Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / RowType.cs / 1305376 / RowType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.Data.Common; using System.Text; using System.Data.Objects.ELinq; using System.Threading; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Represents the Edm Row Type /// public sealed class RowType : StructuralType { private ReadOnlyMetadataCollection_properties; private readonly InitializerMetadata _initializerMetadata; #region Constructors /// /// Initializes a new instance of RowType class with the given list of members /// /// properties for this row type ///Thrown if any individual property in the passed in properties argument is null internal RowType(IEnumerableproperties) : this(properties, null) { } /// /// Initializes a RowType with the given members and initializer metadata /// internal RowType(IEnumerableproperties, InitializerMetadata initializerMetadata) : base(GetRowTypeIdentityFromProperties(CheckProperties(properties), initializerMetadata), EdmConstants.TransientNamespace, (DataSpace)(-1)) { // Initialize the properties. if (null != properties) { foreach (EdmProperty property in properties) { this.AddProperty(property); } } _initializerMetadata = initializerMetadata; // Row types are immutable, so now that we're done initializing, set it // to be read-only. SetReadOnly(); } #endregion #region Properties /// /// Gets or sets LINQ initializer Metadata for this row type. If there is no associated /// initializer type, value is null. /// internal InitializerMetadata InitializerMetadata { get { return _initializerMetadata; } } ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.RowType; } } ////// Returns the list of properties for this row type /// ////// Returns just the properties from the collection /// of members on this type /// public ReadOnlyMetadataCollectionProperties { get { Debug.Assert(IsReadOnly, "this is a wrapper around this.Members, don't call it during metadata loading, only call it after the metadata is set to readonly"); if (null == _properties) { Interlocked.CompareExchange(ref _properties, new FilteredReadOnlyMetadataCollection ( this.Members, Helper.IsEdmProperty), null); } return _properties; } } /// /// Adds a property /// /// The property to add private void AddProperty(EdmProperty property) { EntityUtil.GenericCheckArgumentNull(property, "property"); AddMember(property); } ////// Validates a EdmMember object to determine if it can be added to this type's /// Members collection. If this method returns without throwing, it is assumed /// the member is valid. /// /// The member to validate ///Thrown if the member is not a EdmProperty internal override void ValidateMemberForAdd(EdmMember member) { Debug.Assert(Helper.IsEdmProperty(member), "Only members of type Property may be added to Row types."); } ////// Calculates the row type identity that would result from /// a given set of properties. /// /// The properties that determine the row type's structure /// Metadata describing materialization of this row type ///A string that identifies the row type private static string GetRowTypeIdentityFromProperties(IEnumerableproperties, InitializerMetadata initializerMetadata) { // The row type identity is formed as follows: // "rowtype[" + a comma-separated list of property identities + "]" StringBuilder identity = new StringBuilder("rowtype["); if (null != properties) { int i = 0; // For each property, append the type name and facets. foreach (EdmProperty property in properties) { if (i > 0) { identity.Append(","); } identity.Append("("); identity.Append(property.Name); identity.Append(","); property.TypeUsage.BuildIdentity(identity); identity.Append(")"); i++; } } identity.Append("]"); if (null != initializerMetadata) { identity.Append(",").Append(initializerMetadata.Identity); } return identity.ToString(); } private static IEnumerable CheckProperties(IEnumerable properties) { if (null != properties) { int i = 0; foreach (EdmProperty prop in properties) { if (prop == null) { throw EntityUtil.CollectionParameterElementIsNull("properties"); } i++; } /* if (i < 1) { throw EntityUtil.ArgumentOutOfRange("properties"); } */ } return properties; } #endregion #region Methods /// /// EdmEquals override verifying the equivalence of all members and their type usages. /// /// ///internal override bool EdmEquals(MetadataItem item) { // short-circuit if this and other are reference equivalent if (Object.ReferenceEquals(this, item)) { return true; } // check type of item if (null == item || BuiltInTypeKind.RowType != item.BuiltInTypeKind) { return false; } RowType other = (RowType)item; // check each row type has the same number of members if (this.Members.Count != other.Members.Count) { return false; } // verify all members are equivalent for (int ordinal = 0; ordinal < this.Members.Count; ordinal++) { EdmMember thisMember = this.Members[ordinal]; EdmMember otherMember = other.Members[ordinal]; // if members are different, return false if (!thisMember.EdmEquals(otherMember) || !thisMember.TypeUsage.EdmEquals(otherMember.TypeUsage)) { return false; } } return true; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
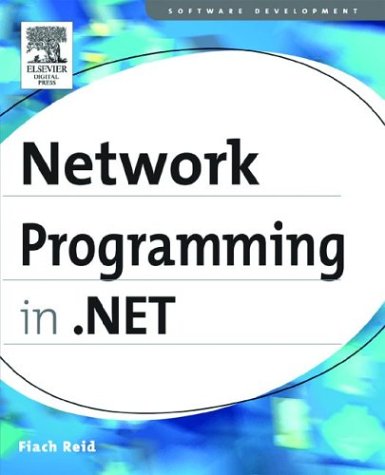
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChannelServices.cs
- CatalogZoneBase.cs
- DataGridViewTextBoxEditingControl.cs
- IItemContainerGenerator.cs
- SafeTimerHandle.cs
- Exception.cs
- Preprocessor.cs
- OleDbDataAdapter.cs
- RenderingEventArgs.cs
- ApplicationDirectory.cs
- DataControlExtensions.cs
- ReadOnlyDictionary.cs
- StructuredTypeInfo.cs
- RuntimeArgumentHandle.cs
- HelpEvent.cs
- RC2CryptoServiceProvider.cs
- HttpListenerPrefixCollection.cs
- SmtpSection.cs
- WindowsFormsSynchronizationContext.cs
- RegexWorker.cs
- DiagnosticEventProvider.cs
- Number.cs
- CodeMemberEvent.cs
- ViewPort3D.cs
- TableLayoutPanel.cs
- SettingsProviderCollection.cs
- StatusBarDrawItemEvent.cs
- IEnumerable.cs
- SemanticBasicElement.cs
- SystemMulticastIPAddressInformation.cs
- OleServicesContext.cs
- X509Certificate2Collection.cs
- ConfigXmlComment.cs
- NativeWrapper.cs
- ActivationService.cs
- TcpSocketManager.cs
- ResourceDictionary.cs
- EntryIndex.cs
- UIElement3DAutomationPeer.cs
- _NetworkingPerfCounters.cs
- NamespaceList.cs
- ImageResources.Designer.cs
- ObjectDataSourceEventArgs.cs
- SmiMetaDataProperty.cs
- ProvideValueServiceProvider.cs
- RequestChannelBinder.cs
- ContentIterators.cs
- recordstate.cs
- SafeProcessHandle.cs
- SqlUDTStorage.cs
- ListCommandEventArgs.cs
- RelationshipType.cs
- WindowsPen.cs
- ControlCommandSet.cs
- ApplicationFileCodeDomTreeGenerator.cs
- DelayedRegex.cs
- DiscreteKeyFrames.cs
- FrameworkRichTextComposition.cs
- SqlDuplicator.cs
- ClientTargetCollection.cs
- NavigateEvent.cs
- KeysConverter.cs
- Size.cs
- NaturalLanguageHyphenator.cs
- TextDocumentView.cs
- BitmapEffectInput.cs
- QuinticEase.cs
- TextTreeDeleteContentUndoUnit.cs
- TransactionFormatter.cs
- ImageDrawing.cs
- Msmq3PoisonHandler.cs
- InputLanguageCollection.cs
- DtrList.cs
- FixUp.cs
- Base64Stream.cs
- TagNameToTypeMapper.cs
- ObjectDataSourceDesigner.cs
- ChangePassword.cs
- MessageBox.cs
- DesignerTransactionCloseEvent.cs
- DataControlCommands.cs
- EffectiveValueEntry.cs
- InputDevice.cs
- UserPersonalizationStateInfo.cs
- SamlAuthorizationDecisionStatement.cs
- PhysicalOps.cs
- StyleXamlTreeBuilder.cs
- DesignerLinkAdapter.cs
- StateBag.cs
- CorrelationManager.cs
- IgnoreSection.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- AddingNewEventArgs.cs
- WebHttpDispatchOperationSelector.cs
- PackageRelationshipCollection.cs
- SafeNativeMethodsCLR.cs
- precedingsibling.cs
- MatrixKeyFrameCollection.cs
- DeclaredTypeElement.cs
- WebPartDeleteVerb.cs