Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DLinq / Dlinq / SqlClient / Common / SqlNodeTypeOperators.cs / 1 / SqlNodeTypeOperators.cs
using System; using System.Collections.Generic; using System.Text; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq.SqlClient { internal static class SqlNodeTypeOperators { ////// Determines whether the given unary operator node type returns a value that /// is predicate. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateUnaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: return true; case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Covar: case SqlNodeType.Convert: case SqlNodeType.Cast: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given unary operator expects a predicate as input. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsUnaryOperatorExpectingPredicateOperand(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: return true; case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Covar: case SqlNodeType.Convert: case SqlNodeType.Cast: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateBinaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether this operator is a binary comparison operator (i.e. >, =>, ==, etc) /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification = "These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsComparisonOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: return true; case SqlNodeType.And: case SqlNodeType.Or: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsBinaryOperatorExpectingPredicateOperands(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.EQ: case SqlNodeType.EQ2V: case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.NE: case SqlNodeType.NE2V: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given node requires support on the client for evaluation. /// For example, LINK nodes may be delay-executed only when the user requests the result. /// internal static bool IsClientAidedExpression(this SqlExpression expr) { switch (expr.NodeType) { case SqlNodeType.Link: case SqlNodeType.Element: case SqlNodeType.Multiset: case SqlNodeType.ClientQuery: case SqlNodeType.TypeCase: case SqlNodeType.New: return true; default: return false; }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Text; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq.SqlClient { internal static class SqlNodeTypeOperators { ////// Determines whether the given unary operator node type returns a value that /// is predicate. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateUnaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: return true; case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Covar: case SqlNodeType.Convert: case SqlNodeType.Cast: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given unary operator expects a predicate as input. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsUnaryOperatorExpectingPredicateOperand(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: return true; case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Covar: case SqlNodeType.Convert: case SqlNodeType.Cast: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateBinaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether this operator is a binary comparison operator (i.e. >, =>, ==, etc) /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification = "These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsComparisonOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: return true; case SqlNodeType.And: case SqlNodeType.Or: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsBinaryOperatorExpectingPredicateOperands(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.EQ: case SqlNodeType.EQ2V: case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.NE: case SqlNodeType.NE2V: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given node requires support on the client for evaluation. /// For example, LINK nodes may be delay-executed only when the user requests the result. /// internal static bool IsClientAidedExpression(this SqlExpression expr) { switch (expr.NodeType) { case SqlNodeType.Link: case SqlNodeType.Element: case SqlNodeType.Multiset: case SqlNodeType.ClientQuery: case SqlNodeType.TypeCase: case SqlNodeType.New: return true; default: return false; }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
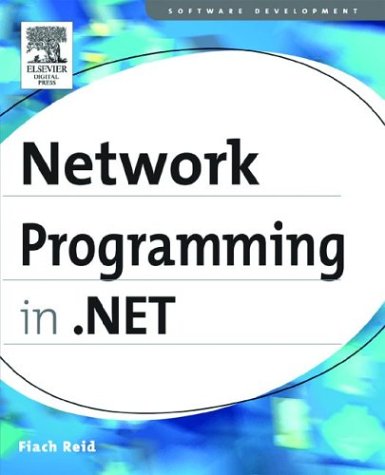
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OdbcEnvironment.cs
- ShapeTypeface.cs
- AstNode.cs
- AdCreatedEventArgs.cs
- SQLInt64Storage.cs
- MembershipSection.cs
- MessageQueuePermissionAttribute.cs
- CollectionBuilder.cs
- BitmapCacheBrush.cs
- ValidatorCollection.cs
- StringBlob.cs
- PeerNameRecord.cs
- DispatcherObject.cs
- XmlSchemaProviderAttribute.cs
- XXXOnTypeBuilderInstantiation.cs
- ThrowHelper.cs
- HostSecurityManager.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- ObjectDataSourceView.cs
- SoapInteropTypes.cs
- WebPartCloseVerb.cs
- DbProviderConfigurationHandler.cs
- InternalMappingException.cs
- FontFamilyConverter.cs
- GeneralTransformGroup.cs
- ClientConfigPaths.cs
- Label.cs
- CheckBoxList.cs
- Rijndael.cs
- DebugView.cs
- Cursors.cs
- AnnotationResourceCollection.cs
- TableLayoutPanelCellPosition.cs
- UniqueIdentifierService.cs
- CngKeyCreationParameters.cs
- QilExpression.cs
- recordstate.cs
- Control.cs
- ToolStripSeparatorRenderEventArgs.cs
- TextEditorLists.cs
- XmlText.cs
- MetadataCache.cs
- DeferredBinaryDeserializerExtension.cs
- EditorAttributeInfo.cs
- FileDialog.cs
- BigInt.cs
- RichTextBoxConstants.cs
- ZipIOCentralDirectoryBlock.cs
- SafeEventHandle.cs
- CodeAttributeDeclarationCollection.cs
- Encoder.cs
- ClaimTypeElementCollection.cs
- XmlJsonWriter.cs
- WebPartZoneCollection.cs
- XmlNullResolver.cs
- XmlEventCache.cs
- TimeSpanStorage.cs
- CodeNamespace.cs
- EntityContainer.cs
- TableChangeProcessor.cs
- ReadOnlyAttribute.cs
- QueryExtender.cs
- PolyBezierSegment.cs
- SqlNotificationRequest.cs
- PartialTrustVisibleAssembly.cs
- SmiEventStream.cs
- IndentTextWriter.cs
- datacache.cs
- TransformedBitmap.cs
- InputReport.cs
- PageThemeBuildProvider.cs
- InstanceCompleteException.cs
- ChildChangedEventArgs.cs
- WebPartConnectionsCloseVerb.cs
- Buffer.cs
- TemplateField.cs
- MissingSatelliteAssemblyException.cs
- BitmapEffectDrawingContent.cs
- MetadataException.cs
- remotingproxy.cs
- XmlSchemaSet.cs
- CorrelationScope.cs
- StringArrayConverter.cs
- PackWebRequestFactory.cs
- RecommendedAsConfigurableAttribute.cs
- CompModSwitches.cs
- InputScope.cs
- BaseServiceProvider.cs
- shaperfactory.cs
- BooleanConverter.cs
- StrokeCollectionDefaultValueFactory.cs
- MulticastNotSupportedException.cs
- XmlParser.cs
- FlatButtonAppearance.cs
- FilteredAttributeCollection.cs
- SqlDataAdapter.cs
- DBBindings.cs
- SqlCacheDependency.cs
- HistoryEventArgs.cs
- EdmFunction.cs