Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Expressions / PropertyReference.cs / 1305376 / PropertyReference.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Expressions { using System.ComponentModel; using System.Reflection; using System.Runtime; using System.Runtime.Serialization; public sealed class PropertyReference: CodeActivity > { PropertyInfo propertyInfo; [DefaultValue(null)] public string PropertyName { get; set; } public InArgument Operand { get; set; } protected override void CacheMetadata(CodeActivityMetadata metadata) { bool isRequired = false; if (typeof(TOperand).IsEnum) { metadata.AddValidationError(SR.TargetTypeCannotBeEnum(this.GetType().Name, this.DisplayName)); } else if (typeof(TOperand).IsValueType) { metadata.AddValidationError(SR.TargetTypeIsValueType(this.GetType().Name, this.DisplayName)); } if (string.IsNullOrEmpty(this.PropertyName)) { metadata.AddValidationError(SR.ActivityPropertyMustBeSet("PropertyName", this.DisplayName)); } else { Type operandType = typeof(TOperand); this.propertyInfo = operandType.GetProperty(this.PropertyName); if (this.propertyInfo == null) { metadata.AddValidationError(SR.MemberNotFound(PropertyName, typeof(TOperand).Name)); } else { MethodInfo getMethod = this.propertyInfo.GetGetMethod(); MethodInfo setMethod = this.propertyInfo.GetSetMethod(); // Only allow access to public properties, EXCEPT that Locations are top-level variables // from the other's perspective, not internal properties, so they're okay as a special case. // E.g. "[N]" from the user's perspective is not accessing a nonpublic property, even though // at an implementation level it is. if (setMethod == null && TypeHelper.AreTypesCompatible(this.propertyInfo.DeclaringType, typeof(Location)) == false) { metadata.AddValidationError(SR.ReadonlyPropertyCannotBeSet(this.propertyInfo.DeclaringType, this.propertyInfo.Name)); } if ((getMethod != null && !getMethod.IsStatic) || (setMethod != null && !setMethod.IsStatic)) { isRequired = true; } } } MemberExpressionHelper.AddOperandArgument(metadata, this.Operand, isRequired); } protected override Location Execute(CodeActivityContext context) { Fx.Assert(this.propertyInfo != null, "propertyInfo must not be null"); return new PropertyLocation (this.propertyInfo, this.Operand.Get(context)); } [DataContract] class PropertyLocation : Location { [DataMember(EmitDefaultValue = false)] object owner; [DataMember] PropertyInfo propertyInfo; public PropertyLocation(PropertyInfo propertyInfo, object owner) : base() { this.propertyInfo = propertyInfo; this.owner = owner; } public override T Value { get { // Only allow access to public properties, EXCEPT that Locations are top-level variables // from the other's perspective, not internal properties, so they're okay as a special case. // E.g. "[N]" from the user's perspective is not accessing a nonpublic property, even though // at an implementation level it is. if (this.propertyInfo.GetGetMethod() == null && TypeHelper.AreTypesCompatible(this.propertyInfo.DeclaringType, typeof(Location)) == false) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.WriteonlyPropertyCannotBeRead(this.propertyInfo.DeclaringType, this.propertyInfo.Name))); } return (T)this.propertyInfo.GetValue(this.owner, null); } set { this.propertyInfo.SetValue(this.owner, value, null); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
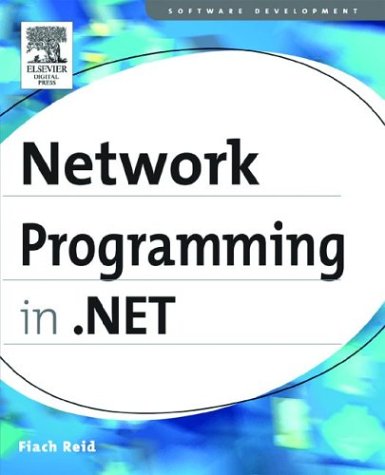
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ManagedIStream.cs
- OleDbMetaDataFactory.cs
- Clause.cs
- PrinterResolution.cs
- TokenBasedSet.cs
- MouseEvent.cs
- Style.cs
- SymmetricSecurityBindingElement.cs
- filewebrequest.cs
- SqlCacheDependencyDatabaseCollection.cs
- PagerSettings.cs
- SoapMessage.cs
- WebBrowserContainer.cs
- SpeechEvent.cs
- WorkflowNamespace.cs
- RsaSecurityTokenParameters.cs
- XPathDocumentNavigator.cs
- SiteMapHierarchicalDataSourceView.cs
- TextTreeTextBlock.cs
- MetadataSource.cs
- typedescriptorpermission.cs
- FrameworkTemplate.cs
- NullableConverter.cs
- PublisherIdentityPermission.cs
- LeftCellWrapper.cs
- Trustee.cs
- CellCreator.cs
- ChildTable.cs
- MimePart.cs
- HttpVersion.cs
- EditorAttribute.cs
- UpdatePanelTriggerCollection.cs
- TimeIntervalCollection.cs
- Point.cs
- WorkItem.cs
- IPEndPointCollection.cs
- GridViewItemAutomationPeer.cs
- FixedDocumentSequencePaginator.cs
- HtmlTernaryTree.cs
- ScrollBarRenderer.cs
- DataMember.cs
- AutomationEvent.cs
- CustomSignedXml.cs
- MsmqIntegrationInputMessage.cs
- Type.cs
- WorkflowApplicationEventArgs.cs
- HostedElements.cs
- DocumentPaginator.cs
- ContentElement.cs
- AssociativeAggregationOperator.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- FormsAuthenticationConfiguration.cs
- panel.cs
- PeerEndPoint.cs
- Crypto.cs
- StreamGeometry.cs
- XsltArgumentList.cs
- MobileCategoryAttribute.cs
- ParentQuery.cs
- FixUpCollection.cs
- Run.cs
- DesignerActionListCollection.cs
- CollectionDataContract.cs
- CfgSemanticTag.cs
- Padding.cs
- MatcherBuilder.cs
- Synchronization.cs
- DataGridViewImageCell.cs
- EntityDataSourceWizardForm.cs
- TemplateControlCodeDomTreeGenerator.cs
- Win32SafeHandles.cs
- DataObjectAttribute.cs
- PrintPreviewControl.cs
- VisualStateGroup.cs
- XmlDeclaration.cs
- ByteAnimation.cs
- WasAdminWrapper.cs
- BamlRecordReader.cs
- BamlTreeNode.cs
- ProxyElement.cs
- ObjectPropertyMapping.cs
- InheritanceRules.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- D3DImage.cs
- SizeKeyFrameCollection.cs
- CalloutQueueItem.cs
- ObjectCacheHost.cs
- ValidationManager.cs
- FieldToken.cs
- ExtendedPropertyCollection.cs
- XsltCompileContext.cs
- ConvertBinder.cs
- RuleAction.cs
- RequiredFieldValidator.cs
- IdentifierCreationService.cs
- Label.cs
- Partitioner.cs
- ThemeableAttribute.cs
- IndicShape.cs
- GradientBrush.cs