Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RoamingStoreFile.cs / 1 / RoamingStoreFile.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.Text; using System.Xml; using System.Xml.Serialization; using System.IO; using System.Security.Cryptography.X509Certificates; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Reads and writes the Roaming store xml file format. // internal sealed class RoamingStoreFile { Listm_infocards; public RoamingStoreFile( ) { m_infocards = new List ( ); } // // Summary: // Get the list of cards that will be written or were read. // public IList Cards { get { return m_infocards; } } public void Clear() { foreach( InfoCard icard in m_infocards ) { icard.ClearSensitiveData(); } m_infocards.Clear(); } // // Summary: // Encrypts and writes the data to the specified xml writer. // public void WriteTo( string password, XmlWriter writer ) { byte[] cipherValue = null; byte[] newSalt = null; using( MemoryStream tempStream = new MemoryStream( ) ) { XmlWriterSettings settings = new XmlWriterSettings( ); settings.OmitXmlDeclaration = true; settings.CloseOutput = false; settings.Encoding = Encoding.UTF8; using( XmlWriter eltWriter = XmlWriter.Create( tempStream, settings ) ) { eltWriter.WriteStartElement( XmlNames.WSIdentity.RoamingStoreElement, XmlNames.WSIdentity.Namespace ); foreach( InfoCard serialzable in m_infocards ) { serialzable.WriteXml( eltWriter ); } eltWriter.WriteEndElement( ); eltWriter.Flush(); } tempStream.Flush( ); tempStream.Seek( 0, SeekOrigin.Begin ); cipherValue = new byte[ RoamingStoreFileUtility.CalculateEncryptedSize( Convert.ToInt32( tempStream.Length ) ) ]; using( MemoryStream encryptedStream = new MemoryStream( cipherValue ) ) { RoamingStoreFileUtility.Encrypt( tempStream, encryptedStream, password, out newSalt ); encryptedStream.Flush( ); } } writer.WriteStartElement( XmlNames.WSIdentity.EncryptedStoreElement, XmlNames.WSIdentity.Namespace ); writer.WriteStartElement( XmlNames.WSIdentity.StoreSaltElement, XmlNames.WSIdentity.Namespace ); writer.WriteBase64( newSalt, 0, newSalt.Length ); writer.WriteEndElement( ); writer.WriteStartElement( XmlNames.XmlEnc.EncryptedData,XmlNames.XmlEnc.Namespace ); writer.WriteStartElement( XmlNames.XmlEnc.CipherData,XmlNames.XmlEnc.Namespace ); writer.WriteStartElement( XmlNames.XmlEnc.CipherValue,XmlNames.XmlEnc.Namespace ); writer.WriteBase64( cipherValue, 0, cipherValue.Length ); writer.WriteEndElement( ); writer.WriteEndElement( ); writer.WriteEndElement( ); writer.WriteEndElement( ); } // // Summary: // Reads and decrypts the xml stream using the specified password. // public void ReadFrom( string password, XmlReader reader ) { if( !reader.IsStartElement( XmlNames.WSIdentity.EncryptedStoreElement, XmlNames.WSIdentity.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } if( !reader.ReadToDescendant( XmlNames.WSIdentity.StoreSaltElement, XmlNames.WSIdentity.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } byte[] salt = new byte[ RoamingStoreFileUtility.SaltLength ]; reader.ReadElementContentAsBase64( salt, 0, salt.Length ); if( 0 != reader.ReadElementContentAsBase64( new byte[ 1 ], 0, 1 ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } if( !reader.IsStartElement( XmlNames.XmlEnc.EncryptedData,XmlNames.XmlEnc.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile )) ); } if( !reader.ReadToDescendant( XmlNames.XmlEnc.CipherData,XmlNames.XmlEnc.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile )) ); } if( !reader.ReadToDescendant( XmlNames.XmlEnc.CipherValue,XmlNames.XmlEnc.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } using( MemoryStream encrypted = new MemoryStream( 4096 ) ) { byte[] block = new byte[ 1024 ]; int bytesRead = 0; while( ( bytesRead = reader.ReadElementContentAsBase64( block, 0, block.Length ) ) > 0 ) { encrypted.Write( block, 0, bytesRead ); } encrypted.Flush( ); encrypted.Seek( 0, SeekOrigin.Begin ); using( MemoryStream decrypted = new MemoryStream( RoamingStoreFileUtility.CalculateDecryptedSize(Convert.ToInt32(encrypted.Length)) ) ) { RoamingStoreFileUtility.Decrypt( encrypted, decrypted, password, salt ); decrypted.Flush( ); decrypted.Seek( 0, SeekOrigin.Begin ); using( XmlReader decryptedReader = InfoCardSchemas.CreateReader( decrypted, reader.Settings ) ) { decryptedReader.Read( ); if( !decryptedReader.IsStartElement( XmlNames.WSIdentity.RoamingStoreElement, XmlNames.WSIdentity.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } if( decryptedReader.ReadToDescendant( XmlNames.WSIdentity.RoamingInfoCardElement, XmlNames.WSIdentity.Namespace ) ) { do { InfoCard card = new InfoCard(); card.ReadXml( decryptedReader ); m_infocards.Add(card); decryptedReader.ReadEndElement( ); } while( decryptedReader.IsStartElement( XmlNames.WSIdentity.RoamingInfoCardElement, XmlNames.WSIdentity.Namespace ) ); } decryptedReader.ReadEndElement( );//RoamingStore } } } reader.ReadEndElement( );//CipherData // // Skip optional encryption properties element. // if( reader.IsStartElement( XmlNames.XmlEnc.EncryptionProperties, XmlNames.XmlEnc.Namespace ) ) { reader.Skip(); } reader.ReadEndElement( );//EncryptedData reader.ReadEndElement( );//EncryptedStore } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
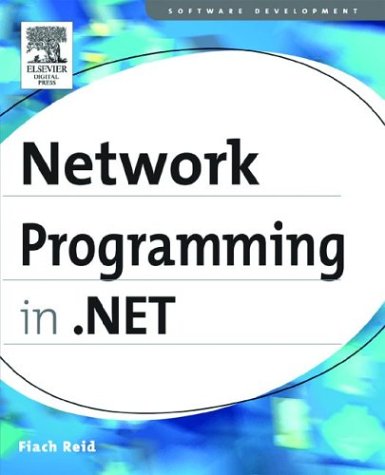
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventLogger.cs
- XmlNodeChangedEventArgs.cs
- AncestorChangedEventArgs.cs
- MultiPropertyDescriptorGridEntry.cs
- ListViewItemSelectionChangedEvent.cs
- SystemIPAddressInformation.cs
- JumpItem.cs
- DefaultTraceListener.cs
- Scalars.cs
- Section.cs
- LinqDataSourceView.cs
- ThreadLocal.cs
- RequestStatusBarUpdateEventArgs.cs
- UpDownBase.cs
- WebPartsPersonalization.cs
- WebPartEditVerb.cs
- CopyAction.cs
- PartialCachingAttribute.cs
- BaseInfoTable.cs
- IndexOutOfRangeException.cs
- ColorKeyFrameCollection.cs
- AppDomainAttributes.cs
- EventDescriptorCollection.cs
- PersistNameAttribute.cs
- AppDomainCompilerProxy.cs
- ParallelQuery.cs
- Configuration.cs
- CodeGenerator.cs
- SessionPageStatePersister.cs
- XMLSyntaxException.cs
- _Events.cs
- SocketConnection.cs
- HtmlAnchor.cs
- GuidelineSet.cs
- UnsafeNativeMethods.cs
- PngBitmapEncoder.cs
- TypedElement.cs
- HttpListenerResponse.cs
- mediaclock.cs
- UnsettableComboBox.cs
- MultipartContentParser.cs
- MouseActionConverter.cs
- SmtpLoginAuthenticationModule.cs
- ToolboxItem.cs
- XmlILAnnotation.cs
- ListControlConvertEventArgs.cs
- SchemaAttDef.cs
- ScriptControl.cs
- XmlTextWriter.cs
- XmlExceptionHelper.cs
- XmlImplementation.cs
- TextElementCollection.cs
- TrackingProfileCache.cs
- OptionalRstParameters.cs
- MemoryRecordBuffer.cs
- ObjectListComponentEditor.cs
- ObfuscateAssemblyAttribute.cs
- cryptoapiTransform.cs
- PageContentAsyncResult.cs
- DataPager.cs
- ByteAnimationUsingKeyFrames.cs
- BrushConverter.cs
- ClientProxyGenerator.cs
- DataGridViewAdvancedBorderStyle.cs
- FaultHandlingFilter.cs
- EntityClientCacheKey.cs
- BindMarkupExtensionSerializer.cs
- AdornerLayer.cs
- SignatureGenerator.cs
- DataPagerCommandEventArgs.cs
- XmlUTF8TextWriter.cs
- FixedSOMImage.cs
- RepeaterCommandEventArgs.cs
- UpdateException.cs
- RowUpdatedEventArgs.cs
- SchemaTypeEmitter.cs
- FontCacheUtil.cs
- Unit.cs
- TableLayoutColumnStyleCollection.cs
- VariableAction.cs
- SurrogateChar.cs
- ListBoxAutomationPeer.cs
- SecureUICommand.cs
- SafeNativeMethods.cs
- HostedElements.cs
- SettingsSavedEventArgs.cs
- MimePart.cs
- DataBindingExpressionBuilder.cs
- PackageRelationshipCollection.cs
- CompiledQuery.cs
- IFormattable.cs
- sqlinternaltransaction.cs
- StyleCollectionEditor.cs
- DrawingServices.cs
- EnumMember.cs
- MatrixTransform.cs
- PartialTrustVisibleAssembliesSection.cs
- BaseValidator.cs
- ClassicBorderDecorator.cs
- PolyLineSegment.cs