Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Printing / PrintDocument.cs / 1 / PrintDocument.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using Microsoft.Win32; using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.Reflection; using System.Security; using System.Security.Permissions; using System.IO; ////// /// [ ToolboxItemFilter("System.Drawing.Printing"), DefaultProperty("DocumentName"), SRDescription(SR.PrintDocumentDesc), DefaultEvent("PrintPage") ] public class PrintDocument : Component { private string documentName = "document"; private PrintEventHandler beginPrintHandler; private PrintEventHandler endPrintHandler; private PrintPageEventHandler printPageHandler; private QueryPageSettingsEventHandler queryHandler; private PrinterSettings printerSettings = new PrinterSettings(); private PageSettings defaultPageSettings; private PrintController printController; private bool originAtMargins; private bool userSetPageSettings; ///Defines a reusable object that sends output to the /// printer. ////// /// public PrintDocument() { defaultPageSettings = new PageSettings(printerSettings); } ///Initializes a new instance of the ////// class. /// /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.PDOCdocumentPageSettingsDescr) ] public PageSettings DefaultPageSettings { get { return defaultPageSettings;} set { if (value == null) value = new PageSettings(); defaultPageSettings = value; userSetPageSettings = true; } } ///Gets or sets the /// default /// page settings for the document being printed. ////// /// [ DefaultValue("document"), SRDescription(SR.PDOCdocumentNameDescr) ] public string DocumentName { get { return documentName;} set { if (value == null) value = ""; documentName = value; } } ///Gets or sets the name to display to the user while printing the document; /// for example, in a print status dialog or a printer /// queue. ///// If true, positions the origin of the graphics object // associated with the page at the point just inside // the user-specified margins of the page. // If false, the graphics origin is at the top-left // corner of the printable area of the page. // [ DefaultValue(false), SRDescription(SR.PDOCoriginAtMarginsDescr) ] public bool OriginAtMargins { get { return originAtMargins; } set { originAtMargins = value; } } /// /// /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.PDOCprintControllerDescr) ] public PrintController PrintController { get { IntSecurity.SafePrinting.Demand(); if (printController == null) { printController = new StandardPrintController(); new ReflectionPermission(PermissionState.Unrestricted).Assert(); try { try { // SECREVIEW 332064: this is here because System.Drawing doesnt want a dependency on // System.Windows.Forms. Since this creates a public type in another assembly, this // appears to be OK. Type type = Type.GetType("System.Windows.Forms.PrintControllerWithStatusDialog, " + AssemblyRef.SystemWindowsForms); printController = (PrintController) Activator.CreateInstance(type, BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.CreateInstance, null, new object[] { printController}, null); } catch (TypeLoadException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (TargetInvocationException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (MissingMethodException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (MethodAccessException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (MemberAccessException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (FileNotFoundException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } } finally { CodeAccessPermission.RevertAssert(); } } return printController; } set { IntSecurity.SafePrinting.Demand(); printController = value; } } ///Gets or sets the ////// that guides the printing process. /// /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.PDOCprinterSettingsDescr) ] public PrinterSettings PrinterSettings { get { return printerSettings;} set { if (value == null) value = new PrinterSettings(); printerSettings = value; // reset the PageSettings that match the PrinterSettings only if we have created the defaultPageSettings.. if (!userSetPageSettings) { defaultPageSettings = printerSettings.DefaultPageSettings; } } } ///Gets or sets the printer on which the /// document is printed. ////// /// [SRDescription(SR.PDOCbeginPrintDescr)] public event PrintEventHandler BeginPrint { add { beginPrintHandler += value; } remove { beginPrintHandler -= value; } } ///Occurs when the ///method is called, before /// the /// first page prints. /// /// [SRDescription(SR.PDOCendPrintDescr)] public event PrintEventHandler EndPrint { add { endPrintHandler += value; } remove { endPrintHandler -= value; } } ///Occurs when ///is /// called, after the last page is printed. /// /// [SRDescription(SR.PDOCprintPageDescr)] public event PrintPageEventHandler PrintPage { add { printPageHandler += value; } remove { printPageHandler -= value; } } ///Occurs when a page is printed. ////// /// [SRDescription(SR.PDOCqueryPageSettingsDescr)] public event QueryPageSettingsEventHandler QueryPageSettings { add { queryHandler += value; } remove { queryHandler -= value; } } internal void _OnBeginPrint(PrintEventArgs e) { OnBeginPrint(e); } ///Occurs ////// /// protected virtual void OnBeginPrint(PrintEventArgs e) { if (beginPrintHandler != null) beginPrintHandler(this, e); } internal void _OnEndPrint(PrintEventArgs e) { OnEndPrint(e); } ///Raises the ////// event. /// /// protected virtual void OnEndPrint(PrintEventArgs e) { if (endPrintHandler != null) endPrintHandler(this, e); } internal void _OnPrintPage(PrintPageEventArgs e) { OnPrintPage(e); } ///Raises the ////// event. /// /// // protected virtual void OnPrintPage(PrintPageEventArgs e) { if (printPageHandler != null) printPageHandler(this, e); } internal void _OnQueryPageSettings(QueryPageSettingsEventArgs e) { OnQueryPageSettings(e); } ///Raises the ////// event. /// /// protected virtual void OnQueryPageSettings(QueryPageSettingsEventArgs e) { if (queryHandler != null) queryHandler(this, e); } ///Raises the ///event. /// /// public void Print() { // It is possible to SetPrinterName using signed secured dll which can be used to by-pass Printing security model. // hence here check if the PrinterSettings.IsDefaultPrinter and if not demand AllPrinting. // Refer : VsWhidbey : 235920 if (!this.PrinterSettings.IsDefaultPrinter && !this.PrinterSettings.PrintDialogDisplayed) { IntSecurity.AllPrinting.Demand(); } PrintController controller = PrintController; controller.Print(this); } ////// Prints the document. /// ////// /// /// public override string ToString() { return "[PrintDocument " + DocumentName + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Provides some interesting information about the PrintDocument in /// String form. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using Microsoft.Win32; using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.Reflection; using System.Security; using System.Security.Permissions; using System.IO; ////// /// [ ToolboxItemFilter("System.Drawing.Printing"), DefaultProperty("DocumentName"), SRDescription(SR.PrintDocumentDesc), DefaultEvent("PrintPage") ] public class PrintDocument : Component { private string documentName = "document"; private PrintEventHandler beginPrintHandler; private PrintEventHandler endPrintHandler; private PrintPageEventHandler printPageHandler; private QueryPageSettingsEventHandler queryHandler; private PrinterSettings printerSettings = new PrinterSettings(); private PageSettings defaultPageSettings; private PrintController printController; private bool originAtMargins; private bool userSetPageSettings; ///Defines a reusable object that sends output to the /// printer. ////// /// public PrintDocument() { defaultPageSettings = new PageSettings(printerSettings); } ///Initializes a new instance of the ////// class. /// /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.PDOCdocumentPageSettingsDescr) ] public PageSettings DefaultPageSettings { get { return defaultPageSettings;} set { if (value == null) value = new PageSettings(); defaultPageSettings = value; userSetPageSettings = true; } } ///Gets or sets the /// default /// page settings for the document being printed. ////// /// [ DefaultValue("document"), SRDescription(SR.PDOCdocumentNameDescr) ] public string DocumentName { get { return documentName;} set { if (value == null) value = ""; documentName = value; } } ///Gets or sets the name to display to the user while printing the document; /// for example, in a print status dialog or a printer /// queue. ///// If true, positions the origin of the graphics object // associated with the page at the point just inside // the user-specified margins of the page. // If false, the graphics origin is at the top-left // corner of the printable area of the page. // [ DefaultValue(false), SRDescription(SR.PDOCoriginAtMarginsDescr) ] public bool OriginAtMargins { get { return originAtMargins; } set { originAtMargins = value; } } /// /// /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.PDOCprintControllerDescr) ] public PrintController PrintController { get { IntSecurity.SafePrinting.Demand(); if (printController == null) { printController = new StandardPrintController(); new ReflectionPermission(PermissionState.Unrestricted).Assert(); try { try { // SECREVIEW 332064: this is here because System.Drawing doesnt want a dependency on // System.Windows.Forms. Since this creates a public type in another assembly, this // appears to be OK. Type type = Type.GetType("System.Windows.Forms.PrintControllerWithStatusDialog, " + AssemblyRef.SystemWindowsForms); printController = (PrintController) Activator.CreateInstance(type, BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.CreateInstance, null, new object[] { printController}, null); } catch (TypeLoadException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (TargetInvocationException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (MissingMethodException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (MethodAccessException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (MemberAccessException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } catch (FileNotFoundException) { Debug.Fail("Can't find System.Windows.Forms.PrintControllerWithStatusDialog, proceeding with StandardPrintController"); } } finally { CodeAccessPermission.RevertAssert(); } } return printController; } set { IntSecurity.SafePrinting.Demand(); printController = value; } } ///Gets or sets the ////// that guides the printing process. /// /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.PDOCprinterSettingsDescr) ] public PrinterSettings PrinterSettings { get { return printerSettings;} set { if (value == null) value = new PrinterSettings(); printerSettings = value; // reset the PageSettings that match the PrinterSettings only if we have created the defaultPageSettings.. if (!userSetPageSettings) { defaultPageSettings = printerSettings.DefaultPageSettings; } } } ///Gets or sets the printer on which the /// document is printed. ////// /// [SRDescription(SR.PDOCbeginPrintDescr)] public event PrintEventHandler BeginPrint { add { beginPrintHandler += value; } remove { beginPrintHandler -= value; } } ///Occurs when the ///method is called, before /// the /// first page prints. /// /// [SRDescription(SR.PDOCendPrintDescr)] public event PrintEventHandler EndPrint { add { endPrintHandler += value; } remove { endPrintHandler -= value; } } ///Occurs when ///is /// called, after the last page is printed. /// /// [SRDescription(SR.PDOCprintPageDescr)] public event PrintPageEventHandler PrintPage { add { printPageHandler += value; } remove { printPageHandler -= value; } } ///Occurs when a page is printed. ////// /// [SRDescription(SR.PDOCqueryPageSettingsDescr)] public event QueryPageSettingsEventHandler QueryPageSettings { add { queryHandler += value; } remove { queryHandler -= value; } } internal void _OnBeginPrint(PrintEventArgs e) { OnBeginPrint(e); } ///Occurs ////// /// protected virtual void OnBeginPrint(PrintEventArgs e) { if (beginPrintHandler != null) beginPrintHandler(this, e); } internal void _OnEndPrint(PrintEventArgs e) { OnEndPrint(e); } ///Raises the ////// event. /// /// protected virtual void OnEndPrint(PrintEventArgs e) { if (endPrintHandler != null) endPrintHandler(this, e); } internal void _OnPrintPage(PrintPageEventArgs e) { OnPrintPage(e); } ///Raises the ////// event. /// /// // protected virtual void OnPrintPage(PrintPageEventArgs e) { if (printPageHandler != null) printPageHandler(this, e); } internal void _OnQueryPageSettings(QueryPageSettingsEventArgs e) { OnQueryPageSettings(e); } ///Raises the ////// event. /// /// protected virtual void OnQueryPageSettings(QueryPageSettingsEventArgs e) { if (queryHandler != null) queryHandler(this, e); } ///Raises the ///event. /// /// public void Print() { // It is possible to SetPrinterName using signed secured dll which can be used to by-pass Printing security model. // hence here check if the PrinterSettings.IsDefaultPrinter and if not demand AllPrinting. // Refer : VsWhidbey : 235920 if (!this.PrinterSettings.IsDefaultPrinter && !this.PrinterSettings.PrintDialogDisplayed) { IntSecurity.AllPrinting.Demand(); } PrintController controller = PrintController; controller.Print(this); } ////// Prints the document. /// ////// /// /// public override string ToString() { return "[PrintDocument " + DocumentName + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Provides some interesting information about the PrintDocument in /// String form. /// ///
Link Menu
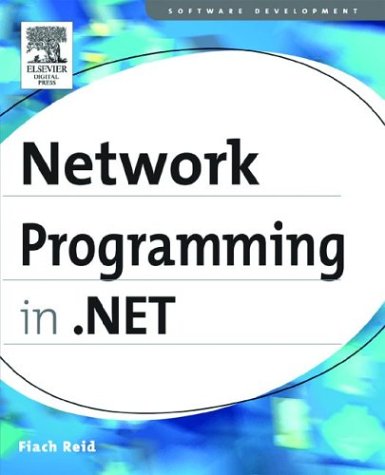
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BindingNavigator.cs
- SequenceRange.cs
- BitmapEffectOutputConnector.cs
- cookiecollection.cs
- FlatButtonAppearance.cs
- RtfToXamlReader.cs
- XPathDocument.cs
- GradientStopCollection.cs
- EntryIndex.cs
- OracleCommand.cs
- MDIControlStrip.cs
- Rect3DValueSerializer.cs
- DictionaryBase.cs
- MultiBinding.cs
- GuidelineCollection.cs
- SqlMultiplexer.cs
- UnionCqlBlock.cs
- GradientStop.cs
- XmlILStorageConverter.cs
- FillBehavior.cs
- XmlAtomicValue.cs
- DataList.cs
- precedingsibling.cs
- HttpEncoderUtility.cs
- XPathAncestorQuery.cs
- listitem.cs
- UserControl.cs
- SchemaImporter.cs
- ObjectRef.cs
- RuleSettings.cs
- ExpressionBuilder.cs
- EntitySqlException.cs
- ButtonBase.cs
- ExceptionUtil.cs
- SecurityListenerSettingsLifetimeManager.cs
- ValidatingReaderNodeData.cs
- WindowsScrollBar.cs
- ReadOnlyNameValueCollection.cs
- propertytag.cs
- UserControlAutomationPeer.cs
- WindowsClaimSet.cs
- SapiRecognizer.cs
- NamespaceDisplay.xaml.cs
- ClientType.cs
- FixUp.cs
- WsatTransactionFormatter.cs
- Vector3DAnimation.cs
- GenerateTemporaryAssemblyTask.cs
- HttpDebugHandler.cs
- MonthCalendar.cs
- WebPartConnectionsConfigureVerb.cs
- OrderByQueryOptionExpression.cs
- TypedLocationWrapper.cs
- CodeMemberEvent.cs
- StateWorkerRequest.cs
- Error.cs
- BaseAppDomainProtocolHandler.cs
- securitycriticaldataformultiplegetandset.cs
- EmptyTextWriter.cs
- WebBrowserBase.cs
- InputReferenceExpression.cs
- KeyPressEvent.cs
- contentDescriptor.cs
- GlyphTypeface.cs
- Attributes.cs
- ExtendedProtectionPolicyTypeConverter.cs
- MobileControlDesigner.cs
- XPathNavigator.cs
- PriorityQueue.cs
- XmlSchemaAttributeGroupRef.cs
- WebFaultException.cs
- ModelUIElement3D.cs
- TextEffectCollection.cs
- WebBrowserHelper.cs
- CanExecuteRoutedEventArgs.cs
- TextCollapsingProperties.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- NativeMethods.cs
- Expression.cs
- NativeMethods.cs
- ModifierKeysValueSerializer.cs
- QueryGeneratorBase.cs
- SHA384.cs
- WizardPanel.cs
- SortDescription.cs
- Decoder.cs
- EventData.cs
- ShortcutKeysEditor.cs
- FormView.cs
- ProfileManager.cs
- ServiceObjectContainer.cs
- StoreContentChangedEventArgs.cs
- DataSvcMapFileSerializer.cs
- FontTypeConverter.cs
- ImageCodecInfoPrivate.cs
- XmlSerializerFormatAttribute.cs
- BooleanExpr.cs
- ExitEventArgs.cs
- SplashScreenNativeMethods.cs
- VerticalConnector.xaml.cs