Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / BinaryMessageEncodingBindingElement.cs / 1 / BinaryMessageEncodingBindingElement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Reflection; using System.ServiceModel.Description; using System.Runtime.Serialization; using System.ServiceModel; using System.Xml; using System.Collections.Generic; public sealed class BinaryMessageEncodingBindingElement : MessageEncodingBindingElement, IWsdlExportExtension, IPolicyExportExtension { int maxReadPoolSize; int maxWritePoolSize; XmlDictionaryReaderQuotas readerQuotas; int maxSessionSize; BinaryVersion binaryVersion; MessageVersion messageVersion; public BinaryMessageEncodingBindingElement() { this.maxReadPoolSize = EncoderDefaults.MaxReadPoolSize; this.maxWritePoolSize = EncoderDefaults.MaxWritePoolSize; this.readerQuotas = new XmlDictionaryReaderQuotas(); EncoderDefaults.ReaderQuotas.CopyTo(this.readerQuotas); this.maxSessionSize = BinaryEncoderDefaults.MaxSessionSize; this.binaryVersion = BinaryEncoderDefaults.BinaryVersion; this.messageVersion = MessageVersion.CreateVersion(BinaryEncoderDefaults.EnvelopeVersion); } BinaryMessageEncodingBindingElement(BinaryMessageEncodingBindingElement elementToBeCloned) : base(elementToBeCloned) { this.maxReadPoolSize = elementToBeCloned.maxReadPoolSize; this.maxWritePoolSize = elementToBeCloned.maxWritePoolSize; this.readerQuotas = new XmlDictionaryReaderQuotas(); elementToBeCloned.readerQuotas.CopyTo(this.readerQuotas); this.MaxSessionSize = elementToBeCloned.MaxSessionSize; this.BinaryVersion = elementToBeCloned.BinaryVersion; this.messageVersion = elementToBeCloned.messageVersion; } /* public */ BinaryVersion BinaryVersion { get { return binaryVersion; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); } this.binaryVersion = value; } } public override MessageVersion MessageVersion { get { return this.messageVersion; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } if (value.Envelope != BinaryEncoderDefaults.EnvelopeVersion) { string errorMsg = SR.GetString(SR.UnsupportedEnvelopeVersion, this.GetType().FullName, BinaryEncoderDefaults.EnvelopeVersion, value.Envelope); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(errorMsg)); } this.messageVersion = MessageVersion.CreateVersion(BinaryEncoderDefaults.EnvelopeVersion, value.Addressing); } } public int MaxReadPoolSize { get { return this.maxReadPoolSize; } set { if (value <= 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", value, SR.GetString(SR.ValueMustBePositive))); } this.maxReadPoolSize = value; } } public int MaxWritePoolSize { get { return this.maxWritePoolSize; } set { if (value <= 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", value, SR.GetString(SR.ValueMustBePositive))); } this.maxWritePoolSize = value; } } public XmlDictionaryReaderQuotas ReaderQuotas { get { return this.readerQuotas; } } public int MaxSessionSize { get { return this.maxSessionSize; } set { if (value < 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", value, SR.GetString(SR.ValueMustBeNonNegative))); } this.maxSessionSize = value; } } public override IChannelFactoryBuildChannelFactory (BindingContext context) { return InternalBuildChannelFactory (context); } public override IChannelListener BuildChannelListener (BindingContext context) { return InternalBuildChannelListener (context); } public override bool CanBuildChannelListener (BindingContext context) { return InternalCanBuildChannelListener (context); } public override BindingElement Clone() { return new BinaryMessageEncodingBindingElement(this); } public override MessageEncoderFactory CreateMessageEncoderFactory() { return new BinaryMessageEncoderFactory(this.MessageVersion, this.MaxReadPoolSize, this.MaxWritePoolSize, this.MaxSessionSize, this.ReaderQuotas, this.BinaryVersion); } public override T GetProperty (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (typeof(T) == typeof(XmlDictionaryReaderQuotas)) { return (T)(object)this.readerQuotas; } else { return base.GetProperty (context); } } void IPolicyExportExtension.ExportPolicy(MetadataExporter exporter, PolicyConversionContext policyContext) { if (policyContext == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("policyContext"); } XmlDocument document = new XmlDocument(); policyContext.GetBindingAssertions().Add(document.CreateElement( MessageEncodingPolicyConstants.BinaryEncodingPrefix, MessageEncodingPolicyConstants.BinaryEncodingName, MessageEncodingPolicyConstants.BinaryEncodingNamespace)); } void IWsdlExportExtension.ExportContract(WsdlExporter exporter, WsdlContractConversionContext context) { } void IWsdlExportExtension.ExportEndpoint(WsdlExporter exporter, WsdlEndpointConversionContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } SoapHelper.SetSoapVersion(context, exporter, MessageVersion.Soap12WSAddressing10.Envelope); } internal override bool IsMatch(BindingElement b) { if (!base.IsMatch(b)) return false; BinaryMessageEncodingBindingElement binary = b as BinaryMessageEncodingBindingElement; if (binary == null) return false; if (this.maxReadPoolSize != binary.MaxReadPoolSize) return false; if (this.maxWritePoolSize != binary.MaxWritePoolSize) return false; // compare XmlDictionaryReaderQuotas if (this.readerQuotas.MaxStringContentLength != binary.ReaderQuotas.MaxStringContentLength) return false; if (this.readerQuotas.MaxArrayLength != binary.ReaderQuotas.MaxArrayLength) return false; if (this.readerQuotas.MaxBytesPerRead != binary.ReaderQuotas.MaxBytesPerRead) return false; if (this.readerQuotas.MaxDepth != binary.ReaderQuotas.MaxDepth) return false; if (this.readerQuotas.MaxNameTableCharCount != binary.ReaderQuotas.MaxNameTableCharCount) return false; if (this.MaxSessionSize != binary.MaxSessionSize) return false; return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
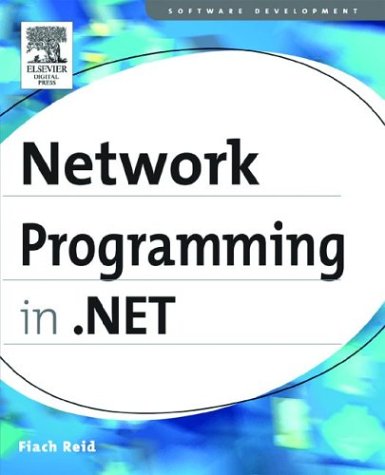
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FamilyTypeface.cs
- SearchForVirtualItemEventArgs.cs
- WebSysDefaultValueAttribute.cs
- ToolStripItemClickedEventArgs.cs
- UnsafeNativeMethods.cs
- ContractHandle.cs
- RecognizerInfo.cs
- HwndSubclass.cs
- GatewayIPAddressInformationCollection.cs
- IdentityReference.cs
- AnnotationHelper.cs
- PerformanceCounterLib.cs
- _FtpDataStream.cs
- ConfigXmlCDataSection.cs
- TextEditor.cs
- InstanceNotReadyException.cs
- ComponentEditorForm.cs
- LongCountAggregationOperator.cs
- ClientUrlResolverWrapper.cs
- ContentWrapperAttribute.cs
- SourceElementsCollection.cs
- CharConverter.cs
- Font.cs
- Visual3DCollection.cs
- FilteredReadOnlyMetadataCollection.cs
- UriScheme.cs
- Item.cs
- TypeDescriptionProvider.cs
- PtsHelper.cs
- EventLogConfiguration.cs
- CapabilitiesSection.cs
- IdentityHolder.cs
- CellCreator.cs
- MasterPageParser.cs
- ProcessRequestArgs.cs
- EntityContainer.cs
- DetailsViewInsertEventArgs.cs
- HtmlGenericControl.cs
- BatchWriter.cs
- MimeXmlImporter.cs
- DbConnectionOptions.cs
- GridViewRowEventArgs.cs
- QueryParameter.cs
- HttpListenerResponse.cs
- WebBaseEventKeyComparer.cs
- CompressionTransform.cs
- SkipStoryboardToFill.cs
- KeyEventArgs.cs
- XmlCDATASection.cs
- ApplicationServiceHelper.cs
- DataGridTemplateColumn.cs
- InterleavedZipPartStream.cs
- DurationConverter.cs
- DockPanel.cs
- MarshalByValueComponent.cs
- ActiveDesignSurfaceEvent.cs
- GeometryModel3D.cs
- XPathNodeList.cs
- EmptyStringExpandableObjectConverter.cs
- ElementProxy.cs
- RSAPKCS1SignatureFormatter.cs
- nulltextnavigator.cs
- XmlSchemaObjectCollection.cs
- PlanCompilerUtil.cs
- ParseChildrenAsPropertiesAttribute.cs
- ArrangedElementCollection.cs
- SqlDataReaderSmi.cs
- ResXFileRef.cs
- LabelDesigner.cs
- VirtualizingPanel.cs
- XmlBufferReader.cs
- DecimalFormatter.cs
- XmlSchemaDatatype.cs
- XPathNavigatorException.cs
- ParameterModifier.cs
- DurableOperationContext.cs
- HwndHostAutomationPeer.cs
- ToolStripProgressBar.cs
- ParseChildrenAsPropertiesAttribute.cs
- CookielessHelper.cs
- DataControlLinkButton.cs
- CustomAttribute.cs
- SamlAssertionKeyIdentifierClause.cs
- ServiceHostingEnvironment.cs
- DBPropSet.cs
- WindowsAuthenticationModule.cs
- ConnectionManagementSection.cs
- NonBatchDirectoryCompiler.cs
- DataSourceProvider.cs
- CompilationLock.cs
- Point3DCollectionConverter.cs
- TypedServiceChannelBuilder.cs
- ResourceKey.cs
- COMException.cs
- FixedSOMFixedBlock.cs
- XmlSchemaAttributeGroup.cs
- ListViewInsertionMark.cs
- MenuEventArgs.cs
- NamespaceEmitter.cs
- TheQuery.cs