Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Hosting / ObjectCacheHost.cs / 1305376 / ObjectCacheHost.cs
//// Copyright (c) 2009 Microsoft Corporation. All rights reserved. // using System; using System.Runtime.Caching; using System.Runtime.Caching.Hosting; using System.Collections.Generic; using System.Web.Util; namespace System.Web.Hosting { internal sealed class ObjectCacheHost: IServiceProvider, IApplicationIdentifier, IFileChangeNotificationSystem, IMemoryCacheManager { private Object _lock = new Object(); private Dictionary_cacheInfos; internal sealed class FileChangeEventTarget { private OnChangedCallback _onChangedCallback; private FileChangeEventHandler _handler; private void OnChanged(Object sender, FileChangeEvent e) { _onChangedCallback(null); } internal FileChangeEventHandler Handler { get { return _handler; } } internal FileChangeEventTarget(OnChangedCallback onChangedCallback) { _onChangedCallback = onChangedCallback; _handler = new FileChangeEventHandler(this.OnChanged); } } internal sealed class MemoryCacheInfo { internal MemoryCache Cache; internal long Size; } Object IServiceProvider.GetService(Type service) { if (service == typeof(IFileChangeNotificationSystem)) { return this as IFileChangeNotificationSystem; } else if (service == typeof(IMemoryCacheManager)) { return this as IMemoryCacheManager; } else if (service == typeof(IApplicationIdentifier)) { return this as IApplicationIdentifier; } else { return null; } } String IApplicationIdentifier.GetApplicationId() { return HttpRuntime.AppDomainAppIdInternal; } void IFileChangeNotificationSystem.StartMonitoring(string filePath, OnChangedCallback onChangedCallback, out Object state, out DateTimeOffset lastWrite, out long fileSize) { if (filePath == null) { throw new ArgumentNullException("filePath"); } if (onChangedCallback == null) { throw new ArgumentNullException("onChangedCallback"); } FileChangeEventTarget target = new FileChangeEventTarget(onChangedCallback); FileAttributesData fad; HttpRuntime.FileChangesMonitor.StartMonitoringPath(filePath, target.Handler, out fad); if (fad == null) { fad = FileAttributesData.NonExistantAttributesData; } state = target; #if DBG Debug.Assert(fad.UtcLastWriteTime.Kind == DateTimeKind.Utc, "fad.UtcLastWriteTime.Kind == DateTimeKind.Utc"); #endif lastWrite = fad.UtcLastWriteTime; fileSize = fad.FileSize; } void IFileChangeNotificationSystem.StopMonitoring(string filePath, Object state) { if (filePath == null) { throw new ArgumentNullException("filePath"); } if (state == null) { throw new ArgumentNullException("state"); } HttpRuntime.FileChangesMonitor.StopMonitoringPath(filePath, state); } void IMemoryCacheManager.ReleaseCache(MemoryCache memoryCache) { if (memoryCache == null) { throw new ArgumentNullException("memoryCache"); } long delta = 0; lock (_lock) { if (_cacheInfos != null) { MemoryCacheInfo info = null; if (_cacheInfos.TryGetValue(memoryCache, out info)) { delta = 0 - info.Size; _cacheInfos.Remove(memoryCache); } } } if (delta != 0) { ApplicationManager appManager = HostingEnvironment.GetApplicationManager(); if (appManager != null) { appManager.GetUpdatedTotalCacheSize(delta); } } } void IMemoryCacheManager.UpdateCacheSize(long size, MemoryCache memoryCache) { if (memoryCache == null) { throw new ArgumentNullException("memoryCache"); } long delta = 0; lock (_lock) { if (_cacheInfos == null) { _cacheInfos = new Dictionary (); } MemoryCacheInfo info = null; if (!_cacheInfos.TryGetValue(memoryCache, out info)) { info = new MemoryCacheInfo(); info.Cache = memoryCache; _cacheInfos[memoryCache] = info; } delta = size - info.Size; info.Size = size; } ApplicationManager appManager = HostingEnvironment.GetApplicationManager(); if (appManager != null) { appManager.GetUpdatedTotalCacheSize(delta); } } internal long TrimCache(int percent) { long trimmedOrExpired = 0; Dictionary .KeyCollection caches = null; lock (_lock) { if (_cacheInfos != null && _cacheInfos.Count > 0) { caches = _cacheInfos.Keys; } } if (caches != null) { foreach (MemoryCache cache in caches) { trimmedOrExpired += cache.Trim(percent); } } return trimmedOrExpired; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
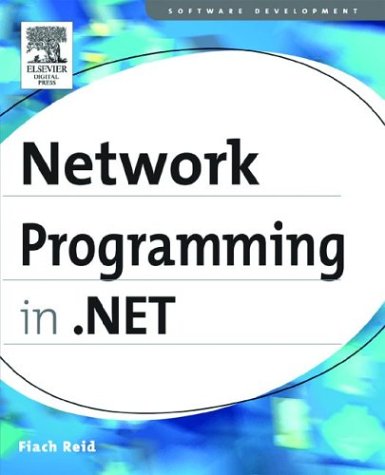
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PersonalizationStateInfo.cs
- ProtectedConfiguration.cs
- CharacterBufferReference.cs
- IgnoreSectionHandler.cs
- CheckBoxPopupAdapter.cs
- ISAPIApplicationHost.cs
- ThicknessConverter.cs
- CallbackValidatorAttribute.cs
- SerialStream.cs
- CharacterString.cs
- WebPartDisplayModeEventArgs.cs
- TypeConverterMarkupExtension.cs
- EventPrivateKey.cs
- PropertyDescriptorCollection.cs
- DSASignatureDeformatter.cs
- ClaimSet.cs
- ConfigurationManagerInternalFactory.cs
- RelationshipConstraintValidator.cs
- isolationinterop.cs
- DLinqTableProvider.cs
- WebConfigManager.cs
- ListBindingHelper.cs
- DataGridViewColumnCollectionEditor.cs
- WeakHashtable.cs
- SqlClientFactory.cs
- PageBuildProvider.cs
- X509IssuerSerialKeyIdentifierClause.cs
- HttpNamespaceReservationInstallComponent.cs
- ExpressionBuilderContext.cs
- TimeoutValidationAttribute.cs
- WmlObjectListAdapter.cs
- DBConcurrencyException.cs
- ServiceNotStartedException.cs
- ParallelTimeline.cs
- GetReadStreamResult.cs
- OpenFileDialog.cs
- CompoundFileReference.cs
- IERequestCache.cs
- ColorInterpolationModeValidation.cs
- PersistenceProviderFactory.cs
- BmpBitmapDecoder.cs
- FileIOPermission.cs
- SimpleLine.cs
- EditorZoneBase.cs
- StatusBar.cs
- Stroke.cs
- XXXInfos.cs
- WorkflowServiceHostFactory.cs
- BuildManager.cs
- FileCodeGroup.cs
- ModifiableIteratorCollection.cs
- SqlMethodAttribute.cs
- SecureUICommand.cs
- BuilderPropertyEntry.cs
- Condition.cs
- NetMsmqSecurityMode.cs
- TextServicesCompartmentEventSink.cs
- DataObjectMethodAttribute.cs
- MexBindingElement.cs
- NullableDoubleMinMaxAggregationOperator.cs
- ParameterCollection.cs
- ContourSegment.cs
- InheritanceContextHelper.cs
- TextClipboardData.cs
- BitHelper.cs
- InfoCardTrace.cs
- MenuStrip.cs
- X509ChainPolicy.cs
- WmpBitmapEncoder.cs
- SimpleType.cs
- SEHException.cs
- InvalidDataContractException.cs
- ReachSerializationCacheItems.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ProviderManager.cs
- SessionStateUtil.cs
- SecondaryViewProvider.cs
- contentDescriptor.cs
- Itemizer.cs
- Compiler.cs
- FixedSOMImage.cs
- AssemblyResourceLoader.cs
- DebugInfo.cs
- RuntimeHelpers.cs
- ServiceTimeoutsElement.cs
- WebPartsSection.cs
- userdatakeys.cs
- Int16Storage.cs
- UnsafeNativeMethods.cs
- XmlWriterTraceListener.cs
- ListViewUpdateEventArgs.cs
- GridItemPatternIdentifiers.cs
- Function.cs
- WebServicesInteroperability.cs
- ScrollItemPattern.cs
- EndpointInfo.cs
- PropertyNames.cs
- StorageModelBuildProvider.cs
- AdRotator.cs
- BinaryFormatter.cs