Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Activation / SharedMemory.cs / 1 / SharedMemory.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security.Principal; using System.ServiceModel.Channels; using System.Threading; using System.ServiceModel; using System.ComponentModel; unsafe class SharedMemory : IDisposable { SafeFileMappingHandle fileMapping; SharedMemory(SafeFileMappingHandle fileMapping) { this.fileMapping = fileMapping; } public static unsafe SharedMemory Create(string name, Guid content, ListallowedSids) { int errorCode = UnsafeNativeMethods.ERROR_SUCCESS; byte[] binarySecurityDescriptor = SecurityDescriptorHelper.FromSecurityIdentifiers(allowedSids, UnsafeNativeMethods.GENERIC_READ); SafeFileMappingHandle fileMapping; UnsafeNativeMethods.SECURITY_ATTRIBUTES securityAttributes = new UnsafeNativeMethods.SECURITY_ATTRIBUTES(); fixed (byte* pinnedSecurityDescriptor = binarySecurityDescriptor) { securityAttributes.lpSecurityDescriptor = (IntPtr)pinnedSecurityDescriptor; fileMapping = UnsafeNativeMethods.CreateFileMapping((IntPtr)(-1), securityAttributes, UnsafeNativeMethods.PAGE_READWRITE, 0, sizeof(SharedMemoryContents), name); errorCode = Marshal.GetLastWin32Error(); } if (fileMapping.IsInvalid) { fileMapping.SetHandleAsInvalid(); fileMapping.Close(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(errorCode)); } SharedMemory sharedMemory = new SharedMemory(fileMapping); SafeViewOfFileHandle view; // Ignore return value. GetView(fileMapping, true, out view); try { SharedMemoryContents* contents = (SharedMemoryContents*)view.DangerousGetHandle(); contents->pipeGuid = content; Thread.MemoryBarrier(); contents->isInitialized = true; return sharedMemory; } finally { view.Close(); } } public void Dispose() { if (fileMapping != null) { fileMapping.Close(); fileMapping = null; } } static bool GetView(SafeFileMappingHandle fileMapping, bool writable, out SafeViewOfFileHandle handle) { handle = UnsafeNativeMethods.MapViewOfFile(fileMapping, writable ? UnsafeNativeMethods.FILE_MAP_WRITE : UnsafeNativeMethods.FILE_MAP_READ, 0, 0, (IntPtr)sizeof(SharedMemoryContents)); int errorCode = Marshal.GetLastWin32Error(); if (!handle.IsInvalid) { return true; } else { handle.SetHandleAsInvalid(); fileMapping.Close(); // Only return false when it's reading time. if (!writable && errorCode == UnsafeNativeMethods.ERROR_FILE_NOT_FOUND) { return false; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(errorCode)); } } public static string Read(string name) { string content; if (Read(name, out content)) { return content; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(UnsafeNativeMethods.ERROR_FILE_NOT_FOUND)); } public static bool Read(string name, out string content) { content = null; SafeFileMappingHandle fileMapping = UnsafeNativeMethods.OpenFileMapping(UnsafeNativeMethods.FILE_MAP_READ, false, ListenerConstants.GlobalPrefix + name); int errorCode = Marshal.GetLastWin32Error(); if (fileMapping.IsInvalid) { fileMapping.SetHandleAsInvalid(); fileMapping.Close(); if (errorCode == UnsafeNativeMethods.ERROR_FILE_NOT_FOUND) { return false; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(errorCode)); } try { SafeViewOfFileHandle view; if (!GetView(fileMapping, false, out view)) { return false; } try { SharedMemoryContents* contents = (SharedMemoryContents*)view.DangerousGetHandle(); content = contents->isInitialized ? contents->pipeGuid.ToString() : null; return true; } finally { view.Close(); } } finally { fileMapping.Close(); } } [StructLayout(LayoutKind.Sequential)] struct SharedMemoryContents { public bool isInitialized; public Guid pipeGuid; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
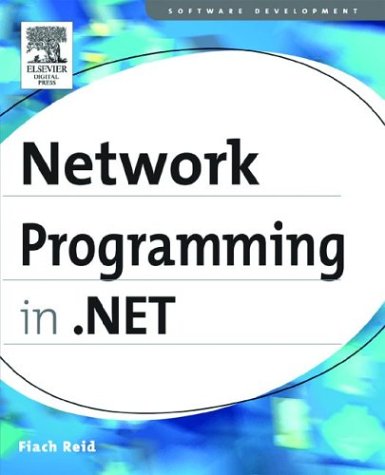
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageRequestManager.cs
- WebEncodingValidator.cs
- Query.cs
- ExtensibleClassFactory.cs
- BrowserDefinitionCollection.cs
- HttpRequest.cs
- View.cs
- DiscoveryEndpointValidator.cs
- MLangCodePageEncoding.cs
- Helper.cs
- GridViewUpdateEventArgs.cs
- QuaternionRotation3D.cs
- Vector3DAnimationUsingKeyFrames.cs
- TransactionInformation.cs
- EditorZoneAutoFormat.cs
- FrameSecurityDescriptor.cs
- TrackingRecordPreFilter.cs
- TaskHelper.cs
- ParseNumbers.cs
- IndicCharClassifier.cs
- BuildProvider.cs
- Certificate.cs
- EmptyQuery.cs
- ACE.cs
- WebPartConnectionsConfigureVerb.cs
- XmlQueryTypeFactory.cs
- EdmProperty.cs
- SqlProfileProvider.cs
- UnsafeNativeMethods.cs
- OleDbCommand.cs
- DeclarativeCatalogPart.cs
- ObjectParameter.cs
- ConnectionsZone.cs
- CLRBindingWorker.cs
- JsonReaderDelegator.cs
- ClientOptions.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- recordstatefactory.cs
- Authorization.cs
- DeliveryRequirementsAttribute.cs
- X509Utils.cs
- TextView.cs
- DeferrableContent.cs
- PaginationProgressEventArgs.cs
- AsyncStreamReader.cs
- ConnectionPoolManager.cs
- LinearKeyFrames.cs
- WorkflowElementDialog.cs
- HttpContext.cs
- PropertyMappingExceptionEventArgs.cs
- PinnedBufferMemoryStream.cs
- HtmlInputHidden.cs
- HttpHandlerAction.cs
- Signature.cs
- EntityCollectionChangedParams.cs
- BamlResourceContent.cs
- HtmlEmptyTagControlBuilder.cs
- FlowDocumentPaginator.cs
- PKCS1MaskGenerationMethod.cs
- AttributeData.cs
- DrawingVisual.cs
- SignHashRequest.cs
- CollectionConverter.cs
- Int32Animation.cs
- JavascriptCallbackBehaviorAttribute.cs
- CompilerLocalReference.cs
- CodeDirectionExpression.cs
- SessionStateModule.cs
- OletxEnlistment.cs
- DataColumn.cs
- JournalEntry.cs
- GcHandle.cs
- CellLabel.cs
- DataGridHelper.cs
- TogglePatternIdentifiers.cs
- XmlName.cs
- ImageListImageEditor.cs
- XmlILTrace.cs
- PointF.cs
- AuthenticationModulesSection.cs
- EmptyImpersonationContext.cs
- DataGridViewRowEventArgs.cs
- AddInController.cs
- CodeArgumentReferenceExpression.cs
- StylusDevice.cs
- ChannelManager.cs
- CodeTypeMember.cs
- TargetControlTypeCache.cs
- UpdatePanelTriggerCollection.cs
- ExtensionFile.cs
- DbConnectionPoolGroup.cs
- AspNetCacheProfileAttribute.cs
- SwitchAttribute.cs
- ResourceKey.cs
- BaseUriHelper.cs
- RuntimeEnvironment.cs
- AttributeXamlType.cs
- SystemUnicastIPAddressInformation.cs
- ListViewDeletedEventArgs.cs
- SmiConnection.cs