // that is produced. // Nodes are collected from left to right with newly combined nodes overwriting // nodes from the previous iteration that have already been consumed (as can // be seen by 'writePos' lagging 'readPos' in the main statement of the loop below). // When a single available leaf node remains, this node is the root of the // balanced binary tree and can be returned to the caller. // int nodesToPair = nodes.Count; while (nodesToPair != 1) { bool combineModulo = ((nodesToPair & 0x1) == 1); if (combineModulo) { nodesToPair--; } int writePos = 0; for (int readPos = 0; readPos < nodesToPair; readPos += 2) { nodes[writePos++] = combinator(nodes[readPos], nodes[readPos + 1]); } if (combineModulo) { int updatePos = writePos - 1; nodes[updatePos] = combinator(nodes[updatePos], nodes[nodesToPair]); } nodesToPair /= 2; } return nodes[0]; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
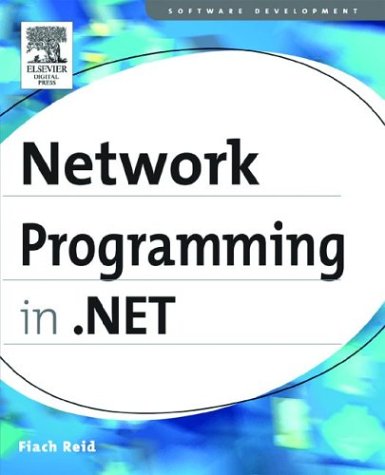
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReadOnlyDictionary.cs
- ViewValidator.cs
- DirectoryObjectSecurity.cs
- TraceProvider.cs
- ExpressionBuilderCollection.cs
- CollectionChange.cs
- DataSet.cs
- WebPartAddingEventArgs.cs
- CookieHandler.cs
- CategoriesDocumentFormatter.cs
- AuthorizationRuleCollection.cs
- ImplicitInputBrush.cs
- XmlCollation.cs
- Calendar.cs
- HashCryptoHandle.cs
- ProjectionPruner.cs
- KeyValueConfigurationCollection.cs
- HTMLTagNameToTypeMapper.cs
- ValuePatternIdentifiers.cs
- DBCommand.cs
- StrokeNodeData.cs
- RSAPKCS1SignatureFormatter.cs
- UpdateCompiler.cs
- DataListItemCollection.cs
- AppDomainAttributes.cs
- UserControlParser.cs
- DesignerProperties.cs
- XmlProcessingInstruction.cs
- Debug.cs
- ResourcePool.cs
- HtmlTextArea.cs
- PropertyReference.cs
- AutomationAttributeInfo.cs
- HtmlHead.cs
- M3DUtil.cs
- ValidationError.cs
- WsdlContractConversionContext.cs
- MulticastNotSupportedException.cs
- HttpModuleAction.cs
- DomainUpDown.cs
- XamlPoint3DCollectionSerializer.cs
- MessageSmuggler.cs
- EventRouteFactory.cs
- HyperLinkColumn.cs
- WebConfigurationManager.cs
- RelatedImageListAttribute.cs
- Number.cs
- TabPanel.cs
- PassportAuthenticationEventArgs.cs
- WindowsTab.cs
- TreeIterators.cs
- Block.cs
- ApplicationFileParser.cs
- LineProperties.cs
- EntryWrittenEventArgs.cs
- WindowsIPAddress.cs
- TrackingProfileCache.cs
- Helpers.cs
- Rect3D.cs
- Calendar.cs
- ItemsControl.cs
- WindowsGraphicsCacheManager.cs
- ZoneMembershipCondition.cs
- TransformProviderWrapper.cs
- UndoManager.cs
- DataObjectCopyingEventArgs.cs
- SecurityDocument.cs
- UrlAuthorizationModule.cs
- DeclarationUpdate.cs
- NameValuePair.cs
- HttpCacheParams.cs
- DispatcherExceptionFilterEventArgs.cs
- ArgumentNullException.cs
- AutoGeneratedFieldProperties.cs
- StatusBarDrawItemEvent.cs
- TypedDataSetSchemaImporterExtension.cs
- PackageRelationshipSelector.cs
- FontNamesConverter.cs
- BevelBitmapEffect.cs
- PixelShader.cs
- DataGridViewLayoutData.cs
- InvalidOperationException.cs
- RoleManagerSection.cs
- RegistrySecurity.cs
- DBDataPermission.cs
- ProfileServiceManager.cs
- DynamicILGenerator.cs
- RoleServiceManager.cs
- AttributeProviderAttribute.cs
- CalendarAutoFormatDialog.cs
- IntSecurity.cs
- IPPacketInformation.cs
- EmptyStringExpandableObjectConverter.cs
- BulletedList.cs
- CacheForPrimitiveTypes.cs
- UserPreferenceChangedEventArgs.cs
- MeasureItemEvent.cs
- PrinterResolution.cs
- HierarchicalDataBoundControlAdapter.cs
- IPPacketInformation.cs