Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Automation / Peers / ItemAutomationPeer.cs / 1 / ItemAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public abstract class ItemAutomationPeer : AutomationPeer { /// protected ItemAutomationPeer(object item, ItemsControlAutomationPeer itemsControlAutomationPeer): base() { _item = item; _itemsControlAutomationPeer = itemsControlAutomationPeer; } internal UIElement GetWrapper() { UIElement wrapper = null; ItemsControl owner = (ItemsControl)(_itemsControlAutomationPeer.Owner); if (owner != null) { if (((MS.Internal.Controls.IGeneratorHost)owner).IsItemItsOwnContainer(_item)) wrapper = _item as UIElement; else wrapper = owner.ItemContainerGenerator.ContainerFromItem(_item) as UIElement; } return wrapper; } internal AutomationPeer GetWrapperPeer() { AutomationPeer wrapperPeer = null; UIElement wrapper = GetWrapper(); if(wrapper != null) { wrapperPeer = UIElementAutomationPeer.CreatePeerForElement(wrapper); if(wrapperPeer == null) //fall back to default peer if there is no specific one { if(wrapper is FrameworkElement) wrapperPeer = new FrameworkElementAutomationPeer((FrameworkElement)wrapper); else wrapperPeer = new UIElementAutomationPeer(wrapper); } } return wrapperPeer; } /// override protected string GetItemTypeCore() { return string.Empty; } /// protected override ListGetChildrenCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) { //We need to update children here since the wrapperPeer.UpdateSubtree(); List children = wrapperPeer.GetChildren(); return children; } return null; } /// protected override Rect GetBoundingRectangleCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetBoundingRectangle(); else return new Rect(); } /// protected override bool IsOffscreenCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsOffscreen(); else return true; } /// protected override AutomationOrientation GetOrientationCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetOrientation(); else return AutomationOrientation.None; } /// protected override string GetItemStatusCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetItemStatus(); else return string.Empty; } /// protected override bool IsRequiredForFormCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsRequiredForForm(); else return false; } /// protected override bool IsKeyboardFocusableCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsKeyboardFocusable(); else return false; } /// protected override bool HasKeyboardFocusCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.HasKeyboardFocus(); else return false; } /// protected override bool IsEnabledCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsEnabled(); else return false; } /// protected override bool IsPasswordCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsPassword(); else return false; } /// protected override string GetAutomationIdCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetAutomationId(); else return string.Empty; } /// protected override string GetNameCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); string name = null; if(wrapperPeer != null) name = wrapperPeer.GetName(); if(name == null && _item is string) name = (string)_item; if(name == null) name = string.Empty; return name; } /// protected override bool IsContentElementCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsContentElement(); else return true; } /// protected override bool IsControlElementCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsControlElement(); else return true; } /// protected override AutomationPeer GetLabeledByCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetLabeledBy(); else return null; } /// protected override string GetHelpTextCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetHelpText(); else return string.Empty; } /// protected override string GetAcceleratorKeyCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetAcceleratorKey(); else return string.Empty; } /// protected override string GetAccessKeyCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetAccessKey(); else return string.Empty; } /// protected override Point GetClickablePointCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetClickablePoint(); else return new Point(double.NaN, double.NaN); } /// protected override void SetFocusCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) wrapperPeer.SetFocus(); } /// public object Item { get { return _item; } } /// public ItemsControlAutomationPeer ItemsControlAutomationPeer { get { return _itemsControlAutomationPeer; } } private object _item; private ItemsControlAutomationPeer _itemsControlAutomationPeer; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public abstract class ItemAutomationPeer : AutomationPeer { /// protected ItemAutomationPeer(object item, ItemsControlAutomationPeer itemsControlAutomationPeer): base() { _item = item; _itemsControlAutomationPeer = itemsControlAutomationPeer; } internal UIElement GetWrapper() { UIElement wrapper = null; ItemsControl owner = (ItemsControl)(_itemsControlAutomationPeer.Owner); if (owner != null) { if (((MS.Internal.Controls.IGeneratorHost)owner).IsItemItsOwnContainer(_item)) wrapper = _item as UIElement; else wrapper = owner.ItemContainerGenerator.ContainerFromItem(_item) as UIElement; } return wrapper; } internal AutomationPeer GetWrapperPeer() { AutomationPeer wrapperPeer = null; UIElement wrapper = GetWrapper(); if(wrapper != null) { wrapperPeer = UIElementAutomationPeer.CreatePeerForElement(wrapper); if(wrapperPeer == null) //fall back to default peer if there is no specific one { if(wrapper is FrameworkElement) wrapperPeer = new FrameworkElementAutomationPeer((FrameworkElement)wrapper); else wrapperPeer = new UIElementAutomationPeer(wrapper); } } return wrapperPeer; } /// override protected string GetItemTypeCore() { return string.Empty; } /// protected override List GetChildrenCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) { //We need to update children here since the wrapperPeer.UpdateSubtree(); List children = wrapperPeer.GetChildren(); return children; } return null; } /// protected override Rect GetBoundingRectangleCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetBoundingRectangle(); else return new Rect(); } /// protected override bool IsOffscreenCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsOffscreen(); else return true; } /// protected override AutomationOrientation GetOrientationCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetOrientation(); else return AutomationOrientation.None; } /// protected override string GetItemStatusCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetItemStatus(); else return string.Empty; } /// protected override bool IsRequiredForFormCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsRequiredForForm(); else return false; } /// protected override bool IsKeyboardFocusableCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsKeyboardFocusable(); else return false; } /// protected override bool HasKeyboardFocusCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.HasKeyboardFocus(); else return false; } /// protected override bool IsEnabledCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsEnabled(); else return false; } /// protected override bool IsPasswordCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsPassword(); else return false; } /// protected override string GetAutomationIdCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetAutomationId(); else return string.Empty; } /// protected override string GetNameCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); string name = null; if(wrapperPeer != null) name = wrapperPeer.GetName(); if(name == null && _item is string) name = (string)_item; if(name == null) name = string.Empty; return name; } /// protected override bool IsContentElementCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsContentElement(); else return true; } /// protected override bool IsControlElementCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.IsControlElement(); else return true; } /// protected override AutomationPeer GetLabeledByCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetLabeledBy(); else return null; } /// protected override string GetHelpTextCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetHelpText(); else return string.Empty; } /// protected override string GetAcceleratorKeyCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetAcceleratorKey(); else return string.Empty; } /// protected override string GetAccessKeyCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetAccessKey(); else return string.Empty; } /// protected override Point GetClickablePointCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) return wrapperPeer.GetClickablePoint(); else return new Point(double.NaN, double.NaN); } /// protected override void SetFocusCore() { AutomationPeer wrapperPeer = GetWrapperPeer(); if(wrapperPeer != null) wrapperPeer.SetFocus(); } /// public object Item { get { return _item; } } /// public ItemsControlAutomationPeer ItemsControlAutomationPeer { get { return _itemsControlAutomationPeer; } } private object _item; private ItemsControlAutomationPeer _itemsControlAutomationPeer; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
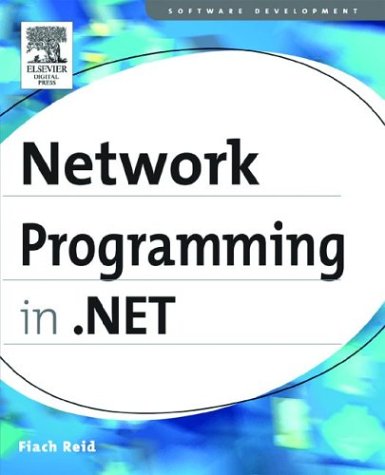
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedSOMGroup.cs
- Environment.cs
- MailAddress.cs
- InstanceDescriptor.cs
- ConsoleKeyInfo.cs
- DbDataReader.cs
- MailDefinition.cs
- Merger.cs
- PngBitmapEncoder.cs
- SudsParser.cs
- TypeLibConverter.cs
- ExpressionReplacer.cs
- PrinterSettings.cs
- ToolStripDropDownItem.cs
- ToolStripItemTextRenderEventArgs.cs
- XmlNodeList.cs
- ToolStripDropDown.cs
- TraceHandler.cs
- SqlTrackingWorkflowInstance.cs
- ErrorTableItemStyle.cs
- RequiredAttributeAttribute.cs
- DashStyles.cs
- GridViewRowPresenter.cs
- DbExpressionVisitor_TResultType.cs
- KeyedHashAlgorithm.cs
- PopupControlService.cs
- TextDecoration.cs
- KeyNotFoundException.cs
- TraceRecords.cs
- IERequestCache.cs
- PointAnimationUsingPath.cs
- LongValidator.cs
- DataGridViewSelectedCellCollection.cs
- PtsHelper.cs
- PtsPage.cs
- sqlser.cs
- EntityDataSourceViewSchema.cs
- CommandTreeTypeHelper.cs
- XmlTextReader.cs
- WhitespaceRule.cs
- FontClient.cs
- ResourceProviderFactory.cs
- URLMembershipCondition.cs
- DataGridPreparingCellForEditEventArgs.cs
- ScrollPattern.cs
- UserControlParser.cs
- UnlockInstanceAsyncResult.cs
- TouchEventArgs.cs
- Simplifier.cs
- Attribute.cs
- QueryCacheKey.cs
- IPAddress.cs
- WsdlHelpGeneratorElement.cs
- DataGridViewRowsAddedEventArgs.cs
- XmlBinaryReader.cs
- UpdatePanelControlTrigger.cs
- BrushConverter.cs
- ValidationPropertyAttribute.cs
- StylusTip.cs
- TableProvider.cs
- InstanceNameConverter.cs
- ToolboxItemCollection.cs
- TypeSchema.cs
- ParameterCollection.cs
- Errors.cs
- GacUtil.cs
- CommandHelper.cs
- HostedElements.cs
- ValueType.cs
- PaintEvent.cs
- WebPartCloseVerb.cs
- Permission.cs
- Message.cs
- ObjectViewFactory.cs
- ButtonColumn.cs
- Subtree.cs
- XmlSchemaAttributeGroupRef.cs
- InvalidContentTypeException.cs
- OracleBFile.cs
- EventSetterHandlerConverter.cs
- FormViewUpdateEventArgs.cs
- TranslateTransform.cs
- ResourcePart.cs
- QualificationDataItem.cs
- XsltSettings.cs
- Constants.cs
- DataServiceStreamResponse.cs
- BitFlagsGenerator.cs
- TransformerConfigurationWizardBase.cs
- PackageStore.cs
- ColorContextHelper.cs
- Light.cs
- ToolStripPanelRow.cs
- processwaithandle.cs
- OleDbConnectionFactory.cs
- CodeAttachEventStatement.cs
- LongValidatorAttribute.cs
- WSSecurityJan2004.cs
- ImageSourceConverter.cs
- TagPrefixAttribute.cs