Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / FilteredReadOnlyMetadataCollection.cs / 2 / FilteredReadOnlyMetadataCollection.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Diagnostics; using System.Reflection; using System.Text; namespace System.Data.Metadata.Edm { internal interface IBaseList: IList { T this[string identity] { get;} new T this[int index] { get;} int IndexOf(T item); } #pragma warning disable 1711 // compiler bug: reports TDerived and TBase as type parameters for non-existing IsReadOnly property /// /// Class to filter stuff out from a metadata collection /// /* */ internal class FilteredReadOnlyMetadataCollection: ReadOnlyMetadataCollection , IBaseList where TDerived : TBase where TBase : MetadataItem { #region Constructors /// /// The constructor for constructing a read-only metadata collection to wrap another MetadataCollection. /// /// The metadata collection to wrap ///Thrown if collection argument is null /// Predicate method which determines membership internal FilteredReadOnlyMetadataCollection(ReadOnlyMetadataCollectioncollection, Predicate predicate) : base(FilterCollection(collection, predicate)) { Debug.Assert(collection != null); Debug.Assert(collection.IsReadOnly, "wrappers should only be created once loading is over, and this collection is still loading"); _source = collection; _predicate = predicate; } #endregion #region Private Fields // The original metadata collection over which this filtered collection is the view private readonly ReadOnlyMetadataCollection _source; private readonly Predicate _predicate; #endregion #region Properties /// /// Gets an item from the collection with the given identity /// /// The identity of the item to search for ///An item from the collection ///Thrown if identity argument passed in is null ///Thrown if setter is called public override TDerived this[string identity] { get { TBase item = _source[identity]; if (_predicate(item)) { return (TDerived)item; } throw EntityUtil.ItemInvalidIdentity(identity, "identity"); } } #endregion #region Methods ////// Gets an item from the collection with the given identity /// /// The identity of the item to search for /// Whether case is ignore in the search ///An item from the collection ///Thrown if identity argument passed in is null ///Thrown if the Collection does not have an item with the given identity public override TDerived GetValue(string identity, bool ignoreCase) { TBase item = _source.GetValue(identity, ignoreCase); if (_predicate(item)) { return (TDerived)item; } throw EntityUtil.ItemInvalidIdentity(identity, "identity"); } ////// Determines if this collection contains an item of the given identity /// /// The identity of the item to check for ///True if the collection contains the item with the given identity ///Thrown if identity argument passed in is null ///Thrown if identity argument passed in is empty string public override bool Contains(string identity) { TBase item; if (_source.TryGetValue(identity, false/*ignoreCase*/, out item)) { return (_predicate(item)); } return false; } ////// Gets an item from the collection with the given identity /// /// The identity of the item to search for /// Whether case is ignore in the search /// An item from the collection, null if the item is not found ///True an item is retrieved ///if identity argument is null public override bool TryGetValue(string identity, bool ignoreCase, out TDerived item) { item = null; TBase baseTypeItem; if (_source.TryGetValue(identity, ignoreCase, out baseTypeItem)) { if (_predicate(baseTypeItem)) { item = (TDerived)baseTypeItem; return true; } } return false; } internal static ListFilterCollection(ReadOnlyMetadataCollection collection, Predicate predicate) { List list = new List (collection.Count); foreach (TBase item in collection) { if (predicate(item)) { list.Add((TDerived)item); } } return list; } /// /// Get index of the element passed as the argument /// /// ///public override int IndexOf(TDerived value) { TBase item; if (_source.TryGetValue(value.Identity, false /*ignoreCase*/, out item)) { if (_predicate(item)) { // Since we are gauranteed to have a unique identity per collection, this item must of T Type return base.IndexOf((TDerived)item); } } return -1; } #endregion #region IBaseList Members TBase IBaseList .this[string identity] { get { return this[identity]; } } TBase IBaseList .this[int index] { get { return this[index]; } } /// /// Get index of the element passed as the argument /// /// ///int IBaseList .IndexOf(TBase item) { if (_predicate(item)) { return this.IndexOf((TDerived)item); } return -1; } #endregion } #pragma warning restore 1711 } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Diagnostics; using System.Reflection; using System.Text; namespace System.Data.Metadata.Edm { internal interface IBaseList: IList { T this[string identity] { get;} new T this[int index] { get;} int IndexOf(T item); } #pragma warning disable 1711 // compiler bug: reports TDerived and TBase as type parameters for non-existing IsReadOnly property /// /// Class to filter stuff out from a metadata collection /// /* */ internal class FilteredReadOnlyMetadataCollection: ReadOnlyMetadataCollection , IBaseList where TDerived : TBase where TBase : MetadataItem { #region Constructors /// /// The constructor for constructing a read-only metadata collection to wrap another MetadataCollection. /// /// The metadata collection to wrap ///Thrown if collection argument is null /// Predicate method which determines membership internal FilteredReadOnlyMetadataCollection(ReadOnlyMetadataCollectioncollection, Predicate predicate) : base(FilterCollection(collection, predicate)) { Debug.Assert(collection != null); Debug.Assert(collection.IsReadOnly, "wrappers should only be created once loading is over, and this collection is still loading"); _source = collection; _predicate = predicate; } #endregion #region Private Fields // The original metadata collection over which this filtered collection is the view private readonly ReadOnlyMetadataCollection _source; private readonly Predicate _predicate; #endregion #region Properties /// /// Gets an item from the collection with the given identity /// /// The identity of the item to search for ///An item from the collection ///Thrown if identity argument passed in is null ///Thrown if setter is called public override TDerived this[string identity] { get { TBase item = _source[identity]; if (_predicate(item)) { return (TDerived)item; } throw EntityUtil.ItemInvalidIdentity(identity, "identity"); } } #endregion #region Methods ////// Gets an item from the collection with the given identity /// /// The identity of the item to search for /// Whether case is ignore in the search ///An item from the collection ///Thrown if identity argument passed in is null ///Thrown if the Collection does not have an item with the given identity public override TDerived GetValue(string identity, bool ignoreCase) { TBase item = _source.GetValue(identity, ignoreCase); if (_predicate(item)) { return (TDerived)item; } throw EntityUtil.ItemInvalidIdentity(identity, "identity"); } ////// Determines if this collection contains an item of the given identity /// /// The identity of the item to check for ///True if the collection contains the item with the given identity ///Thrown if identity argument passed in is null ///Thrown if identity argument passed in is empty string public override bool Contains(string identity) { TBase item; if (_source.TryGetValue(identity, false/*ignoreCase*/, out item)) { return (_predicate(item)); } return false; } ////// Gets an item from the collection with the given identity /// /// The identity of the item to search for /// Whether case is ignore in the search /// An item from the collection, null if the item is not found ///True an item is retrieved ///if identity argument is null public override bool TryGetValue(string identity, bool ignoreCase, out TDerived item) { item = null; TBase baseTypeItem; if (_source.TryGetValue(identity, ignoreCase, out baseTypeItem)) { if (_predicate(baseTypeItem)) { item = (TDerived)baseTypeItem; return true; } } return false; } internal static ListFilterCollection(ReadOnlyMetadataCollection collection, Predicate predicate) { List list = new List (collection.Count); foreach (TBase item in collection) { if (predicate(item)) { list.Add((TDerived)item); } } return list; } /// /// Get index of the element passed as the argument /// /// ///public override int IndexOf(TDerived value) { TBase item; if (_source.TryGetValue(value.Identity, false /*ignoreCase*/, out item)) { if (_predicate(item)) { // Since we are gauranteed to have a unique identity per collection, this item must of T Type return base.IndexOf((TDerived)item); } } return -1; } #endregion #region IBaseList Members TBase IBaseList .this[string identity] { get { return this[identity]; } } TBase IBaseList .this[int index] { get { return this[index]; } } /// /// Get index of the element passed as the argument /// /// ///int IBaseList .IndexOf(TBase item) { if (_predicate(item)) { return this.IndexOf((TDerived)item); } return -1; } #endregion } #pragma warning restore 1711 } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
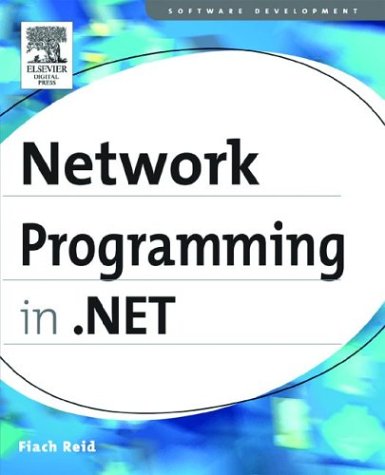
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DSASignatureFormatter.cs
- AnnotationAdorner.cs
- TextTreeDeleteContentUndoUnit.cs
- ComponentSerializationService.cs
- XPathPatternBuilder.cs
- TextDecorations.cs
- PageAction.cs
- Stroke2.cs
- XmlChoiceIdentifierAttribute.cs
- COM2Properties.cs
- PropertyPushdownHelper.cs
- BitmapEffectState.cs
- AnchoredBlock.cs
- Exceptions.cs
- ToolStripItemCollection.cs
- UIntPtr.cs
- BufferedStream.cs
- Style.cs
- DiffuseMaterial.cs
- MenuItemCollection.cs
- PackageFilter.cs
- DataBoundControlAdapter.cs
- StructuredTypeEmitter.cs
- InputLanguageCollection.cs
- EmbeddedMailObjectsCollection.cs
- RegisteredDisposeScript.cs
- ConstructorArgumentAttribute.cs
- BindValidator.cs
- NativeMethods.cs
- BamlResourceDeserializer.cs
- FormattedText.cs
- Version.cs
- WebDescriptionAttribute.cs
- ConfigurationStrings.cs
- HandleCollector.cs
- ActivationServices.cs
- EdmValidator.cs
- SigningCredentials.cs
- SecurityDocument.cs
- TableLayoutPanelDesigner.cs
- ColorPalette.cs
- XslVisitor.cs
- ConfigurationElement.cs
- FormClosedEvent.cs
- SetStoryboardSpeedRatio.cs
- __ConsoleStream.cs
- TimeoutConverter.cs
- ObjectToIdCache.cs
- ListenerHandler.cs
- WebBrowsableAttribute.cs
- Attributes.cs
- TemplateBindingExtensionConverter.cs
- ExternalCalls.cs
- MemoryRecordBuffer.cs
- HttpException.cs
- WSAddressing10ProblemHeaderQNameFault.cs
- XmlSchemaIdentityConstraint.cs
- PartManifestEntry.cs
- PkcsUtils.cs
- BlockUIContainer.cs
- SqlInfoMessageEvent.cs
- DomainConstraint.cs
- NavigationWindowAutomationPeer.cs
- BaseTemplateCodeDomTreeGenerator.cs
- ScriptControl.cs
- ServiceAuthorizationManager.cs
- XmlSchemaProviderAttribute.cs
- ObjectAnimationUsingKeyFrames.cs
- OrderPreservingMergeHelper.cs
- SelectionUIHandler.cs
- ContextMenuAutomationPeer.cs
- WebExceptionStatus.cs
- XmlCodeExporter.cs
- documentsequencetextpointer.cs
- CodeTypeReference.cs
- XmlSchemaElement.cs
- ComEventsMethod.cs
- FastEncoder.cs
- DataColumnChangeEvent.cs
- Predicate.cs
- InputManager.cs
- AtomServiceDocumentSerializer.cs
- RefreshInfo.cs
- NetworkInformationPermission.cs
- ModelTreeManager.cs
- MarkupObject.cs
- TextBox.cs
- TextInfo.cs
- TextElementEnumerator.cs
- ReflectionTypeLoadException.cs
- NativeMethodsCLR.cs
- CodeTypeParameterCollection.cs
- Splitter.cs
- TaiwanLunisolarCalendar.cs
- CheckBoxField.cs
- FrameworkTextComposition.cs
- DataTrigger.cs
- StringDictionary.cs
- MultiView.cs
- WpfWebRequestHelper.cs