Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / WebControls / DataListItem.cs / 1 / DataListItem.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Web.UI; using System.Security.Permissions; ////// [ ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class DataListItem : WebControl, IDataItemContainer { private int itemIndex; private ListItemType itemType; private object dataItem; ///Represents an item in the ///. /// public DataListItem(int itemIndex, ListItemType itemType) { this.itemIndex = itemIndex; this.itemType = itemType; } ///Initializes a new instance of the ///class. /// public virtual object DataItem { get { return dataItem; } set { dataItem = value; } } ///Represents an item in the ///. /// public virtual int ItemIndex { get { return itemIndex; } } ///Indicates the index of the item in the ///. This property is /// read-only. /// public virtual ListItemType ItemType { get { return itemType; } } ///Indicates the type of the item in the ///. /// protected override Style CreateControlStyle() { return new TableItemStyle(); } ////// /// protected override bool OnBubbleEvent(object source, EventArgs e) { if (e is CommandEventArgs) { DataListCommandEventArgs args = new DataListCommandEventArgs(this, source, (CommandEventArgs)e); RaiseBubbleEvent(this, args); return true; } return false; } ////// public virtual void RenderItem(HtmlTextWriter writer, bool extractRows, bool tableLayout) { HttpContext con = Context; if ((con != null) && con.TraceIsEnabled) { int presize = con.Response.GetBufferedLength(); RenderItemInternal(writer, extractRows, tableLayout); int postsize = con.Response.GetBufferedLength(); con.Trace.AddControlSize(UniqueID, postsize - presize); } else RenderItemInternal(writer, extractRows, tableLayout); } private void RenderItemInternal(HtmlTextWriter writer, bool extractRows, bool tableLayout) { if (extractRows == false) { if (tableLayout) { // in table mode, style information has gone on the containing TD RenderContents(writer); } else { // in non-table mode, the item itself is responsible for putting // out the style information RenderControl(writer); } } else { IEnumerator controlEnum = this.Controls.GetEnumerator(); Table templateTable = null; bool hasControls = false; while (controlEnum.MoveNext()) { hasControls = true; Control c = (Control)controlEnum.Current; if (c is Table) { templateTable = (Table)c; break; } } if (templateTable != null) { IEnumerator rowEnum = templateTable.Rows.GetEnumerator(); while (rowEnum.MoveNext()) { TableRow r = (TableRow)rowEnum.Current; r.RenderControl(writer); } } else if (hasControls) { // there was a template, since there were controls but // none of them was a table... so throw an exception here throw new HttpException(SR.GetString(SR.DataList_TemplateTableNotFound, Parent.ID, itemType.ToString())); } } } ///Displays a ///on the client. /// /// protected internal virtual void SetItemType(ListItemType itemType) { this.itemType = itemType; } ////// /// object IDataItemContainer.DataItem { get { return DataItem; } } int IDataItemContainer.DataItemIndex { get { return ItemIndex; } } int IDataItemContainer.DisplayIndex { get { return ItemIndex; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Web.UI; using System.Security.Permissions; ////// [ ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class DataListItem : WebControl, IDataItemContainer { private int itemIndex; private ListItemType itemType; private object dataItem; ///Represents an item in the ///. /// public DataListItem(int itemIndex, ListItemType itemType) { this.itemIndex = itemIndex; this.itemType = itemType; } ///Initializes a new instance of the ///class. /// public virtual object DataItem { get { return dataItem; } set { dataItem = value; } } ///Represents an item in the ///. /// public virtual int ItemIndex { get { return itemIndex; } } ///Indicates the index of the item in the ///. This property is /// read-only. /// public virtual ListItemType ItemType { get { return itemType; } } ///Indicates the type of the item in the ///. /// protected override Style CreateControlStyle() { return new TableItemStyle(); } ////// /// protected override bool OnBubbleEvent(object source, EventArgs e) { if (e is CommandEventArgs) { DataListCommandEventArgs args = new DataListCommandEventArgs(this, source, (CommandEventArgs)e); RaiseBubbleEvent(this, args); return true; } return false; } ////// public virtual void RenderItem(HtmlTextWriter writer, bool extractRows, bool tableLayout) { HttpContext con = Context; if ((con != null) && con.TraceIsEnabled) { int presize = con.Response.GetBufferedLength(); RenderItemInternal(writer, extractRows, tableLayout); int postsize = con.Response.GetBufferedLength(); con.Trace.AddControlSize(UniqueID, postsize - presize); } else RenderItemInternal(writer, extractRows, tableLayout); } private void RenderItemInternal(HtmlTextWriter writer, bool extractRows, bool tableLayout) { if (extractRows == false) { if (tableLayout) { // in table mode, style information has gone on the containing TD RenderContents(writer); } else { // in non-table mode, the item itself is responsible for putting // out the style information RenderControl(writer); } } else { IEnumerator controlEnum = this.Controls.GetEnumerator(); Table templateTable = null; bool hasControls = false; while (controlEnum.MoveNext()) { hasControls = true; Control c = (Control)controlEnum.Current; if (c is Table) { templateTable = (Table)c; break; } } if (templateTable != null) { IEnumerator rowEnum = templateTable.Rows.GetEnumerator(); while (rowEnum.MoveNext()) { TableRow r = (TableRow)rowEnum.Current; r.RenderControl(writer); } } else if (hasControls) { // there was a template, since there were controls but // none of them was a table... so throw an exception here throw new HttpException(SR.GetString(SR.DataList_TemplateTableNotFound, Parent.ID, itemType.ToString())); } } } ///Displays a ///on the client. /// /// protected internal virtual void SetItemType(ListItemType itemType) { this.itemType = itemType; } ////// /// object IDataItemContainer.DataItem { get { return DataItem; } } int IDataItemContainer.DataItemIndex { get { return ItemIndex; } } int IDataItemContainer.DisplayIndex { get { return ItemIndex; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
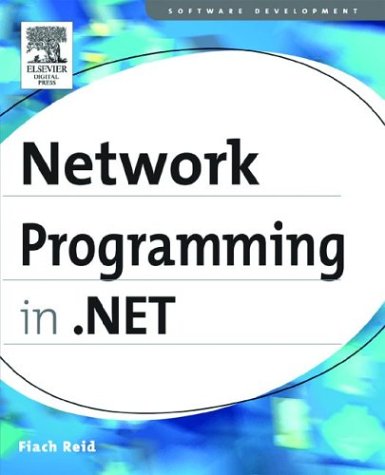
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TabControl.cs
- FileDialog.cs
- HitTestParameters.cs
- HtmlButton.cs
- TrackingServices.cs
- ComboBoxRenderer.cs
- CodeParameterDeclarationExpression.cs
- RemoteCryptoRsaServiceProvider.cs
- MaskedTextProvider.cs
- DataGridTextBoxColumn.cs
- RuntimeCompatibilityAttribute.cs
- StoreAnnotationsMap.cs
- SecuritySessionFilter.cs
- TreeNodeBinding.cs
- JsonReader.cs
- IdentityReference.cs
- PlatformNotSupportedException.cs
- TextAction.cs
- ClassDataContract.cs
- MissingManifestResourceException.cs
- CodeGotoStatement.cs
- PermissionSetTriple.cs
- DataGridViewSortCompareEventArgs.cs
- FixedBufferAttribute.cs
- CacheRequest.cs
- KnownBoxes.cs
- HtmlInputCheckBox.cs
- SafeNativeHandle.cs
- IdentityHolder.cs
- ValueUnavailableException.cs
- LingerOption.cs
- BigInt.cs
- Pair.cs
- ExpandCollapseProviderWrapper.cs
- ContextMarshalException.cs
- Animatable.cs
- UserControlCodeDomTreeGenerator.cs
- TreeNodeCollection.cs
- NativeActivityMetadata.cs
- TraceLog.cs
- WeakReadOnlyCollection.cs
- WebPartActionVerb.cs
- Utility.cs
- HttpBrowserCapabilitiesWrapper.cs
- LoginName.cs
- SubpageParaClient.cs
- DynamicILGenerator.cs
- Missing.cs
- QilUnary.cs
- QuaternionRotation3D.cs
- SamlAudienceRestrictionCondition.cs
- ContextDataSource.cs
- AbandonedMutexException.cs
- SchemaImporterExtensionElement.cs
- Animatable.cs
- SimpleApplicationHost.cs
- EventManager.cs
- PriorityItem.cs
- Enum.cs
- ListSortDescription.cs
- FilterQuery.cs
- CryptoKeySecurity.cs
- Mutex.cs
- MultipartContentParser.cs
- CodeVariableReferenceExpression.cs
- SymbolType.cs
- XsltFunctions.cs
- ISAPIRuntime.cs
- UnsafeNativeMethods.cs
- SAPIEngineTypes.cs
- FolderLevelBuildProviderAppliesToAttribute.cs
- TerminatorSinks.cs
- SystemIcmpV6Statistics.cs
- WebBrowser.cs
- SettingsAttributes.cs
- FileClassifier.cs
- ScrollItemPattern.cs
- ConditionalDesigner.cs
- Delay.cs
- MsmqChannelFactory.cs
- FamilyMapCollection.cs
- PropertyDescriptorCollection.cs
- FieldToken.cs
- ACE.cs
- ControlCachePolicy.cs
- SynchronizedDispatch.cs
- TableTextElementCollectionInternal.cs
- RSAOAEPKeyExchangeDeformatter.cs
- NullableDoubleAverageAggregationOperator.cs
- ContentPlaceHolder.cs
- ListViewHitTestInfo.cs
- Rijndael.cs
- RegistryPermission.cs
- DeclarationUpdate.cs
- ImageSource.cs
- HandledEventArgs.cs
- DesignerAdapterUtil.cs
- SharedDp.cs
- SchemaNamespaceManager.cs
- IItemProperties.cs