Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Runtime / InteropServices / StandardOleMarshalObject.cs / 2 / StandardOleMarshalObject.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Runtime.InteropServices { using System.Diagnostics; using System; using Microsoft.Win32; using System.Security; using System.Security.Permissions; ////// /// /// Replaces the standard CLR free-threaded marshaler with the standard OLE STA one. This prevents the calls made into /// our hosting object by OLE from coming in on threads other than the UI thread. /// /// [ComVisible(true)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Interoperability", "CA1403:AutoLayoutTypesShouldNotBeComVisible")] public class StandardOleMarshalObject : MarshalByRefObject, UnsafeNativeMethods.IMarshal { protected StandardOleMarshalObject() { } [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] private IntPtr GetStdMarshaller(ref Guid riid, int dwDestContext, int mshlflags) { IntPtr pStdMarshal = IntPtr.Zero; IntPtr pUnk = Marshal.GetIUnknownForObject(this); if (pUnk != IntPtr.Zero) { try { if (NativeMethods.S_OK == UnsafeNativeMethods.CoGetStandardMarshal(ref riid, pUnk, dwDestContext, IntPtr.Zero, mshlflags, out pStdMarshal)) { Debug.Assert(pStdMarshal != null, "Failed to get marshaller for interface '" + riid.ToString() + "', CoGetStandardMarshal returned S_OK"); return pStdMarshal; } } finally { Marshal.Release(pUnk); } } throw new InvalidOperationException(SR.GetString(SR.StandardOleMarshalObjectGetMarshalerFailed, riid.ToString())); } ////// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.GetUnmarshalClass(ref Guid riid, IntPtr pv, int dwDestContext, IntPtr pvDestContext, int mshlflags, out Guid pCid){ pCid = typeof(UnsafeNativeMethods.IStdMarshal).GUID; return NativeMethods.S_OK; } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.GetMarshalSizeMax(ref Guid riid, IntPtr pv, int dwDestContext, IntPtr pvDestContext, int mshlflags, out int pSize) { // 98830 - GUID marshaling in StandardOleMarshalObject AVs on 64-bit Guid riid_copy = riid; IntPtr pStandardMarshal = GetStdMarshaller(ref riid_copy, dwDestContext, mshlflags); try { return UnsafeNativeMethods.CoGetMarshalSizeMax(out pSize, ref riid_copy, pStandardMarshal, dwDestContext, pvDestContext, mshlflags); } finally { Marshal.Release(pStandardMarshal); } } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.MarshalInterface(object pStm, ref Guid riid, IntPtr pv, int dwDestContext, IntPtr pvDestContext, int mshlflags) { IntPtr pStandardMarshal = GetStdMarshaller(ref riid, dwDestContext, mshlflags); try { return UnsafeNativeMethods.CoMarshalInterface(pStm, ref riid, pStandardMarshal, dwDestContext, pvDestContext, mshlflags); } finally { Marshal.Release(pStandardMarshal); if (pStm != null) { Marshal.ReleaseComObject(pStm); } } } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.UnmarshalInterface(object pStm, ref Guid riid, out IntPtr ppv) { // this should never be called on this interface, but on the standard one handed back by the previous calls. Debug.Fail("IMarshal::UnmarshalInterface should not be called."); ppv = IntPtr.Zero; if (pStm != null) { Marshal.ReleaseComObject(pStm); } return NativeMethods.E_NOTIMPL; } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.ReleaseMarshalData(object pStm) { // this should never be called on this interface, but on the standard one handed back by the previous calls. Debug.Fail("IMarshal::ReleaseMarshalData should not be called."); if (pStm != null) { Marshal.ReleaseComObject(pStm); } return NativeMethods.E_NOTIMPL; } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.DisconnectObject(int dwReserved) { // this should never be called on this interface, but on the standard one handed back by the previous calls. Debug.Fail("IMarshal::DisconnectObject should not be called."); return NativeMethods.E_NOTIMPL; } } }
Link Menu
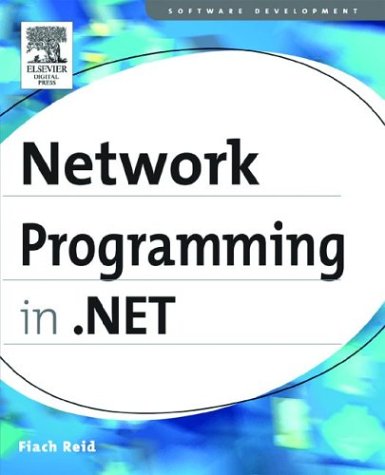
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Stylus.cs
- BindingSourceDesigner.cs
- XmlWrappingWriter.cs
- ScriptingWebServicesSectionGroup.cs
- Int32Converter.cs
- MdiWindowListStrip.cs
- StyleCollection.cs
- SmiMetaDataProperty.cs
- ComAdminInterfaces.cs
- Resources.Designer.cs
- UserPreferenceChangedEventArgs.cs
- ItemContainerPattern.cs
- DeclarationUpdate.cs
- ObjectIDGenerator.cs
- HtmlElementErrorEventArgs.cs
- TextUtf8RawTextWriter.cs
- ObjectStateEntry.cs
- ApplicationFileCodeDomTreeGenerator.cs
- AppDomainUnloadedException.cs
- StreamUpdate.cs
- SQLInt32.cs
- HostDesigntimeLicenseContext.cs
- WMIInterop.cs
- DerivedKeyCachingSecurityTokenSerializer.cs
- NonValidatingSecurityTokenAuthenticator.cs
- XmlHierarchicalEnumerable.cs
- PageEventArgs.cs
- NullReferenceException.cs
- DesignTimeTemplateParser.cs
- DropShadowBitmapEffect.cs
- AutoResizedEvent.cs
- CodeCommentStatementCollection.cs
- SqlWebEventProvider.cs
- GregorianCalendarHelper.cs
- SendMailErrorEventArgs.cs
- BinaryFormatter.cs
- ColorTransformHelper.cs
- TextTreeDeleteContentUndoUnit.cs
- Splitter.cs
- EmptyEnumerable.cs
- HtmlTableCell.cs
- DependsOnAttribute.cs
- BaseAddressPrefixFilterElementCollection.cs
- MenuEventArgs.cs
- Parser.cs
- webeventbuffer.cs
- ParserContext.cs
- ImpersonateTokenRef.cs
- InvalidPropValue.cs
- ProfilePropertySettingsCollection.cs
- ConfigUtil.cs
- HttpListenerResponse.cs
- ElementHostPropertyMap.cs
- VersionedStream.cs
- NullableIntMinMaxAggregationOperator.cs
- Adorner.cs
- CommandCollectionEditor.cs
- ActiveDocumentEvent.cs
- SafeFreeMibTable.cs
- ListView.cs
- Link.cs
- PointAnimation.cs
- SmtpTransport.cs
- MetaTable.cs
- DurableRuntimeValidator.cs
- SystemInfo.cs
- IndexOutOfRangeException.cs
- CollectionsUtil.cs
- Rect.cs
- ConfigurationSectionGroupCollection.cs
- SoapConverter.cs
- SqlDependency.cs
- StylusPlugInCollection.cs
- SerialErrors.cs
- ReadContentAsBinaryHelper.cs
- DataQuery.cs
- SignatureDescription.cs
- TypeToken.cs
- CodeExporter.cs
- DbConnectionStringCommon.cs
- SizeAnimation.cs
- RectangleHotSpot.cs
- RepeaterDataBoundAdapter.cs
- Storyboard.cs
- GCHandleCookieTable.cs
- FloaterBaseParaClient.cs
- HtmlProps.cs
- COAUTHINFO.cs
- ThicknessAnimation.cs
- BulletChrome.cs
- HttpCacheVary.cs
- UrlMapping.cs
- BuildProvider.cs
- CustomCredentialPolicy.cs
- PrinterSettings.cs
- AlternationConverter.cs
- Point4DConverter.cs
- _LocalDataStore.cs
- ConnectionsZoneAutoFormat.cs
- SetState.cs