Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ScrollProperties.cs / 1 / ScrollProperties.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Security.Permissions; using System.Runtime.Serialization.Formatters; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; using System.Windows.Forms; using System.Globalization; ////// /// public abstract class ScrollProperties { internal int minimum = 0; internal int maximum = 100; internal int smallChange = 1; internal int largeChange = 10; internal int value = 0; internal bool maximumSetExternally; internal bool smallChangeSetExternally; internal bool largeChangeSetExternally; ////// Basic Properties for Scrollbars. /// ///private ScrollableControl parent; protected ScrollableControl ParentControl { get { return parent; } } /// /// Number of pixels to scroll the client region as a "line" for autoscroll. /// ///private const int SCROLL_LINE = 5; internal bool visible = false; //Always Enabled private bool enabled = true; /// protected ScrollProperties(ScrollableControl container) { this.parent = container; } /// /// /// [ SRCategory(SR.CatBehavior), DefaultValue(true), SRDescription(SR.ScrollBarEnableDescr) ] public bool Enabled { get { return enabled; } set { if (parent.AutoScroll) { return; } if (value != enabled) { enabled = value; EnableScroll(value); } } } ////// Gets or sets a bool value controlling whether the scrollbar is enabled. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(10), SRDescription(SR.ScrollBarLargeChangeDescr), RefreshProperties(RefreshProperties.Repaint) ] public int LargeChange { get { // We preserve the actual large change value that has been set, but when we come to // get the value of this property, make sure it's within the maximum allowable value. // This way we ensure that we don't depend on the order of property sets when // code is generated at design-time. // return Math.Min(largeChange, maximum - minimum + 1); } set { if (largeChange != value ) { if (value < 0) { throw new ArgumentOutOfRangeException("LargeChange", SR.GetString(SR.InvalidLowBoundArgumentEx, "LargeChange", (value).ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } largeChange = value; largeChangeSetExternally = true; UpdateScrollInfo(); } } } ////// Gets or sets a value to be added or subtracted to the ////// property when the scroll box is moved a large distance. /// /// /// [ SRCategory(SR.CatBehavior), DefaultValue(100), SRDescription(SR.ScrollBarMaximumDescr), RefreshProperties(RefreshProperties.Repaint) ] public int Maximum { get { return maximum; } set { if (parent.AutoScroll) { return; } if (maximum != value) { if (minimum > value) minimum = value; // bring this.value in line. if (value < this.value) Value = value; maximum = value; maximumSetExternally = true; UpdateScrollInfo(); } } } ////// Gets or sets the upper limit of values of the scrollable range. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(0), SRDescription(SR.ScrollBarMinimumDescr), RefreshProperties(RefreshProperties.Repaint) ] public int Minimum { get { return minimum; } set { if (parent.AutoScroll) { return; } if (minimum != value) { if (value < 0) { throw new ArgumentOutOfRangeException("Minimum", SR.GetString(SR.InvalidLowBoundArgumentEx, "Minimum", (value).ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } if (maximum < value) maximum = value; // bring this.value in line. if (value > this.value) this.value = value; minimum = value; UpdateScrollInfo(); } } } internal abstract int PageSize { get; } internal abstract int Orientation { get; } internal abstract int HorizontalDisplayPosition { get; } internal abstract int VerticalDisplayPosition { get; } ////// Gets or sets the lower limit of values of the scrollable range. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(1), SRDescription(SR.ScrollBarSmallChangeDescr) ] public int SmallChange { get { // We can't have SmallChange > LargeChange, but we shouldn't manipulate // the set values for these properties, so we just return the smaller // value here. // return Math.Min(smallChange, LargeChange); } set { if (smallChange != value) { if (value < 0) { throw new ArgumentOutOfRangeException("SmallChange", SR.GetString(SR.InvalidLowBoundArgumentEx, "SmallChange", (value).ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } smallChange = value; smallChangeSetExternally = true; UpdateScrollInfo(); } } } ////// Gets or sets the value to be added or subtracted to the /// ////// property when the scroll box is /// moved a small distance. /// /// /// [ SRCategory(SR.CatBehavior), DefaultValue(0), Bindable(true), SRDescription(SR.ScrollBarValueDescr) ] public int Value { get { return value; } set { if (this.value != value) { if (value < minimum || value > maximum) { throw new ArgumentOutOfRangeException("Value", SR.GetString(SR.InvalidBoundArgument, "Value", (value).ToString(CultureInfo.CurrentCulture), "'minimum'", "'maximum'")); } this.value = value; UpdateScrollInfo(); parent.SetDisplayFromScrollProps(HorizontalDisplayPosition, VerticalDisplayPosition); } } } ////// Gets or sets a numeric value that represents the current /// position of the scroll box /// on /// the scroll bar control. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.ScrollBarVisibleDescr) ] public bool Visible { get { return visible; } set { if (parent.AutoScroll) { return; } if (value != visible) { visible = value; parent.UpdateStylesCore (); UpdateScrollInfo(); parent.SetDisplayFromScrollProps(HorizontalDisplayPosition, VerticalDisplayPosition); } } } ////// Gets or sets a bool value controlling whether the scrollbar is showing. /// ////// /// Internal helper method /// ///internal void UpdateScrollInfo() { if (parent.IsHandleCreated && visible) { NativeMethods.SCROLLINFO si = new NativeMethods.SCROLLINFO(); si.cbSize = Marshal.SizeOf(typeof(NativeMethods.SCROLLINFO)); si.fMask = NativeMethods.SIF_ALL; si.nMin = minimum; si.nMax = maximum; si.nPage = (parent.AutoScroll) ? PageSize : LargeChange; si.nPos = value; si.nTrackPos = 0; UnsafeNativeMethods.SetScrollInfo(new HandleRef(parent, parent.Handle), Orientation, si, true); } } /// /// /// Internal helper method for enabling or disabling the Vertical Scroll bar. /// ///private void EnableScroll(bool enable) { if (enable) { UnsafeNativeMethods.EnableScrollBar(new HandleRef(parent, parent.Handle), Orientation, NativeMethods.ESB_ENABLE_BOTH); } else { UnsafeNativeMethods.EnableScrollBar(new HandleRef(parent, parent.Handle), Orientation, NativeMethods.ESB_DISABLE_BOTH); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Security.Permissions; using System.Runtime.Serialization.Formatters; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; using System.Windows.Forms; using System.Globalization; ////// /// public abstract class ScrollProperties { internal int minimum = 0; internal int maximum = 100; internal int smallChange = 1; internal int largeChange = 10; internal int value = 0; internal bool maximumSetExternally; internal bool smallChangeSetExternally; internal bool largeChangeSetExternally; ////// Basic Properties for Scrollbars. /// ///private ScrollableControl parent; protected ScrollableControl ParentControl { get { return parent; } } /// /// Number of pixels to scroll the client region as a "line" for autoscroll. /// ///private const int SCROLL_LINE = 5; internal bool visible = false; //Always Enabled private bool enabled = true; /// protected ScrollProperties(ScrollableControl container) { this.parent = container; } /// /// /// [ SRCategory(SR.CatBehavior), DefaultValue(true), SRDescription(SR.ScrollBarEnableDescr) ] public bool Enabled { get { return enabled; } set { if (parent.AutoScroll) { return; } if (value != enabled) { enabled = value; EnableScroll(value); } } } ////// Gets or sets a bool value controlling whether the scrollbar is enabled. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(10), SRDescription(SR.ScrollBarLargeChangeDescr), RefreshProperties(RefreshProperties.Repaint) ] public int LargeChange { get { // We preserve the actual large change value that has been set, but when we come to // get the value of this property, make sure it's within the maximum allowable value. // This way we ensure that we don't depend on the order of property sets when // code is generated at design-time. // return Math.Min(largeChange, maximum - minimum + 1); } set { if (largeChange != value ) { if (value < 0) { throw new ArgumentOutOfRangeException("LargeChange", SR.GetString(SR.InvalidLowBoundArgumentEx, "LargeChange", (value).ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } largeChange = value; largeChangeSetExternally = true; UpdateScrollInfo(); } } } ////// Gets or sets a value to be added or subtracted to the ////// property when the scroll box is moved a large distance. /// /// /// [ SRCategory(SR.CatBehavior), DefaultValue(100), SRDescription(SR.ScrollBarMaximumDescr), RefreshProperties(RefreshProperties.Repaint) ] public int Maximum { get { return maximum; } set { if (parent.AutoScroll) { return; } if (maximum != value) { if (minimum > value) minimum = value; // bring this.value in line. if (value < this.value) Value = value; maximum = value; maximumSetExternally = true; UpdateScrollInfo(); } } } ////// Gets or sets the upper limit of values of the scrollable range. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(0), SRDescription(SR.ScrollBarMinimumDescr), RefreshProperties(RefreshProperties.Repaint) ] public int Minimum { get { return minimum; } set { if (parent.AutoScroll) { return; } if (minimum != value) { if (value < 0) { throw new ArgumentOutOfRangeException("Minimum", SR.GetString(SR.InvalidLowBoundArgumentEx, "Minimum", (value).ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } if (maximum < value) maximum = value; // bring this.value in line. if (value > this.value) this.value = value; minimum = value; UpdateScrollInfo(); } } } internal abstract int PageSize { get; } internal abstract int Orientation { get; } internal abstract int HorizontalDisplayPosition { get; } internal abstract int VerticalDisplayPosition { get; } ////// Gets or sets the lower limit of values of the scrollable range. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(1), SRDescription(SR.ScrollBarSmallChangeDescr) ] public int SmallChange { get { // We can't have SmallChange > LargeChange, but we shouldn't manipulate // the set values for these properties, so we just return the smaller // value here. // return Math.Min(smallChange, LargeChange); } set { if (smallChange != value) { if (value < 0) { throw new ArgumentOutOfRangeException("SmallChange", SR.GetString(SR.InvalidLowBoundArgumentEx, "SmallChange", (value).ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } smallChange = value; smallChangeSetExternally = true; UpdateScrollInfo(); } } } ////// Gets or sets the value to be added or subtracted to the /// ////// property when the scroll box is /// moved a small distance. /// /// /// [ SRCategory(SR.CatBehavior), DefaultValue(0), Bindable(true), SRDescription(SR.ScrollBarValueDescr) ] public int Value { get { return value; } set { if (this.value != value) { if (value < minimum || value > maximum) { throw new ArgumentOutOfRangeException("Value", SR.GetString(SR.InvalidBoundArgument, "Value", (value).ToString(CultureInfo.CurrentCulture), "'minimum'", "'maximum'")); } this.value = value; UpdateScrollInfo(); parent.SetDisplayFromScrollProps(HorizontalDisplayPosition, VerticalDisplayPosition); } } } ////// Gets or sets a numeric value that represents the current /// position of the scroll box /// on /// the scroll bar control. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.ScrollBarVisibleDescr) ] public bool Visible { get { return visible; } set { if (parent.AutoScroll) { return; } if (value != visible) { visible = value; parent.UpdateStylesCore (); UpdateScrollInfo(); parent.SetDisplayFromScrollProps(HorizontalDisplayPosition, VerticalDisplayPosition); } } } ////// Gets or sets a bool value controlling whether the scrollbar is showing. /// ////// /// Internal helper method /// ///internal void UpdateScrollInfo() { if (parent.IsHandleCreated && visible) { NativeMethods.SCROLLINFO si = new NativeMethods.SCROLLINFO(); si.cbSize = Marshal.SizeOf(typeof(NativeMethods.SCROLLINFO)); si.fMask = NativeMethods.SIF_ALL; si.nMin = minimum; si.nMax = maximum; si.nPage = (parent.AutoScroll) ? PageSize : LargeChange; si.nPos = value; si.nTrackPos = 0; UnsafeNativeMethods.SetScrollInfo(new HandleRef(parent, parent.Handle), Orientation, si, true); } } /// /// /// Internal helper method for enabling or disabling the Vertical Scroll bar. /// ///private void EnableScroll(bool enable) { if (enable) { UnsafeNativeMethods.EnableScrollBar(new HandleRef(parent, parent.Handle), Orientation, NativeMethods.ESB_ENABLE_BOTH); } else { UnsafeNativeMethods.EnableScrollBar(new HandleRef(parent, parent.Handle), Orientation, NativeMethods.ESB_DISABLE_BOTH); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
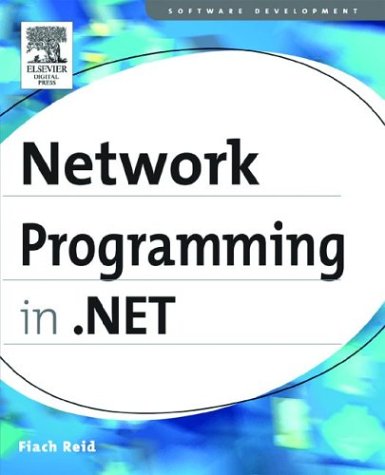
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Compiler.cs
- BooleanStorage.cs
- EnumerableRowCollection.cs
- TlsnegoTokenProvider.cs
- HGlobalSafeHandle.cs
- ContentTextAutomationPeer.cs
- Configuration.cs
- DefaultShape.cs
- EastAsianLunisolarCalendar.cs
- _ChunkParse.cs
- Overlapped.cs
- HighlightComponent.cs
- _LoggingObject.cs
- TreeNode.cs
- XmlSchemaExternal.cs
- ObjectIDGenerator.cs
- SessionSwitchEventArgs.cs
- ToolstripProfessionalRenderer.cs
- SafeFileMapViewHandle.cs
- WaitHandleCannotBeOpenedException.cs
- CompareValidator.cs
- SoapExtensionReflector.cs
- _NTAuthentication.cs
- ConstraintEnumerator.cs
- AppDomainResourcePerfCounters.cs
- MultiPropertyDescriptorGridEntry.cs
- RichTextBox.cs
- newitemfactory.cs
- InfoCardRSACryptoProvider.cs
- XmlSchemaExporter.cs
- TimerEventSubscription.cs
- ProtocolsConfigurationEntry.cs
- PersonalizationStateInfoCollection.cs
- OracleDataAdapter.cs
- UInt16.cs
- UndirectedGraph.cs
- SerializationInfoEnumerator.cs
- StackBuilderSink.cs
- DocumentViewerConstants.cs
- FormParameter.cs
- ValueTypeFixupInfo.cs
- ParsedAttributeCollection.cs
- AttachInfo.cs
- XmlObjectSerializerReadContext.cs
- ExitEventArgs.cs
- SecurityContextKeyIdentifierClause.cs
- UnmanagedMemoryStreamWrapper.cs
- OrderingQueryOperator.cs
- CodeGeneratorAttribute.cs
- SerializableReadOnlyDictionary.cs
- DataGridViewCellParsingEventArgs.cs
- GifBitmapDecoder.cs
- Rect3D.cs
- SqlRowUpdatedEvent.cs
- TreeView.cs
- XmlDictionaryReaderQuotasElement.cs
- DeploymentExceptionMapper.cs
- Trace.cs
- LinearGradientBrush.cs
- dataobject.cs
- QilVisitor.cs
- RectIndependentAnimationStorage.cs
- ColumnWidthChangedEvent.cs
- XamlFigureLengthSerializer.cs
- DescendentsWalker.cs
- FieldTemplateFactory.cs
- RegisteredHiddenField.cs
- XamlPathDataSerializer.cs
- GeneralTransformGroup.cs
- TableLayoutSettingsTypeConverter.cs
- WebSysDisplayNameAttribute.cs
- CompoundFileStorageReference.cs
- BuildResultCache.cs
- RegexWorker.cs
- LinkLabel.cs
- ReaderOutput.cs
- SqlXmlStorage.cs
- GridViewDesigner.cs
- EntityWithKeyStrategy.cs
- BindToObject.cs
- DataPointer.cs
- RegisteredScript.cs
- ClientTargetCollection.cs
- IssuedTokenClientElement.cs
- ServiceNameCollection.cs
- SqlCacheDependency.cs
- X509Extension.cs
- UpdateEventArgs.cs
- ConnectorMovedEventArgs.cs
- List.cs
- _HeaderInfo.cs
- EventTask.cs
- PrePrepareMethodAttribute.cs
- SqlError.cs
- ImageMapEventArgs.cs
- ModelItemCollectionImpl.cs
- SmiConnection.cs
- GPPOINTF.cs
- UpdateException.cs
- TranslateTransform.cs