Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Data / System / Data / Common / DBCommand.cs / 1 / DBCommand.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.ComponentModel; using System.Data; #if WINFSInternalOnly internal #else public #endif abstract class DbCommand : Component, IDbCommand { // V1.2.3300 protected DbCommand() : base() { } [ DefaultValue(""), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandText), ] abstract public string CommandText { get; set; } [ ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandTimeout), ] abstract public int CommandTimeout { get; set; } [ DefaultValue(System.Data.CommandType.Text), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandType), ] abstract public CommandType CommandType { get; set; } [ Browsable(false), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_Connection), ] public DbConnection Connection { get { return DbConnection; } set { DbConnection = value; } } IDbConnection IDbCommand.Connection { get { return DbConnection; } set { DbConnection = (DbConnection)value; } } abstract protected DbConnection DbConnection { // V1.2.3300 get; set; } abstract protected DbParameterCollection DbParameterCollection { // V1.2.3300 get; } abstract protected DbTransaction DbTransaction { // V1.2.3300 get; set; } // @devnote: By default, the cmd object is visible on the design surface (i.e. VS7 Server Tray) // to limit the number of components that clutter the design surface, // when the DataAdapter design wizard generates the insert/update/delete commands it will // set the DesignTimeVisible property to false so that cmds won't appear as individual objects [ DefaultValue(true), DesignOnly(true), Browsable(false), EditorBrowsableAttribute(EditorBrowsableState.Never), ] public abstract bool DesignTimeVisible { get; set; } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_Parameters), ] public DbParameterCollection Parameters { get { return DbParameterCollection; } } IDataParameterCollection IDbCommand.Parameters { get { return (DbParameterCollection)DbParameterCollection; } } [ Browsable(false), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResDescriptionAttribute(Res.DbCommand_Transaction), ] public DbTransaction Transaction { get { return DbTransaction; } set { DbTransaction = value; } } IDbTransaction IDbCommand.Transaction { get { return DbTransaction; } set { DbTransaction = (DbTransaction)value; } } [ DefaultValue(System.Data.UpdateRowSource.Both), ResCategoryAttribute(Res.DataCategory_Update), ResDescriptionAttribute(Res.DbCommand_UpdatedRowSource), ] abstract public UpdateRowSource UpdatedRowSource { get; set; } abstract public void Cancel(); public DbParameter CreateParameter(){ // V1.2.3300 return CreateDbParameter(); } IDbDataParameter IDbCommand.CreateParameter() { // V1.2.3300 return CreateDbParameter(); } abstract protected DbParameter CreateDbParameter(); abstract protected DbDataReader ExecuteDbDataReader(CommandBehavior behavior); abstract public int ExecuteNonQuery(); public DbDataReader ExecuteReader() { return (DbDataReader)ExecuteDbDataReader(CommandBehavior.Default); } IDataReader IDbCommand.ExecuteReader() { return (DbDataReader)ExecuteDbDataReader(CommandBehavior.Default); } public DbDataReader ExecuteReader(CommandBehavior behavior){ return (DbDataReader)ExecuteDbDataReader(behavior); } IDataReader IDbCommand.ExecuteReader(CommandBehavior behavior) { return (DbDataReader)ExecuteDbDataReader(behavior); } abstract public object ExecuteScalar(); abstract public void Prepare(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.ComponentModel; using System.Data; #if WINFSInternalOnly internal #else public #endif abstract class DbCommand : Component, IDbCommand { // V1.2.3300 protected DbCommand() : base() { } [ DefaultValue(""), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandText), ] abstract public string CommandText { get; set; } [ ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandTimeout), ] abstract public int CommandTimeout { get; set; } [ DefaultValue(System.Data.CommandType.Text), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandType), ] abstract public CommandType CommandType { get; set; } [ Browsable(false), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_Connection), ] public DbConnection Connection { get { return DbConnection; } set { DbConnection = value; } } IDbConnection IDbCommand.Connection { get { return DbConnection; } set { DbConnection = (DbConnection)value; } } abstract protected DbConnection DbConnection { // V1.2.3300 get; set; } abstract protected DbParameterCollection DbParameterCollection { // V1.2.3300 get; } abstract protected DbTransaction DbTransaction { // V1.2.3300 get; set; } // @devnote: By default, the cmd object is visible on the design surface (i.e. VS7 Server Tray) // to limit the number of components that clutter the design surface, // when the DataAdapter design wizard generates the insert/update/delete commands it will // set the DesignTimeVisible property to false so that cmds won't appear as individual objects [ DefaultValue(true), DesignOnly(true), Browsable(false), EditorBrowsableAttribute(EditorBrowsableState.Never), ] public abstract bool DesignTimeVisible { get; set; } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_Parameters), ] public DbParameterCollection Parameters { get { return DbParameterCollection; } } IDataParameterCollection IDbCommand.Parameters { get { return (DbParameterCollection)DbParameterCollection; } } [ Browsable(false), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResDescriptionAttribute(Res.DbCommand_Transaction), ] public DbTransaction Transaction { get { return DbTransaction; } set { DbTransaction = value; } } IDbTransaction IDbCommand.Transaction { get { return DbTransaction; } set { DbTransaction = (DbTransaction)value; } } [ DefaultValue(System.Data.UpdateRowSource.Both), ResCategoryAttribute(Res.DataCategory_Update), ResDescriptionAttribute(Res.DbCommand_UpdatedRowSource), ] abstract public UpdateRowSource UpdatedRowSource { get; set; } abstract public void Cancel(); public DbParameter CreateParameter(){ // V1.2.3300 return CreateDbParameter(); } IDbDataParameter IDbCommand.CreateParameter() { // V1.2.3300 return CreateDbParameter(); } abstract protected DbParameter CreateDbParameter(); abstract protected DbDataReader ExecuteDbDataReader(CommandBehavior behavior); abstract public int ExecuteNonQuery(); public DbDataReader ExecuteReader() { return (DbDataReader)ExecuteDbDataReader(CommandBehavior.Default); } IDataReader IDbCommand.ExecuteReader() { return (DbDataReader)ExecuteDbDataReader(CommandBehavior.Default); } public DbDataReader ExecuteReader(CommandBehavior behavior){ return (DbDataReader)ExecuteDbDataReader(behavior); } IDataReader IDbCommand.ExecuteReader(CommandBehavior behavior) { return (DbDataReader)ExecuteDbDataReader(behavior); } abstract public object ExecuteScalar(); abstract public void Prepare(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
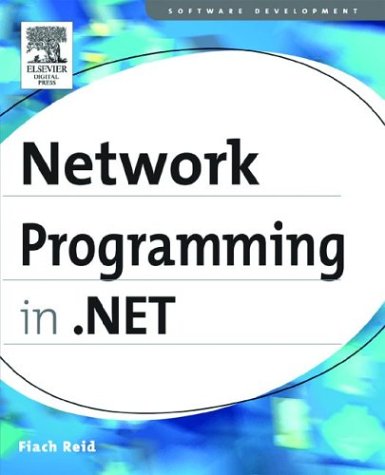
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridCheckBoxColumn.cs
- TimeoutException.cs
- ScriptResourceHandler.cs
- ListInitExpression.cs
- Viewport3DAutomationPeer.cs
- QilStrConcat.cs
- NullableBoolConverter.cs
- RijndaelManagedTransform.cs
- CryptoConfig.cs
- OdbcCommandBuilder.cs
- HashRepartitionStream.cs
- SignalGate.cs
- CryptoConfig.cs
- Form.cs
- DbConnectionClosed.cs
- TableRow.cs
- UnmanagedHandle.cs
- ToolStrip.cs
- JsonMessageEncoderFactory.cs
- ToolStripInSituService.cs
- HttpRequestTraceRecord.cs
- HtmlInputSubmit.cs
- FileUpload.cs
- HandleCollector.cs
- ClientSideQueueItem.cs
- _ConnectOverlappedAsyncResult.cs
- AvTraceFormat.cs
- CookieHandler.cs
- EdmComplexPropertyAttribute.cs
- Menu.cs
- EntityClassGenerator.cs
- HMAC.cs
- CalendarDataBindingHandler.cs
- smtpconnection.cs
- nulltextnavigator.cs
- CopyNodeSetAction.cs
- ElementProxy.cs
- SchemaConstraints.cs
- DataGridViewTopLeftHeaderCell.cs
- DirectoryInfo.cs
- SweepDirectionValidation.cs
- Storyboard.cs
- StringStorage.cs
- TabletDevice.cs
- AssemblyBuilder.cs
- MouseEventArgs.cs
- ListViewTableCell.cs
- TableLayoutSettings.cs
- BrowserTree.cs
- SpellCheck.cs
- EmptyStringExpandableObjectConverter.cs
- GridToolTip.cs
- RuntimeUtils.cs
- RSAOAEPKeyExchangeFormatter.cs
- ListParaClient.cs
- AstNode.cs
- DataFormats.cs
- ComponentSerializationService.cs
- ApplicationTrust.cs
- TemplateComponentConnector.cs
- GridView.cs
- AsymmetricSignatureFormatter.cs
- ZoneButton.cs
- XmlText.cs
- Table.cs
- NamedServiceModelExtensionCollectionElement.cs
- SslStream.cs
- DataObjectEventArgs.cs
- WindowsToolbar.cs
- ToolStripItemTextRenderEventArgs.cs
- RowBinding.cs
- ValidatorCompatibilityHelper.cs
- DisposableCollectionWrapper.cs
- XmlWrappingWriter.cs
- SqlCommandBuilder.cs
- IdentityHolder.cs
- ResourceProperty.cs
- CachedFontFamily.cs
- BitmapDecoder.cs
- DefaultDiscoveryService.cs
- Matrix.cs
- ArgIterator.cs
- XamlBrushSerializer.cs
- X509PeerCertificateAuthenticationElement.cs
- COM2IDispatchConverter.cs
- FixedSOMTableCell.cs
- DrawItemEvent.cs
- InfiniteTimeSpanConverter.cs
- CommentGlyph.cs
- DocumentDesigner.cs
- HandlerFactoryCache.cs
- Stackframe.cs
- RectangleF.cs
- ConfigurationException.cs
- TransactionFlowBindingElementImporter.cs
- InternalControlCollection.cs
- CodeExporter.cs
- SynchronizationContext.cs
- TemplateKeyConverter.cs
- SmtpException.cs